33 Javascript Find Unique Values In Array
find intersection between two object arrays javascript @SterlingDiaz if your values are all positive, yes you can optimize it by using the values as indexes in another array, so only the values set are unique - LemonCool Jun 8 '19 at 16:39 Add a comment |
How To Find Unique Values By Property In An Array Of Objects
Definition and Usage. The find() method returns the value of the array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find() returns the value of that array element (and does not check the remaining values)
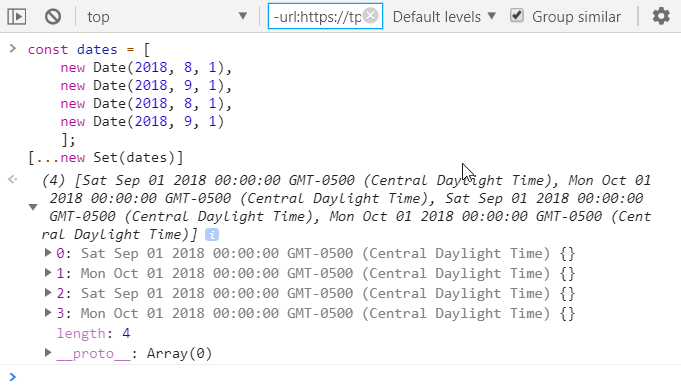
Javascript find unique values in array. Let's tackle the classical problem of getting an array of unique values in a JavaScript array. Method 1: ES5 Syntax This method uses the inbuilt Array.prototype.filter method available as a part ... How to get distinct values from an array of objects in JavaScript? Posted by: admin November 26, 2017 Leave a comment. ... This will be O(n) where n is the number of objects in array and m is the number of unique values. There is no faster way than O(n) because you must inspect each value at least once. For this example, we take the first array, arr1 and loop through all the values, checking to see if each value is found in arr2. If they are unique, then we add them to our final array, combinedArr. Once the forEach is complete, we append the entire contents of arr2 onto the end of combinedArr because we know that all the values in there will ...
Here is a code snippet demonstrating using Set with primitive values, like numbers and strings, to find the unique values in a JavaScript array. View raw code as a Github Gist. const array: string[] = [ 'Scooby', 'Dooby', 'Dooby', 'Doo' ]; The Good, The Bad, and The Ugly. Approach One: The Ugly. The most-straightforward way might be to keep two arrays: the original array and the array intended for unique values, and only add over values into the unique array if we've confirmed that the value doesn't already exist. The values () method returns a new Array Iterator object that contains the values for each index in the array.
Sep 09, 2019 - It's often to have an array that contains duplicate values and you want to make them unique. For example, you might have ["a", 1, "a", 2, "1", 1] and want to get ["a", 1, 2, "1"]. ... Utilizing many features in JavaScript like prototypes and anonymous functions, you can get that result with ... Method 1: This method checked each value of the original array (listArray) with each value of output array (outputArray) where the duplicate values are removed. If the current value does not exist in the output array with unique values, then add the element to the output array. Example 1: This example generates an unique array of string values. Javascript queries related to "get distinct values from list of objects typescript" array unique by key js; js only unique keys; javascript get unique values from key
There are multiple methods available to check if an array contains duplicate values in JavaScript. You can use the indexOf() method, the Set object, or iteration to identify repeated items in an array . Jan 04, 2021 - Finding the unique strings from a JavaScript array is a common task for web developers — here’s how to do it. Finding Unique Objects in a JavaScript Array. The powerful ES6 feature Set only accepts one copy of each value added to it, making it perfect for finding distinct objects in JavaScript. "The Set object lets you store unique values of any type, whether primitive values or object references." — MDN docs
10/3/2021 · This example will teach you three javascript methods or functions to get unique values from arrays in JavaScript ES6. Three methods to get unique values from arrays in ES6. Using Set; Using Array.filter; Using Iteration; Using Set. In ES6, a Set is a collection of unique values. If you can pass an array into a Set, it return unique values. We have a Javascript array and we want to find the all non-unique elements of the array. To get the all non-unique values from the array here are few examples. Array Slice () method. This method returns the selected elements in a new array object. This method gets the elements starting from the specified start argument, and ends at excluding ... Mar 03, 2020 - Find the unique elements in the array. ... given an array of integers,create 2 dimensional arrray where the first elemnet is a distinct values from the array and second element is that value frequency with the array
Jan 20, 2019 - Here are three ways to filter out duplicates from an array and return only the unique values. My favorite is using Set cause it’s the shortest and simplest 😁 See the Pen JavaScript -Find the unique elements from two arrays-array-ex- 42 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous:Write a JavaScript function to generate an array between two integers of 1 step length. This will be O(n) where n is the number of objects in array and m is the number of unique values. There is no faster way than O(n) because you must inspect each value at least once. ... Browse other questions tagged javascript arrays unique or ask your own question.
To get an array with unique values you could now do this: var myArray = ['a', 1, 'a', 2, '1'];let unique = [...new Set(myArray)];console.log(unique); // unique is ['a', 1, 2, '1'] The constructor of Settakes an iterable object, like Array, and the spread operator ...transform the set back into an Array. filter () method takes a callback function in which we can provide the condition to filter the unique items. If the condition is true then the item will be added to the new array. It return's the new array with unique items. Jan 04, 2021 - How to Find Unique Values by Property in an Array of Objects in JavaScript · Let’s find the distinct values for a given property among all of the JavaScript objects in an array in this short tutorial.
