24 Object Oriented Javascript Exercises
Exercise Time! "Object-oriented" JavaScript. JS is not a strict OO language like Java, but can still be used in an OO-like way. Future versions of JS may have more OO concepts built into the language. Simply put, object-oriented programming (OOP) is a popular style of programming. It comes up often in technical interviews, and it's an essential skill for every developer. If you're looking for a course that teaches you OOP from the ground up without any jargons or fluff, this course is for you. A perfect mix of theory and practice, packed ...
Amazon Com The Principles Of Object Oriented Javascript
How are the exercises organized? Each exercise is a small react application containing the following files: app.js: represents the entry python file that will be executed by the computer. index.html: represents the entry website. style.css: your website styles, they have to be imported from the index.html; README.md: contains exercise instructions.
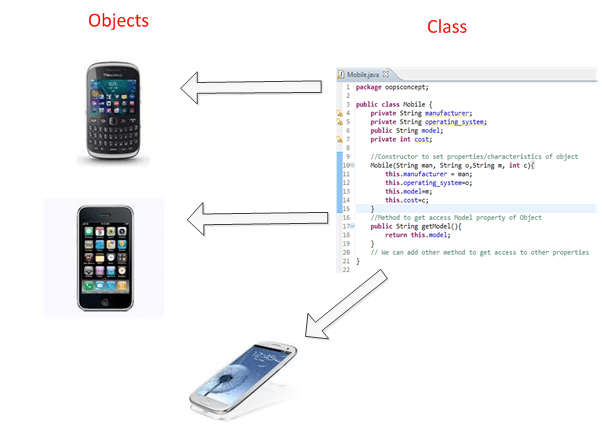
Object oriented javascript exercises. The characteristics of an Object are called as Property, in Object Oriented Programming and the actions are called methods. An Object is an instance of a class. Objects are everywhere in JavaScript almost every element is an Object whether it is a function,arrays and string. Note: A Method in javascript is a property of an object whose value is ... JavaScript has a built-in method called create () that allows you to do that. With it, you can create a new object, using an existing object as the prototype of the newly created object. With your finished exercise from the previous sections loaded in the browser, try this in your JavaScript console: Object oriented programming is a method of programming that attempts to model some process or thing in the world as a class or object. An object in this case is not the same as JavaScript's version of an object. Instead, you can conceptually think of a class or object as something that has data and can perform operations on that data.
Learn Object Oriented programming in Javascript (Prototypes, classes, modules) - ton of hands-on examples and exercises Rating: 4.0 out of 5 4.0 (101 ratings) 17,071 students Access over 7,500 Programming & Development eBooks and videos to advance your IT skills. Enjoy unlimited access to over 100 new titles every month on the latest technologies and trends Do exercises for Chapter 5 of Object Oriented Javascript (and maybe read it) Do exercises for Chapter 6 of Object Oriented Javascript (and maybe read it) Day 4: Deeper Dive and Final Project. Complete the Object Decorator Pattern Lesson on Udacity; Complete the Functional Classes Lesson on Udacity; Complete the Pseudoclassical Patterns Lesson ...
learn-js is a free interactive JavaScript tutorial for people who want to learn JavaScript, fast. Jan 18, 2017 - (Object Oriented JavaScript: Only Two Techniques Matter) [sc:mongodb-book] Prerequisite: JavaScript Objects in Detail JavaScript Prototype Object Oriented Programming (OOP) refers to using self-contained pieces of code to develop applications. We... Jun 16, 2021 - Udemy is an online learning and teaching marketplace with over 130,000 courses and 35 million students. Learn programming, marketing, data science and more.
