35 Javascript Compare To Undefined
Jul 20, 2021 - Note: The strict equality operator (as opposed to the standard equality operator) must be used here, because x == undefined also checks whether x is null, while strict equality doesn't. This is because null is not equivalent to undefined. See comparison operators for details. The best way to compare value is the undefined value or not in JavaScript is by using typeof keyword. Note: null is assigned by a program explicitly, whereas undefined is the value assigned by the JavaScript machine internally.
7 Tips To Handle Undefined In Javascript
Jun 15, 2021 - In JavaScript, null is a primitive value that is used to signify the intentional absence of an object value, whereas undefined is a primitive value that acts as a placeholder for a variable that has not been assigned a value. Null and undefined values are equal when compared using the JavaScript ...
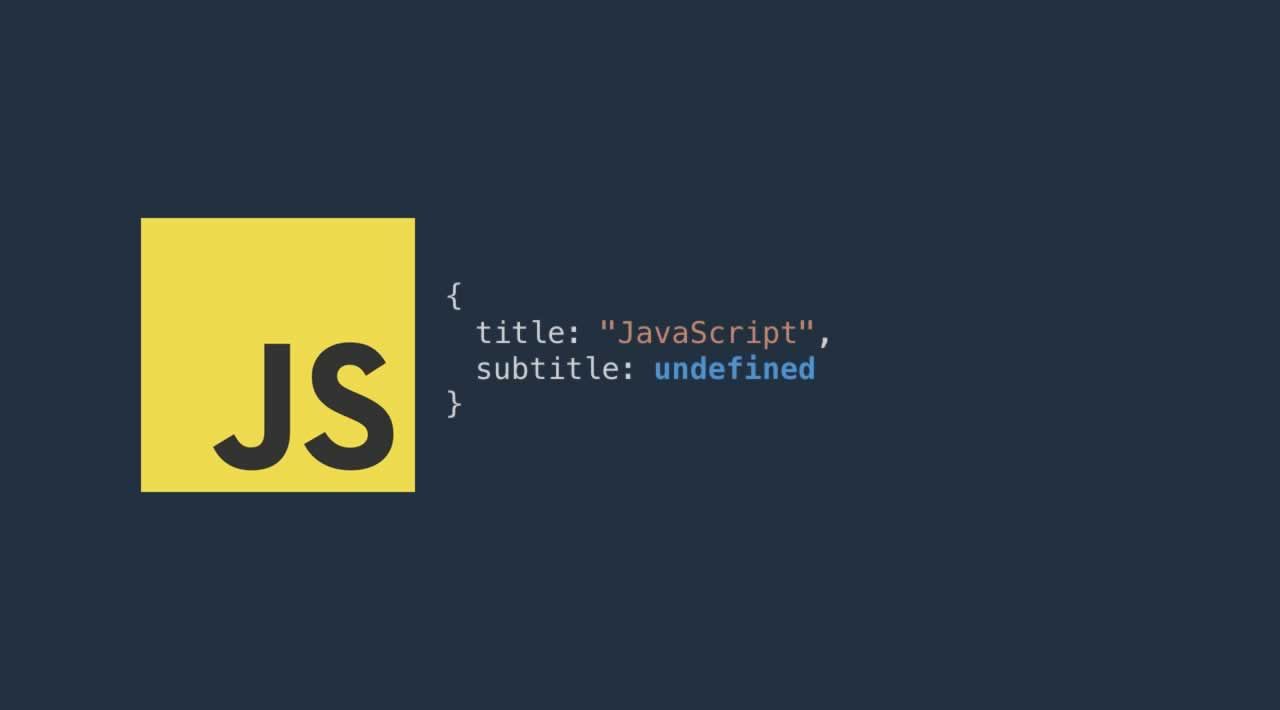
Javascript compare to undefined. 16/9/2015 · How do you check if a value is undefined in JavaScript? The short answer. In modern browsers you can safely compare the variable directly to undefined: if (name === undefined) {...} Some people argue against comparing with undefined because old browsers allowed its value to be re-assigned like this: undefined = "test" Comparing if something is null or undefined is a trivial task but can take different color depending to whom you talk. This article will try to make it simple to understand. Since TypeScript is built on top of JavaScript, it has to handle the craziness of how JavaScript compare. executes the same whether you compare the name variable to null or to undefined, as long as the abstract equality operator is used. Additionally, null is treated as falsy in Boolean operations ...