Find the unique elements in the array. ... Create a function unique(arr) that should return an array with unique items of arr. ... For each number in the array that is a duplicate, remove that number from the array and store it in an object as javascript Aug 22, 2016 - How can I get a list of unique values in an array? Do I always have to use a second array or is there something similar to java's hashmap in JavaScript? I am going to be using JavaScript and jQuer... To resolve or get unique value from the filtered array, we added the filter () method again and using the indexOf () to compare values from self and fetch unique values. Moreover, you can also wrap it in a function as shown below:
Get the Unique Values From an Array Using the Set () Function in JavaScript To get unique values from an array, we can use the Set () function, which creates a new array with unique values from the existing array. Find Unique pair in an array with pairs of numbers. 03, Oct 17. Minimum Increment operations to make Array unique. 26, Nov 18. How to get all unique values (remove duplicates) in a JavaScript array? 23, Apr 19. JavaScript | Get all non-unique values from an array. 13, May 19. 24/9/2020 · Here are a few ways to filter unique values in javascript. 1. Using Set: Set is a new data structure introduced in ES6. It is somewhat similar to an array but it does not allow us to store duplicate values. To filter unique values we pass an array to the Set constructor and we frame back an array from the Set object using Array.from method.
5/8/2021 · We have used a few JavaScript techniques to manipulate a JavaScript Array of Objects to get a unique list of values for a particular key. Paramount to this is the use of the Array.map() method as well as the extremely useful Set Object and Spread operator. The values in a set are unique in the Set's collection. Using this property of a Set, we can pass an array into a Set and because each value in the Set has to be unique, the value equality will be checked. This means the Set will only retain unique values. We can, therefore, using the spread operator return a new array of unique values. The find () method returns the value of the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined is returned. If you need the index of the found element in the array, use findIndex (). If you need to find the index of a value, use Array.prototype.indexOf ().
Mar 27, 2021 - A simple JavaScript function to find all unique values ... In a previous article — Three Easy Ways to Remove Duplicate Array Values in JavaScript—we covered three different algorithms to create an array of unique values. The most common request that came from that article was a similar ... Here are 3 ways to filter out duplicates from an array and return only the unique values... Because each value in the Set has to be unique, the value equality will be checked. In an earlier version of ECMAScript specification, this was not based on the same algorithm as the one used in the === operator. Specifically, for Sets, +0 (which is strictly equal to -0) and -0 were different values. However, this was changed in the ECMAScript 2015 specification.
UPDATED: The following uses an optimized combined strategy. It optimizes primitive lookups to benefit from hash O(1) lookup time (running unique on an array of primitives is O(n)). Object lookups are optimized by tagging objects with a unique id while iterating through so so identifying duplicate objects is also O(1) per item and O(n) for the whole list. Find the unique elements in the array. ... Create a function unique(arr) that should return an array with unique items of arr. ... javascript create variable containing an object that will contain three properties that store the length of each side of the box There are two common ways (in ES5 and ES6, respectively) of getting unique values in JavaScript arrays of primitive values. Basically, in ES5, you first define a distinct callback to check if a value first occurs in the original array, then filter the original array so that only first-occurred elements are kept. In ES6, the code is much simpler.
Dec 12, 2019 - For a while I have been thinking about a simple problem, why can’t we use .filter on array's in Javascript to get all the unique values in the array. The short answer is, you can! But I went on a short journey down JavaScript lane to find the best way to do this. 11/4/2019 · If you are looking for a solution for how to get a unique javascript array, then your answer is […new Set (arr)] method. The triple dot (…) is spread operator. Primitive values in JavaScript are immutable values except for objects. Types of primitive values include Null, Undefined, Boolean, Number, Symbol, and String.
Python Unique List How To Get All The Unique Values In A
How To Remove Array Duplicates In Es6 By Samantha Ming
How To Create An Array Of Unique Values In Javascript Using
How To Find Unique Objects In An Array In Javascript By
How To Find Unique Values By Property In An Array Of Objects
Javascript Array Distinct Ever Wanted To Get Distinct
How To Get Distinct Values From An Array Of Objects In
How To Remove Array Duplicates In Es6 By Samantha Ming
Javascript Array Distinct Ever Wanted To Get Distinct
C Program To Count Frequency Of Each Element In An Array
Javascript Array Distinct Values Code Example
Find Unique Elements In Array Java Javatpoint
How To Check All Values Of An Array Are Equal Or Not In
How To Get Values From Html Input Array Using Javascript
5 Easy Ways To Extract Unique Distinct Values
Getting Unique Values In Javascript Arrays By Raunaq
Javascript Array Find Unique Values
How To Get Unique Values In An Array In Javascript Dev
Get Only Unique Values From Array Javascript Code Example
Excel Vba Get Unique Values From Array Code Example
Numpy Find The Set Difference Of Two Arrays W3resource
5 Easy Ways To Extract Unique Distinct Values
How To Get Unique Values In An Array In Javascript Dev
Javascript Array Distinct Ever Wanted To Get Distinct
Python Unique List How To Get All The Unique Values In A
Getting Unique Values From Array Of Objects Code Example
Javascript Get All Non Unique Values From An Array
Javascript Get All Non Unique Values From An Array
Write C Program To Print All Unique Element In An Array
Filter An Array For Unique Values In Javascript Dev Community
How To Find Unique Values By Property In An Array Of Objects
Javascript Array Distinct Ever Wanted To Get Distinct
0 Response to "33 Javascript Find Unique Values In Array"
Post a Comment