29 Mongodb Javascript Use Database
An introduction into MongoDB using Javascript MongoDB is a NoSQL database that stores data in flexible, JSON like documents which means that fields can vary from document to document and data structure can be changed over time. In our modern world data is vast, unstructured, and sometimes unwieldy. A perhaps easier alternative to using the MongoDB Native API is using Mongoose API. In the next tutorial , we will learn how to implement relational database functionality with Mongoose. This tutorial might seem long and incredibly tedious,but this topic is incredibly easy to wrap your head around if you apply the examples in your code.
Mongodb For Node Js Developers Allen S Blog
Dec 21, 2011 - Instead you will need to explicitly define the database in the connection (/dbname in the example above). Alternately, you can also create a connection within the script: ... The answer below is the correct one. For an overview of the difference between interactive and scripted JS: docs.mongodb.c...
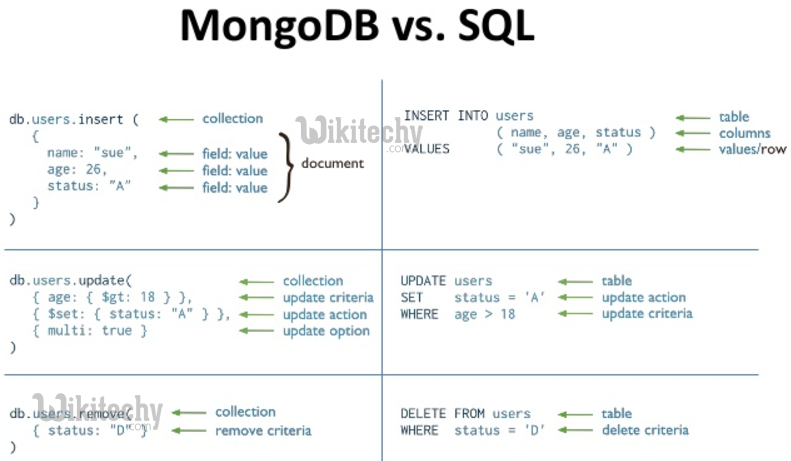
Mongodb javascript use database. Nov 24, 2018 - In this tutorial I'll show you how to interact with a MongoDB database from Node.js This tutorial is helpful to create your own Database, collections(tables), document(tuple/row) in the Mongobooster either directly or by using Node.js. Dec 02, 2019 - Introduction to MongoDB and JavaScript ... a MongoDB database for testing JavaScript code Executing a JavaScript file using the Mongo Shell interface ‘Console not defined’ exception when executing Javascript with MongoDB Create a JavaScript file for MongoDB Use Mongo Shell’s ...
How do I programmatically create a database using the MongoDB Node.JS driver? This looks promising, but I'm not sure how to connect to with the admin credentials and create a new database. ... Browse other questions tagged javascript node.js mongodb or ask your own question. The Overflow Blog Podcast 367: Extending the legacy of Admiral Grace ... Page shows up on the client's browser. Step 1. The missing step for you is (1), because the server is not up and running. This is done by typing the following on your terminal: $ node name_of_file_here.js. If there are errors in your syntax, or missing dependencies the console will log the errors. Create Connection Create Database Create Collection MongoDB Insert MongoDB Select MongoDB Query MongoDB Sorting MongoDB Remove ... Node.js Interview AngularJS Interview JavaScript Interview jQuery Interview Backbone.js Interview Ember.js Interview Neo4j Interview
MongoDB : An introduction. MongoDB, the most popular NoSQL database, is an open-source document-oriented database. The term 'NoSQL' means 'non-relational'. It means that MongoDB isn't based on the table-like relational database structure but provides an altogether different mechanism for storage and retrieval of data. The use Command. MongoDB use DATABASE_NAME is used to create database. The command will create a new database if it doesn't exist, otherwise it will return the existing database. Syntax. Basic syntax of use DATABASE statement is as follows −. use DATABASE_NAME Example. If you want to use a database with name <mydb>, then use DATABASE ... Nov 17, 2019 - MongoDB University Free Course: M220JS: MongoDB for Javascript Developers · Questions? Comments? We'd love to connect with you. Join the conversation on the MongoDB Community Forums. All posts in the Quick Start: Node.js and MongoDB series: How to connect to a MongoDB database using Node.js ...
