24 Javascript Date Add Hour
JavaScript Datetime: Exercise-6 with Solution. Write a JavaScript function to add specified minutes to a Date object. Test Data: console.log (add_minutes (new Date (2014,10,2), 30).toString ()); Output : "Sun Nov 02 2014 00:30:00 GMT+0530 (India Standard Time)" Given a date and the task is to add minutes to it. To add minutes to the date object in javascript, some methods are used which are listed below: JavaScript getMinutes () Method: This method returns the Minutes (from 0 to 59) of the provided date and time. Return value: It returns a number, from 0 to 59, representing the minutes.
The Date Object Getting Setting Amp Formatting Dates In Javascript Tutorial For Beginners
Javascript to add one hour to NOW in CRM Verified Also the solution with setHours and getHours was a lot better, so I would also do this as in your first version of code (just use "new")
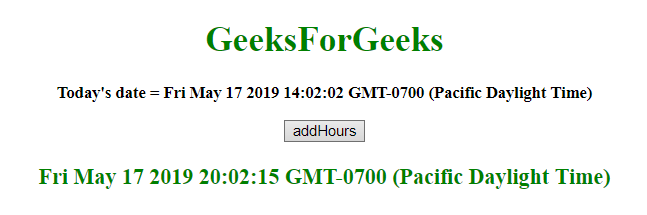
Javascript date add hour. Date Object¶ The Date is a built-in object in JavaScript. Once a Date object is created with the New Date(), a set of methods become available to operate on it. These methods allow getting and setting the year, month, day, hour, minute, second, and millisecond using either local time or UTC time. Formatting a JavaScript date is a common thing. There are a date and time string everywhere on websites. A considerable number of application dates are formatted with JavaScript code. There are some methods and libraries that are used to format dates. Let's consider each of them separately. Despite the JavaScript Date warts, it's straightforward to add day(s) to a date in JavaScript. While it would be very easy to reach for moment.js or another date manipulation library (date-fns, luxon, dayjs) to do something as simple as adding days to a Date in JavaScript, writing a short helper function might just be easier.
First, here's how it's done in plain old JavaScript: var date = new Date(); // Todays date - the Date () constructor will default to the current date/time if no value is passed to it. var addDays = 4; var addHours = 3; var addMinutes = 2; var addSeconds = 1; // Add hours. // The getTime () method returns the number of milliseconds since ... The current system time was 11:14:30 and I added 5 hours to it in the above JavaScript program. date1.setHours (date1.getHours ()+hours_to_be_added); In the above line, you have to put the hours in numeric in order to add hours to the current hour. ( put how many hours you want to add instead of hours_to_be_added ) var date1 = new Date (); 25/5/2017 · The below code is to add 4 hours to date(example today's date) var today = new Date(); today.setHours(today.getHours() + 4); It will not cause error if you try to add 4 to 23 (see the docs): If a parameter you specify is outside of the expected range, setHours() attempts to update the date information in the Date object accordingly
The current time in my system was 19:42:03 but this JavaScript program print the current time by adding 5 minutes. date1.setMinutes (date1.getMinutes ()+n); You can change the value of n. here n will be the time in minutes you want to be added with the current time. var date1 = new Date (); here you can also pass the date and time as per your ... Luckily, the JavaScript Date object has a method called getTime() which returns the number of milliseconds since 01/01/1970, so we can first calculate the number of milliseconds of the old date, then add the milliseconds of the offset to create a new date. In this section, we'll discuss how you can add time to a JavaScript Date object in vanilla JavaScript. In JavaScript, the getTime() function returns the number of milliseconds since the Unix epoch time. That's just a computer time convention that counts the number of seconds (milliseconds in JavaScript) since midnight UTC on January 1st, 1970.
JavaScript getHours() method returns the hour (from 0 to 23) of the specified date and time. Using JavaScript setHours() and getHours() method you can easily add hours to Date object. Here we'll provide a short and simple code to add hours to JavaScript Date object. Use the following JavaScript code to add hours to current date. month name array javascript; date add hours javascript; javascript get 1 hour from now; json datetime; compare two dates using moment; javascript get remaining time from start and end datetime; javascript difference between two dates in days; currentTime(); javascript; angular moment pipe timeAgo; jquery datepicker only month and year; js date ... To add minutes to a JavaScript Date object, use the setMinutes () method. Under that, get the current minutes and 30 minutes to it. JavaScript date setMinutes () method sets the minutes for a specified date according to local time.
