21 How To Parse A Date In Javascript
Jul 20, 2018 - The best string format for string parsing is the date ISO format together with the JavaScript Date object constructor. Examples of ISO format: YYYY-MM-DD or YYYY-MM-DDTHH:MM:SS. But wait! Just using the "ISO format" doesn't work reliably by itself. String are sometimes parsed as UTC and sometimes ... First declare variable time and store the milliseconds of current date using new date () for current date and getTime () Method for return it in milliseconds since 1 January 1970. Convert time into date object and store it into new variable date. Convert the date object's contents into a string using date.toString () function
Is There Any Workaround For Broken V8 Date Parser Stack
JavaScript - How to Use Date.parse in JavaScript The Date.parse () method can help while dealing with calculations including dates. These calculations are far easier, more accurate, and written more logically when the underlying date is represented as a Number rather than a string.
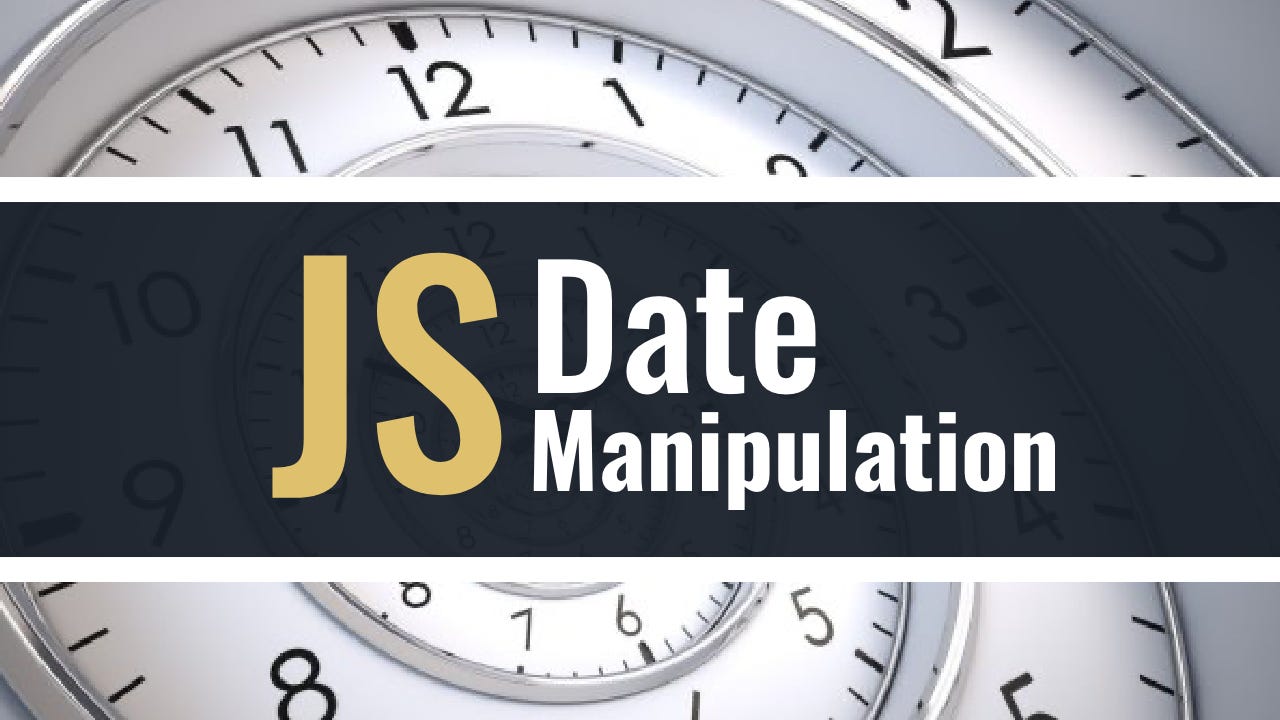
How to parse a date in javascript. Define two date variables, i.e., date1 and date2; Initialize the date1 variables by creating the date objects using new Date(), which will take system date by default. Initialize the date2 variables by creating the date objects using new Date() and provide a date in it. Now use the Math.abs() method to calculate the total seconds between two dates. Date libraries help in many ways to make your life easier. They greatly simplify date parsing, date arithmetic and logical operations, and date formatting. You can find a reliable date library for both the front end and the back end to do most of the heavy lifting for you. Mar 24, 2015 - Some browsers will try to guess the format. Some will return NaN. ... JavaScript Long Dates. Long dates are most often written with a "MMM DD YYYY" syntax like this: ... Commas are ignored. Names are case insensitive: ... If you have a valid date string, you can use the Date.parse() method ...
