23 Flow Control Statements In Javascript
Control Flow in JavaScript: program the browser to take different actions in different situations. In the last tutorial, we learned how to initialize variables. But that's not really all that much fun, is it? The real fun starts when we can apply logic to things; to control flow of the website's behavior. "If" Statements. if, while, and do-while have conditions that are, in principle, boolean. However, a condition only has to be truthy (true if coerced to boolean) in order to be accepted. In other words, the following two control flow statements are equivalent: if (value) {} if (Boolean(value) === true) {}
Javascript If Else Control Statements Simple Snippets
JavaScript Control Statements is a very crucial component in deciding the program flow control . Depending upon the JavaScript control statements the programs may split in many branches, or repeat certain steps certain number of times or may jump certain statements. JavaScript Control Statements are of three types. · Selection Statements.
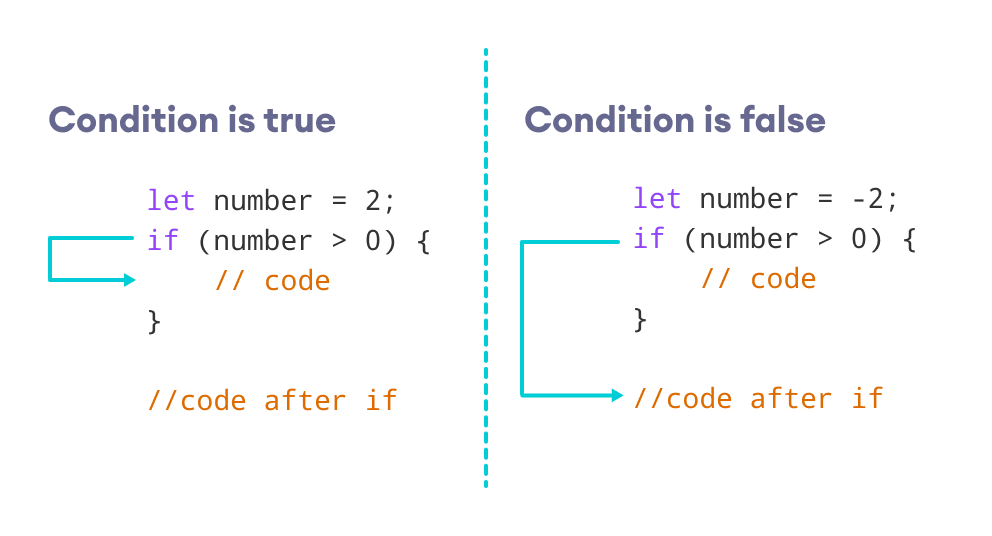
Flow control statements in javascript. Control structure actually controls the flow of execution of a program. Following are the several control structure supported by javascript. if … else. switch case. do while loop. while loop. for loop. If … else. The if statement is the fundamental control statement that allows JavaScript to make decisions and execute statements ... What are control statements in JavaScript? Control statements are designed to allow you to create scripts that can decide which lines of code are evaluated, or how many times to evaluate them. There are two different types of control statements: conditional statements and loop statements. What are expressions in JavaScript? This article is part of my Control Flow in JavaScript Series. In this article, we will be discussing conditional statements. Photo by Rohan Makhecha / UnsplashWhat is Control Flow and why do we need it? "In computer science, control flow is the order in which individual statements, instructions, or function
Using Labels to Control the Flow Starting from JavaScript 1.2, a label can be used with break and continue to control the flow more precisely. A label is simply an identifier followed by a colon (:) that is applied to a statement or a block of code. We will see two different examples to understand how to use labels with break and continue. It has been suggested that this page or section be merged into Wikibooks:JavaScript. (Discuss) A control flow statement modifies a program's control flow. A control structure additionally contains another statement which is executed under specified conditions, by modification and/or validation ... JavaScript offers several ways to control the flow of a program. Normally, a JavaScript program will begin to execute a program starting from the first line and continuing with each line to the end of the program. There are several different ways to control the flow of the program, including conditional statements and loops. Each offer the ...
Controlling the flow of our programs JavaScript lets us control what blocks of code to execute using if statements, if - else statements, if - else if - else statements, ternary operators, and switch statements. You'll be writing your code in flow-control.js. Make sure to run the tests using learn. The control flow is the order in which the computer executes statements in a script. Code is run in order from the first line in the file to the last line, unless the computer runs across the (extremely frequent) structures that change the control flow, such as conditionals and loops. Oct 29, 2012 - This tutorial will focus on the ... in Javascript. Control Flow is an important concept to learn in all programming languages, so the theory carries across multiple languages. ... Probably the most common Control Flow Structure you will encounter is some variety of the if, else, else if structure. ... The if statement simply makes ...
In computer programming, flow control refers to the logic that decides whether certain blocks of code will be executed or not. The most common source of flow conrtol in JavaScript is BEDMAS - which is an acronym that describes the order of operations in algebra. For those unfamiliar with BEDMAS, it stands for: The control structures within JavaScript allow the program flow to change within a unit of code or function. These statements can determine whether or not given statements are executed - and provide the basis for the repeated execution of a block of code. Block Statements Block statements (sometimes called compound statements) are not control flow statements, but they go hand in hand with them. A block statement is just a series of statements enclosed in curly braces that is treated by JavaScript as a single unit. While it is possible to have a block statement by itself, it has little utility.
