30 Javascript Add Two Event Listeners
Touch Event Listeners. Handling touch events in JavaScript is done by adding touch event listeners to the HTML elements to handle touch events for. This is done similarly to adding a click listener: var theElement = document.getElementById ("theElement"); theElement.addEventListener ("touchend", handlerFunction, false); function handlerFunction ... In JavaScript you add an event listener to a single element using this syntax: document.querySelector('.my-element').addEventListener('click', event => { //handle click }) Enter fullscreen mode. Exit fullscreen mode. But how can you attach the same event to multiple elements? In other words, how to call addEventListener () on multiple elements ...
How To Implement Addeventlistener Method In Javascript
The addEventListener () method allows you to add event listeners to any HTML DOM elements, the document object, the window object, or any other object that support events, e.g, XMLHttpRequest object. Here's an example that attaches an event listener to the window "resize" event: Example. Try this code ».
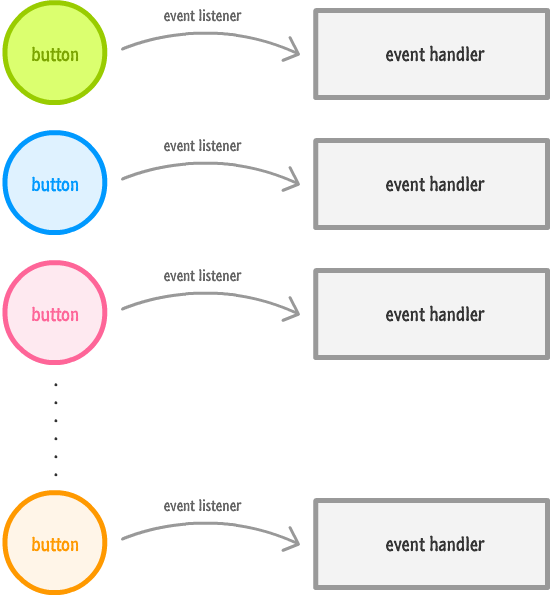
Javascript add two event listeners. Node.js allows us to add a listener for an event with the on () function of an event emitter object. This listens for a particular event name and fires a callback when the event is triggered. Adding a listener typically looks like this: eventEmitter.on(event_name, callback_function) { action } An event handler is also known as an event listener. It listens to the event and responds accordingly to the event fires. An event listener is a function with an explicit name if it is resuable or an anonymous function in case it is used one time. An event can be handled by one or multiple event handlers. If an event has multiple event handlers ... 7/4/2020 · To add the event listener to the multiple elements, first we need to access the multiple elements with the same class name or id using document.querySelectorAll () method then we need to loop through each element using the forEach () method and add an event listener to it. If your elements having different class-names rather than the same class ...
The JavaScript addEventListener () method lets you add event listeners to HTML DOM objects, such as HTML elements, the document that the HTML is in itself, the window object, and any other object that supports events (like the xmlHttpRequest object). How to Call Multiple JavaScript Functions in onClick Event. Topic: JavaScript / jQuery Prev|Next. Answer: Use the addEventListener() Method. If you want to call or execute multiple functions in a single click event of a button, you can use the JavaScript addEventListener() method, as shown in the following example: EventListener. The EventListener interface represents an object that can handle an event dispatched by an EventTarget object. Note: Due to the need for compatibility with legacy content, EventListener accepts both a function and an object with a handleEvent () property function. This is shown in the example below.
The addEventListener () is an inbuilt function in JavaScript which takes the event to listen for, and a second argument to be called whenever the described event gets fired. Any number of event handlers can be added to a single element without overwriting existing event handlers. Semi-related, but this is for initializing one unique event listener specific per element. You can use the slider to show the values in realtime, or check the console. On the <input> element I have a attr tag called data-whatever , so you can customize that data if you want to. When the event occurs, an event object is passed to the function as the first parameter. The type of the event object depends on the specified event. For example, the "click" event belongs to the MouseEvent object. useCapture: Optional. A Boolean value that specifies whether the event should be executed in the capturing or in the bubbling phase.
javascript adding click event listener to class . Posted by: admin February 6, 2018 Leave a comment. Questions: I have a listview for delete id. I'd like to add a listener to all elements with a particular class and do a confirm alert. ... Combining two form values in a loop using jquery; jquery - Get id of element in Isotope filtered items ... In JavaScript you add an event listener to a single element using this syntax: document.querySelector('.my-element').addEventListener('click', event => { //handle click }) But how can you attach the same event to multiple elements? In other words, how to call addEventListener () on multiple elements at the same time? Mouse button. Click-related events always have the button property, which allows to get the exact mouse button.. We usually don't use it for click and contextmenu events, because the former happens only on left-click, and the latter - only on right-click.. From the other hand, mousedown and mouseup handlers may need event.button, because these events trigger on any button, so button allows ...
