32 Javascript If Function Defined
A function is a set of statements that take inputs, do some specific computation, and produces output. Basically, a function is a set of statements that performs some tasks or does some computation and then return the result to the user. Pre-defined Functions; User-defined Functions; Here we are going to learn, how to write a user-defined function in JavaScript: To create a function in JavaScript we have to use the"function " keyword before writing the name of our function as you can see in given syntax: Syntax of creating function
How To Check A Function Is Defined In Javascript
Normally, a function defined would not be accessible to all places of a page. Say a function is mentioned using "function checkCookie ()" on a external file loaded first on a page. This function cannot be called by the JavaScript file loaded last. So you have to make it a Global function that can be called from anywhere on a page.
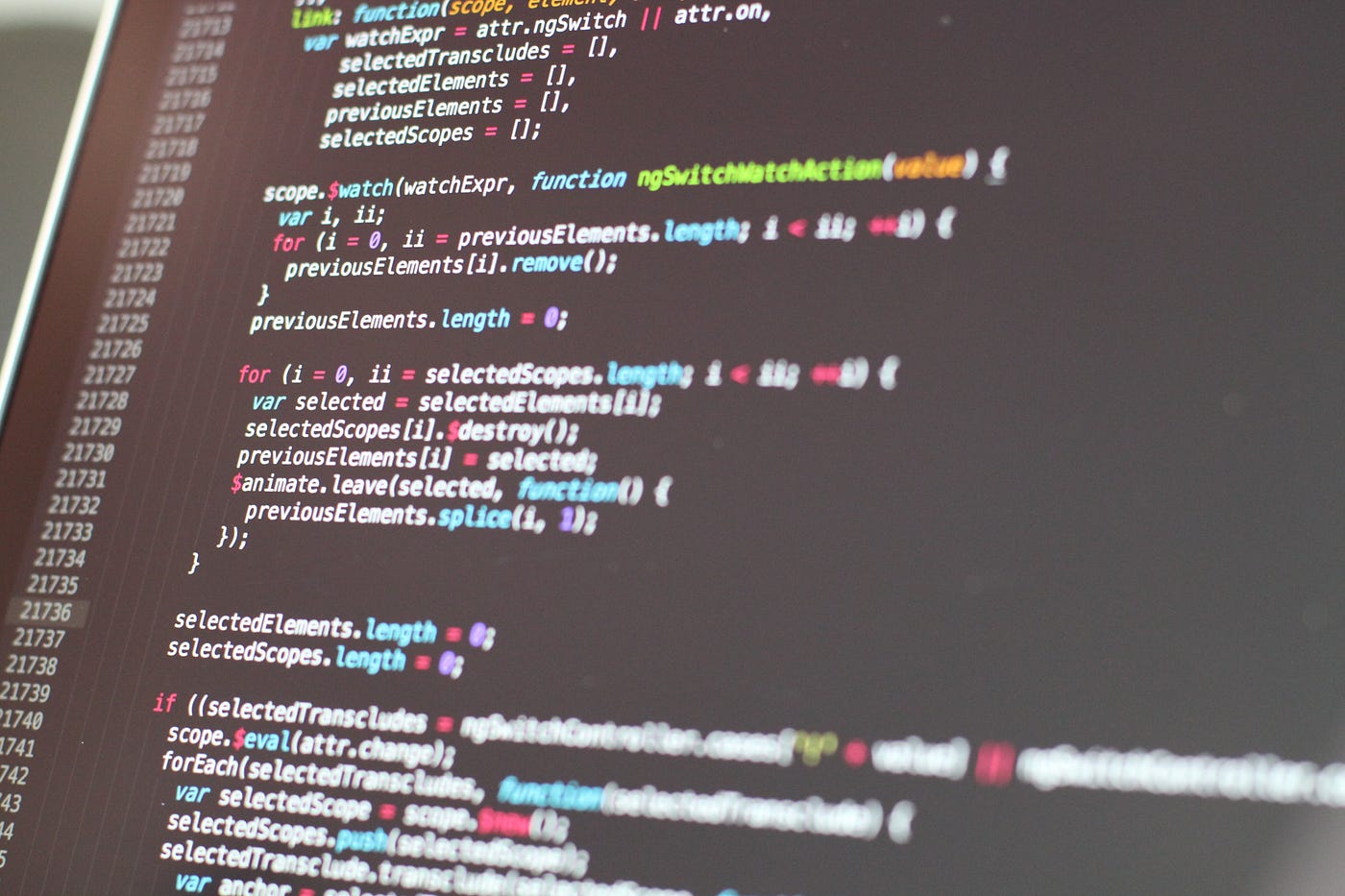
Javascript if function defined. Functions can be defined as object methods in JavaScript. The example below creates a new object simpleObject with two properties ( numberX and numberY ) and one method ( sumNumbers ): Example Copy Check if a JavaScript function exists before calling it. To check if a particular function name has been defined, you can use JavaScript’s typeof operator: //Use the typeof operator to check if a JS function exists. if (typeof bad_function_call === "function") { bad_function_call (); } A function defined like this is accessible from anywhere within its context by its name. But sometimes it can be useful to treat function references like object references. For example, you can assign an object to a variable based on some set of conditions and then later retrieve a property from one or the other object:
Aug 02, 2019 - In this tutorial, I will show you how to check if a JavaScript function is defined or not. Mar 13, 2016 - A simple way to check if a Javascript function exists before trying to call it. This helps you avoid common function undefined error. Basically $ is an alias of jQuery() so when you try to call/access it before declaring the function, it will endup throwing this $ is not defined error . This usually indicates that jQuery is not loaded and JavaScript does not recognize the $. Even with $(document).ready , $ is still going ...