Exercises. Create an object called shape that has the type property and a getType () method. Define a Triangle () constructor function whose prototype is shape. Objects created with Triangle () should have three own properties— a, b, and c, representing the lengths of the sides of a triangle. Add a new method to the prototype called getPerimeter (). Object-orientation's longevity can largely be explained by the fact that the ideas at its core are very solid and useful. In this chapter, we will discuss these ideas, along with JavaScript's (rather eccentric) take on them. The above paragraphs are by no means meant to discredit these ideas. Look at this code:function F() { function C() { return this; } return C(); } var o = new F();Does the value of this refer to the global object or the This website uses cookies and other tracking technology to analyse traffic, personalise ads and learn how we can improve the experience for our visitors and customers.
Jul 07, 2021 - JavaScript Exercises, Practice, Solution: JavaScript is a cross-platform, object-oriented scripting language. Inside a host environment, JavaScript can be connected to the objects of its environment to provide programmatic control over them. JavaScript Object [18 exercises with solution] [ An editor is available at the bottom of the page to write and execute the scripts.] 1. Write a JavaScript program to list the properties of a JavaScript object. Go to the editor. Sample object: var student = {. name : "David Rayy", Object-oriented programming allows developers to build applications with reusable and maintainable blocks of code, which leads to efficiency and simplified software design. With object-oriented JavaScript, you'll be able to build classes to construct objects that encapsulate both data and functionality. You'll also learn how to leverage prototypal inheritance to maintain DRY code, allowing you ...
Get Object-Oriented JavaScript - Second Edition now with O’Reilly online learning. O’Reilly members experience live online training, plus books, videos, and digital content from 200+ publishers. ... Create an object called shape that has the type property and a getType() method. In the guide Object-oriented JavaScript for beginners towards the end under the Further exercises title, there is additional task to change the bio() method, so that it works better. After completing the tasks I noticed that there could be another improvement added to the solution. Right now the way it is written the second to last item also shows with a comma, which isn't completely ... Create an object called shape that has a type property and a getType method. Define a Triangle constructor function whose prototype is shape. Objects created with Triangle should have three own properties: a, b and c representing the sides of a triangle. Add a new method to the prototype called getPerimeter.
Questions and Exercises: Object-Oriented Programming Concepts. Questions. Real-world objects contain ___ and ___. A software object's state is stored in ___. A software object's behavior is exposed through ___. Hiding internal data from the outside world, and accessing it only through publicly exposed methods is known as data ___. This example requires you to enter commands in your browser's JavaScript console (see What are browser developer tools for more information).What are browser ... JavaScript is a quite versatile language, used in both programming paradigms, i.e. functional & OOP (object-oriented programming). Absolute beginners need not worry about that just yet though! We'll cover this in an upcoming, intermediate post. 👍
Object-Oriented JavaScript [82 ] JavaScript is an object-based language. Just as in C#, you can create objects, call their methods, pass them as parameters, and so on. You could see this clearly when working with the DOM, where you manipulated the HTML document through the methods and properties of the implicit document object. When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ... Never forget another recipe · Keep track of which books you read and which books you want to read
Sep 24, 2020 - Continuing the idea of ten exercises for arrays, I made a collection of tasks for objects. Like the previous one, this collection is oriented for junior and middle javascript developers. For every… Learn to write cleaner, more modular, and more scalable code in JavaScript by gaining mastery of Object Oriented Programming (OOP). You'll start with the basics of object-oriented programming and build up to more advanced concepts such as prototypal inheritance, prototype chaining, method overriding and mixins. 🔥Get the COMPLETE course (70% OFF - LIMITED TIME): http://bit.ly/2keDCnaObject-oriented programming in JavaScript: learn all about objects, prototypes, prot...
