24 Javascript Slice String After Character
The easiest method is to use the slice method of the string, which allows negative positions (corresponding to offsets from the end of the string):. var s = "your string"; var withoutLastFourChars = s.slice(0, -4); If you needed something more general to remove everything after (and including) the last underscore, you could do the following (so long as s is guaranteed to contain at least one ... JavaScript Array subString, Slice & More JavaScript has native members of the String object to help extract sub-strings based on character position: substring, substr and slice. Each return a string, extracted from an initial string based on start position and a length. On the surface they look almost identical, but have some subtle differences.
4 Ways To Remove Character From String In Javascript
Jul 26, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
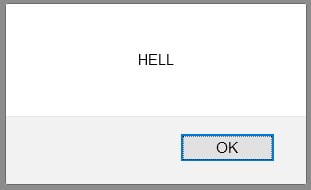
Javascript slice string after character. Definition and Usage The split () method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split () method does not change the original string. The slice () method extracts parts of a string and returns the extracted parts in a new string. Use the start and end parameters to specify the part of the string you want to extract. The first character has the position 0, the second has position 1, and so on. Tip: Use a negative number to select from the end of the string. In all cases, the second argument is optional. If it is not provided, the substring will consist of the start index all the way through the end of the string. For both the slice () and substring () methods, the second argument is exclusive; that is, the resultant substring will not contain the character at the final index.
take everything but first character javascript; slice string after character javascript; get from back string after a specific substring javascript; get a string after a specific substring javascript; output everything after js; javascript get substring after string; print only particuar part of sting js; html get certain string after a string Sep 16, 2020 - Get code examples like "get string after specific character javascript" instantly right from your google search results with the Grepper Chrome Extension. Jul 06, 2020 - Get code examples like "javascript get string after string" instantly right from your google search results with the Grepper Chrome Extension.
The js string slice () method extracts a part of a string (substring) and returns a new string. The syntax of slice () method is the following: 1 let substr = str.slice (begin [, end ]); Dec 02, 2017 - I have this string 'john smith~123 Street~Apt 4~New York~NY~12345' Using JavaScript, what is the fastest way to parse this into var name = "john smith"; var street= "123 Street"; //etc... May 20, 2020 - The substr() method returns a portion of the string, starting at the specified index and extending for a given number of characters afterwards.
As you can see, the first character in the string is "H," which has an index value of "0". The last character is "e," which has an index value of "10." Note that the space between the two words also has an index value. JavaScript Split. The JavaScript string split() method splits up a string into multiple substrings. Sep 10, 2019 - Here is my code : var string1= "Hello how are =you"; I want a string after "=" i.e. "you" only from this whole string. Suppose the string will always have one "=" character and i want all the str... JavaScript - remove first 3 characters from string. In JavaScript it is possible to remove first 3 characters from string in following ways. 1. Using String slice () method. In second example we can see that we can also use string slice on empty or shorter string then 3 characters (or in case if we want to remove n characters from our string).
slice() substring() substr() All these methods extracts parts of a string and returns the extracted parts in a new string. And all of them does not change the original string. 1. slice() method can take 2 arguments: Argument 1: begin, Required. The position where to begin the extraction. First character is at position 0. Remove substring from end of a string in javascript using slice() Example:- ... Remove last N characters from string in javascript; Javascript: Remove spaces from a string; Javascript: String remove until a character; Javascript: Replace all occurrences of string (4 ways) Jul 12, 2018 - How can I cut a string after a specific number of characters in JavaScript? I then want to append the '…' Unicode character. How can I do this?
JavaScript substring(),substr(),slice() are predefined methods in string prototype used to get the substring from a string.In this tutorial, we will understand each one of them & differences between them using examples.. Table of Contents. JavaScript substring(): JavaScript substring() syntax: JavaScript substring() Description: Javascript substring() Examples: The zero-based index before which to end extraction. The character at this index will not be included. If endIndex is omitted or undefined, slice () extracts to the end of the string. (E.g. "test".slice (2) returns "st" ) If endIndex is greater than str.length , slice () also extracts to the end of the string. If startIndex > endIndex, the slice( ) method returns an empty string; If startIndex is a negative number, then the first character begins from the end of the string (reverse): Note: We can use the slice( ) method also for JavaScript arrays. You can find here my other article about the slice method to see the usage for arrays. 3. The substr ...
lastIndexOf () is used to find the last instance. For both of these methods, you can also search for multiple characters in the string. It will return the index number of the first character in the instance. The slice () method, on the other hand, returns the characters between two index numbers. 29/5/2019 · split () method: This method is used to split a string into an array of substrings, and returns the new array. Syntax: string.split (separator, limit) Parameters: separator: It is optional parameter. It specifies the character, or the regular expression, to use for splitting the string. Method 2 - slice function. Use the javascript string slice function to remove the last character from your user based any string in JavaScript. This function simple check count and then extracts a part of any user string as well as simple return as fresh string. When We can set in a JavaScript Based variable.
