22 Javascript Array Replace Element At Index
The arr.splice method is a swiss army knife for arrays. It can do everything: insert, remove and replace elements. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Array Prototype Splice Javascript Mdn
// Replace 1 array element at index with item arr.splice(index,1,item);
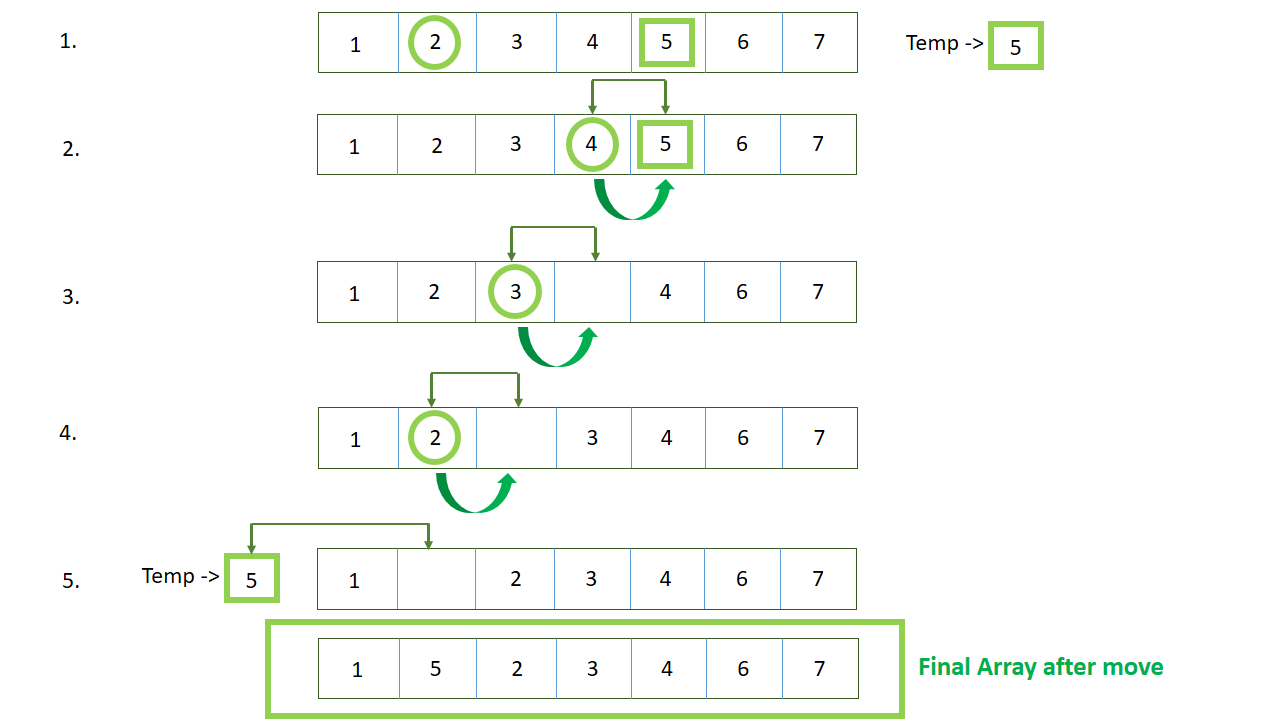
Javascript array replace element at index. ECMAScript 3rd Edition (ECMA-262) Standard. Initial definition. Implemented in JavaScript 1.2. ECMAScript 5.1 (ECMA-262) The definition of 'Array.prototype.splice' in that specification. Standard. ECMAScript 2015 (6th Edition, ECMA-262) The definition of 'Array.prototype.splice' in that specification. Get the array and the index. Form an ArrayList with the array elements. Remove the specified index element using remove() method. Form a new array of the ArrayList using mapToInt() and toArray() methods. Return the formed array. Below is the implementation of the above approach: You can simply set up a a new array as such: const newItemArray = array.slice (); And then set value for the index which you wish to have a value for. newItemArray [position] = newItem
Replacing elements at different positions of an array · Splice() is a powerful method in JavaScript array handling and it almost gives all type of element handling inside an array. We can add new element, we can remove some element, we can create new array by using (or taking) elements from ... Dec 17, 2019 - JavaScript, like any other programming language, has many handy tricks that let us write our programs more easily. In this article, we will look at how to do different things that involve arrays… JavaScript Array type provides a very powerful splice () method that allows you to insert new elements into the middle of an array. However, you can use this method to delete and replace existing elements as well. Deleting elements using JavaScript Array's splice () method
index: Required. The position to add/remove items. Negative values a the position from the end of the array. howmany: Optional. Number of items to be removed. item1, ..., itemX: Optional. New elements(s) to be added To remove elements or items from any position in an array, you can use the splice() array method in JavaScript. Jump to full code; Consider this array of numbers, // number array const numArr = [23, 45, 67, 89]; What if we need to remove the element at index 1 in the array, in our case 45. We can do that with the splice() array method. The ... 15/7/2019 · An item can be replaced in an array using two approaches: Method 1: Using splice () method. The array type in JavaScript provides us with splice () method that helps us in order to replace the items of an existing array by removing and inserting new elements at the required/desired index. …
The pop() and shift() methods change the length of the array.. You can use unshift() method to add a new element to an array.. splice()¶ The Array.prototype.splice() method is used to change the contents of an array by removing or replacing the existing items and/or adding new ones in place. The first argument defines the location at which to begin adding or removing elements. The splice()method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place. N.B :In case you're working with reactive frameworks, it will update the "view", your array "knowing" you've updated it. 7/5/2011 · Well if anyone is interresting on how to replace an object from its index in an array, here's a solution. Find the index of the object by its id: const index = items.map(item => item.id).indexOf(objectId) Replace the object using Object.assign() method: …
