32 Facade Design Pattern Javascript
The facade design pattern is one of the 23 GoF design patterns, which were published in 1994 by authors Erich Gamma, Ralph Johnson, Richard Helm, and John Vlissides in "Design Patterns: Elements of Reusable Object-Oriented Software" as guidance for software developers.In general, these patterns aim to simplify the creation of flexible, reusable software. 🎉 Ultra-simplified explanation to design patterns! 🎉. A topic that can easily make anyone's mind wobble. Here I try to make them stick in to your mind (and maybe mine) by explaining them in the simplest way possible. Based on "Design patterns for humans"
Understanding Design Patterns In Javascript
Sep 21, 2017 - Definition The Façade design pattern defines a unified, higher-level interface to wrap a complicated set of interfaces in a subsystem. It protects client code from sophisticated functionality in one or more subsystems by providing an interface or API to work with that is better and significantly ...
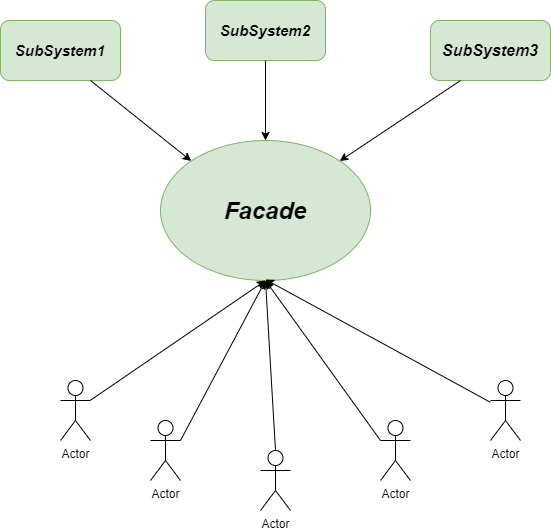
Facade design pattern javascript. Facade Pattern: Basic Idea. The facade pattern (also spelled façade) is a software-design pattern commonly used in object-oriented programming. Analogous to a facade in architecture, a facade is an object that serves as a front-facing interface masking more complex underlying or structural code. — Wikipedia. JavaScript Design Patterns: Facade. 2011/12/06. In our 4 th installment of the JavaScript Design Patterns Series, we'll be taking a look at the Facade pattern. Whether you know it or not, I can almost guarantee that you've used the Facade pattern if you've been programming in any language for more than a second (that might be a bit of a ... Jan 14, 2020 - There are 23 classic design patterns which are described in the original book Design Patterns: Elements of Reusable Object-Oriented Software. These patterns provide solutions to particular problems…
The Bridge Pattern. This pattern allows 2 components, a client and service to work together with each having its interface. The main goal is to write better code with two levels of abstraction. A good example of Bridge Pattern would be an application (client) and a database driver (service), the application writes to a well-defined database API, but you will find that each driver's ... The facade pattern is one of my favorite design patterns, because of how incredibly useful it is when refactoring code. The idea of the facade pattern is to ... :books: Educational list of Javascript Design Patterns - KleoPetroff/javascript-design-patterns
Aug 06, 2020 - We created an adapter object called personAdapter so that we can use backEnd to set all the attributes. The adapter has an interface that people can understand more easily since the method names are… A design pattern is a well-defined solution applied to commonly occurring problems in the software engineering industry. It is an illustration or a template for problem-solving that can be used in different conditions. Some of the problems solved by design patterns include: Creating classes. Instantiating an object. Some parts of facade need to carry some variables to javascript but in javascript I need assigned these values to computer. - Jaroslav Moravec Jul 4 '11 at 10:35 I have not real facade.
The facade pattern (also spelled façade) is a software-design pattern commonly used in object-oriented programming. Analogous to a facade in architecture, a facade is an object that serves as a front-facing interface masking more complex underlying or structural code. Javascript Application Design Patterns 4/23 Each Sandbox instance is now a Facade on its own; it handles interactions with adapter modules, e.g. lb.base.ajax for asynchronous communication with the host server. Simply put, Facade is a design pattern while Gateway is an architectural pattern. Application Gateway, for example, is an infrastructure architecture pattern. The node resides in DMZ and insulates internal nodes from external clients who can only connect to application gateway. When you think about architecture patterns, think about nodes.
Introduction. In this quick tutorial, we're going to take a look at one of the structural design patterns: the Facade. First, we'll give an overview of the pattern, list its benefits and describe what problems it solves. Then, we'll apply the facade pattern to an existing, practical problem with Java. 2. javascript design patterns. The Facade Pattern All the 23 (GoF) design patterns implemented in Javascript with Real World Example using Node.js APIs. javascript construction factory prototype resource composite design-patterns observer-pattern complex node-js gof concrete design-thinking factory-pattern gang-of-four facade-pattern composes software-architecture-and-design gang-of-four ...
JavaScript Facade Design Pattern with Example Code. Facade Pattern — a software design pattern commonly used with object-oriented programming. The name is by analogy to an architectural facade Prototype Design Pattern. The prototype design pattern lets us create clones of the existing objects. This is similar to the prototypal inheritance in JavaScript. All of the properties and methods of an object can be made available on any other object by leveraging the power of the __proto__ property.