Home › get string after character javascript › get string after first character javascript › get string after last character javascript. 34 Get String After Character Javascript Written By Ryan M Collier. Monday, ... Javascript Chop Slice Trim Off Last Character In String. Javascript Basic Remove A Character At The Specified. JavaScript function that generates all combinations of a string. The substring () method swaps its two arguments if indexStart is greater than indexEnd , meaning that a string is still returned. The slice () method returns an empty string if this is the case. If either or both of the arguments are negative or NaN, the substring () method treats them as if they were 0 . slice () also treats NaN arguments as 0 ...
For those trying to get everything after the first occurance: Something like "Nic K Cage" to "K Cage".. You can use slice to get everything from a certain character. In this case from the first space: As you can see from the above function, in the input string value there is whitespace at the end of the string which is successfully removed in the final output. Using slice() method. slice() method retrieves the text from a string and delivers a new string. Let's see how to remove the first character from the string using the slice method. Jul 21, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
May 23, 2017 - I have a string test/category/1. I have to get substring after test/category/. How can I do that? See the Pen JavaScript Get a part of string after a specified character - string-ex-22 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to repeat a string a specified times. Next: Write a JavaScript function to strip leading and trailing spaces from a ... Slice () and splice () methods are for arrays. The split () method is used for strings. It divides a string into substrings and returns them as an array. It takes 2 parameters, and both are optional.
How to get a part of string after a specified character in JavaScript? Javascript Object Oriented Programming Programming To get a part of a string, string.substring () method is used in javascript. Using this method we can get any part of a string that is before or after a particular character. Approach 1: Split the string by.split () method and put it in a variable (array). Use the.length property to get the length of the array. From the array return the element at index = length-1. Is there a way to remove everything after a certain character or just choose everything up to that character? I'm getting the value from an href and up to the "?", and it's always going to be a different amount of characters. ... javascript find string and delete all the following character. 1. ... JavaScript chop/slice/trim off last character ...
I have developed a function with a similar outcome to jay's Checks if the last character is or isnt a space. (does it the normal way if it is) It explodes the string into an array of seperate works, the effect is... it chops off anything after and including the last space. Javascript remove everything after a certain character using slice () The slice (startIndex, endIndex) method will return a new string which is a portion of the original string. The startIndex and endIndex describe from where the extraction needs to begin and end. If any of these indexes are negative, it is considered -> string.length - index. Strings in JavaScript are iterable and behave somewhat like arrays as each character in the string has its own index number. As a result, we can get a range of indexes from a string. JavaScript provides three methods for getting parts of a string each of which has slightly different behaviours; substring (), substr () and slice ().
Python Slice String Know The Reason Why We Use Python Slice
Javascript Remove First Last Specific Character From String
Javascript Substring How Is It Different From Substr
Postgresql Substring Function W3resource
How To Use Slice Amp Substring To Get Parts Of A String In
Regex To Split String Before Character In Javascript Code Example
Python Slice Constructor Python Slice String Amp Slicing
Python Slice String Know The Reason Why We Use Python Slice
How Can I Get Last Characters Of A String Stack Overflow
Remove Last Character From A String In Javascript Speedysense
Javascript Substring Vs Substr Vs Slice Differences With
How To Index And Slice Strings In Python 3 Digitalocean
Golang String Between Before And After Dot Net Perls
Basics Of Javascript String Slice Method By Jakub
Get First N Characters Of String Javascript Simple Example Code
Strings In Powershell Replace Compare Concatenate Split
Javascript Basic Remove A Character At The Specified
Understanding The Slice Method In Javascript The Basics The
How To Force Javascript To Deep Copy A String Stack Overflow
Get String After Specific Character Javascript Code Example
4 Ways To Convert String To Character Array In Javascript
Strings Slice Method In Javascript Demo
0 Response to "24 Javascript Slice String After Character"
Post a Comment