JavaScript in MongoDB Although these methods use JavaScript, most interactions with MongoDB do not use JavaScript but use an idiomatic driver in the language of the interacting application. If you do not need to perform server-side execution of JavaScript code, see Disable Server-Side Execution of JavaScript . Querying for data in a MongoDB database - Using the MongoDB driver we can also fetch data from the MongoDB database.. The below section will show how we can use the driver to fetch all of the documents from our Employee collection in our EmployeeDB database. This is the collection in our MongoDB database, which contains all the employee-related documents. Jul 15, 2020 - Create a MongoDB Database in Node.js. Understand MongoDB & language compatibility. Learn to import data, use MongoDB driver for node.js & Node.js based CRUD operations.
A schema is the structure of the database, defined as tables in case of RDBs. Since document-oriented databases do not use such tables, they are essentially structure-less, or schemaless. As for BSON, well, that stands for Binary JSON, and it's the data storage format for the MongoDB database. That's all you need to know about it at this stage. Run this file to create database. Database. Check MongoDB again to see the available databases. See, a database javatpoint is created. Collection. See, the created collection employee. Record. See the inserted record. Well, we can see that the Java program is executing fine and we can also perform other databases operations as well. And it's a wonderful feature of MongoDB that you can actually insert a file to execute the command. Let's see how we can do this. Create any javascript file, I'll name it findByEmail.js. and insert this data into it. To execute this file, run the following command: mongo blog < findByEmail.js.
Express apps can use any database supported by Node (Express itself doesn't define any specific additional behavior/requirements for database management). There are many popular options, including PostgreSQL, MySQL, Redis, SQLite, and MongoDB.. When choosing a database, you should consider things like time-to-productivity/learning curve, performance, ease of replication/backup, cost, community ... May 25, 2017 - I am able to drop database using node.js but I dont know how to create new database? I want to create monogo database using node.js. Can someone help me about how to create mongo database using nod... Open a console and navigate to the code/hour03 directory. 6. Execute the following command to run the JavaScript file created in Steps 2 and 3. Listing 3.4 shows the output of the script. mongo functions.js. LISTING 3.3 functions.js: Creating and Using JavaScript Functions in a MongoDB Shell Script.
Feb 13, 2015 - Next up, we’ll take a look at how to use this data in our site, by connecting MongoDB to Node.js. ... Author of "Getting MEAN" and "Mongoose for Application Development". Lover of JavaScript development. Founder of Full Stack Training. ... Having a node.js website without a database in the ... MongoDB Query Document: db.collection.find () with Example. The method of fetching or getting data from a MongoDB database is carried out by using MongoDB queries. While performing a query operation, one can also use criteria's or conditions which can be used to retrieve specific data from the database. MongoDB provides a function called db ... In this tutorial, we walk you through seven steps for developing a RESTFul API using popular JavaScript frameworks such as Node.JS and Express.JS. In doing so, we learn how to integrate our API with the MongoDB database. Here are the main steps: 1. Project Initialization. 2. Install Application Dependencies.
MongoDB sharding has some limitations: not all operations work with it, and a bad design on shard-keys can decrease query performance, create unevenly distributed data, and impact cluster internal operation as automatic data splitting, and on a worse scenario demanding manual re-sharding, which is an extensive and error-prone operation. MongoDB provides an interactive JavaScript interface called the mongo shell that you can use to connect to a running MongoDB instance from your command-line terminal.. The mongo shell can be used to query and update data as well as to perform administrative tasks. It is already included as part of the MongoDB installation package, so you don't need to install anything. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... MongoDB Get Started MongoDB Create Database MongoDB Create Collection MongoDB Insert MongoDB Find MongoDB Query MongoDB Sort MongoDB Delete MongoDB Drop Collection MongoDB ...
You must run some commands on the admin database. Normally, these operations resemble the following: However, there's also a command helper that automatically runs the command in the context of the ... © MongoDB, Inc 2008-present. MongoDB, Mongo, and the leaf logo are registered trademarks ... In this case, the official MongoDB ... from MongoDB and is easy to use. Installing it in your Node.js project is as simple as running the following command: ... The driver makes connecting to your database, authentication, CRUD operations, and even logging/monitoring as simple as running a command. The driver also supports JavaScript promise/async ... The Databases tab in MongoDB Compass has a Create Database button. In MongoDB Compass, you create a database and add its first collection at the same time: Click Create Database to open the dialog. Enter the name of the database and its first collection. Click Create Database. Find out more here.
