31 How To Use Url In Javascript
You can use this function. function getParmFromUrl(url, parm) { var re = new RegExp(".*[?&]" + parm + "=([^&]+)(&|$)"); var match = url.match(re); return(match ? match[1] : ""); } The URL API is a clean interface for building and validating URLs with JavaScript. It's availabile in Node.js and in most modern browsers. The URL API offers a first layer of validation for URLs, although it doesn't enforce the TLD (top level domain).
How To Open Url In A New Tab With Javascript
How to build a simple URL shortener with just HTML and JavaScript. You might have used a URL shortener before, such as bit.ly, goo.gl. They are useful for shortening long URLs so that you can easily share them with your friends, family or co-workers. You might be wondering how these things work.
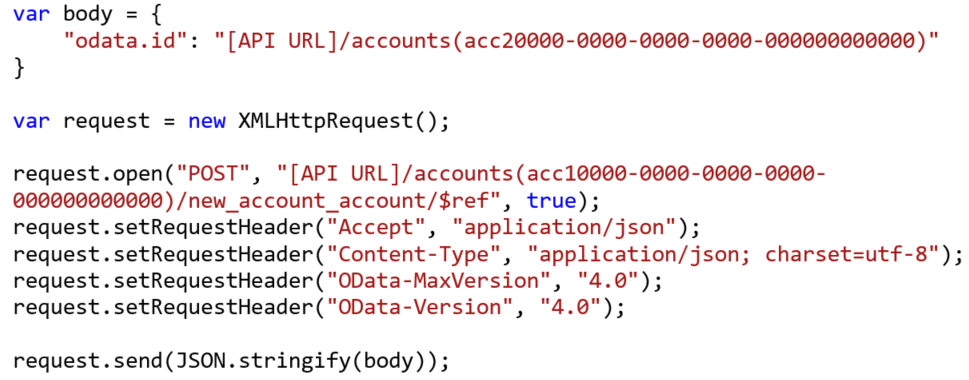
How to use url in javascript. JavaScript - Redirect a URL. Javascript Web Development Object Oriented Programming. To redirect a URL using JavaScript, the code is as follows −. In Javascript, window.location function is used to redirect to a URL. Below code takes an input url from the user and the url is stored in a variable through getElementById () and window.location takes the page to that url. document.write ("Redirecting to the url in 3 seconds..."); At first this will be the output, and then if the link like ... The downside of using the GET method is that it's visible to the visitor and it affects the link of the page. If you don't prefer this method, you can use the POST method, session Cookies or database to store the variables. The JavaScript function below parses and returns the parameters.
How to Get Current URL in JavaScript JavaScript suggests a bunch of methods that help to get the current URL displayed at the address bar. All of the methods use the Location object (contains information about the current URL), which is a property of the Window object (provides current page address (URL) and redirects the browser to a new page). The split () method first splits the url into an array of elements separated by the / then pop () method helps us to get the last element of an array (that is our url last segment). Similarly, we can also get the last segment by using the combination of substring (), lastIndexOf () methods. I just gave the button an id, and attached an onclick handler, and in that handler, when the button is clicked, it gets the value of the text input, creates the URL, as you can see in the variables, and then creates an image element, sets the src property of the image to the URL, and appends that image to the body.
Encoding a JavaScript URL can be done with two JavaScript functions depending on what you are going to do. These functions are: encodeURI () and encodeURIComponent () . Both are used to encode a URI by replacing each instance of certain characters by up to four escape sequences representing the UTF-8 encoding of the character. How to Turn an Object into Query String Parameters in JavaScript Unit Testing in JavaScript - Mocha, Chai and Sinon - a Beginner's Guide How to Copy an Object in JavaScript Given a URL and the task is to add an additional parameter (name & value) to the URL using JavaScript. URL.searchParams: This readonly property of the URL interface returns a URLSearchParams object providing access to the GET decoded query arguments in the URL. Syntax: var URLSearchParams = URL.searchParams;
Create a custom javaScript function with arguments parameter and assign it to a variable getQueryStringValue. Get the current page URL and assign to a variable currentPageURL Get all the query string and assign to a variable queryString Declare a for loop with queryString Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. How to get URL Parameters using JavaScript ? Method 1: Using the URLSearchParams Object: The URLSearchParams is an interface used to provide methods that can be used to work with an URL. The URL string is first separated to get only the parameters portion of the URL. The split () method is used on the given URL with the "?" separator.
However, sometimes there's a need to do the same using Javascript. In this case window.open() method proves to be helpful. The window.open() method is used to open a new browser window or a new tab depending on the browser setting and the parameter values. To know how to get query parameters using URL and URLSearchParams, refer the tutorial Get URL Parameters with Javascript. Edit / Update a Parameter The value of a parameter can be updated with the set () method of URLSearchParams object. After setting the new value you can get the new query string with the toString () method. The URL () constructor is handy to parse (and validate) URLs in JavaScript. new URL (relativeOrAbsolute [, absoluteBase]) accepts as first argument an absolute or relative URL. When the first argument is relative, you have to indicate the second argument as an abolsute URL that serves the base for the first argument.
