27 Commonly Used Javascript Functions
A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. Functions are the main "building blocks" of the program. They allow the code to be called many times without repetition. We've already seen examples of built-in functions, like alert (message), prompt (message, default) and confirm (question). But we can create functions of our own as well.
What Is Javascript Used For Hack Reactor
The JSON is a JavaScript Object Notation. This function is a common setup to express conditions and objects. The JSON function can be used for data exchange when the client utilizes JavaScript and the server is based on Ruby/PHP. It gives two methods JSON.stringify and JSON.parse.
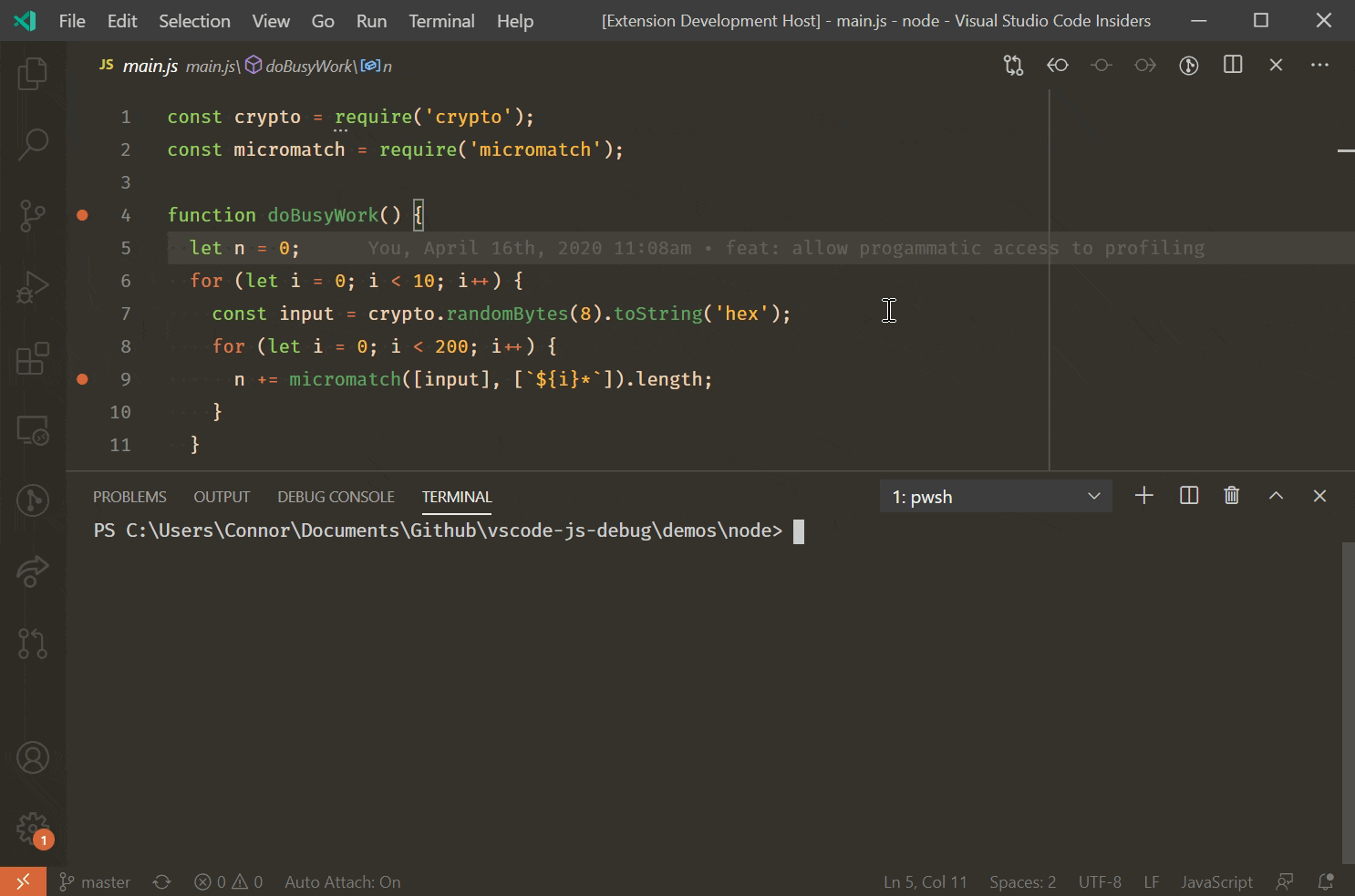
Commonly used javascript functions. Introduction to JavaScript Math Functions. The JavaScript Math is a built-in object that provides properties and methods for mathematical constants and functions to execute mathematical operations. It is not a function object, not a constructor. Often, when events happen, you may want to do something. JavaScript lets you execute code when events are detected. HTML allows event handler attributes, with JavaScript code, to be added to HTML elements. Because this function is fairly verbose and used frequently in JavaScript, the $ has long been used as its alias, and many of the libraries available for use with JavaScript create a $ () function that references an element from the DOM if you pass it the id of that element. There is nothing about $ that requires it to be used this way, however.
Strings - Learn how to work with JS strings and find the most common functions to work with this data type. Events - Use JavaScript event listeners to trigger functions. Numbers and math - Work with JS numbers, predefined constants and perform math functions. Dates - Get or modify current time and date. The number type represents both integer and floating point numbers.. There are many operations for numbers, e.g. multiplication *, division /, addition +, subtraction -, and so on.. Besides regular numbers, there are so-called "special numeric values" which also belong to this data type: Infinity, -Infinity and NaN. Infinity represents the mathematical Infinity ∞. Of all the functions in this article, as well as in the standard JavaScript library, reduce() is among the frontrunners for the crowns of "confusing and weird". While this function is highly important and results in elegant code in many situations, it's avoided by most JavaScript developers and they prefer to write more verbose code instead.