Mongoose has a very simple Model.find function for MongoDB queries in Node.js, as shown below. In this case the model is Comment, as defined using the var statement near the end.'UserName' in the Comment.find statement is just the field you're searching by. So {UserName: 'Nick'} is just instructing it to search for comments made under the username 'Nick'. Creating a Database. To create a database in MongoDB, start by creating a MongoClient object, then specify a connection URL with the correct ip address and the name of the database you want to create. MongoDB will create the database if it does not exist, and make a connection to it. Create and use a MongoDB database with Azure portal. Use the Azure portal to create a Cosmos DB API for MongoDB. On the Basics page, make sure you select the version of MongoDB you intend to use. Learn more about MongoDB versions: 4.0; 3.6; 3.2; Once the resource is created, use the Data Explorer for your resource to create a new database and ...
Populating MongoDB database would be much easier, if you used JavaScript, or even TypeScript for import data definition. That's possible — just pick Mongo Seeding , presumably the most ... We use the mongodb module. const client = mongo.MongoClient; MongoClient is used to connect to the MongoDB server. const url = 'mongodb://localhost:27017'; This is the URL to the database. The 27017 is the default port on which the MongoDB server listens. MongoClient.connect (url, { useNewUrlParser: true }, (err, client) => {. Nov 29, 2017 - Node.js Create Database in MongoDB : Learn to create a MongoDB database from Node.js Application using mongodb package, with the help of an Example.
As we see the number type can be ... in Javascript. The latest driver will do correct conversion up to 53 bits of complexity. If you need to handle big integers the recommendation is to use the Long class to operate on the numbers. ... Let’s get around to setting up a connection with the Mongo DB database... This course will teach you how to use MongoDB as the database for a Node.js application. You will play the role of a back-end developer for a Node.js application, where your job is to implement the application's communication with MongoDB. Using the Node.js driver you will read and write data to the database, use the aggregation framework ... If connecting to a MongoDB instance that enforces access control, you can use the db.auth() method to authenticate.. Additionally, you can use the connect() method to connect to the MongoDB instance. The following example connects to the MongoDB instance that is running on localhost with the non-default port 27020 and set the global db variable:
Mongo Script - Learn to execute multiple commands from a JavaScript file using mongo program, with the help of an example. This is a short tutorial and an example app which reads data from a MongoDB database. The app is using MongoDB, Express.js and Node.js. I'm using Apple macOS High Sierra 10.13.4 (17E199), Node.js…
Express Tutorial Part 3 Using A Database With Mongoose
Node Js Application Writing To Mongodb Kafka Streams
Node Js Node Js Mongodb Insert Record By Microsoft Award
How To Build Crud Application Using Node Js And Mongodb By
Creating A Database In Mongodb Bmc Software Blogs
How Developers Use Node Js Survey Results Risingstack
Crud Create Read Update Delete Operations On Nosql
How To Create A Simple Restful Api Using Nodejs Expressjs
Using Mongodb As A Realtime Database With Change Streams By
Using Mongodb As A Realtime Database With Change Streams
Angular 10 Server Side Pagination In Nodejs Mongodb Example
Node Js Mongodb User Authentication Amp Authorization With
Building A Restful Api Using Node Js And Mongodb Nordic Apis
How To Connect Mongodb Atlas With Node Js Using Mongoose
Using A Database With Node Js Mongodb
Fetch Data From Mongodb Using Mongoose And Node Js Express
Working With Mongodb In Visual Studio Code
5 Insert Data In Mongodb Database Using Node Js Codez Up
How To Use Mongodb Mongoose With Node Js Best Practices
Chapter 6 Writing A Rest Api Exposing The Mongodb Database
Mongo In Node Js Second Part Inmediatum
Query Code Mongodb To Node Js Java C Python Php Ruby
How To Create Mongodb Database In Node Js
Connect A Mongodb Application To Azure Cosmos Db Microsoft Docs
Read Data From Mongodb With Queries
Node Js Mongodb Tutorial How To Build Crud Application
How To Use Mongodb Mongoose With Node Js Best Practices
How To Use Express Js Node Js And Mongodb Js
0 Response to "29 Mongodb Javascript Use Database"
Post a Comment