You can also add event listeners using a method called addEventListener. However, this method isn't supported in Internet Explorer 8, so if you use this method, you need to add some conditional functions to check for browser functionality before running the function. In native JavaScript, we need to first select the DOM element that we want to add the event listener to. The querySelector () method selects the first element that matches a specified selector. So in our example, it selects the first <button> element on the page. var addEventListener = (function() { if(document.addEventListener) { return function(element, event, handler) { element.addEventListener(event, handler, false); }; } else { return function(element, event, handler) { element.attachEvent('on' + event, handler); }; } }());
In vanilla JavaScript, each event type requires its own event listener. Unfortunately, you can't pass in multiple events to a single listener like you might in jQuery and other frameworks. For example, you cannot do this: document.addEventListener('click mouseover', function (event) { // do something... }, false); The addEventListener () method attaches an event handler to the specified element. The addEventListener () method attaches an event handler to an element without overwriting existing event handlers. You can add many event handlers to one element. You can add many event handlers of the same type to one element, i.e two "click" events. Adding event handlers. We added an event listener of click, and called a showMore function. In the showMore function, we selected the class moreInfo. If you check on our css you find out that the text was set to display none. So now we are saying that onclick of the button, let the display none change to display block. We have created a dog ...
The example contains two event sources (JButton instances) and two event listeners. One of the event listeners (an instance of a class called MultiListener) listens for events from both buttons. When it receives an event, it adds the event's "action command" (which is set to the text on the button's label) to the top text area. Adding an event handler to the window object. The addEventListener() method allows you to add event listeners to any DOM object like HTML elements, the HTML document, and the window object. For example, here is an event listener that fires when the user scrolls the document: Copy and Paste the JavaScript code in your console to see how it looks like! Remove Event Listener. Another way to remove the event is the .removeEventListener function. Important: the .removeEventListener needs to have the same arguments of the .addEventListener that we are trying to remove. That includes the callback function, which needs to ...
The better ways to handle the scroll events. Many scroll events fire while you are scrolling a page or an element. If you attach an event listener to the scroll event, the code in the event handler needs to take time to execute.. This will cause an issue which is known as the scroll jank. The scroll jank effect causes a delay that the page doesn't feel anchored to your finger. The answer is to add an object to catch the key presses. let keysPressed = {}; document.addEventListener ('keydown', (event) => { keysPressed [event.key] = true; }); When a key is pressed we add the value as the object key and set it to true. We now have a flag to tell us when certain key values are active. Im able to animate two SVGs, each with a class of widget, upon loading the page. But when I add event listeners for mouse events to animate them on rollover, nothing happens when I mouse over or out of the SVG objects. Do SVGs have to be treated differently than other images for the event listene...
To work with events in JavaScript, you need to know about two concepts: event handlers and event listeners. An event handler is a function that is run when an event is triggered on a web page. Event listeners connect an event function to an HTML element, so when that function is executed, the web element is changed. type A case-sensitive string representing the event type to listen for. listener The object that receives a notification (an object that implements the Event interface) when an event of the specified type occurs. This must be an object implementing the EventListener interface, or a JavaScript function.See The event listener callback for details on the callback itself. An event listener is a JavaScript's procedure that waits for the occurrence of an event. The addEventListener () method is an inbuilt function of JavaScript. We can add multiple event handlers to a particular element without overwriting the existing event handlers.
The addEventListener method is the most preferred way to add an event listener to window, document or any other element in the DOM. There is one more way called "on" property onclick, onmouseover, and so on. But is not as useful, as it does not allow us to add multiple event listeners on the same element. The other methods allow it.
Running Event Listeners Outside Of The Ngzone Angular Indepth
Chapter 4 Javascript Interactivity Introduction To Web Mapping
Debug Javascript Using Chrome Developer Tools Dzone Web Dev
Debug Javascript Using Chrome Developer Tools Dzone Web Dev
4 Multiple Event Handlers Two S Company Head First Ajax
How To Dynamically Create Javascript Elements With Event
How To Handle Dom And Window Events With React Digitalocean
Gtmtips Simple Custom Event Listeners With Google Tag
How To Handle Event Handling In Javascript Examples And All
Debug Javascript Using Chrome Developer Tools Dzone Web Dev
Introduction To Keyboard Events In Javascript Engineering
Handling Events For Many Elements Kirupa
Getting To Know React Dom S Event Handling System Inside Out
Custom Events In Javascript A Complete Guide Logrocket Blog
How To Create Native Drag And Drop Functionality In
How To Find Event Listeners On A Dom Node In Javascript Or In
How To Make Your Web Page Interactive With Jquery Web
Add Event Listener On Multiple Elements Vanilla Js
Pause Your Code With Breakpoints Chrome Developers
Javascript Event Types 8 Essential Types To Shape Your Js
Concurrency Model And The Event Loop Javascript Mdn
Introduction To Event Listeners The Java Tutorials
Handling Events For Many Elements Kirupa
Multiple Events To A Listener With Javascript Dev Community
Javascript How To List All Active Event Listeners On A Web Page
Visual Tagger Segment Documentation
Handling Events Eloquent Javascript
How To Add Event Listener To Dynamically Added Elements Dev
Android App To Add Two Numbers Geeksforgeeks
0 Response to "30 Javascript Add Two Event Listeners"
Post a Comment