32 Difference Between Spread And Rest Operator In Javascript
Spread syntax is used to unpack iterables such as arrays, objects, and function calls. Rest parameter syntax will create an array from an indefinite number of values. Destructuring, rest parameters, and spread syntax are useful features in JavaScript that help keep your code succinct and clean. Rest parameters are exactly opposite of spread operator. Spread operator is used to expand all the items of the arrays while Rest parameters is used to condense different items to form an array. Rest parameters are also indicated with ... in javascript. The destructuring removed the first and second item and added the remaining items to the ...
Es6 What Is The Difference Between Rest And Spread By
Yes it concatenated between 2 arrays and unpacked myName into single elements and, unlike the rest parameter, the spread operator can be the first element, but rest parameter needs to be the last to collect the rest elements . It can be used to copy one array into another or to concatenate 2 arrays together.
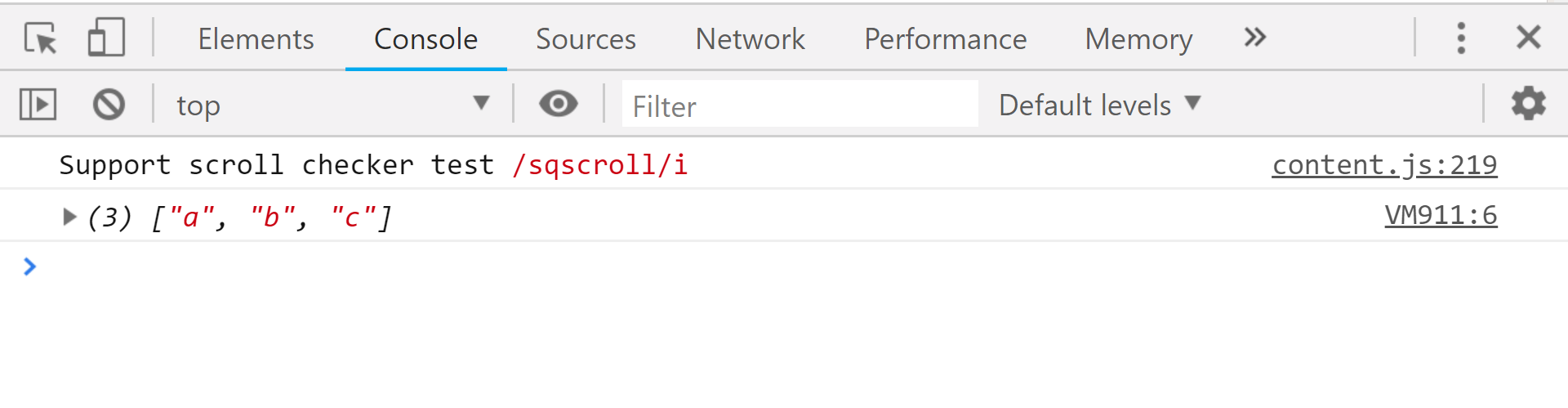
Difference between spread and rest operator in javascript. Spread Syntax in JavaScript. The Spread Syntax (also known as the Spread Operator) is another excellent feature of ES6. As the name indicates, it takes an iterable (like an array) and expands (spreads) it into individual elements. We can also expand objects using the spread syntax and copy its enumerable properties to a new object. JavaScript: A Beginner's Guide to Understanding Spread and Rest Syntax ... as a rest pattern and as the spread operator. ... The difference between the output of doubleNested and spreadArr ... Though the spread operator and the rest parameter look quite the same, their functionalities are quite different. So what's the difference between them? Let's gulp in things one at a time starting with what a Spread Operator is. The Spread operator allows an object that is capable of returning its elements one at a time to expand in places ...
24/11/2015 · Rest parameters are used to create functions that accept any number of arguments. The spread operator is used to pass an array to functions that normally require a list of many arguments. Together they help to travel between a list and an array of parameters with ease. For more information about this click here Javascript's three dots ( ... ): Spread vs rest operators. Javascript's ECMA6 came out with some cool new features; ... is one of these new Javascript functionalities. It can be used in two different ways; as a spread operator OR as a rest parameter. Rest parameter: collects all remaining elements into an array. are used as spread operator and rest parameter. In this video I am explaining the difference with examples for both.Want to crack any regex problem eff...
15/3/2021 · The difference is that spread syntax spreads the elements of an array or object into individual variables, whereas rest syntax condenses individual variables and puts them into an array or object. Examples of Using Spread Syntax 1. Spreading variables in a function call In this quick post, we've seen the difference between the Spread and Rest operators in JavaScript (ES6) which both makes use of the same three dots syntax. As a recap, you can use the Spread operator if you like to expand (unpack) the elements of an iterable like object, array or string literal. Spread operator allows an iterable to expand in places where 0+ arguments are expected. It is mostly used in the variable array where there is more than 1 values are expected. It allows us the privilege to obtain a list of parameters from an array. Syntax of Spread operator is same as Rest parameter but it works completely opposite of it.
In this lesson I cover the difference between the Spread and Rest syntax using an array of animals as an example. Spread is used to expand a list of items into an array or object and Rest is often used to help deal with function arguments, but can be used in a variety of circumstances to collect all items remaining excluding the assigned ones. Additional Resources: https://developer.mozilla ... Spread syntax can be used when all elements from an object or array need to be included in a list of some kind. In the above example, the defined function takes x, y, and z as arguments and returns the sum of these values. An array value is also defined. When we invoke the function, we pass it all the values in the array using the spread syntax ... In this quick post, we've seen the difference between the Spread and Rest operators in JavaScript (ES6) which both makes use of the same three dots syntax. As a recap, you can use the Spread operator if you like to expand (unpack) the elements of an iterable like object, array or string literal. In the contrary, you can use the Rest operator ...
