31 How To Require In Javascript
If JavaScript has been disabled within your browser, the content or the functionality of the web page can be limited or unavailable. This article describes the steps for enabling JavaScript in web browsers. More Information Internet Explorer. To allow all websites within the Internet zone to run scripts within Internet Explorer: Require: It is the builtin function and it is the easiest way to include modules that exist in separate files. The basic functionality of require is that it reads a JavaScript file, executes the file, and then proceeds to return the export object.
An Introduction To The Javascript Dom
Why do I need JavaScript enabled on Microsoft Edge? Microsoft Edge is a fast and secure browser that's built for great web experiences, but to get the most out of it, you'll need to enable JavaScript - a popular programming language that's essential to nearly every action you take online.
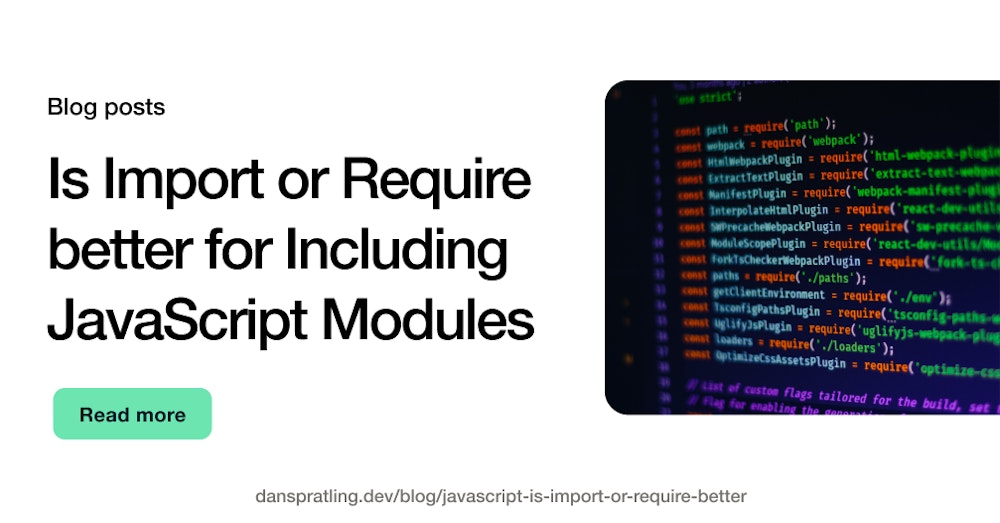
How to require in javascript. Node.js introduced support for the import statement in Node.js 12, although you need to opt in by setting a package.json configuration option.However, Node.js has no plans to drop support for require() (CommonJS). Which should you use? Below is a high-level summary of the tradeoffs: Before proceeding with this tutorial, you should have a basic understanding of HTML, CSS, JavaScript, Document Object Model (DOM) and any text editor. As we are going to develop a web based application using RequireJS, it will be good if you have an understanding on how internet and web based applications work. Now we just need to apply the main.js module to our HTML page. This is very similar to how we apply a regular script to a page, with a few notable differences. First of all, you need to include type="module" in the <script> element, to declare this script as a module. To import the main.js script, we use this:
JavaScript Form Validation. HTML form validation can be done by JavaScript. If a form field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted: 5/5/2019 · We have already seen that. That’s easy - just use require. 1. const aweFunky = require("/js/awefunky.js"); The require statement was used for the last thousand years and people seemed to be happy about them. Except when they weren’t, and used use or some other freakish statements to reference functions in other files. defaultExport. Name that will refer to the default export from the module. module-name. The module to import from. This is often a relative or absolute path name to the .js file containing the module. Certain bundlers may permit or require the use of the extension; check your environment.
13/9/2015 · The require() function, in Node.js, can read in both .js and .json files. If a file ends with .js, the file is parsed and interpreted as a JavaScript file and is expected to use the module syntax. And, if the file ends with .json, the file is parsed and interpreted as a JSON text file and is expected to adhere to the JSON syntax. require () in JavaScript This is an in-built node.js statement and is most commonly used to include modules from other separate files. It works by reading the JS file, executing it, and returning the object being exported. It is very popular for its ability to incorporate core node modules, community-based, and even local modules. 26/8/2011 · The basic functionality of require is that it reads a JavaScript file, executes the file, and then proceeds to return the exports object. An example module: console . log ( "evaluating example.js" ) ; var invisible = function ( ) { console . log ( "invisible" ) ; } exports . message = "hi" ; exports . say = function ( ) { console . log ( exports . message ) ; }
JavaScript is one of the 3 languages all web developers must learn: 1. HTML to define the content of web pages. 2. CSS to specify the layout of web pages. 3. JavaScript to program the behavior of web pages. This tutorial covers every version of JavaScript: The Original JavaScript ES1 ES2 ES3 (1997-1999) The Document Object Model (DOM) is the method by which JavaScript (and jQuery) interact with the HTML in a browser. To view exactly what the DOM is, in your web browser, right click on the current web page select Inspect. This will open up Developer Tools. The HTML code you see here is the DOM. Modules are a key construct to know JavaScript. We'll cover JavaScript modules: require and import during this Require vs Import article. These modules permit you to put in writing reusable code. By using Node Package Manager (NPM), you'll publish your module to the community.
