28 Javascript Anonymous Function Syntax
A function expression is similar to and has the same syntax as a function declaration (see function expression for details). A function expression may be a part of a larger expression. One can define "named" function expressions (where the name of the expression might be used in the call stack for example) or "anonymous" function expressions. 27/6/2021 · Using Anonymous function as a value to a function. So, we can use an anonymous function as a value to be assigned to a variable: let someFunction = function() => {// Body of the function.}; The above example demonstrates the anonymous function being assigned to a variable. In JavaScript, functions can be created in multiple ways.
An Immediately-invoked Function Expression (IIFE for friends) is a way to execute functions immediately, as soon as they are created.. IIFEs are very useful because they don't pollute the global object, and they are a simple way to isolate variables declarations.. This is the syntax that defines an IIFE: (function { /* */})()
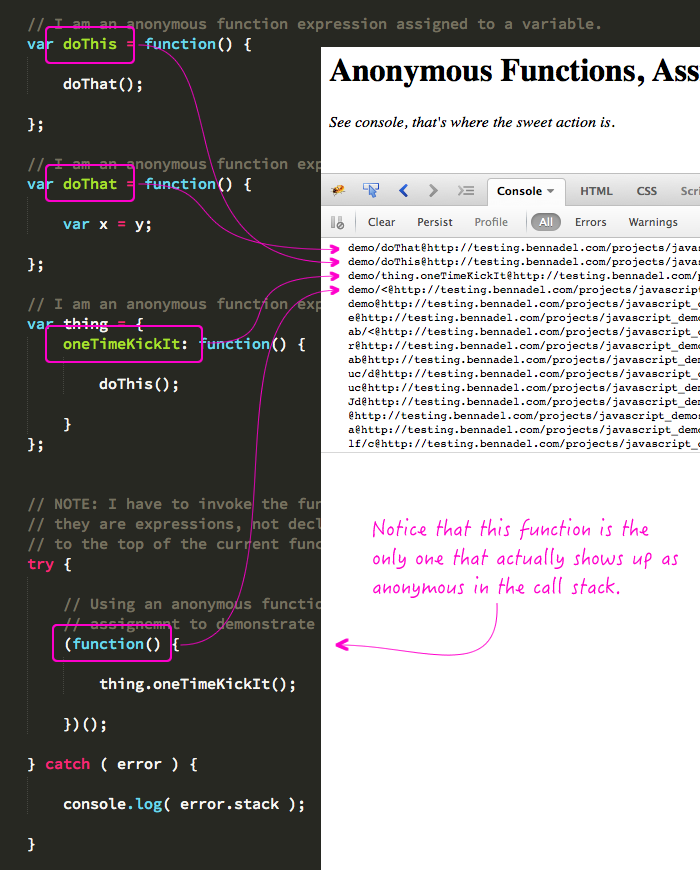
Javascript anonymous function syntax. The call, apply and bind methods are NOT suitable for Arrow functions -- as they were designed to allow methods to execute within different scopes -- because Arrow functions establish "this" based on the scope the Arrow function is defined within. For example call, apply and bind work as expected with Traditional functions, because we establish the scope for each of the methods: Javascript Front End Technology Object Oriented Programming A function expression is similar to and has the same syntax as a function declaration One can define "named" function expressions (where the name of the expression might be used in the call stack for example) or "anonymous" function expressions. Example Defining an Anonymous Function. When a function is defined, you often give it a name and then invoke it using that name, like so: foo(); function foo(){ // ... } When you define a function this way, the Javascript runtime stores your function in memory and then creates a reference to that function, using the name you've assigned it.
It sounds like, in lamda calculus, the functions are all anonymous and, I'm assuming, are called lambdas in discussion. At some point in time the term pollinated into JavaScript discussions to describe functions that were anonymous. Ultimately, I'm of the stance that the term lambda, is merely a synonymous term for an anonymous function. It is anonymous because the function has no name. The syntax consists of the function keyword, followed by zero or more parameters, and concludes with the function statements. The syntax looks like this: const name = function (parameters) { If you use an anonymous function as a callback, for example, you lose this useful feature. This is exemplified in the demo below where the anonymous function inside the .setInterval() method can ...
When you add a JavaScript event listener, the function to be executed upon an event can be either anonymous or named. In the example below, you can see the anonymous function used. In this case, you need to define the code in the function: ES6 added many amazing new features to JavaScript, but by far one of the best features is arrow functions. Arrow functions not only make code much more conci... In this tutorial, you will learn everything about JavaScript anonymous functions. Intro to JavaScript anonymous functions As the name suggest of these function, An anonymous function is declared without any name. See following the following example: In this example, theRead More JavaScript: Anonymous Functions
Anonymous Function is a function that does not have any name associated with it. Normally we use the function keyword before the function name to define a function in JavaScript, however, in anonymous functions in JavaScript, we use only the function keyword without the function name. 25/8/2021 · An anonymous function is a function that does not have any name associated with it. Normally we use the function keyword before the function name to define a function in JavaScript, however, in anonymous functions in JavaScript, we use only the function keyword without the function … The first example uses a regular function, and the second example uses an arrow function. The result shows that the first example returns two different objects (window and button), and the second example returns the window object twice, because the window object is the "owner" of the function.