Spread Operator. We have seen rest parameter converting individual arguments passed to a function into an array, in this section we will learn about spread operator. Spread operator does the exact opposite to what rest parameters did. It is used to convert an array into individual array elements. @DrazenBjelovuk .concat(x) makes the reader assume that x is an array as well. Sure, concat can handle non-array values as well, but imo that's not its main mode of operation. Especially if x is an arbitrary (unknown) value, you would need to write .concat([x]) to make sure it always works as intended. And as soon as you have to write an array literal anyway, I say that you should just use ... 23/12/2020 · The spread operator and the rest parameter have the same operator …. The difference is that with the spread operator we give or spread individual data of an array to another data. On the other hand, the rest parameter is used in a function or an array to get all the arguments or values and put them in an array or extract some pieces of them.
Use patterns: Rest parameters are used to create functions that accept any number of arguments. The spread syntax is used to pass an array to functions that normally require a list of many arguments. Together they help to travel between a list and an array of parameters with ease. rest When used to define the parameters of a function, it is the rest operator: function push ( ...items ) { console .log(items); } let a = 4 ; push(a, 1 , 2 , 3 ) //[4,1,2,3] Rest is mainly to convert multiple parameters of the function into an array, and can only be placed in the last position of the function parameter, otherwise, such as ... The spread operator. The ES6 spread operator is also represented by three dots (…) like the rest parameters, but if the rest operator can turn a variable number of parameters into an array, the spread operator can do the opposite: turn an array into a list of values or function parameters.
The Spread Operator has more than one function. In a sense, it is overloaded. It can also be used as rest operator in conjugation with destructuring. In ES6, the spread operator worked only for arrays. However, according to a proposal that has reached Stage 4, you can use the spread operator on objects too. Spread operator. While rest parameters use the rest operator to combine zero or more parameters into a single array parameter, the spread operator does just the opposite. It separates an array into zero or more parameters. But before we get into how the spread operator works, lets first take a look at the ES5 code it is intending to replace. But, the difference between the rest and spread syntax is that while spread copies everything, rest is used when we want to retrieve all remaining elements (or all existing elements) after a ...
14/6/2020 · The rest operator, is used to group remaining arguments in, usually in functions. The spread operator, on the other hand, is used to split a group of values in JavaScript. Key takeaway: rest groups, spread splits. Now in detail, The Rest Operator. This operator is used to get all or remaining arguments in a function as an array. For example: Rest and Spread operators in JavaScript. The rest operator (…) allows us to call a function with any number of arguments and then access those excess arguments as an array. The rest operator also allows us in destructuring array or objects. The spread operator (…) allows us to expand an iterable like array into its individual elements. There are three main differences between rest parameters and the arguments object: The arguments object is not a real array, while rest parameters are Array instances, meaning methods like sort, map, forEach or pop can be applied on it directly; ; The arguments object has additional functionality specific to itself (like the callee property).
The spread operator (...) spreads out the elements of an array (or iterable object). The rest operator condenses elements. The spread and rest operators are ... Difference between Spread and Rest Operators. Spread and rest operators are represented by … operator. Rest syntax means collecting rest of the remaining elements in the array. It will be a part of the function definition. Let me explain with a simple example JavaScript ES6 provides a new syntax represented by the "..." token which can be used as a spread operator and/or rest parameter. Knowing the difference between them gives you the confidence to when and where to use this token. Thus, that is what we are going to discuss in this post.
In the above code, the sum function accepts three arguments a, b and remaining.where the first two arguments values are 1, 2 everything we passed after is represented as an array [3,4,5,6,7].. Spread operator. Spread operator helps us to expand the strings or array literals or object literals. Splitting the strings
Javascript S Three Dots Spread Vs Rest Operators
A Simple Guide To Destructuring And Es6 Spread Operator By
Using Rest And Spread Operators In Javascript The Ninja Blog
Three Dots In Javascript Spread Amp Rest Operators Kanishk
Spread And Rest Operator In Javascript Devconquer
Javascript Es2018 Understanding The Spread And Rest Operator
Rest Operator Vs Spread Operator In Functions By Muesingb
The Difference Between The Spread Operator And The Rest
Difference Between Rest Amp Spread Operator Es6 Youtube
Javascript Spread Operator Geeksforgeeks
Adrian Oprea Website Performance And Core Web Vitals
Using Spread Rest Operator In Map Function Generates Error
Javascript Spread Operator And Rest Parameter Es6
Typescript Error When Using The Spread Operator Stack Overflow
Javascript Spread Operator Geeksforgeeks
Javascript Spread Operator And Rest Parameters
Rest Parameter And Spread Operator In Javascript Dev Community
The Difference Between Rest And Spread Operator In Javascript
Rest Vs Spread Operator In Javascript Simplified Thewebfor5
Javascript Object Destructuring Spread Syntax And The Rest
Javascript Es6 Spread Operator And Rest Parameters By Luke
Rest Operator Vs Spread Operator In Functions By Muesingb
How To Use The Spread And Rest Operator Dev Community
Javascript Spread Operator Code Example
Javascript Tutorial Spread Operator Vs Rest Operator
Tools Qa How To Use Javascript Rest Parameters Arguments
Javascript Es6 Spread Operator And Rest Parameters By Luke
A Simple Guide To Destructuring And Es6 Spread Operator By
Spread Operator Vs Rest Operator Parameters Dev Community
Javascript Es6 Spread And Rest Operators In Tamil
0 Response to "32 Difference Between Spread And Rest Operator In Javascript"
Post a Comment