32 Ajax Error Handling In Javascript
Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor. The explanation. A callback is required because an AJAX, as the name A synchronous J avaScript A nd X ML suggests, is asynchronous. When you initiate a AJAX request using either the native XMLHttpRequest method or via jQuery, the HTTP request is sent, but the JavaScript engine does not wait for a response.
Handle Ajax Requests In Asp Net Core Razor Pages
Offical.ajaxError () API Note: If $.ajax () or $.ajaxSetup () is called with the global option set to false, the.ajaxError () method will not fire. Below is an older function which you can get the...
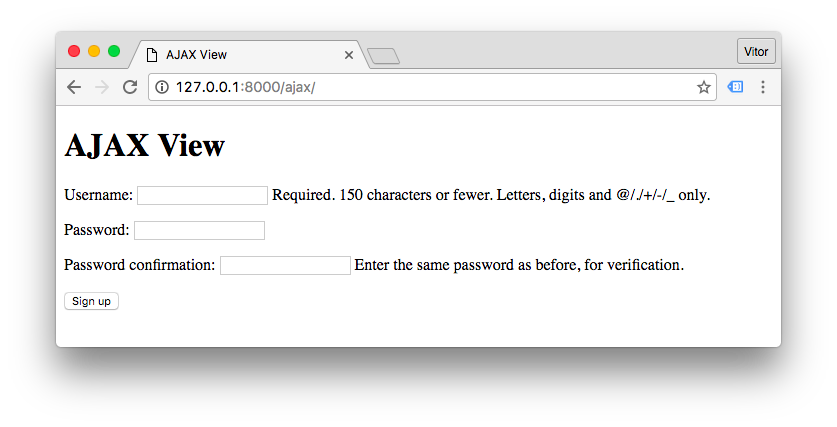
Ajax error handling in javascript. Whenever an Ajax request completes with an error, jQuery triggers the ajaxError event. Any and all handlers that have been registered with the.ajaxError () method are executed at this time. Note: This handler is not called for cross-domain script and cross-domain JSONP requests. To observe this method in action, set up a basic Ajax load request. 21/1/2020 · Error handling is vital while making AJAX requests in any tool. The solution I propose can be applicable for all kinds of frameworks or libraries. I am used to working with React.JS and in this blog, I talk about the approach I use handling errors that occur during AJAX requests. There can be 3 kinds of situation where we might receive errors. Nov 21, 2016 - Some times when we request to server we didn't get proper response (HTTP Status 200) as per our expectation. For ex. we can get 1) Internal Server Error 2) Network Connection Error 3) Page Not Found Error, 4) Access Denied Error. So if we are making call to server via http post request/simple h
May 01, 2019 - Ah, the perils of error handling in JavaScript. If you believe Murphy’s law, anything that can go wrong, will go wrong. In this article, I would like to explore error handling in JavaScript. I will cover pitfalls, good practices, and finish with asynchronous code and Ajax. but what if you have multiple ajax requests and you only want to write success or error handler once, or you want to catch error in load () method. here is what you can do $ (document).ajaxError (function (event, jqxhr, settings) { //your code here }).ajaxError () will be triggered no matter which request is completed. The ajaxError () method specifies a function to be run when an AJAX request fails. Note: As of jQuery version 1.8, this method should only be attached to document.
At least 1 upper-case and 1 lower-case letter. Minimum 8 characters and Maximum 50 characters Jun 09, 2020 - Error handling and feedback for AJAX requests can be a tedious undertaking for JavaScript heavy web applications. Often the AJAX functions are spread out in your code and, if you’re like me, you may put off error handling until late in the game. In a significant web project we’re wrapping ... With this method and my unified AJAX response, handling errors is actually quite easy. All AJAX errors are piped through my AJAXFailHandler () method which creates a "fail" AJAX response (sets SUCCESS flag to false) and then manually executes the AJAX callback, passing in the fail response. This way, from the AJAX response handler's point of ...