date validation in javascript - Validate Date Using Regular Expressions, the moment.js Library and Date.parse() Method in JavaScript Example with demo. How to check a date is valid or not using JavaScript? A JavaScript function is used to check a valid date format against a regular expression. Parsing a date from a string with Moment.js is easy, and the library accepts strings in the ISO 8601 or RFC 2822 Date Time format, along with any string accepted by the JavaScript Date object. ISO 8601 strings are recommended since it is a widely accepted format. @Duke— new Date(Date.parse('02.02.1999')) will produce identical results to new Date('02.02.1999'), including Invalid Date in some browsers. Using the built-in parser is arguably the worst way to parse a timestamp. - RobG Aug 7 '19 at 1:04
Mar 03, 2021 - Date Parsing purpose is whenever we get data from the third party, we don’t know that the given date in their documents is date object or date String object. So, we must perform parsing to do required actions like the comparison of dates, subtraction of dates, etc. In JavaScript, the default ... Learn how to work with the Dates in JavaScript. JavaScript provides Date object to work with date & time including days, months, years, hours, minutes, seconds and milliseconds. Convert Unix Timestamp to Date in JavaScript When we create a new object from the Date () class using new Date (), it returns the time in milliseconds when it is created. If we need to get an object from the Date class at a specific point of time, we can pass the epoch timestamp to that class's constructor.
Date.parse () internally uses the new Date () for parsing of date, hence the formats accepted by the new Date () function will also be supported in the Date.parse () function. Split String and Convert It to Date in JavaScript Both the Date.parse () and the new Date () functions are designed based on the ISO 8601 extended date format. Aug 07, 2018 - If we have a UNIX timestamp, we can instantiate a JavaScript Date object by using · const timestamp = 1530826365 new Date(timestamp * 1000) unless the timestamp was generated by JS, in which case it’s already in the correct scale. Make sure you pass a number (a string will get you an “invalid date” result - use parseInt... JavaScript date parse () method The parse () method in JavaScript is used to parse the specified date string and returns the number of milliseconds between the specified date and January 1, 1970. If the string does not have valid values or if it is not recognized, then the method returns NaN.
The best string format for string parsing is the date ISO format together with the JavaScript Date object constructor. Examples of ISO format: YYYY-MM-DD or YYYY-MM-DDTHH:MM:SS. But wait! Just using the "ISO format" doesn't work reliably by itself. String are sometimes parsed as UTC and sometimes ... For those reasons, today we're going to talk about what JavaScript's Date constructor and parse() methods look for in a date string so that your scripts will have the very best chance of succeeding. Parsing Date Strings using the Date Object. Both the Date(string) constructor and parse() method work on exactly the the same date formats. Mar 01, 2013 - I recently wrote a script to parse emails on my online account for dates so that it could create reminders for article due dates. The experience made me realize the many difficulties inherent to converting a date string into a proper Date object. JavaScript's Date(string) constructor can be ...
JavaScript Date objects represent a single moment in time in a platform-independent format. ... Date.parse() Parses a string representation of a date and returns the number of milliseconds since 1 January, 1970, 00:00:00 UTC, with leap seconds ignored. The problem with dates in JSON - and really JavaScript in general - is that JavaScript doesn't have a date literal. You can represent strings, numbers, Booleans and even objects, arrays and RegEx expressions with language specific literals, but there's no equivalent literal representation for dates. Date.parse from a string. The method Date.parse(str) can read a date from a string. The string format should be: YYYY-MM-DDTHH:mm:ss.sssZ, where: YYYY-MM-DD - is the date: year-month-day. The character "T" is used as the delimiter. HH:mm:ss.sss - is the time: hours, minutes, seconds and milliseconds.
Date Methods. When a Date object is created, a number of methods allow you to operate on it.. Date methods allow you to get and set the year, month, day, hour, minute, second, and millisecond of date objects, using either local time or UTC (universal, or GMT) time. date as a timestamp is a numeric 10bytes per record to store a numeric string that represents date and time select * from table where date >date1 and date <date2 is so much faster to select date ranges when the dates are stored numeric formatting is done on output echo date (Y-m-d,$date); to store a date and time 2002-10-21, 12:35pm 18bytes, Aug 28, 2008 - JavaScript - Date parse() Method - Javascript date parse() method takes a date string and returns the number of milliseconds since midnight of January 1, 1970.