3/7/2018 · Install all your prerequisites. Create a directory, navigate and initialize your project using NPM by issuing the below command. $ mkdir es6-app. $ cd es6-app. $ npm init. Fill in your details. Refer the below image for more info. Package.json configuration. You can leave fields empty and … This usually happens because your JavaScript environment doesn't understand how to handle the require() function reference. The require() function is only available by default on Node.js environment. 26. It's a way to include node.js packages. Luckily, the first package you linked to, markdown-js, is very smart. It checks whether it is included as a node package, and if not, will set the markdown object to window.markdown. So all you have to do is include this file in a <script> tag and you should be able to use the markdown object from the ...
TOP Interview Coding Problems/Challenges Run-length encoding (find/print frequency of letters in a string) Sort an array of 0's, 1's and 2's in linear time complexity In native JavaScript before ES6 Modules 2015 has been introduced had no import, include, or require, functionalities. Before that, we can load a JavaScript file into another JavaScript file using a script tag inside the DOM that script will be downloaded and executed immediately. Javascript is not specifically HTML related, but interacts with HTML and DHTML when used in a browser. It's a more traditional programming language, in that you can write a series of instructions to compute what kinds of actions should be taken based on various conditions, repeat things a variable number of times, and just generally take more complex and sophisticated actions.
Require.jsRequireJS is a JavaScript file and module loader. It is optimized for in-browser use, but it can be used in other JavaScript environments, like Rhi... require.ensure() is specific to webpack and superseded by import(). require. ensure (dependencies: String [], callback: function (require), errorCallback: function (error), chunkName: String ) Split out the given dependencies to a separate bundle that will be loaded asynchronously. When using CommonJS module syntax, this is the only way to ... 16/5/2013 · Inside the module aDirectory/aModule you can call require('./anotherModule') to load /my/page/aDirectory/anotherModule.js. You can also refer to parent directories, but you won’t be able to go higher than the module root path. Therefore require('../yourModule') and require('../../yourModule') both try to load /my/page/yourModule.js.
Run example ». Notice that we use ./ to locate the module, that means that the module is located in the same folder as the Node.js file. Save the code above in a file called "demo_module.js", and initiate the file: Initiate demo_module.js: C:\Users\ Your Name >node demo_module.js. If you have followed the same steps on your computer, you will ... 20/2/2021 · These are used when you try to use an external module inside the page you are currently working on. The main difference is : Import. import module from "module"; Lexical; will be sorted to the top of the current file. Only can be called in the beginning. Able to load partially (saving a lot of memories). Require. In the search bar, enter javascript.enabled, and then, in the search results, under "Preference Name", locate javascript.enabled. Right-click (Windows) or Ctrl-click (macOS) javascript.enabled, and then select Toggle to change its "Value" entry to true (enabled) or false (disabled). When you're finished, close the "about:config" tab.
These are the steps to install JavaScript in IE (Internet Explorer). Now as we have installed JavaScript we need an editor to write a script. So now we will see how to install JavaScript editor PyCharm, where we can write JavaScript. Pycharm is a cross-platform editor, here we will be using it for JavaScripts. PyCharm Supports Which languages? In NodeJS, require () is a built-in function to include external modules that exist in separate files. require () statement basically reads a JavaScript file, executes it, and then proceeds to return the export object. require () statement not only allows to add built-in core NodeJS modules but also community-based and local modules. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... The required property sets or returns whether a text field must be filled out before submitting a form.
In the search box, search for javascript.enabled; Toggle the "javascript.enabled" preference (right-click and select "Toggle" or double-click the preference) to change the value from "false" to "true". Click on the "Reload current page" button of the web browser to refresh the page. 19/3/2017 · require = function() { return { mocked: true }; } After doing the above reassignment of require, every require('something') call in the script will just return the mocked object. The require object also has properties of its own. We’ve seen the resolve property, which is a function that performs only the resolving step of the require process.
Is Import Or Require Better For Including Javascript Modules
Zero To Hashing In Under 10 Minutes Argon2 In Nodejs
Everything You Should Know About Module Amp Require In Node Js
Javascript Fab Framework On Node Js By Jamie Munro Medium
Omnis Technical Notes Using The Javascript Worker Object
Properly Deploying Typescript Libraries Using Require Js
Debug A Javascript Or Typescript App Visual Studio Windows
How To Use Scripts And Macros For Google Docs Or Sheets
Requiring Modules In Node Js Everything You Need To Know
Import Export Statements In Javascript Not Working Ides
Magento 2 Javascript Merge Harms Performance Explained
What Is Javascript And Why Is Gmail Blocking It
Require Vs Import Syntax In React Codemirror2 Stack Overflow
Webpacker In Rails 6 Using Javascript By Santiago Sanchez
How To Locate The Right Path For A Js File When Using
Github Suchipi Require Browser Easy To Use Require
Webstorm 2021 2 Reload Pages On Save Auto Import For
A Simple Tutorial On Requirejs Codeproject
Javascript Reference Guide Js Module Ecosystem Logrocket Blog
Getting Started With Node Js Modules Require Exports
How To Use Bootstrap Jquery And Other Libraries In Rails 6
Is Import Or Require Better For Including Javascript Modules
A Simple Guide To Javascript Code Splitting With Es5 And Es6
8 Best Nodejs Certification Amp Courses 2021 August Updated
Handling Common Javascript Problems Learn Web Development Mdn
Mixing Nodejs And Openjdk Easily Using Npm Modules On The
Introduction To Node Js A Beginners Guide To Node Js And
How To Control Hue Lights With Javascript
5 Reasons Why You Need Javascript In Your Website
How To Make Your Electron App Launch 1 000ms Faster By
0 Response to "31 How To Require In Javascript"
Post a Comment