Array indexes start from 0, so if you want to add the item first, you'll use index 0, in the second place the index is 1, and so on. To perform this operation you will use the splice () method of an array. This function is very powerful and in addition to the use we're going to make now, it also allows to delete items from an array. 20/8/2021 · Replace item in array using Splice. Another way to replace an item in an array is by using the JavaScript splice method. The splice function allows you to update an array's content by removing or replacing existing elements. As usual, if you want to replace an item, you will need its index. Here are the parameters you will use with splice: Zero or more values to be inserted into array, beginning at the index specified by start. ... An array containing the elements, if any, deleted from array. ... splice( ) deletes zero or more array elements starting with and including the element start and replaces them with zero or more values ...
We can use the spice () method on our array which is used to add and remove elements from an array. This method takes the first argument as an index which specifies the position of the element to be added or removed. The next argument it takes is the number of elements to be removed and is optional. In JavaScript, the Array.splice () method can be used to add, remove, and replace elements from an array. This method modifies the contents of the original array by removing or replacing existing elements and/or adding new elements in place. Array.splice () returns the removed elements (if any) as an array. Aug 25, 2020 - // Replace 1 array element at index with item arr.splice(index,1,item);
In this tutorial, you will learn how to use the JavaScript Array splice() method to remove existing elements, add new elements, and replace elements in an array. Here, you will take a look at examples of the JavaScript array splice() method for better demonstration.. JavaScript splice() Definition:-The javascript splice() method is used to add, remove and replace elements from an array. javascript: how to remove a section of the middle of an array string and replace it with something else ... Install and run react js project... ... Error: Node Sass version 5.0.0 is incompatible with ^4.0.0. ... Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin ... Definition and Usage. The findIndex() method returns the index of the first array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, findIndex() returns the index of that array element (and does not check the remaining values)
It basically stores different elements in one box and can be later assesssed with the variable. Declaring an array: let myBox = []; // Initial Array declaration in JS. Arrays can contain multiple data types. let myBox = ['hello', 1, 2, 3, true, 'hi']; Arrays can be manipulated by using several actions known as methods. 7/7/2020 · Array.splice will modify your original array and return the removed elements so you can do the following: const arr = [1,2,3,4,5]; const index = arr.indexOf(2); const splicedArr = arr.splice(index,1); arr; // [1,3,4,5]; splicedArr; // [2] Enter fullscreen mode. Exit fullscreen mode. Sep 10, 2019 - I am trying to replace each element in the left array with the elements in the right array of equivalent position but in a case where I encounter duplicate value, assign the same value to both … @tickct ... I would suggest looking at the findIndex function of arrays. It returns the index of the ...