Inside the () is an anonymous function. Its anonymous because there is no name assigned to it. The function can have parameters passed in, as we will see in a moment, just like any other function. Inside the function is the actual code you want to execute. After the function definition is another set of parenthesis. 21/3/2015 · First off, there is no "jQuery syntax". jQuery is a library, written in JavaScript, so the syntax is JavaScript. Example 1: I create an anonymous JavaScript-function. This function is executed right in that moment the browser reads it. I pass it the jQuery-Object (with ($) but I don't know why this is important at that point). Summary: in this tutorial, you will learn about JavaScript immediately invoked function expressions (IIFE). TL;DR. A JavaScript immediately invoked function expression is a function defined as an expression and executed immediately after creation. The following shows the syntax of defining an immediately invoked function expression:
The returned value. The context this when the function is invoked. Named or an anonymous function. The variable that holds the function object. arguments object (or missing in an arrow function) This post teaches you six approaches to declare JavaScript functions: the syntax, examples and common pitfalls. ES5 and Anonymous functions. JavaScript makes heavy use of anonymous functions. An anonymous function is a function that does not have a name attached to it. Anonymous functions are used during function callback. The following example illustrates the use of an anonymous function in ES5 − The meaning of the word 'anonymous' defines something that is unknown or has no identity. In JavaScript, an anonymous function is that type of function that has no name or we can say which is without any name. When we create an anonymous function, it is declared without any identifier.
Combining these two core concepts, JavaScript gives us a beautiful syntax called self-invoking anonymous functions. It simply means an unnamed function that executes itself. This special syntax can give us some extraordinary powers 💪, Power to expose only selected set of variables and methods to its outer scope. In computer programming, an anonymous function (function literal, lambda abstraction, lambda function, lambda expression or block) is a function definition that is not bound to an identifier.Anonymous functions are often arguments being passed to higher-order functions, or used for constructing the result of a higher-order function that needs to return a function. Anonymous Functions are different from Named Functions in JavaScript by not having a function name with which it refers to. The function without a name is called an "anonymous function" whereas the function with a name is called a "named function" Below is the example that depicts the difference between them.
Anonymous functions in JavaScript are functions that have no name; They can be used as an argument to other functions or as an immediately invoked function execution; All arrow functions are anonymous One of the types of JavaScript functions is called anonymous functions. As its name suggests, an anonymous function is a function that is declared without a name. Said differently, an anonymous function does not have an identifier. Anonymous functions are usually arguments passed to higher-order functions. JavaScript functions are defined with the function keyword. ... The function above is actually an anonymous function (a function without a name). ... You can only omit the return keyword and the curly brackets if the function is a single statement. Because of this, it might be a good habit to always keep them: Example.
Introduction to JavaScript anonymous functions An anonymous function is a function without a name. An anonymous function is often not accessible after its initial creation. The following shows an anonymous function that displays a message: 15/2/2020 · The anonymous function is declared with a variable name division and expecting two values from anonymous function calling. After passing 2 values from division(20,10) to function call, then we will get 2 as output. 3. Anonymous Function with Return Statement. Syntax: function functionName(parameters1,parameters2,…) {return function(a,b,…..) The most salient point which differentiates lambda functions from anonymous functions in JavaScript is that lambda functions can be named. This naming could be of help during debugging. An example ...
Anonymous Functions Assigned To References Show Up Well
Javascript Arrow Functions Fat Amp Concise Syntax Sitepoint
Var Functionname Function Vs Function Functionname
Anonymous Function An Overview Sciencedirect Topics
Anatomy Of The Lambda Expression
Javascript For Abap Programmers 4 7 Scope
Cse 154 Lecture 19 Events And Timers Anonymous Functions
Solve The Problem That Js File Running Under Node Terminal
An Visual Guide To Javascript Constructor Prototype Pattern
How To Use Python Lambda Functions Real Python
Avoiding Anonymous Javascript Functions Ultimate Courses
Anonymous Function An Overview Sciencedirect Topics
Javascript Anonymous Functions Tuts Make
Handling Common Javascript Problems Learn Web Development Mdn
Self Invoking Anonymous Function What Is It Amp Why And How
8 Daily Javascript Anonymous Functions
Python Lambda Expression Declaring Lambda Expression Amp Its
5 Differences Between Arrow And Regular Functions
Python Lambda Function Overview Squares Value Examples
Python Lambda Anonymous Function
What Are Javascript Arrow Functions Programming With Mosh
What Is The Purpose Of Self Executing Function In Javascript
Anonymous Functions In Practice Manning
Kotlin On Twitter If You Need A Local Return From Lambda
Var Functionname Function Vs Function Functionname
Javascript Self Invoking Anonymous Function Blocks Full
0 Response to "28 Javascript Anonymous Function Syntax"
Post a Comment