29 Javascript Add Two Strings
The JavaScript += operator can merge two strings together. This operator is more convenient than the long-form "variable = x + y" syntax. For instance, say you have a user's forename and the surname in two strings. You could use the += operator to merge these values into one string. JavaScript add strings with + operator The easiest way of concatenating strings is to use the + or the += operator. The + operator is used both for adding numbers and strings; in programming we say that the operator is overloaded.
How To Convert Strings To Numbers Using Javascript
4 different ways to Join two Strings in JavaScript. javascript1min read. In this tutorial, we are going to learn about 4 different ways to join two (or more) strings in JavaScript. First way: plus operator. We can use the plus + operator to join one string with another string.
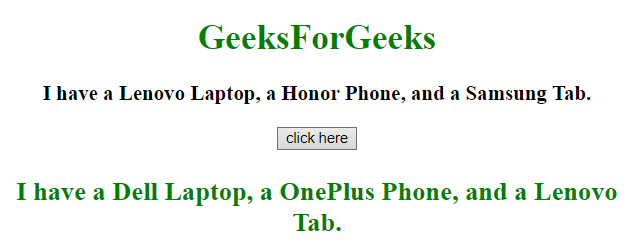
Javascript add two strings. The above program asks the user to enter two numbers. Here, prompt () is used to take inputs from the user. parseInt () is used to convert the user input string to number. const num1 = parseInt(prompt ('Enter the first number ')); const num2 = parseInt(prompt ('Enter the second number ')); Then, the sum of the numbers is computed. There are 3 ways to concatenate strings in JavaScript. In this tutorial, you'll the different ways and the tradeoffs between them. The + Operator. The same + operator you use for adding two numbers can be used to concatenate two strings.. const str = 'Hello' + ' ' + 'World'; str; // 'Hello World'. You can also use +=, where a += b is a shorthand for a = a + b. ... Add two string time values (HH:mm:ss) with javascript - add_two_times.js
javascript string concatenation es6. In this tutorial, we will explain the javascript string.concat() method with the definition of this method, syntax, parameters, and several examples. JavaScript String concat() JavaScript concat() method, which is used to add or join or combine two or more strings together and it will return a new string. Syntax Create a string with multiple spaces in JavaScript. We have a string with extra-spaces and if we want to display it in the browser then extra spaces will not be displayed. Adding the number of spaces to the string can be done in the following ways. This method gets a part of a string, starts at the character at the defined position, and returns ... Strings are used to store a sequence of characters in Java, they are treated as objects. The String class of the java.lang package represents a String.. You can create a String either by using the new keyword (like any other object) or, by assigning value to the literal (like any other primitive datatype).
The concat () function concatenates the string arguments to the calling string and returns a new string. Changes to the original string or the returned string don't affect the other. If the arguments are not of the type string, they are converted to string values before concatenating. Addition of two numbers using JavaScript. In this JavaScript program, ... JavaScript code to add these values < script language = " javascript " > function addNumbers () ... (find/print frequency of letters in a string) Sort an array of 0's, 1's and 2's in linear time complexity; The concat () method joins two or more strings. concat () does not change the existing strings, but returns a new string.
For just two strings, you can append as follows: Basically, you can think of it as concatenating str2 to str1. The left-hand side is getting the right-hand side added to it. Appending two or more strings to another Code language: JavaScript (javascript) Summary. Generally, if the strings contain only ASCII characters, you use the === operator to check if they are equal. When the strings contain characters that include combining characters, you normalize them first before comparing them for equality. How to join two strings in JavaScript Find out how to combine strings using JavaScript. Published Sep 22, 2019. ... you can use the += operator to add the second string to the first: name += surname. Alternatively you can also use the concat() method of the String object, which returns a new string concatenating the one you call this method on, ...
JavaScript offers us three different ways to concatenate or joining strings. The first way is using the plus + operator to join two strings. The second way is using the string interpolation with the help of es6 string literals. The third way is using the += operator to assign a string to the existing string. JavaScript String object has a built-in concat () method. As the name suggests, this method joins or merges two or more strings. The concat () method doesn't modify the string in place. Instead, it creates and returns a new string containing the text of the joined strings. I have two strings which contain only numbers: var num1 = '20', num2 = '30.5'; I would have expected that I could add them together, but they are being concatenated instead: num1 + num2; // = '
str.concat () function is used to join two or more strings together in JavaScript. Front End Technology Object Oriented Programming Javascript To add two strings we need a '+' operator to create some space between the strings, but when the first string itself has a space with in it, there is no need to assign space explicitly. As in the introduction above, we can simply use the + operator to "add" two strings together. But in this case, we call it "concatenation" (concat for short). Also, here is a convenient shorthand if you do not already know - first = first + second can be shortened to first += second.
JavaScript String Operators The + operator can also be used to add (concatenate) strings. I showed a few examples of using the different ways of concatenating strings. Which way is better all depends on the situation. When it comes to stylistic preference, I like to follow Airbnb Style guide. When programmatically building up strings, use template strings instead of concatenation. eslint: prefer-template template-curly-spacing Description In JavaScript, concat () is a string method that is used to concatenate strings together. The concat () method appends one or more string values to the calling string and then returns the concatenated result as a new string.
First, we will clarify the two types of strings. JavaScript differentiates between the string primitive, an immutable datatype, and the String object. In order to test the difference between the two, we will initialize a string primitive and a string object. // Initializing a new string primitive const stringPrimitive = "A new string."; 6 Answers6. var myString = "Hello "; myString += "World"; myString += "!"; the result would be -> Hello World! simply used the + operator. Javascript concats strings with +. To use String.concat, you need to replace your existing text, since the function does not act by reference. Of course, the join () or += suggestions offered by others will ... In JavaScript, there are three ways you can create multi-line strings: Using new line characters (\n). Using string concatenation. Using template string literals. In this guide, we're going to talk about how to use each of these three approaches to write a multi-line string. We'll walk through examples of each approach so you can easily get ...
In Javascript, you can do that in a multitude of ways, and one of them is by using the String concatenation operator, or the "+" operator. Now, this "+" operator, if used with numbers, like this: var a = 1 + 2; that would just add these two numbers and give out 3 as the output.
Javascript Basic Concatenate Two Strings And Return The
How To Work With Strings In Jmeter Blazemeter
Concatenate Strings C Slide Share
Js Add String To Url Code Example
Python Program To Concatenate Strings
Strings Concatenation In C Javatpoint
Search For A Text String And Return Multiple Adjacent Values
Adding Two Numbers Concatenates Them Instead Of Calculating
Javascript How To Add Two Strings Demo
C Program To Concatenate Two Strings Without Using Strcat
Optimum Way To Compare Strings In Javascript Geeksforgeeks
C Program To Concatenate Two Strings Without Using Strcat
3 Ways To Concatenate Strings In Javascript Mastering Js
Javascript Replace Multiple Strings With Multiple Other
Python Concatenate Strings Step By Step Guide Career Karma
How To Create Multi Line String With Template Literals In
Add Two Strings In Javascript Demo
Splitting Concatenating And Joining Strings In Python
5 Way To Append Item To Array In Javascript Samanthaming Com
C Program To Concatenate Two Strings Using Strcat
Javascript Algorithm Es6 String Addition By Erica N
Sql Server Concatenate Operations With Sql Plus And Sql
Javascript Strings Find Out Different Methods Of String
Javascript String Concat How To Concat String In Javascript
How To Obtain An Url String By Concatenating Two Strings In
Javascript Basics String Concatenation With Variables And
0 Response to "29 Javascript Add Two Strings"
Post a Comment