Functions don't need to be declared directly inside a tag. You can also put the code: function functionname() { Alert('hello world') } anywhere between the two script tags. As for your question on having the code run after the user clicks the table cell, you can do this. 17/11/2020 · // missingVar is defined let existingVar; try {existingVar; console. log ('existingVar is defined')} catch (e) {console. log ('existingVar is not defined');} // logs 'existingVar is defined' Compared to typeof approach, the try/catch is more precise because it determines solely if the variable is not defined , … A JavaScript UDTF is defined using the same syntax as a SQL UDTF, but requires the LANGUAGE JAVASCRIPT clause. In addition, instead of a SQL block in the function definition, JavaScript code is passed. Below is the basic syntax for creating a JavaScript UDTF:
A function is a block of code that performs an action or returns a value. Functions are custom code defined by programmers that are reusable, and can therefore make your programs more modular and efficient. In this tutorial, we will learn several ways to define a function, call a function, and use function parameters in JavaScript. Mar 10, 2021 - One way to see if you have any error is to run the HTML page and check on the console as follows: ... You may find the ReferenceError fixed itself as you fix JavaScript errors from your scripts. Make sure the script is loaded before the call. Finally, the function is not defined error can also ... JavaScript functions are defined with the function keyword. You can use a function declaration or a function expression.
Please keep in mind that functions defined through function expressions must be defined before the call. Function expressions are functions that you defined through a variable keyword as follows: There are a few different ways to define a function in JavaScript: A Function Declaration defines a named function. To create a function declaration you use the function keyword followed by the name of the function. When using function declarations, the function definition is hoisted, thus allowing the function to be used before it is defined ... A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when "something" invokes it (calls it).
In JavaScript, a function allows you to define a block of code, give it a name and then execute it as many times as you want. A JavaScript function can be defined using function keyword. To create a JavaScript user-defined function in your Stream Analytics job, select Functions under Job Topology. Then, select JavaScript UDF from the +Add dropdown menu. You must then provide the following properties and select Save. Enter a name to invoke the function in your query. However, a function can access all variables and functions defined inside the scope in which it is defined. In other words, a function defined in the global scope can access all variables defined in the global scope.
Functions defined in a JavaScript file. Non-trivial program logic is best separated into a separate JavaScript file. This file can be imported into QML using an import statement, like the QML modules. For example, the fibonacci() method in the earlier example could be moved into an external file named fib.js, and accessed like this: In JavaScript, function is defined by using the function keyword followed by the function name and parentheses (), to hold params (inputs) if any. A function can have zero or more parameters separated by commas. Dec 02, 2018 - Functions always return a value. In JavaScript, if no return value is specified, the function will return undefined. Functions are objects. Define a Function.
Nov 02, 2019 - JavaScript allows to declare functions in 6 ways. The article describes how to choose the right declaration type, depending on the function purpose. May 26, 2018 - In a JavaScript program, the correct way to check if an object property is undefined is to use the `typeof` operator. See how you can use it with this simple explanation 29/1/2018 · To check if a JavaScript function is defined or not, checks it with “undefined”.ExampleYou can try to run the following example to check for a function is d ...