string.replace () description When you want to do a search and replace on a string in JavaScript, the replace () function is the one you want to make use of. This function searches the provided haystack, or string, for one or several substrings that match a given pattern or regular expression. It then replaces those matches with a replacement. 23/2/2018 · Like other programming languages, JavaScript also supports the use of functions. You must already have seen some commonly used functions in JavaScript like alert(), this is a built-in function in JavaScript. But JavaScript allows us to create user-defined functions also. We can create functions in JavaScript using the keyword function. In this article, I want to show you the commonly used Math functions that every JavaScript developer should know. In addition to general examples, I have provided different use-case examples to clarify the context of functions. Let's dive in and have fun! Introduction
Returns a number indicating the Unicode value of the character at the given index. 3. concat () Combines the text of two strings and returns a new string. 4. indexOf () Returns the index within the calling String object of the first occurrence of the specified value, or -1 if not found. 5. JavaScript is a client scripting language which is used for creating web pages. It is a standalone language developed in Netscape. It is used when a webpage is to be made dynamic and add special effects on pages like rollover, roll out and many types of graphics. It is mostly used by all websites for the purpose of validation. A user-defined function (UDF) is a function that you can create yourself and then add to the list of available functions in Excel when Excel doesn't provide the type of function that you want right out of the box. Excel Services already allows you to create UDFs using managed code, so if you have worked with the existing Excel Services UDFs ...
I remember the early days of JavaScript where you needed a simple function for just about everything because the browser vendors implemented features differently, and not just edge features, basic features, like addEventListener and attachEvent.. Times have changed but there are still a few functions each developer should have in their arsenal, for performance for functional ease purposes. A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). ... Functions can be used the same way as you use variables, in all types of formulas, assignments, and calculations. Example. Instead of using a variable to store the return value of a function: In this video, I'm going to show you my list of the most common javascript snippets!Source code: https://github /mdbootstrap/knowledge-base/tree/main/usef...