Required. An integer representing the hour. Expected values are 0-23, but other values are allowed: -1 will result in the last hour of the previous day. 24 will result in the first hour of the next day. min. Optional. An integer representing the minutes. Expected values are 0-59, but other values are allowed: The following example uses a dateWith (delay) function illustrating how to add seconds to a date. First, create a new date representing "now". Then, replace the seconds of the "now" date by adding the delay to the current time: The JavaScript runtime also handles delays with more than 59 seconds properly. Date Methods. When a Date object is created, a number of methods allow you to operate on it.. Date methods allow you to get and set the year, month, day, hour, minute, second, and millisecond of date objects, using either local time or UTC (universal, or GMT) time.
17/5/2019 · To add hours to date in javascript, we’re going to discuss a few techniques. First few methods to know. JavaScript getHours() Method This method returns the hour (from 0 to 23) of the provided date and time. Syntax: Date.getHours() Return value: It returns a number, from 0 to 23, representing the hour. JavaScript setHours() Method The second statement below assigns the value 23 to the variable hours , based on the value of the Date object Xmas95. let Xmas95 = new Date('December 25, 1995 23:15:30'); let hours = Xmas95.getHours(); console.log( hours); In Javascript, date and time are handled with the built-in Date object. Displaying the Current Date & Time. Creating a new Date object sets its date and time to the current date and time as given by the browser. The timezone is also set to the browser timezone. var dt = new Date(); The Date object has several methods to get individual date & time :
Formatting time. The toLocaleTimeString() method also accepts two arguments, locales and options. These are some available options. timeZone : Iana time zones List hour12 : true or false.(true is 12 hour time ,false is 24hr time) hour: numeric, 2-digit. minute: numeric, 2-digit. second: numeric, 2-digit. To add days to a date in JavaScript, you can use the setDate () and getDate () methods of the Date object. These methods are used to set and get the day of the month of the Date object. The following example demonstrates how you can add a day to the new instance of JavaScript Date object: You could even add a new method to the Date 's prototype ... To add hours to a JavaScript Date object, use the setHours() method. Under that, get the current hours and 2 hours to it. JavaScript date setHours() method sets the hours for a specified date according to local time. Example. You can try to run the following code to add 2 hours to date. Live Demo
Individual date and time component values Given at least a year and month, this form of Date() returns a Date object whose component values (year, month, day, hour, minute, second, and millisecond) all come from the following parameters. Any missing fields are given the lowest possible value (1 for day and 0 for every other component).The parameter values are all evaluated against the local ... Let's meet a new built-in object: Date.It stores the date, time and provides methods for date/time management. For instance, we can use it to store creation/modification times, to measure time, or just to print out the current date. Let's see the list of JavaScript date methods with their description. Methods. Description. getDate () It returns the integer value between 1 and 31 that represents the day for the specified date on the basis of local time. getDay () It returns the integer value between 0 and 6 that represents the day of the week on the basis of local time.
Creates date based on specified date and time. To demonstrate the different ways to refer to a specific date, we'll create new Date objects that will represent July 4th, 1776 at 12:30pm GMT in three different ways. usa.js. new Date(-6106015800000); new Date("July 4 1776 12:30"); new Date(1776, 6, 4, 12, 30, 0, 0); Copy. Before Java 8, java.util.Date was one of the most commonly used classes for representing date-time values in Java. Then Java 8 introduced java.time.LocalDateTime and java.time.ZonedDateTime.Java 8 also allows us to represent a specific time on the timeline using java.time.Instant.. In this tutorial, we'll learn to add or subtract n hours from a given date-time in Java.
Using Laravel S Signed Urls To Add Action Links To Emails
10 Best Date And Time Picker Javascript Plugins 2021 Update
Getting Date And Time In Node Js
How To Format A Utc Date As A Yyyy Mm Dd Hh Mm Ss String
Moment Js Cheat Sheet By Nothingspare Download Free From
How To Calculate Time In Google Sheets
Dateadd Sql Function Introduction And Overview
How To Add Time In Excel Add Hours And Minutes In Excel
Learn How To Get Current Date Amp Time In Javascript
Adding One To Date And Time Enter Date New Date Add Chegg Com
Learn How To Get Current Date Amp Time In Javascript
A Guide To Handling Date And Time For Full Stack Javascript
Convert Text To Time Values With Power Query Excel Campus
How To Get Current Formatted Date Dd Mm Yyyy In Javascript
Using Python Datetime To Work With Dates And Times Real Python
Javascript Adding Hours To The Date Object Geeksforgeeks
Display Date And Time Using Javascript Pranav Dave S Blog
Js Add Minutes To Hour Code Example
The Definitive Guide To Javascript Dates
Java Add Subtract Years Months Days Hours Minutes Or
Date And Time Momentjs Edition
Dateadd Sql Function Introduction And Overview
How To Implement Javascript Date Date Object Methods Edureka
0 Response to "24 Javascript Date Add Hour"
Post a Comment