29 Get Element Attribute Javascript
I need a way to find this element by ID, similar to document.getElementById(), but instead of using a general ID, I want to search for the element using my custom "tokenid" attribute. Something like this: To get attributes of an HTML Element using JavaScript, get reference to this HTML Element using a selector, and read the attributes property of this HTML Element. In the following example, we have HTML Element with id "myElement", and we shall get the attributes of this HTML Element in JavaScript by reading this element's attributes property.
A Complete Guide To Data Attributes Css Tricks
Syntax: var elementVar = document.getElementById ("element_id"); elementVar.setAttribute ("attribute", "value"); So what basically we are doing is initializing the element in JavaScript by getting its id and then using setAttribute () to modify its attribute. Example: Below is the implementation of above approach. <!DOCTYPE html>.
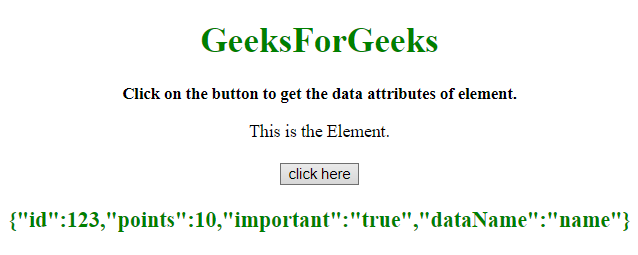
Get element attribute javascript. First, select the element which is having data attributes. We can either use dataset property to get access to the data attributes or use.getAttribute () method to select them by specifically typing their names. Example 1: This example uses dataset property to get the data attributes of an element. We can get all attributes of an element with JavaScript by using the node.attributes property. Also, we can use the jQuery each method to loop through all the attributes and the attribute name as the property name and the attribute value as the value of the property. JavaScript getAttribute () method The getAttribute () method is used to get the value of an attribute of the particular element. If the attribute exists, it returns the string representing the value of the corresponding attribute. If the corresponding attribute does not exist, it will return an empty string or null.
Thus, you can use element.dataset.employeeId to fetch the value of the data-employee-id attribute. So that's how the dataset properly works in vanilla JavaScript. The getAttribute () Method In this section, we'll discuss how you can use the getAttribute method to get the value of data attributes. The attributes collection is iterable and has all the attributes of the element (standard and non-standard) as objects with name and value properties. Property-attribute synchronization. When a standard attribute changes, the corresponding property is auto-updated, and (with some exceptions) vice versa. Using Selenium and JavaScript, you can get the data of the attributes of the HTML elements displayed in the current state on the website (or web application). In addition, you can also verify the changed data of the attributes once the occurrence of events changes in the properties (e.g. colour, link updates for anchor tags, etc.) of the ...
The .attr() method gets the attribute value for only the first element in the matched set. To get the value for each element individually, use a looping construct such as jQuery's .each() or .map() method.. Using jQuery's .attr() method to get the value of an element's attribute has two main benefits:. Convenience: It can be called directly on a jQuery object and chained to other jQuery methods. JavaScript provides several methods for adding, removing or changing an HTML element's attribute. In the following sections we will learn about these methods in detail. Getting Element's Attribute Value. The getAttribute() method is used to get the current value of a attribute on the element. If the specified attribute does not exist on the ... Definition and Usage The getAttribute () method returns the value of the attribute with the specified name, of an element. Tip: Use the getAttributeNode () method if you want to return the attribute as an Attr object.
Getting Element's Attribute Value; Setting Attributes on Elements; Removing Attributes from Elements; Getting Element's Attribute Value. The method is used to get the current value of the attribute on the element is called getAttribute( ). If the element does not exist in a specified attribute, it will return or show null. As shown in the ... Now, we need to select the above elements by data attribute in JavaScript. Selecting the Single element To select the single element, we need to use document.querySelector () method by passing a [data-attribute = 'value'] as an argument. getAttribute( ) returns the value of a named attribute of an element. Note that the HTMLElement object defines JavaScript properties that match each of the standard HTML attributes, so you need to use this method with HTML documents only if you are querying the value of nonstandard attributes.
