30 Javascript Remove From Array Without Changing Index
Our goal is to remove all the falsy values from the array then return the array. The good people at freeCodeCamp have told us that falsy values in JavaScript are false, null, 0, "", undefined, and NaN. They have also dropped a major hint for us! They suggest converting each value of the array into a boolean in order to accomplish this challenge. Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript function to remove a specific element from an array
Change Array Index Position In Javascript By Up And Down
Using the splice method: The array.splice () method is used to add or remove items from an array. This method takes in 3 parameters, the index where the element's id is to be inserted or removed, the number of items to be deleted and the new items which are to be inserted.
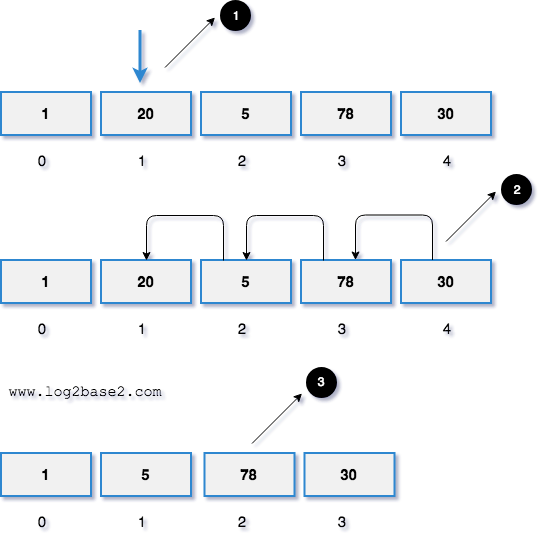
Javascript remove from array without changing index. The second parameter is the number of items to remove from the array. In the example above, two items are removed from the mutatingRemove array (second argument), starting from the index of 0 (the first argument). Like array.pop () and array.shift (), array.splice () returns the items it removes. To remove an element from an array in Angular or Typescript we can use javascript's delete operator or Array splice function. Using delete Operator. Using javascript delete operator we can remove an element from array in Angular or typescript as shown below. May 02, 2018 - JavaScript offers many ways to remove an item from an array. Learn the canonical way, and also find out all the options you have, using plain JavaScript
Sep 15, 2020 - The splice() method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place. To access part of an array without modifying it, see slice(). Array.splice allows us to remove elements from an Array starting from a specific index. We can provide a second argument to specify how many elements to delete. const arr = [1, 2, 3, 4, 5] const index = arr.indexOf(2) Jul 01, 2017 - I am working on a chat and using an array to hold the users. Here is my problem: User1 joins and is given Index 0 in the array via push. User2 joins and is given Index 1 in the array via push. Us...
Jul 23, 2019 - JavaScript's standard library doesn't provide a method for removing a specific element from an array. Be careful when you build your own! shift () methods return the deleted value and remove that value from the original array. Array splice () method:- This removes a specific element based on a given index. Common state action is to add or remove items from an array or to add or remove fields from an object. However, the standard operations are mutating the original object. Let's see how we can apply them in an immutable way. Our goal is to create a new object, rather than changing the existing.
May 06, 2021 - Learn more about Insert, Remove, Splice and Replace elements with Array.splice() from DevelopIntelligence. Your trusted developer training partner. Get a customized quote today: (877) 629-5631. To remove an element from an array, use the splice () method. JavaScript array splice () method changes the content of an array, adding new elements while removing old elements. The following are the parameters −. index − Index at which to start changing the array. howMany − An integer indicating the number of old array elements to remove. Jul 19, 2019 - Get code examples like "delete an element of an array without splice" instantly right from your google search results with the Grepper Chrome Extension.