Check if a Javascript Function Exists or Is Defined It’s always frustrating when you get an error trying to call a function that hasn’t been defined but there’s an easy way to prevent this. To check if a Javascript function exists before calling it, try this: if (typeof yourFunctionName == 'function') { yourFunctionName (); } Aug 19, 2018 - Strangely enough it worked fine the first time I ran it, and ever since then I've been getting an error that says JavaScript Function Not Defined - and it stops running. I have googled around similar problems but none of the advice has been able to resolve the issue. Asp.Net 3.5 Webforms, if it ... Function Expressions. In Javascript, functions can also be defined as expressions. For example, // program to find the square of a number // function is declared inside the variable let x = function (num) { return num * num }; console.log(x(4)); // can be used as variable value for other variables let y = x(3); console.log(y); Output. 16 9
Re: Javascript function not defined. Open Microsoft Internet Explorer. On the Tools menu, click Internet Options. On the Advanced tab, locate the Browsing section, and uncheck the Disable script debugging check box, and then click OK. In JavaScript, a function can be defined based on a condition. For example, the following function definition defines myFunc only if num equals 0: JavaScript user-defined functions (UDFs) are new in Excel Services in SharePoint. This article provides a high-level look at JavaScript UDFs, including basic information on how they work in Excel Services.
using a string so you can have only one place to define function name. //byString var strFunctionName='myFunction' if( (typeof window[strFunctionName])=='function') alert(s+' is function'); else alert(s+' is not defined'); Share. Improve this answer. In a nutshell, function declarations are loaded before JavaScript starts executing while function expressions are executed only when Interpreter hits that line. In JavaScript if you have too many functions and not all are necessary to be called at a particular instance, you should use function expressions. The reason for the "undefined" in the output is: In JavaScript if two functions are defined with same name then the last defined function will overwrite the former function. So in this case the foo(arg1) was overwritten by foo(arg1,arg2), but we only passed one Argument ("Geeks") to the function.
JavaScript functions are basically objects. This tutorial explains, how to write functions with parameters. The examples will also show how to call functions. Let us first look at how to define a function. Syntax to define a JavaScript function. This is how a function is defined in JavaScript: function function_name() {//Code of function} Where ... Sep 04, 2017 - If you are using jQuery, Angular JS, or plain old JavaScript and getting "Uncaught ReferenceError: $ is not defined" error which means $ is either a variable or a method that you are trying to use before declaring it using the var keyword. In jQuery, it's a short name of jQuery() function and most ... May 24, 2019 - Parameter: It contains single value var which is a Javascript variable. Return value: It returns the type of a variable or an expression: Example 1: This example checks the type of the function, If it is function then it is defined otherwise not defined by using typeof operator.
function DoLoad() { alert(" Hello"); } Any idea of why the function would work with the inline JavaScript but not with the imported JavaScript? I would rather have my code defined in an external file, so getting it to work that way would be ideal. FYI, I am using Visual Studio 2008 Professional and I am working with an ASP.NET Web Application. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output.
How To Write Excel If Function Statements
Arrow Functions Vs Regular Functions In Javascript Level Up
How To Write Excel If Function Statements
Ms Excel How To Use The Nested If Functions Ws
Code Navigation In Visual Studio Code
Javert Javascript Verification Toolchain The Morning Paper
How To Check If A Variable Is An Array In Javascript
Accessing Javascript Functions Defined In Different Files
Javascript If Statement Didn T Work Stack Overflow
Javascript Declaring Variables With Var Let And Const By
Reawakening Of Emotet An Analysis Of Its Javascript
Tutorial If Elif Else In Python Datacamp
How To Make A Function In Javascript Code Example
User Defined Functions In Javascript
Use The Javascript Code Below To Answer The Questions Chegg Com
Javascript Switch Statement With Js Switch Case Example Code
Go To Definition For Javascript Functions In Visual Studio
Javascript Fixing Function Is Not Defined Error
Var Functionname Function Vs Function Functionname
Javascript Programming With Visual Studio Code
Use The Javascript Code Below To Answer The Questions Chegg Com
Go To Definition For Javascript Functions In Visual Studio
The Difference Between Function Var And Let Const By
Creating Custom Functions Salient Process Inc
Understanding Hoisting In Javascript Digitalocean
Harnessing The Power And Convenience Of Javascript For Each
Ms Excel How To Use The If Function Ws
Javascript Functions Part 1 Functions In Mathematics Are
Javascript Immediately Invoked Function Expressions Iife
In Javascript With In This Place Is What Do You Mean
0 Response to "32 Javascript If Function Defined"
Post a Comment