They are named using different patterns, they are implemented better, worse, require more or less work. Because I didn't want to overcomplicate, I used simple examples with common response format. But nevertheless, this illustrates the problem well. Design of our facade To create a facade, first thing we need to know every aspect of every vendor. Facade Pattern. Facades are a structural pattern. It provides an interface, which shields clients from complex functionality. When we put up a facade, we present an outward appearance to the world, which may conceal a very different reality, inspiring the name — the facade pattern. ... Pro JavaScript Design Patterns, Authors: Diaz, Dustin ... To refresh our minds on how the ... design patterns together to form a system where: ... Developers have been requesting some sample code from the Aura framework that I've been building based on the concepts in this talk. Whilst the swappable mediator components and facade are still ...
Facade Design Pattern | Introduction. Facade is a part of Gang of Four design pattern and it is categorized under Structural design patterns. Before we dig into the details of it, let us discuss some examples which will be solved by this particular Pattern. So, As the name suggests, it means the face of the building. The Facade Pattern When we put up a facade, we present an outward appearance to the world that may conceal a very different reality. This was the inspiration for the … - Selection from Learning JavaScript Design Patterns [Book] The Decorator Pattern attach additional responsibilities to an object dynamically. Decorators provide a flexible alternative to subclassing for extending functionality. Example ... The Facade Pattern provides a unified interface to a set of interfaces in a subsystem.
Views: 20874. Abstract: In this tutorial, I will look to explain one of the simpler patterns - the Facade Design Pattern, how to implement it, and why it's still relevant in modern development in ASP.NET Core. Part of the development process is constructing code to produce value to clients when the solution is delivered. Facade: a superficial appearance or illusion of something: They managed somehow to maintain a facade of wealth. Very interesting design pattern I cam across which provides protection of code and ... We will be covering the Facade pattern shortly, but for reference purposes some developers may also wonder whether there are similarities between the Mediator and Facade patterns. ... We're now going to examine a variation of the Decorator first presented in a JavaScript form in Pro JavaScript Design Patterns (PJDP) by Dustin Diaz and Ross ...
JavaScript Facade Design Pattern with Example Code. The Mortgage object is the Façade in the example code. It presents a simple interface to the client with only a single method: applyFor.Eut underneath this simple API lies considerable complexity. Facade pattern hides the complexities of the system and provides an interface which the client can access the system. This type of design pattern adds an interface to the existing system to hide ... Jul 17, 2019 - In this article, we are going to talk about design patterns that can be and should be used to write better, maintainable JavaScript code. I assume you have a basic understanding of JavaScript and…
2021 JavaScript Design Patterns Source. ... Facade Pattern - Structural Design Provide a unified interface to a set of interfaces in a subsystem. Facade defines a higher-level interface that makes the subsystem easier to use. I'm actually fairly familiar with the use of a facade. I've been leveraging a pre-built facade on one of the projects I ... Feb 25, 2017 - A facade pattern can help in hiding the inner working of your module, and expose only the necessary public features/properties. ... This depends on the environment you will be building the JavaScript in, each comes with it’s own set of tools to help you get the job done. Design patterns are typical solutions to common problems. in software design. Each pattern is like a blueprint. that you can customize to solve a particular. design problem in your code. Dependency Injection (Simplified) Your palms are sweaty Knees weak, arms are heavy There's vomit on your sweater already, Dev's...
A Facade Pattern says that just "just provide a unified and simplified interface to a set of interfaces in a subsystem, therefore it hides the complexities of the subsystem from the client".. In other words, Facade Pattern describes a higher-level interface that makes the sub-system easier to use. Practically, every Abstract Factory is a type of Facade. Design Patterns - Facade Pattern. Facade pattern hides the complexities of the system and provides an interface to the client using which the client can access the system. This type of design pattern comes under structural pattern as this pattern adds an interface to existing system to hide its complexities. This pattern involves a single class ... Facade is a structural design pattern that provides a simplified interface to a library, a framework, or any other complex set of classes. Problem Imagine that you must make your code work with a broad set of objects that belong to a sophisticated library or framework.
The Facade pattern and applying it to React Hooks. 4. JavaScript design patterns #4. Decorators and their implementation in TypeScript. 5. JavaScript design patterns #5. The Observer pattern with TypeScript. The essential thing when approaching design patterns is to utilize them in a way that improves our codebase.
The Mediator Pattern Learning Javascript Design Patterns Book
Comprehensive Guide To Javascript Design Patterns Toptal
Facade Pattern From Head First Design Patterns
Appearance Pattern Of Java Design Pattern Facade Pattern
A Brief Guide To The Design Patterns In Javascript Edureka
Basic Javascript Design Patterns Decorators Facades And
Facade Method Python Design Patterns Geeksforgeeks
Javascript Facade Design Pattern Dofactory
Facade Design Pattern C Codeproject
The Bridge Amp Facade Design Patterns In Javascript By Mohit
Patterns For Large Scale Javascript Application Architecture
Github Jasancheg Js Design Patterns Into Practice Code
Javascript Facade Design Pattern Dofactory
Scalable Javascript Design Patterns
Design Patterns In Javascript Node 2020 By Shivam Gupta
Facade Method Python Design Patterns Geeksforgeeks
Understanding Design Patterns Facade In Javascript Using
Facade Design Pattern Codesolution Org
Design Patterns In Javascript Dev Community
Facade Design Pattern Still Relevant In Asp Net Core
Facade Pattern In Javascript Dev Community
Design Patterns In Javascript Node 2020 By Shivam Gupta
Javascript Design Patterns The Facade Pattern
Facade Pattern In Java Grokonez
0 Response to "32 Facade Design Pattern Javascript"
Post a Comment