22 Javascript Get Column From Array Of Objects
TypeScript answers related to “get particular column value from array of objects javascript”. array of objects create common key as a property and create array of objects. search an array of objects with specific object property value. select column values from array typescript. Sort an Array of Objects in JavaScript. Summary: in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects.
How To Get Row And Column Of Matrix In Js Code Example
The reduce () method works from left to right in our array. It adds each num (number) in the array to the total value. The 0 at the end of the callback indicates the initial value for total. Starting with 1, the reduce () method adds 1 to the total then moves on to the next value in the array (1 + 0).
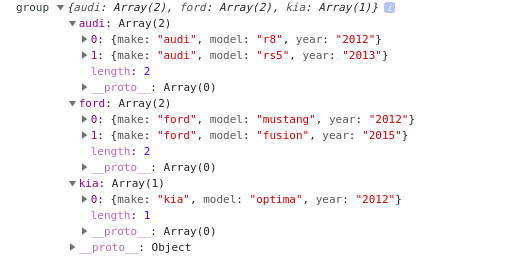
Javascript get column from array of objects. 12/9/2020 · To get only specific values in an array of objects in JavaScript, use the concept of filter(). Example var details = [{ studentName: "John", studentMarks: 92 }, { studentName: "David", studentMarks: 89 }, { studentName: "Mike", studentMarks: 98 }, ]; var specificValuesFromArray = details.filter(obj => obj.studentMarks === 92 || obj.studentMarks === 98); console.log(specificValuesFromArray) Array.from() lets you create Arrays from: array-like objects (objects with a length property and indexed elements); or ; iterable objects (objects such as Map and Set).; Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map ... Now, If we want to get the index of the object with the name key that matches Roy Daniel, we can use the findIndex () method. the findIndex () requires a function as an argument. the argument function will be passed the current array element every time an element is looped. Inside this function, we can check if the name matches Roy Daniel and ...
Array of states for each friend. Getting The Unique List of States. The next step is to use a JavaScript Set.. JavaScript Set. The Set object lets you store unique values of any type, whether primitive values or object references.. The key word to make note of in the description is unique.. The syntax to create a new Set is, Either flatten the objects first, like { brand: 'Audi', color_value: 'black' } or pass a function taking each object in the array, returning the desired value on that object. Eg. instead of const value = obj[key] do const value = keyFn(obj).Another approach would be to pass a key with dots, like 'color.value' and have the function parse that. Star. Extract a column from an array of JavaScript objects. Raw. extractColumn.js. function extractColumn(arr, column) {. function reduction(previousValue, currentValue) {. previousValue.push(currentValue[column]); return previousValue; }
JavaScript destructuring assignment is another feature deployed as a part of the ECMAScript release in 2015. Destructuring is a simple javascript expression, that is used to unpack values from an array, or unpack properties from an object. Basically, it works with any iterable on the right side of an assignment operator. Code language: plaintext (plaintext) Note that the (index) column is for the illustration that indicates the indices of the inner array.. To access an element of the multidimensional array, you first use square brackets to access an element of the outer array that returns an inner array; and then use another square bracket to access the element of the inner array. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
26/9/2018 · Distinct property values of an array of objects. Quite often, the array we are dealing with consists of JSON objects retrieved from server side. Now, we want to get distinct values of a certain property from the objects array. For example, the array is [ {"name":"Joe", "age":17}, {"name":"Bob", "age":17}, {"name":"Tom", "age":35} ] and we want to get distinct ages from the array. In JavaScript, objects are used to store multiple values as a complex data structure. An object is created with curly braces {…} and a list of properties. A property is a key-value pair where the key must be a string and the value can be of any type.. On the other hand, arrays are an ordered collection that can hold data of any type. In JavaScript, arrays are created with square brackets ... 24/10/2013 · Add a comment |. 6. Easily extracting multipleproperties from array of objects: let arrayOfObjects = [ {id:1, name:'one', desc:'something'}, {id:2, name:'two', desc:'something else'}];//below will extract just the id and namelet result = arrayOfObjects.map(({id, name}) => ({id, name}));
