31 Check Type Of Variable In Javascript
In Java, you can use instanceOfor getClass()on a variable to find out its type. How do I find out a variable's type in JavaScript which isn't strongly-typed? For example, how do I know if the baris a Booleanor a Number, or a String? Learn, how to find if a given variable is a string or not in JavaScript. The typeof operator. We can use the typeof operator to check if a given variable is a string. The typeof operator returns the type of a given variable in string format. Here is an example:
Monkey Raptor Javascript Checking Undefined Variable
In the first implementation of JavaScript, JavaScript values were represented as a type tag and a value. The type tag for objects was 0. null was represented as the NULL pointer (0x00 in most platforms). Consequently, null had 0 as type tag, hence the typeof return value "object".
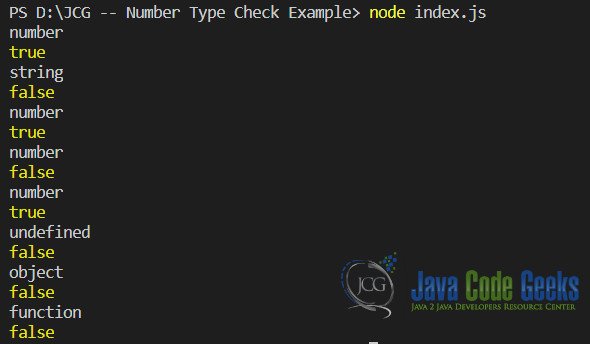
Check type of variable in javascript. Find the type of a variable - null, string, array etc. Javascript provides 2 operators to check the type of a given value : typeof : This checks whether the value is one of the primitive data types. It will return a string specifying the type — "undefined" / "string" / "number" / "boolean" / "object" etc. instanceof : This checks the "kind ... JavaScript has an operator called typeof that can be used to check the variable type during runtime. typeof returns a string of the value's data type, which can be convenient and necessary at times. The sample code below shows 4 examples of the typeof operator, the last two being special cases. JavaScript has a few built-in types, including numbers, strings, booleans, objects. Using the typeof operator we can check what is the type of a value assigned to a variable. For example: typeof 'test' Note that it's not a function, it's an operator, so parentheses are not required. Using it, we will get a string back, returning one of the following values: 'number' 'string' 'boolean ...
typeof is a JavaScript keyword that will return the type of a variable when you call it. You can use this to validate function parameters or check if variables are defined. There are other uses as well. The typeof operator is useful because it is an easy way to check the type of a variable in your code. The following types are considered as false. Null. Undefined. NaN. Empty string ("") Zero (0) So, we first check the type of a variable, and then if the value is of the above type, it has a false value. You put the value in the condition of the if statement. Based on the result, further actions are taken. In JavaScript, the typeof operator returns the data type of its operand in the form of a string. The operand can be any object, function, or variable. Syntax: typeof operand. OR typeof (operand) Note: Operand is an expression representing the object or primitive whose type is to be returned.
30/1/2016 · JavaScript comes with several built-in objects, including objects that correspond to four of the six primitive data types (Boolean, Number, String, Symbol). Use typeof to Check the Type of a Variable at Runtime. The typeof operator allows us to check the type of a variable at runtime. typeof returns "boolean" when applied to a variable of type boolean: JavaScript is a loosely-typed language, meaning that there is no restriction on what type a variable can have. Thus, sometimes, you have to check what type the variable has. In this tutorial, let's learn how to identify the type of a variable in JavaScript. In short, the answer in typeof. In JavaScript, the typeof operator returns the data type of its operand in the form of a string. The operand can be any object, function, or variable.
15/4/2019 · Variable is of function type Using Strict Equal (===) operator: In JavaScript, ‘===’ Operator is used to check whether two entities are of equal values as well as of equal type provides a boolean result. In this example, we use the ‘===’ operator. This operator, called the Strict Equal operator, checks if the operands are of the same type. The value type of a variable can change during the execution of a program and JavaScript takes care of it automatically. JavaScript Variable Scope. The scope of a variable is the region of your program in which it is defined. JavaScript variables have only two scopes. Global Variables − A global variable has global scope which means it can be ... A value in JavaScript is always of a certain type. For example, a string or a number. There are eight basic data types in JavaScript. Here, we'll cover them in general and in the next chapters we'll talk about each of them in detail. We can put any type in a variable. For example, a variable can at one moment be a string and then store a ...