The first thing is using the Date () function to create an object in JavaScript: let currentDate = new Date () Then you should use the following script to get the current date in the "m-d-y" format. You can change the format. Aug 28, 2008 - JavaScript - Date parse() Method, Javascript date parse() method takes a date string and returns the number of milliseconds since midnight of January 1, 1970. You can get the same results using the Date.parse function instead of passing the date string to the Date constructor.Date.parse is indirectly being called inside the constructor whenever you pass a date string.. The format used in these strings is the ISO 8601 calendar extended format. You can refer to its details in the ECMAScript specification.. Pass date arguments
Date.parse () The Date.parse () method parses a string representation of a date, and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC or NaN if the string is unrecognized or, in some cases, contains illegal date values (e.g. 2015-02-31). It is not recommended to use Date.parse as until ES5, parsing of strings was entirely ... The Date Object The Date object is a built-in object in JavaScript that stores the date and time. It provides a number of built-in methods for formatting and managing that data. By default, a new Date instance without arguments provided creates an object corresponding to the current date and time. The best format for string parsing is the date ISO format with the JavaScript Date object constructor. But strings are sometimes parsed as UTC and sometimes as local time, which is based on browser vendor and version. It is recommended is to store dates as UTC and make computations as UTC. To parse a date as UTC, you should append a Z:
You can get the current date and time in JavaScript using the Date object. // the following gives the current date and time object new Date() Using the date object you can print the current date and time. let current_datetime = new Date() console.log(current_datetime.toString()) Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Parses the given string as Date using the given format. The format specifies which date component is expected where in the string. It can also be used to specify the timezone of the input string, and it may specify whether an empty string (including strings containing only whitespace) is allowed.
Different Date libraries approach this problem using a variety of solutions, some better than others. The Moment.js and Luxon Date libraries utilize a similar approach to date parsing using Date format strings. In today's blog, we'll learn how to create Luxon Date objects from Datetime strings. ISO 8601 Date Parsing Methods 3 hours ago - For these reasons, we agree with MDN's statement that parsing strings with the Date object is strongly discouraged. Modern JavaScript environments will also implement the by ECMA-402 specification, which provides the Intl object, and defines behavioral options of the Date object's toLocaleString, ... The date.parse() method is used to know the exact number of milliseconds that have passed since midnight, January 1, 1970, till the date we provide. Syntax: Date.parse(datestring); Parameters: This method accept a single parameter as mentioned above and described below: datestring: This parameter holds the date as a string.
May 14, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. JavaScript only has a Date object, which is misnamed since it is really a date+time. Which means it cannot parse a time string HH:mm:ss without a date, but it can parse a date string. There are a few options. Convert time to a datetime string and parse using Date (). Use Momentjs String + Format parsing function. Javascript parse date to string. Definition and Usage. The parse() method parses a date string and returns the number of milliseconds between the date string and midnight of January 1, 1970 @Duke— new Date(Date.parse('02.02.1999')) will produce identical results to new Date('02.02.1999'), including Invalid Date in some browsers.Using the built-in parser is arguably the worst way to parse a ...
And then you parse that into a true date object new Date('2020-01-01') Now, if you display this date you just created, what displays will depend heavily on your location in the world. To get the real date we need to split out the "/" or "-". For this, we will use a split method and get the real date into the function. Then we will parse the date into a new variable dateConvert. Here we have to care about a tricky part of this code. We should remember that the month index start from 0 that means January is count as 0. This chapter introduces the concepts, objects and functions used to work with and perform calculations using numbers and dates in JavaScript. This includes using numbers written in various bases including decimal, binary, and hexadecimal, as well as the use of the global Math object to perform ...
Learn How To Get Current Date Amp Time In Javascript
A Guide To Handling Date And Time For Full Stack Javascript
How To Create Date Range In Javascript Simple Examples
Learn How To Get Current Date Amp Time In Javascript
Article Parsing Datetime Data Via A Javascript Macro In Flow
Working With Javascript Dates Using Moment Js
Master Handling Date Time In E2e Fiori Scenario Handling
How To Manipulate Date And Time In Javascript
Javarevisited How To Parse Json With Date Field In Java
Github Rickstrahl Json Date Extensions Date Parsing
How To Work With Date In Plain Javascript No Libraries
Convert String Into Date Using Javascript Geeksforgeeks
Managing Dates And Times In Javascript Using Date Fns Sitepoint
Javascript Date Parse Learn How Does Date Parsing Work In
The Definitive Guide To Javascript Dates
Github Litejs Date Format Lite Format Parse And
Another Date Format Conversion Request Questions
How To Format Parse Dates With Localdatetime In Java 8
Date To String Format Javascript Code Example
0 Response to "21 How To Parse A Date In Javascript"
Post a Comment