If you want to simulate clicking on a link, use location.href If you want to simulate an HTTP redirect, use location.replace Note that location.replace does not keep the originating page in the session history. - Agnel Vishal Aug 23 '19 at 13:09 The location.href Method The location.href method is one of the most popular ways to perform JavaScript redirects. If you try to get the value of location.href, it returns the value of the current URL. Similarly, you can also use it to set a new URL, and users will then be redirected to that URL. In this video tutorial I'll be showing you guys how to use the URL interface in JavaScript in order to create, parse, manipulate and display URLs.URL objects...
There are times when this is not possible and you would need to use a JavaScript redirect to a URL. This is pretty simple, just include one of the following snippets: window.location.assign("new target URL"); window.location.replace("new target URL"); I would recommend using replace because the original URL is not valid. The decodeURIComponent () function is used to decode URL components encoding by encodeURIComponent () in JavaScript. It uses UTF-8 encoding scheme to perform the decoding operation. You should use decodeURIComponent () to decode query string parameters and path segments instead of full URLs. Here is an example: JavaScript has the APIs that allow you to redirect to a new URL or page. However, JavaScript redirection runs entirely on the client-side therefore it doesn't return the status code 301 (move permanently) like server redirection.
In this article, we'll show you how to parse and manipulate URL parameters using JavaScript. Getting a URL Parameter In modern browsers, this has become a lot easier, thanks to the ... 25/6/2019 · Parsing an URL. It is very simple to parse an URL in javascript by using DOM method rather than Regular expressions.If regular expressions are used then code will be much more complicated. In DOM method just a function call will return the parsed URL.. In the following example, initially a function is created and then an anchor tag "a" is created inside it using a DOM method. We can use a URL object in fetch or XMLHttpRequest, almost everywhere where a URL-string is expected. Generally, the URL object can be passed to any method instead of a string, as most methods will perform the string conversion, that turns a URL object into a string with full URL.
Is there a way to make the below script pass the javascript values to the url of the href link? <script type="text/javascript"> function geoPreview(lat,long) { var elemA = document.getElemen... JavaScript provides multiple methods to get the current URL displaying in web browser address bar. You can use the Location object property of the Window object to get these details. Below is the list of few properties of location object. Here is the list of options available to get URL and other details using JavaScript. Definition and Usage. The encodeURI () function is used to encode a URI. This function encodes special characters, except: , / ? : @ & = + $ # (Use encodeURIComponent () to encode these characters). Tip: Use the decodeURI () function to decode an encoded URI.
How to modify URL without reloading the page using JavaScript ? Method 1: Replacing the current state with replaceState () Method: The history interface of the browser manages the browser session history. It includes the page visited in the tab or frame where the current page is located. Manipulating this state can be used to change the URL of ... Using URL encoding, these characters are converted to special character sequences. In this article, you'll learn how to encode a URL using JavaScript built-in functions. JavaScript provides two functions to help you encode a URL: encodeURI () and encodeURIComponent (). Both of these methods are intended to be used for different use cases.
Vanilla Javascript To Get Url Parameter Values Query String
Can You Use Javascript To Get Url Parameter Values With Gtm
Encodeuricomponent Vps And Vpn
Get The Current Url With Javascript Stack Overflow
How To Get The Url Parameters In Javascript Brian Cline
Get The Current Url With Javascript Stack Overflow
Add Javascript Your Way Part 2
How To Get Attachments Url In All Folder Use Javascript
How To Run Javascript If A Link Is Clicked On Code Example
How To Add A Parameter To The Url In Javascript Geeksforgeeks
How To Use Python Scrapy To Crawl Javascript Dynamically
Can You Use Javascript To Get Url Parameter Values With Gtm
Axure 9 0 0 3723 Bug Report Open Url Javascript Function
Javascript Url Encode Example How To Use Encodeuricomponent
Javascript In Url From Arguments Not Working Developer
Failed To Use Content Url To Style An Element Stack Overflow
Check If The Url Bar Contains A Specified Or Given String In
4 Ways Javascript Can Redirect Or Navigate To A Url Or
How To Automate The Url Inspection Tool With Python Amp Javascript
Javascript Check If Url Equals String Code Example
Javascript And The Dynamics 365 Webapi For Beginners
How To Use History Api On Javascript By Javascript Jeep
Connect Jquery File To Nintex Form Nintex Community
Debugging In Visual Studio Code
Slash In Url Variable For Dynamic Paths By Marketalk Medium
Can You Use Javascript To Get Url Parameter Values With Gtm
How To Add A Parameter To The Url In Javascript Geeksforgeeks
0 Response to "31 How To Use Url In Javascript"
Post a Comment