Code language: JavaScript (javascript) In this example, we called the filter () method of the cities array object and passed into a function that tests each element. Inside the function, we checked if the population of the each city in the array is greater than 3 million. If it is the case, the function returns true; Otherwise, it returns false ... Get the element for the given ID. Click Here: document.createElement(TAG) Creates a new DOM element. Click Here: ELEMENT.appendChild(OBJECT) Append the given object into the specified element. Click Here: TABLE.insertRow() Add a new row to the table. Click Here: ROW.insertCell() Add a new cell to the table row. Click Here One way to get distinct values from an array of JavaScript objects is to use the array's map method to get an array with the values of a property in each object. Then we can remove the duplicate values with the Set constructor. And then we can convert the set back to an array with the spread operator. For instance, we can write:
In terms of performance, _.find() is faster as it only pulls the first object with property {'b': 6}, on the other hand, if suppose your array contains multiple objects with matching set of properties (key:value), then you should consider using _.filter() method. In the above program, the property value of key a is extracted from each object of an array. Initially, the extractedValue array is empty. The for loop is used to iterate through all the elements of an array. During each iteration, the value of property a is pushed to the extractedValue array. This function works to arrays and objects. obs: it works like array_column php function. It means that an optional third parameter can be passed to define what column will correspond to the indices of return.
A list. Objects, as generic blobs of values, can be used to build all sorts of data structures. A common data structure is the list (not to be confused with array). A list is a nested set of objects, with the first object holding a reference to the second, the second to the third, and so on. reason: When next().done=true or currentIndex>length the for..of loop ends. See Iteration protocols.. Value: there are no values stored in the array Iterator object; instead it stores the address of the array used in its creation and so depends on the values stored in that array. If a property with the same name exists on the object's prototype chain, then, after deletion, the object will use the property from the prototype chain (in other words, delete only has an effect on own properties). Any property declared with var cannot be deleted from the global scope or from a function's scope. Instead you can map().
Its additions really improve the coding experience in JavaScript and once again shows that JavaScript is here to stay. One of the new features is the ability of destructuring arrays and objects. This is an easy and convenient way of extracting data from arrays and objects. Let's take a look at how it's done, starting with the array. JavaScript is more than just strings and numbers. JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number of values that you can iterate through. slice method can also be called to convert Array-like objects/collections to a new Array. You just bind the method to the object. The arguments inside a function is an example of an 'array-like object'. function list() { return Array. prototype.slice.call( arguments) } let list1 = list(1, 2, 3) Copy to Clipboard.
At the time of writing "Array extras" (which are actually standardized methods, rather than extras) are supported by the new versions of all major browsers. Unless stated otherwise, all the discussed methods can be safely used in: Opera 11+ Firefox 3.6+ Safari 5+ Chrome 8+ Internet Explorer 9+ (source: dev.opera ) - Stéphan Aug 24 ... The Array.prototype.findIndex () method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true. As you can see there were two {name: "prashant", age: 16} and we filtered it to get only unique items. Unique properties of array of objects. We can use the spread … along with map and Set to get the unique properties from the array of objects.
Sort an array of objects in JavaScript dynamically. Learn how to use Array.prototype.sort() and a custom compare function, and avoid the need for a library.
Javascript Group An Array Of Objects By Key By Edison
How To Check If Array Includes A Value In Javascript
Javascript Array Distinct Ever Wanted To Get Distinct
Array Programming With Numpy Nature
How To Get Only Value From An Object Array Without Column
Get The Count Of Nested Array Object S Children Property
Javascript Fundamental Es6 Syntax Convert An Array Of
Solved Value From Array Power Platform Community
Typescript Return Array Of Objects Code Example
Convert Column In Data Table To Array Help Uipath
Numpy Access An Array By Column W3resource
2d Arrays In Javascript Experts Exchange
Google Apps Script Getting Selected Google Sheets Columns
Es6 Destructuring An Elegant Way Of Extracting Data From
R Array Function And Create Array In R An Ultimate Cheat
How To Filter Object In Javascript Code Example
Increase The Value Of Object In Array Javascript Code Example
0 Response to "22 Javascript Get Column From Array Of Objects"
Post a Comment