To get a data attribute through the dataset object, get the property by the part of the attribute name after data- (note that dashes are converted to camelCase). const article = document.querySelector('#electric-cars'); article. dataset. columns article. dataset. indexNumber article. dataset. parent The getAttribute () method of the Element interface returns the value of a specified attribute on the element. If the given attribute does not exist, the value returned will either be null or "" (the empty string); see Non-existing attributes for details. The function I wrote for this is as follows: function getElements (attrib) { // get all dom elements var elements = document.getElementsByTagName ("*"); // initialize array to put matching elements into var foundelements = []; // loop through all elements in document for (var i = 0; i < elements.length; i++) { // check to see if element has any ...
The setAttribute () method adds the specified attribute to an element, and gives it the specified value. If the specified attribute already exists, only the value is set/changed. Note: Although it is possible to add the style attribute with a value to an element with this method, it is recommended that you use properties of the Style object ... The querySelector () is a method of the Element interface. The querySelector () allows you to find the first element that matches one or more CSS selectors. You can call the querySelector () method on the document or any HTML element. The following illustrates the syntax of the querySelector () method: In this syntax, the selector is a CSS ... In this article, we looked at different ways to get CSS styles from an element using JavaScript. You should use: The DOM style property to retrieve inline styles applied to an element using the style attribute. The window.getComputedStyle() method to retrieve computed styles applied to an element through <style> elements and external style ...
Get code examples like"javascript get element by rel attribute". Write more code and save time using our ready-made code examples. Here's how to get element in a HTML. Get Current Script Element document.currentScript Return the current script element. [see DOM: Get Current Script Element] Get Element by Matching the Value of the "id" Attribute document.getElementById(id_string) Return a non-live element object. Returns null if not found. javascript. Updated on April 13, ... Just like jQuery's $.attr() method, there are native browser methods that can get and set attributes of an element. Checking whether an Attribute Exists. The hasAttribute method can tell whether the element has the given attribute or not. A true is returned if the element has the attribute, ...
The JavaScript. DOM elements have an attributes property which contains all attribute names and values: Using Array.prototype.slice.call, which is also helpful in converting NodeLists to Arrays, you can convert the result to a true array which you can iterate over: The attributes property of an element is incredibly handy when you're looking to ... document.getElementById or just id If an element has the id attribute, we can get the element using the method document.getElementById (id), no matter where it is. The JavaScript getElementByName () is a dom method to allows you to select an element by its name. The following syntax to represents the getElementsByName () method: 1 let elements = document.getElementsByName (name);
If the attribute exists on the element, the getAttribute () returns a string that represents the value of the attribute. In case the attribute does not exist, the getAttribute () returns null. Note that you can use the hasAttribute () method to check if the attribute exists on the element before getting its value. There are a couple of ways you can do this using Vanilla Javascript: Get the element by its id using the Javascript document.getElementById function. Or grab the element by a dataset itself using the Javascript document.querySelector function. Let's take a look at that in the code.
Methods For Accessing Elements In The Dom File With
How To Get Element Attribute Value In Javascript Code Example
Javascript Tutorial For Beginners 35 Changing Element Attributes
Javascript Dom Tutorial 2 Get Element By Id
Custom Attributes Webflow University
Get Attribute Of Element In Rendered Ui With Javascript In
Capturing The Correct Element In Google Tag Manager Simo
Create Ul And Li Elements Dynamically Using Javascript
The Css Attr Function Got Nothin On Custom Properties
How To Get The Data Attributes Of An Element Using Javascript
Javascript Set Custom Attribute To Element Mywebtuts Com
Semalt Element Attributes Everything You Need To Know
Introduction To Web Scraping Selecting Content On A Web Page
How To Add Update An Attribute To An Html Element Using
Use Angular Component As Element Attribute And Class
View And Change Css Chrome Developers
How To Get Html Attribute Value In Cypress Stack Overflow
Html Attribute Vs Dom Property Dot Net Tutorials
Javascript Change Disabled Attribute Dynamically Disabled
Javascript Get Element By Id Name Class Tag Value Tuts Make
Vue Js Get Element By Id Attribute Infinityknow
How To Modify Attributes Classes And Styles In The Dom
Webelement Operations In Puppeteer
How To Get The Data Attributes Of An Element Using Javascript
Dom Element Variable In Google Tag Manager Analytics Mania
0 Response to "29 Get Element Attribute Javascript"
Post a Comment