30 Javascript Multidimensional Array Example
For example, list of students: var student = [ [ "John", 25, "male"], [ "Steve", 21, "male"], [ "Angela", 22, "female"]] Jagged Array: Jagged is almost the same as a multidimensional array but with a subtle difference in the number of elements in the sub-arrays within an array. The multidimensional Array in which the ... It creates an n-dimensional array view wrapping an underlying storage type. data is a 1D array storage. It is either an instance of Array, a typed array, or an object that implements get(), set(), .length; shape is the shape of the view (Default: data.length) stride is the resulting stride of the new array. (Default: row major)
Introduction To 2d Array 2 Dimensional Array In Javascript
Working of JavaScript Arrays. In JavaScript, an array is an object. And, the indices of arrays are objects keys. Since arrays are objects, the array elements are stored by reference. Hence, when an array value is copied, any change in the copied array will also reflect in the original array. For example,
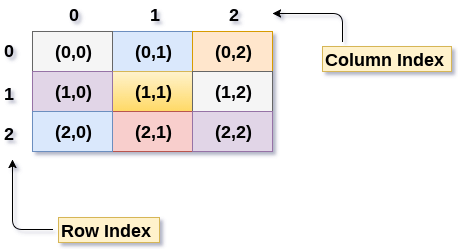
Javascript multidimensional array example. Oct 11, 2019 - In JavaScript, multidimensional arrays are just an array nested in another array. So, to declare a multidimensional array, we can use the same declaration methods as above, except we replace the entities inside with arrays. For example, we can write: Can anyone give me a sample/example of JavaScript with a multidimensional array of inputs? Hope you could help because I'm still new to the JavaScript. Like when you input 2 rows and 2 columns the output of it will be 2 rows of input and 2 columns of input. Like this: [input][input] [input][input] JavaScript multidimensional arrays defined and demonstrated. How to access elements, add and remove elements, and iterate over multidimensional arrays.
Nov 12, 2011 - Can anyone give me a sample/example of JavaScript with a multidimensional array of inputs? Hope you could help because I'm still new to the JavaScript. Like when you input 2 rows and 2 columns the However, we can create a multidimensional array in JavaScript by making an array of arrays i.e. the array will be consisting of other arrays as elements. The easiest way to define a Multi-Dimensional Array in JavaScript is to use the array literal notation. Below examples will create a 2-dimensional array person. var Employee = Here, JavaScript introduces the concept of a multidimensional array. Basically, it allows us to create a nested array or in simple words, arrays inside an array. Today, I'll explain the usage of JavaScript multidimensional array with the help of step by step examples.
26/6/2018 · Basically, multi-dimension arrays are used if you want to put arrays inside an array. Let's take an example. Say you wanted to store every 6 hour's temperature for every weekday. You could do something like − Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The two-dimensional array is a set of items sharing the same name. The two-dimensional array is an array of arrays, that is to say, to create an array of one-dimensional array objects. They are arranged as a matrix in the form of rows and columns. JavaScript suggests some methods of creating two-dimensional arrays.
Using push to add elements to multidimensional array. To summarize, multidimensional arrays are Javascript array of arrays created by using Javascript For Loop and simple arrays in Javascript. Here is our first Javascript code example which can be used to define multi dimensional Javascript array. Our first Javascript tutorial example will create two dimensional array (10,20) for a minesweeper board. Access Items Of Multidimensional Array. How to access items of a multidimensional array. Let's we will explain. Use the Square brackets of access to array items as following below example. document.write (arr [1] [1]); // output. // mysql. The JavaScript array index starts with zero. The first bracket refers to the desired item in the outer ...
Iterate multi dimensional array; Print and display array of arrays; Multi dimensional array examples; one and two dimensional arrays; Javascript has no multi dimensional arrays synax inbuilt. But you can create a array of arrays. where each array element is an array of values. Multi dimensional arrays can be 2 by 2 called matrix or 2 by 3. How ... Simple Javascript Nested Array Examples - Create Push Pop Loop Check. By W.S. Toh / Tips & Tutorials - Javascript / May 26, 2021 May 26, 2021. Welcome to a quick tutorial on the nested array in Javascript. So you have finally met the worthy opponent called the multidimensional array, and this "array in an array" thing sure is confusing ... Lesson Code: http://www.developphp /video/JavaScript/Multidimensional-Array-Programming-Tutorial Learn to write and process multidimensional arrays using ...
Create a Multidimensional Array. Here is how you can create multidimensional arrays in JavaScript. Example 1. let studentsData = [['Jack', 24], ['Sara', 23], ['Peter', 24]]; Example 2. let student1 = ['Jack', 24]; let student2 = ['Sara', 23]; let student3 = ['Peter', 24]; // multidimensional array … A JavaScript multi-dimensional array is an array of arrays. The container array for those one-dimensional arrays will be used and manipulated as multidimensional array. There are two different ways to create it which we will be illustrating in this article later on, however, the most common approach is known as Array Literals. The two-dimensional array is a collection of items which share a common name and they are organized as a matrix in the form of rows and columns. The two-dimensional array is an array of arrays, so we create an array of one-dimensional array objects. The following program shows how to create an 2D array : Example-1:
Output: javascript- array -join-example. Code language: PHP (php) How it works: First, split the title string by the space into an array by using the split () string method. Second, concatenate all elements in the result array into a string by using the join () method. Third, convert the result string to lower case by using the toLowerCase ... Arrays-of-Arrays. Perhaps the most popular way to represent higher dimensional arrays in JavaScript is as arrays-of-arrays. For example, var A = [ [1, 0, 0], [0, 1, 0], [0, 0, 1]] This is the approach that numeric.js uses, and it has some merits. For example, the syntax for accessing elements in an array-of-arrays is quite pleasingly simple: JS Multidimensional Array. Multidimensional array has more than 1 dimension, such as 2 dimensional array, 3 dimensional array etc. Define a dimensional array once at initialization. var arr = [ [2,4], [20,40], [200,400] ]; arr[1][1]; //40 Define a dimensional array dynamically.