Object-Oriented JavaScript A bit of history Browser wars and renaissance The present The future ECMAScript 5 Strict mode in ES6 ECMAScript 6 Browser support for ES6 ... Exercises Summary 4. Objects From arrays to objects Elements, properties, methods, and members Hashes and associative arrays Oct 30, 2015 - Contribute to gSchool/JS-Intro-OOP-Exercises development by creating an account on GitHub. Exercises Create an object called shape that has the type property and a getType() method. Define a Triangle() constructor function whose prototype is shape. Objects created with Triangle() should … - Selection from Object-Oriented JavaScript - Second Edition [Book]
Nov 01, 2011 - There are several methods for creating objects using JavaScript. These exercises teach you how to create objects using those methods as well as how to add properties and methods. Exercises. We have gathered a variety of JavaScript exercises (with answers) for each JavaScript Chapter. Try to solve an exercise by editing some code, or show the answer to see what you've done wrong. Count Your Score. You will get 1 point for each correct answer. Your score and total score will always be displayed. Jul 12, 2017 - ✔️ To complete this challenge: Write and test your code in the provided REPL.it links below (remember to click Save to save your work!), and copy the links to your solutions and paste them into a c...
In this exercise, you need to implement inheritance between two classes, Vehicle, and Car. This Object-Oriented Programming (OOP) exercise aims to help Python developers to learn and practice OOP concepts. Here I am providing 8 OOP programs to help you in brushing up your coding skills. All questions are tested on Python 3. Python Object-oriented programming ... 30 Exercises: variables, strings, functions, closure, JQuery, AJAX, JSON, arrays, loops. Link a JS file into a HTML file, put an alert, calculate average number of weeks in human lifetime, create variables to store a string, program that tells time of the day (morning, afternoon, night),etc. 6) NodeSchool-Github
Familiarize yourself with the basic syntax and structure of JavaScript. This popular programming language is great for beginners looking to dive into coding. Uber—Access to the Parent from a Child Object Isolating the Inheritance Part into a Function Copying Properties ; Heads-up When Copying by Reference ; Objects Inherit from Objects; Deep Copy object() Using a Mix of Prototypal Inheritance and Copying Properties Multiple Inheritance ; Parasitic Inheritance ; Borrowing a Constructor ; Summary JavaScript is an object-oriented programming (OOP) language we can use to model real-world items. In this lesson, you will learn how to make classes. Classes are a tool that developers use to q… ... In the last exercise, you created a class called Dog, and used it to produce a Dog object.
Object-Oriented JavaScript - Second Edition. $49.99 Print + eBook Buy; $29.99 eBook version Buy; More info. 1. Object-oriented JavaScript. Object-oriented JavaScript; A bit of history; ... Exercises; 7. The Browser Environment. The Browser Environment; Including JavaScript in an HTML page; BOM and DOM - an overview; BOM; DOM; Events ... This script gets a reference to the <canvas> element, then calls the getContext() method on it to give us a context on which we can start to draw. The resulting constant (ctx) is the object that directly represents the drawing area of the canvas and allows us to draw 2D shapes on it.Next, we set constants called width and height, and the width and height of the canvas element (represented by ...
Learn Javascript Visually With Interactive Exercises By
Github Obrown23 Js Oop Exercise
An Introduction To Object Oriented Javascript
Object Oriented Javascript Learn Javascript Free
A Beginner S Guide To Python Object Oriented Programming Oop
99 Bottles Sample Php Sandi Metz
Object Oriented Javascript Learn Javascript Free
C Essential Training 1 Syntax And Object Oriented
Top 10 Best Object Oriented Programming Courses In 2021
Java Object Oriented Programming Concepts W3resource
Javascript Beginner Start Here Exercises Included Snipcart
Bit 116 Javascript Bit 116 Scripting2 Today Ch 9 Object
Object Oriented Programming And Java Springerlink
Object Oriented Programming In Javascript By Priyanshu
Comprehensive Guide To Javascript Design Patterns Toptal
How To Hire A Javascript Developer Toptal
Best Books To Learn Object Oriented Programming In 2021
Javascript Exercises Practice Projects Exams
Object Oriented Javascript Learn Javascript Free
Object Oriented Analysis And Design Multiple Choice Questions
Object Oriented Javascript For Beginners Learn Web
0 Response to "24 Object Oriented Javascript Exercises"
Post a Comment