Aug 02, 2020 - It’s short, expressive and no direct comparisons with undefined. Adding a default value to parameter b = 2 looks better: ... ES2015 default parameters feature is intuitive and expressive. Always use it to set default values for optional parameters. ... Implicitly, without return statement, a JavaScript ... Since the null and undefined values are both equal with these operators, errors can occur when a missing object property is used in a null-comparison. By using the === and !== operators, no implicit type conversion will be done. The values null and undefined are not equal using these operators. When comparing a string with a number, JavaScript will convert the string to a number when doing the comparison. An empty string converts to 0. A non-numeric string converts to NaN which is always false. When comparing two strings, "2" will be greater than "12", because (alphabetically) 1 is less than 2.
Many programming languages have one “non-value” called null. It indicates that a variable does not currently point to an object – for example, when it hasn’t been initialized yet. In contrast, JavaScript has two of them: undefined and null. 28/11/2010 · Simple enough, you have a variable that is not defined, but it DOES exist, and you are comparing it against a global variable “undefined’, that is not defined but also exists, and ultimately, can be broken and not give what you expect. Take this example: var a, undefined = "defined!"; alert(a === undefined); // woah, not what we expected Dictionary comparison, hence false. "a" is smaller than "p". Again, dictionary comparison, first char "2" is greater than the first char "1". Values null and undefined equal each other only. Strict equality is strict. Different types from both sides lead to false. Similar to (4), null only equals undefined. Strict equality of different types.
JavaScript has two visually similar, but very different ways to test equality: == (Double equals operator): the equality or abstract comparison operator. === (Triple equals operator): the identity or strict comparison operator. Here are the differences between == and ===: before showing comparison == converts the variable values of the same type; If both operands are null or both operands are undefined, return true. If either operand is NaN, return false. Otherwise, compare the two operand's values: Numbers must have the same numeric values. +0 and -0 are considered to be the same value. 16/1/2018 · Similarities between null and undefined. In JavaScript there are only six falsy values. Both null and undefined are two of the six falsy values. Here’s a full list: false; 0 (zero) “” (empty string) null; undefined; NaN (Not A Number) Any other value in JavaScript is considered truthy.
Three most accurate ways to compare strings correctly in JavaScript. The == operator has its limitations here because Java is not designed to support it. Why the == operator doesn't work in JavaScript. The explanation is simple; Java wasn't built to work with == as an option to compare strings. Null vs. Undefined ... Fact is you will need to deal with both. Interestingly in JavaScript with == May 26, 2018 - In a JavaScript program, the correct way to check if an object property is undefined is to use the `typeof` operator. See how you can use it with this simple explanation
JavaScript provides 3 ways to compare values: The strict equality operator ===. The loose equality operator ==. Object.is () function. When comparing objects using any of the above, the comparison evaluates to true only if the compared values reference the same object instance. This is the referential equality. Get code examples like "javascript compare string to undefined" instantly right from your google search results with the Grepper Chrome Extension. All of us use "==" daily for performing the comparison in JavaScript to execute particular code block only when certain condition meets. But do you know how "==" actually works in JavaScript? In JavaScript "==" compare number values instead of boolean values by performing implicit coercion. Here is a simple example :
Oct 04, 2019 - Hey there people One of the most common errors we encounter in JavaScript is the undefined error whe... C hecking for null is a common task that every JavaScript developer has to perform at some point or another. The typeof keyword returns "object" for null, so that means a little bit more effort is required. Comparisons can be made: null === null to check strictly for null or null == undefined to check loosely for either null or undefined. Nov 12, 2018 - When you declare a variable but don’t declare its value, JavaScript assigns “undefined” value to it. ... NaN(you can just trust me with this one or check it out on your own 🙃). Similar as with null, negating undefined gives true, but comparing it to either true or false gives false.
The short answer In modern browsers you can easily compare the variable with undefined if (name === undefined) {...} Some people disagree with comparing undefined to define because the old browsers made it possible to assign its value again like this. variable === undefined typeof variable === "undefined" Here the assigned variables don't have any value but the variable exists. Here the type of variable is undefined. If you assigned a value(var geeks === undefined ) it will show, if not it will also show undefined but in different meaning. Here the undefined is the typeof undefined. Comparing null and undefined. In JavaScript, null equals undefined. It means that the following expression returns true. console.log(null == undefined); // true. Code language: JavaScript (javascript) Comparing NaN with other values. If either operand is NaN, then the equal operator(==) returns false.