Be aware that the sort method operates on the array in place. It changes the array. Most other array methods return a new array, leaving the original one intact. This is especially important to note if you use a functional programming style and expect functions to not have side-effects. An array is a variable type in any programming language used to store multiple values simultaneously. Arrays come in helpful when there is a need to store several different values in a single variable. Arrays application is seen at many places, like implementing the matrices, data structures or storing data in tabular form. In this article, JavaScript Arrays are explained with examples. The following example shows you how a two-dimensional array can be produced in JavaScript. You can enter a value in the box labelled 'Person ID', which is the first number of a two-dimensional array, and then select one of two numbers in the Name/Profession box.
Since arrays are a particular kind of objects, they can store JavaScript objects, functions, and even other arrays as their content. Take a look at the example below to see a JavaScript array containing an object, a function, and an array: Example. Array [ 0] = Date .now; Array [ 1] = myFunction; Array [ 2] = myPhones; Copyright © 1999-2011, JavaScripter Above is a very basic example of a multi-dimensional array, only going deep by one level - basically you group values into [ ] brackets to define an array within the array, say you wanted to get "grouped" from the above example, it is located at index 1 of the main array, and index 1 of the inner array - so you would access it as follows:
We can sort a multidimensional array ascending and descending order using JavaScript and modern JavaScript.In both ways we use .sort method. Related posts: JavaScript sorting array detail analysis Jul 20, 2021 - This chapter introduces collections of data which are ordered by an index value. This includes arrays and array-like constructs such as Array objects and TypedArray objects. Mar 09, 2019 - You now know how to create and use nested arrays in JavaScript. You’ll find nested arrays are useful when you want to store highly structured data — such as our animals example above — and when you’re creating multidimensional data structures (commonly used in games and graphics ...
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: So multidimensional arrays in JavaScript is known as arrays inside another array. We need to put some arrays inside an array, then the total thing is working like a multidimensional array. The array, in which the other arrays are going to insert, that array is use as the multidimensional array in our code. To define a multidimensional array its ... Not the answer you're looking for? Browse other questions tagged javascript arrays multidimensional-array or ask your own question.
An array inside an array is called a multidimensional or two dimensional array. 0:33. That might sound a bit strange and confusing right now, but you can start to 0:38. think of a multidimensional array as a list containing other lists. 0:43. You can picture a two-dimensional array as a spreadsheet. 0:46. Think of the spreadsheet as the master ... The call to new Array(number) creates an array with the given length, but without elements. The length property is the array length or, to be precise, its last numeric index plus one. It is auto-adjusted by array methods. If we shorten length manually, the array is truncated. We can use an array as a deque with the following operations: Adding elements to the JavaScript multidimensional array. You can use the Array methods such as push () and splice () to manipulate elements of a multidimensional array. For example, to add a new element at the end of the multidimensional array, you use the push () method as follows: activities.push ( ['Study',2] ); console.table ( activities );
Array.from() Creates a new Array instance from an array-like or iterable object.. Array.isArray() Returns true if the argument is an array, or false otherwise.. Array.of() Creates a new Array instance with a variable number of arguments, regardless of number or type of the arguments. Feb 09, 2020 - Some languages, C#, Java and even Visual Basic, to name a few, let one declare multi-dimensional arrays. But not JavaScript. In JavaScript you have to build them. A two-dimensional array is a… 6/7/2018 · A multidimensional array is nothing but an array of arrays. If we take forward our example, each row will represent a day while each entry in the row will represent a temp entry. For example, let temps = [ [35, 28, 29, 31], [33, 24, 25, 29] ]; You can chain array access. For example, if you want the 3rd element in the second row, you could just query for temps[1][2]. Note that the order is rows than columns.
Java Multidimensional Array 2d And 3d Array
Javascript Functions And Arrays Ppt Download
Implementing Multidimensional Arrays In Javascript 0 Fps
Php 5 Multidimensional Arrays Code Bridge Plus
Multidimensional Array In Java Operations On
An Introduction To Multidimensional Arrays In Javascript
Multidimensional Arrays In Java Geeksforgeeks
Java Swap Rows And Columns Clockwise In Two Dimensional Array
How To Convert A Two Dimensional Array To One Dimensional
Multi Dimensional Arrays In C Programming Definition Amp Example Video
Difference Between A Multi Dimensional Array And Nested Array
How Can I Create A Two Dimensional Array In Javascript
Transpose A Two Dimensional 2d Array In Javascript
Javascript Multidimensional Array Push Code Example
Multidimensional Or Arrays Of Arrays In Java
What Is Two Dimensional Array Quora
Filter Multidimensional Array Javascript Code Example
Javascript Array Splice Delete Insert And Replace
Multidimensional Arrays Matlab Amp Simulink
2d Arrays In Javascript Experts Exchange
Tree View For Arrays And Objects In Javascript By Manish
How To Flattening Multidimensional Arrays In Javascript
Github Scijs Ndarray Multidimensional Arrays For Javascript
Insert A Multidimensional Array Into A Multidimensional Array
Console Log A Multi Dimensional Array Stack Overflow
An Introduction To Multidimensional Arrays In Javascript
0 Response to "30 Javascript Multidimensional Array Example"
Post a Comment