this.state.images.slice (0, index) is a new array has items start from 0 to index - 1 (index is not included) this.state.images.slice (index) is a new array has items starts from index and afterwards. To correctly replace item at index 4, answer should be: The index at which to start changing the array. If greater than the length of the array, start will be set to the length of the array. In this case, no element will be deleted but the method will behave as an adding function, adding as many element as item [n*] provided. If negative, it will begin that many elements from the end of the array. The array.splice () method is usually used to add or remove items from an array. This method takes in 3 parameters, the index where the element id is to be inserted or removed, the number of items to be deleted and the new items which are to be inserted. The only insertion can be done by specifying the number of elements to be deleted to 0.
// Replace 1 array element at index with item arr.splice(index,1,item); In this snippet, we are going to discuss a question concerning JavaScript array. Today's topic covers the insertion of an item into the existing array at a specific index. However, there is no inbuilt method that directly allows inserting an element at any arbitrary index of the array. One of the methods is the splice function. To replace element at specified index, use ArrayList.set (int index, E element) method. 1. ArrayList.add (int index, E element) - Add element at specified index This method inserts the specified element E at the specified position in this list.
Nov 18, 2020 - If you don’t know the index of the item, you might need to first find the index of the item in the array. Download my free JavaScript Beginner's Handbook and check out my JavaScript Masterclass! There are different methods and techniques you can use to remove elements from JavaScript arrays: pop - Removes from the End of an Array shift - Removes from the beginning of an Array splice - removes from a specific Array index A Javascript tip for searching the index of an element in an array, and then delete or replace it. A Javascript tip for searching the index of an element in an array, and then delete or replace it
May 06, 2021 - Learn more about Insert, Remove, Splice and Replace elements with Array.splice() from DevelopIntelligence. Your trusted developer training partner. Get a customized quote today: (877) 629-5631. Let's begin with a simple approach, using an array of char. Here, the idea is to convert the String to char[] and then assign the new char at the given index. Finally, we construct the desired String from that array.. public String replaceCharUsingCharArray(String str, char ch, int index) { char[] chars = str.toCharArray(); chars[index] = ch; return String.valueOf(chars); } In order to replace an element we need to know its index, so let's see some examples using the methods we just learned: const arr = [1, 2, 3, 4, 5] const index = arr.indexOf(2) arr[index] = 0
In the above example, we removed seahorse from the array, and pushed a new value into index 3. Looping Through an Array. We can loop through the entirety of the array with the for keyword, taking advantage of the length property. In this example, we can create an array of shellfish and print out each index number as well as each value to the ... The splice () function in JavaScript is used to remove or replace elements in an array at a specified range of keys. The splice () Syntax Before we jump into some examples, let's look at the splice () syntax to understand how it works. removed_elements = array.splice (index, count, item1, item2)
How To Work With Arrays In Ruby Digitalocean
Why Can T You Modify Lists Through For In Loops In Python
Javascript Array Findindex Method
React Reactjs Update An Array State
Remove Element From Array Javascript First Last Value
More Javascript Array Tips Replacing And Mapping Entries
How To Move An Array Element From One Array Position To
Javascript Array Indexof And Lastindexof Locating An Element
How To Add Remove And Replace Items Using Array Splice In
Creating Indexing Modifying Looping Through Javascript
Python Arrays Create Update Remove Index And Slice
How To Replace An Item From An Array In Javascript
Insert An Element In Specific Index In Javascript Array By
Javascript Array A Complete Guide For Beginners Dataflair
How To Move An Array Element From One Array Position To
Javascript Array Splice How To Splice Array In Javascript
Arraylist In Java With Example Programs Collections Framework
How To Insert An Item Into Array At Specific Index In
How To Replace An Element In Array In Java Code Example
0 Response to "22 Javascript Array Replace Element At Index"
Post a Comment