To remove empty elements from a JavaScript Array, the filter () method can be used, which will return a new array with the elements passing the criteria of the callback function. The filter () method creates an array filled with all array elements that pass a test. To remove null or undefined values do the following: Aug 11, 2017 - That's where the second version of Array.remove() comes in. This one accepts a second boolean parameter to indicate that we want ALL occurrences of matching values. There are two very important points to notice in the following for loop: It goes backward. Otherwise, the index will access the ... 3/6/2020 · In JavaScript, the Array.splice() method can be used to add, remove, and replace elements from an array. This method modifies the contents of the original array by removing or replacing existing elements and/or adding new elements in place. Array.splice() returns the removed elements (if any) as an array. Syntax. Here is the syntax of Array.splice():
Aug 18, 2017 - Here’s a way to remove an array element without mutating the array. ... By default, the array methods in JavaScript will mutate your array. Mutation means that the original array is changed in place. A common way to do this is with splice. With splice, you tell the array at which index to start ... NAVIGATION Use splice() to remove arbitrary item Use shift() to remove from beginning Use pop() to remove from end Using delete creates empty spots Remember this This classic question pops up once in May 25, 2016 - It is that time of the week for Obvious Javascript Tip of the week. You probably already know how to delete an item from an array, maybe not. Sometimes it can be confusing, do I use the delete keyword, do I use slice or do I use splice? Splice of life Using splice we can […]
Here, the startIndex parameter specify the index at which to start splicing the array, it is required; the second parameter deleteCount is the number of elements to remove (if it is set to 0 no element will be removed). Let's check out an example to understand how it works: vue delete method. For Vue 2.2.0 + version, use vue.delete. Here is an syntax. Vue.delete( object/array, name/index ) First parameter is an object iteration or array Second parameter is index or an property name of an object. Inside javascript code, create an method for remove an object. with delete method, You can remove an property from an ... 17/11/2020 · How to remove an element at a specific position or index from an array in JavaScript? Published November 17, 2020 . To remove elements or items from any position in an array, you can use the splice() array method in JavaScript. Jump to full code; Consider this array of numbers, // number array const numArr = [23, 45, 67, 89];
JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any element whose index is greater than or equal to the new length will be removed. Remove last by changing length property Properties of the solution: May 23, 2017 - Possible Duplicate: Remove item from array by value | JavaScript How can I remove dog from the below array using Javascript. I do not want to use index if I can avoid it but rather the word dog Dec 03, 2020 - Without further ado, let’s first explore how to remove an item from an array in JavaScript by index and value.
Nov 19, 2020 - A common question in JavaScript is what’s the fastest way to remove a value from an array using built-in Javascript methods (vanilla JS). This article tests the performance of 4 different methods for… The pop() function will delete the element at a given index, and due to that index position of all the elements after the deleted elements will change (decrease by 1). The best solution is to sort the index positions in decreasing order and start calling the pop() function on index positions highest to lowest. In Javascript, there are two methods in Array.prototype for removing the first element of an array: shift() and splice(). shift() It returns the first element of the array and removes the element ...
Find the indexof the array element you want to remove using indexOf, and then remove that index with splice. The splice() method changes the contents of an array by removing existing elements and/or adding new elements. const array = [2, 5, 9]; 25/7/2018 · How to Remove an Element from an Array in JavaScript JavaScript suggests several methods to remove elements from existing Array . You can delete items from the end of an array using pop() , from the beginning using shift() , or from the middle using splice() functions. How the.splice () method works: The splice method is used to extract the range of elements from an array. It takes three arguments index, number of items to delete, an array of items to be appended. The index (first parameter) is required and the rest of the parameters are optional.
You can make use of ES6 Set to remove duplicate from an array. Here is how the code looks: function removeDuplicateUsingSet(arr) { let unique_array = Array.from(new Set(arr)) return unique_array } console.log(removeDuplicateUsingSet(array_with_duplicates)); As seen in the above code, you created a set using the duplicate array. Let's know first that what's Javascript filter method. Javascipt filter is a array method used to filter an array based on condition and it return a new array. Array.filter (function (elem, index, arr) { }) Array.filter ( (elem, index, arr)=> { }) We use a filter method to remove duplicate values from an array. The map() method in JavaScript creates an array by calling a specific function on each element present in the parent array. arr.filter() the function is used to create a new array from a given array consisting of only those elements from the given array which satisfy a condition set by the argument function.