10/8/2020 · JavaScript check undefined To check undefined in JavaScript, use (===) triple equals operator. In modern browsers, you can compare the variable directly to undefined using the triple equals operator. There are also two more ways to check if the variable is undefined or not in JavaScript, but those ways are not recommended. 2 weeks ago - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Jul 22, 2019 - Une erreur JavaScript fatale s'est produite. Faut-il envoyer un rapport d'erreurs ... No matching version found for swagger-jsdoc@^6.0.0. ... ReactElement Function lacks ending return statement and return type does not include 'undefined'.
5/5/2010 · !undefined is true in javascript, so if you want to know whether your variable or object is undefined and want to take actions, you could do something like this: if(<object or variable>) { //take actions if object is not undefined } else { //take actions if object is undefined } The expression comparing objects is true only if the operands refer to the same object. Null and undefined are strictly equal to themselves and abstractly equal to each other. JavaScript comparison operator Equality (==) The equal or weak operator converts the operands during the comparison (temporarily). null and undefined are in equal in JavaScript because. they are both primitives; both null and undefined are values but with not much meaning and; null is used to represent the intentional absence of some object value whereas undefined represents a variable with no value. To test for equality in JavaScript, we can use the double equal sign ==.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Now, the long version (which I originally penned in response to some flawed advice I found online about how to compare numbers in JavaScript). The problem, and a flawed approach to solving it Take this ("bad") code, which addresses the classic floating point problem of (0.1 + 0.2) == 0.3 returning false: Apr 19, 2013 - > typeof iDontKnowThisVariable ... === undefined ReferenceError: iDontKnowThisVariable is not defined ... or via falsiness. It is normally more important for code to be easy to understand than to be completely safe. Therefore, checking via ... Please enable JavaScript to view the ...
In JavaScript if a variable has been declared, but has not been assigned a value, is automatically assigned the value undefined. Therefore, if you try to display the value of such variable, the word "undefined" will be displayed. Whereas, the null is a special assignment value, which can be assigned to a variable as a representation of no value. 6 days ago - After conversions (one or both sides may undergo conversions), the final equality comparison is performed exactly as === performs it. Loose equality is symmetric: A == B always has identical semantics to B == A for any values of A and B (except for the order of applied conversions). undefined and ... Undefined is also a primitive value in JavaScript. A variable or an object has an undefined value when no value is assigned before using it. So you can say that undefined means lack of value or unknown value.
12/4/2021 · So when it comes to comparing null, undefined, and "": null == undefined in the sense that they both have a “nothing” value. null !== undefined because one is an object while the other is undefined. The empty string is the “odd one” that will not match with null and undefined.
Determine If Variable Is Undefined Or Null
Javascript Boolean Grasp All Its Concepts With A Single
Javascript Lesson 25 Difference Between Arrays And Objects
Is There A Standard Function To Check For Null Undefined Or
Javascript Classes State Management V2 Full Stack Feed
Assertionerror Expected To Deeply Equal Help Postman
Javascript Null Vs Undefined Understanding The Difference
Understanding Null Undefined And Nan By Kuba Michalski
How To Check If An Object Has A Specific Property In Javascript
What S The Difference Between Null And Undefined In Javascript
Understanding Null Undefined And Nan By Kuba Michalski
Understanding Null Undefined And Nan By Kuba Michalski
Typescript Comparison Of Null And Undefined Patrick
Let S Compare Javascript Testing Frameworks Van Wilson S Site
Values Javascript For Impatient Programmers Es2021 Edition
Convert Undefined To String With String In Javascript
Comparison Between Uninitialized Arrays Fail Issue 62
Check If Undefined Javascript Es6 Code Example
Compare Two Dates With Javascript Stack Overflow
Should I Use Or Equality Comparison Operator In
What S The Difference Between Null And Undefined In Javascript
Everything About Javascript Objects By Deepak Gupta
Gtmtips Check For Undefined Value Simo Ahava S Blog
How To Check If A Javascript Object Property Is Undefined
How To Check If An Object Has A Specific Property In
Why Inner Loop Return Undefined Javascript The
How To Check For An Undefined Or Null Variable In Javascript
Truthy And Falsy When All Is Not Equal In Javascript Sitepoint
Javascript Checking For Null Vs Undefined And Difference
How To Compare Arrays In Javascript Efficiently Dev Community
Javascript Undefined Property Geeksforgeeks
Jswatchdog Javascript Code Checker Kintone Developer Program
A Perfect Guide For Cracking A Javascript Interview A
And Operators Of Javascript In Simple Words Dhananjay Kumar
0 Response to "35 Javascript Compare To Undefined"
Post a Comment