Javascript Object Oriented Programming Programming In javascript we can check whether a variable is array or not by using three methods. 1) isArray () method The Array.isArray () method checks whether the passed variable is array or not. JavaScript Data Types. JavaScript variables can hold numbers like 100 and text values like "John Doe". In programming, text values are called text strings. JavaScript can handle many types of data, but for now, just think of numbers and strings. Strings are written inside double or single quotes. Numbers are written without quotes. Variable means anything that can vary. In JavaScript, a variable stores the data value that can be changed later on. Use the reserved keyword var to declare a variable in JavaScript.
The variable is not of function type The variable is of function type In the above program, the typeof operator is used with strict equal to === operator to check the type of variable. The typeof operator gives the variable data type. === checks if the variable is equal in terms of value as well as the data type. 10/11/2020 · JavaScript, however, is a loosely typed (or dynamically typed) language. This means that a variable can contain a value of any type. JavaScript code can execute like this: let one = 1; one = 'one'; one = true; one = Boolean(true); one = String('It is possible'); With this in mind, it is critical to know the type of a variable at any given time. The type of a variable is determined by the type of the value assigned to it. In JavaScript, different data types require distinct forms of checking. Strings, numbers, booleans and functions can be easily checked by using the typeof operator. For null and undefined, you can use a simple comparison with the strict equality operator. Arrays can be recognized by using the Array.isArray static method
javaScript check if variable is a number: isNaN () JavaScript - isNaN Stands for "is Not a Number", if a variable or given value is not a number, it returns true, otherwise it will return false. typeof JavaScript - If a variable or given value is a number, it will return a string named "number". In JavaScript, the typeof operator ... You can use the typeof operator to check the variable. It returns a string which indicates the type of the unevaluated operand. console .log( typeof '' === 'string' ); console .log( typeof function ( ) {} === 'function' ); console .log( typeof true === 'boolean' ); console .log( typeof Symbol () === 'symbol' ); console .log( typeof { key : … In JavaScript, strict equality comparison (===) Operator is used to check whether two entities are of not only equal values but also of equal type. The typeof operator returns a string which indicates the type of the unevaluated operand. Both of these operators provide a Boolean result.
The best way is to use the typeof keyword. typeof "hello" // "string" The typeof operator maps an operand to one of six values: "string", "number", "object", "function", "undefined" and "boolean". The instanceof method tests if the provided function's prototype is in the object's prototype chain. Use the typeof operator to get the type of an object or variable in JavaScript. In JavaScript, a variable can be either defined or not defined, as well as initialized or uninitialized. typeof myVar === 'undefined' evaluates to true if myVar is not defined, but also defined and uninitialized. That's a quick way to determine if a variable is defined.
The quickest and accurate way to check if a variable is an object is by using the Object.prototype.toString () method. This method is part of Object 's prototype and returns a string representing the object: Object. prototype.toString.call( fruits); Object. prototype.toString.call( user); As you can see above, for objects, the toString ... The Concept of Data Types. In programming, data types is an important concept. To be able to operate on variables, it is important to know something about the type. Without data types, a computer cannot safely solve this:
Use Typeof To Check Javascript Data Types At Runtime
How To Check If A Variable Is A Function In Javascript
Get More Data About A User With Javascript Variable
How To Declare A Variable In Javascript With Pictures Wikihow
Understanding Javascript Data Types And Variables By
Javascript Check If A Variable Is A String Geeksforgeeks
Get Started With Debugging Javascript In Microsoft Edge
How To Check If A Variable Is A Function In Javascript
Check If Variable Is Exists In Javascript Mkyong Com
Javascript Mcq Multi Choice Questions Javatpoint
Variables In Javascripts The Engineering Projects
Javascript Variables A To Z Guide For A Newbie In
Javascript Programming With Visual Studio Code
Javascript Typeof Understanding Type Checking In Javascript
Debugging Freecodecamp Freya Yuki
Error Flow Functions Not Working With App Variable Appgyver
How To Store Data In Javascript Variables Dummies
A Quick Overview Of Regression Algorithms In Machine Learning
Identify If A Variable Is An Array Or Object In Javascript
How To Check Data Types In Javascript Using Typeof By Dr
How To Check If A Variable Exists Or Is Defined In Javascript
Javascript Typeof How To Check The Type Of A Variable Or
How To Check If Something Is An Array Weekly Webtips
Write Your Code In Low Code No Code Platform Sap Blogs
How To Find Type Of Variable In Javascript Poopcode
How Can I Check Property In Object With Variable In Loop
Javascript Check If Variable Is A Number Example Java Code
0 Response to "31 Check Type Of Variable In Javascript"
Post a Comment