Expressions and operators. This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. A complete and detailed list of operators and expressions is also available in the reference. JavaScript Functions . JavaScript provides functions similar to most of the scripting and programming languages. In JavaScript, a function allows you to define a block of code, give it a name and then execute it as many times as you want. A JavaScript function can be defined using function keyword. The returned value. The context this when the function is invoked. Named or an anonymous function. The variable that holds the function object. arguments object (or missing in an arrow function) This post teaches you six approaches to declare JavaScript functions: the syntax, examples and common pitfalls.
21/10/2010 · Javascript Math Functions. Here are a few of the most commonly used Javascript Math Functions. isNaN(variableToTest) : Returns true if the variable is a number and false otherwise; Math.abs(variableToTest) : Performs an absolute value calculation; Math.ceil(variableToTest) : Rounds a number up; Math.floor(variableToTest) : Rounds a number down It returns a numeric value representing positive/negative infinity. undefined. It checks whether a variable is assigned a value or not. NaN. It represents Not-A-Number. location.protocol. It returns the protocol of the current URL. escape () It returns the encoded string. 16/9/2018 · Javascript is one of the most popular and commonly used programming languages in the world right now. It is a versatile language which is just one of the reasons why it is so popular. It can be used to program webpages, desktop and server programs, applications, etc.
Commonly Used JavaScript Functions. Contribute to YoussefZidan/JavaScript-Functions development by creating an account on GitHub. Also, functions in JavaScript can be used as objects and can be passed to other functions too. 4. Platform Independent. This implies that JavaScript is platform-independent or we can say it is portable; which simply means that you can simply write the script once and run it anywhere and anytime. What is JavaScript used for? JavaScript is mainly used for web-based applications and web browsers. But JavaScript is also used beyond the Web in software, servers and embedded hardware controls. Here are some basic things JavaScript is used for: 1. Adding interactive behavior to web pages. JavaScript allows users to interact with web pages.
The JavaScript filter () function is very useful for doing exactly what it says, filtering down a collection of elements based on a given test. When you call the filter () function, you need to pass it a callback. This callback is executed against every element in the array. Before we use a function, we need to define it. The most common way to define a function in JavaScript is by using the function keyword, followed by a unique function name, a list of parameters (that might be empty), and a statement block surrounded by curly braces. Global functions are functions built into every browser capable of running JavaScript. decodeURI () — Decodes a Uniform Resource Identifier (URI) created by encodeURI or similar decodeURIComponent () — Decodes a URI component encodeURI () — Encodes a URI into UTF-8
Where And How Can I Use Memoization In Javascript 30
What Is Javascript Learn Web Development Mdn
Top 20 Javascript Libraries You Should Know In 2021
Most Common Javascript Methods And Gotchas By Julia Zhao Xu
Web Design 101 How Html Css And Javascript Work
Aws Lambda In Production State Of Serverless Report 2017
What Are The Uses Of Javascript Javatpoint
String Functions In Javascript Collection Of Helpful Guides
The Top 15 Most Popular Javascript String Functions Vegibit
Important Javascript Functions You Need To Know About Edureka
Features Of Javascript Top 10 Characteristics Amp Comments Of
Refactoring Source Code In Visual Studio Code
Uses Of Javascript How And When Javascript Application Is
10 Amazing Libraries And Frameworks For Your Web Based
Javascript Functions Concept To Ease Your Web Development
Top 50 Javascript Interview Questions And Answers For 2021
1 9 Java Script Functions 2008 Pearson Education
Important Javascript Functions You Need To Know About Edureka
How To Write And Run Your First Program In Node Js Digitalocean
Tools Qa What Is Functions In Javascript And How To Define
Most Used Javascript Ecmascript 2015 Es6 And Ecmascript
Debug Node Js Apps Using Visual Studio Code
Learn Javascript History With This Infographic Checkmarx
0 Response to "27 Commonly Used Javascript Functions"
Post a Comment