How to use length property, delete operator, and pop, shift, and splice methods to remove elements from the end, beginning, and middle of arrays in JavaScript. javascript has various methods like, new Set(), forEach() method, for loop, reduct(), filter() with findIndex() to remove duplicate objects from javascript array. In the below, we will demonstrate to you javascript methods with examples for removing duplicate objects from the array. I want to know if there is a way to do this without make a full copy of the array and then splice the copy. var arr = [{id:1, name:'name'},{id:2, name:'name'},{id:3, name:'name'}]; I need to temp remove element by his index and use the array without this element, but i dont want to change the original array. You can give me way even with lodash.
The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice () method returns an array with the deleted items: Introduction to Dynamic Array in JavaScript. Dynamic Array in JavaScript means either increasing or decreasing the size of the array automatically. JavaScript is not typed dependent so there is no static array. JavaScript directly allows array as dynamic only. We can perform adding, removing elements based on index values. Summary: in this tutorial, you will learn how to remove duplicates from an array in JavaScript. 1) Remove duplicates from an array using a Set. A Set is a collection of unique values. To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements.
7/7/2020 · Array.splice will modify your original array and return the removed elements so you can do the following: const arr = [1,2,3,4,5]; const index = arr.indexOf(2); const splicedArr = arr.splice(index,1); arr; // [1,3,4,5]; splicedArr; // [2] Enter fullscreen mode. Exit fullscreen mode. Declaring an array: let myBox = []; // Initial Array declaration in JS. Arrays can contain multiple data types. let myBox = ['hello', 1, 2, 3, true, 'hi']; Arrays can be manipulated by using several actions known as methods. Some of these methods allow us to add, remove, modify and do lots more to arrays. In the above example, we removed seahorse from the array, and pushed a new value into index 3. Looping Through an Array. We can loop through the entirety of the array with the for keyword, taking advantage of the length property. In this example, we can create an array of shellfish and print out each index number as well as each value to the ...
1/1/2014 · JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any element whose index is greater than or equal to the new length will be removed. var ar = [1, 2, 3, 4, 5, 6]; ar.length = 4; console.log( ar ); Jun 02, 2013 - The JavaScript splice method allows for easy item removal from JavaScript arrays.
How To Replace Array Element In Javascript Without Mutation
Javascript Remove Object From Array By Index Code Example
Removing Items From An Array In Javascript Ultimate Courses
Java67 How To Remove An Element From Array In Java With Example
Remove Duplicates From Unsorted Array 3 Approaches
Understanding Arrays And Slices In Go Digitalocean
Javascript Remove Undefined From Array Code Example
Javascript Remove Empty Arrays From Array Code Example
7 Ways To Remove Duplicates From An Array In Javascript By
Using Powershell To Split A String Into An Array
How To Remove Array Duplicates In Es6 By Samantha Ming
Javascript Array Remove Element From Array Tuts Make
Remove A Specific Element From Array
Javascript Remove The Last Item From An Array Geeksforgeeks
Access Single Value Array Without Index Javascript Code Example
5 Way To Append Item To Array In Javascript Samanthaming Com
Remove An Array Element Without Mutation Jake Trent
How To Remove And Add Elements To A Javascript Array
How To Add Remove And Replace Items Using Array Splice In
Remove Duplicate Values From Js Array Stack Overflow
Javascript Remove A Specific Element From An Array Dev
How To Delete A Value From An Array In Javascript
How To Move An Array Element From One Array Position To
5 Ways To Delete An Element From An Array In Javascript Dev
Java Exercises Find The Index Of An Array Element W3resource
Python Arrays Create Update Remove Index And Slice
Javascript Remove Object From Array If Value Exists In Other
Picking A Random Item From An Array Kirupa Com
Javascript Splice How To Use The Splice Js Array Method
0 Response to "30 Javascript Remove From Array Without Changing Index"
Post a Comment