Oct 27, 2018 - Examines ways to recognize particular conditions and have your JavaScript program act in a prescribed way as a result. Oct 27, 2016 - All of this logic and flow control is achieved using some very simple structures. These are: ... Conditional statements control whether a part of a script is executed depending the result of a particular expression (i.e. whether an expression returns a boolean true or false value). Jan 26, 2021 - The control flow is the order in which the computer executes statements in a script.
Types of Loops or Loops in JavaScript are: For Loop. For - in Loop. While Loop. Do-While Loop. 1. For Loop: For loop is there to execute a block of code multiple times, For Loop is one of the best examples of a control flow of programs in programming statement in Javascript. Below is the syntax of FOR LOOP. Once, the test expression is found false, the loop breaks, and the flow control will be moved to the next line. The break and continue Statements break Statement. In JavaScript, a break Statement is used to stop the execution of the code and moves the flow control to the line next to the closing braces of the switch statement. In this video tutorial, we have explained the overview of control statements / control structures / flow control statements in JavaScript.#itechnica#tutorial...
Control flow is in computer science the order that the instructions or statements or functions are executed. In javascript, we read the code starting from the first line till the last line unless of course in the code there is some instructions or statements that changes that control flow. Control Flow (continued) In the last lesson, we learned about the if operator. We can use if to check and see if an expression is true. If it is, run some code. If it is not, skip the code and keep running the program. To add on to if, we can also use the else if and else statements. JavaScript has two types of control statements. Conditional and Iterative (Looping) with their particular uses. We learned about conditional statements like IF, IF-ELSE, and SWITCH, along with their respective syntaxes. And for Iterative Statements, we learned about WHILE, DO-WHILE and FOR along with syntaxes.
Java compiler executes the code from top to bottom. The statements in the code are executed according to the order in which they appear. However, Java provides statements that can be used to control the flow of Java code. Such statements are called control flow statements. The default control flow is for statements to be read and executed in order from left-to-right, top-to-bottom in a program file. Control structures such as conditionals (if statements and the like) alter control flow by only executing blocks of code if certain conditions are met. Control flow in JavaScript is how your computer runs code from top to bottom. It starts from the first line and ends at the last line, unless it hits any statement that changes the control flow of...
Flow Control allows the execution of code only under certain conditions. In Ruby, we used if statements, if/else statements, if/elsif/else statements, ternary operators, and case statements to control what code runs when. JavaScript has similar methods to control what blocks of code to execute: ... Control Statements. Javascript Keywords. Javascript keywords Control Statements. if statement Already covered; Important new terminology in terms of control flow: if is a single-selection statement. It selects or ignores a single action (program statement(s)) In JavaScript, if is a conditional statement that is used to control the program flow just like in C, C++, etc programming languages. It is one of the most basic and simplest way to control the flow of program based on conditions. You can use the if statement when we want to execute code statements only when a particular condition is true.
if/else statements are how programs process yes/no questions programmatically. If the first condition evaluates to true, then the program will run the first block of code. Otherwise, it will run the else block · else if statements are used to add more conditions to an if/else statement Conditional statements control behavior in JavaScript and determine whether or not pieces of code can run. There are multiple different types of conditionals in JavaScript including: "If" statements: where if a condition is true it is used to specify execution for a block of code. JavaScript lets us control what blocks of code to execute using if statements, if - else statements, if - else if - else statements, ternary operators, and switch statements. You'll be writing your code in flow-control.js. Make sure to run the tests using learn.
JavaScript supports a compact set of statements, specifically control flow statements, that you can use to incorporate a great deal of interactivity in your application. This chapter provides an overview of these statements. The JavaScript reference contains exhaustive details about the statements in this chapter. Jun 15, 2018 - Therefore, control flow is the order in which the computer executes statements in a script or a file. Sometimes in an extreme situation the normal top to bottom flow we know about is not obeyed, this is where control flow statements come in, they determine how the program is being executed.
Lesson 07 Introduction To Conditional Statements
Tutorial 4 Decision Making With Control Structures And Statements Section A Decision Making Javascript Tutorial 4 Decision Making With Control
Introduction To Javascript Control Flow By Mahendra
Intro To Javascript Week 3 Control Statements
Javascript Control Statements Conditional Amp Looping Control
Learn Control Flow In Javascript While Do While For For In
If Else If Else Statement Glossary Entry Embedded Systems
Python Control Flow Statements
C Control Flow Structures C Plusplus Tutorial By Wideskills
A Definitive Guide To Conditional Logic In Javascript
Loops In Javascript Geeksforgeeks
Javascript If Else Statement With Examples
Control Flow Else If Statements Javascript Codecademy Forums
8 Javascript Tutorial Control Statements Theory
C Control Flow Structures C Plusplus Tutorial By Wideskills
Control Structures In R Master The Working Of Loops In R
Learn Javascript Journey Part 3 Tarantulo Lt
Javascript Control Flow If Else If Else Codecademy
Control Flow In Javascript What Is Control Flow What Type
Javascript Control Statements Conditional Amp Looping Control
0 Response to "23 Flow Control Statements In Javascript"
Post a Comment