how to jQuery ajax error function and jQuery Error Ajax message when Ajax decision passing information to a page that then returns a worth. If it is a valid number then an alert message is displayed inside the jQuery AJAX Success event handler and if an exception occurs in the WebMethod, the thrown exception is caught inside the jQuery AJAX Error event handler and which makes a call to the OnError JavaScript function which processes and displays the exception details. Angular 9,8,7,6,5,4,2, TypeScript, JavaScript, Java, PHP, NodeJs, MongoDB, Knockout, Maven, R, Go, Groovy, OpenXava, Kafka, Rust, Vue, SEO, Interview
Many pages send AJAX requests to a server. Because this relies on the cooperation of the server and the network between the client and the server, you can expect these AJAX errors: Your JavaScript program receives an error response instead of data; Your program has to wait too long for the response. AJAX. The term AJAX stands for Asynchronous JavaScript And XML. The term AJAX is used in JavaScript for making asynchronous network request to fetch resources. Resources are not limited to XML, as the term suggest which is confusing. The term AJAX is also used to fetch resources as JSON, HTML, or Plain Text. You may have heard that term already. JavaScript catches adddlert as an error, and executes the catch code to handle it. JavaScript try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
You have a JSON object of the exception thrown, in the xhr object. Just use. alert(xhr.responseJSON.Message); The JSON object expose two other properties: 'ExceptionType' and 'StackTrace' The Ajax error function has three parameters: jqXHR. textStatus. errorThrown. In truth, the jqXHR object will give you all of the information that you need to know about the error that just occurred. This object will contain two important properties: status: This is the HTTP status code that the server returned. In the error message from the ajax () function we are getting a generalized message "Internal Server Error" rather than "DevideByZeroException" or our custom message returned by a new exception object. So, we are not getting a prompt with an actual exception. Ok, then this is not the correct way to handle exceptions in the Web API.
If someone is here as in 2016 for the answer, use .fail() for error handling as .error() is deprecated as of jQuery 3.0 $.ajax( "example.php" ) .done(function() { alert( "success" ); }) .fail(function(jqXHR, textStatus, errorThrown) { //handle error here }) I hope it helps We will see, how to handle fetch API errors using promises and async await syntax in JavaScript Our courses website is live at jsmates Learn with Param { P } When an AJAX request is submitted to a site, server-side errors are easily handled with the jQuery promise approach..done (),.fail (), etc. However for some requests (e.g. to an invalid site or one that doesn't accept cross-origin requests), an exception occurs immediately as the call is made. Here's an example of one error in the console:
Oct 07, 2020 - First I am not very good in JavaScript so maybe I do not know some very basics... I apologize in advance. I created a form where I want to save every change in single fields directly without the ne... Here are 24 tips to guide through the process of implementing AJAX technology within your web application. 1. Understand What it All Means. First up, you need to understand what AJAX is, what it stands for and how it has revolutionized parts of the internet. To handle errors, there are 3 different ways which can be categorize into. Local Events. Global Events. Using Promise Interface. You may also like: jQuery : Execute/Run multiple Ajax request simultaneously. jQuery - Load ASP.Net User Control Dynamically. Use jQuery.getScript to load external js files. Local Events.
Handling HTTP Response Codes with $.ajax() Example In addition to .done , .fail and .always promise callbacks, which are triggered based on whether the request was successful or not, there is the option to trigger a function when a specific HTTP Status Code is returned from the server. When an error occurs, the JavaScript interpreter generates an object containing the details about it. This error object is then passed as an argument to catch for handling. Tip: The try-catch statement is an exception handling mechanism. I'm using XMLHttpRequest in JavaScript. However, it gives me an error, and I don't know what my problem is. I have to parse an XML file and assign its contents to the webpage - here's my code:
Error handling and feedback for AJAX requests can be a tedious undertaking for JavaScript heavy web applications. Often the AJAX functions are spread out in your code and, if you're like me, you may put off error handling until late in the game. The code of a promise executor and promise handlers has an "invisible try..catch " around it. If an exception happens, it gets caught and treated as a rejection. For instance, this code: new Promise((resolve, reject) => { throw new Error("Whoops!"); }).catch( alert); // Error: Whoops! …Works exactly the same as this: Whenever an Ajax request completes with an error, jQuery triggers the ajaxError event. Any and all handlers that have been registered with the .ajaxError() method are executed at this time. Note: This handler is not called for cross-domain script and cross-domain JSONP requests.
Having a way to handle errors on individual AJAX calls is a great way to fine-tune your JavaScript and deal with specific error scenarios close to their occurrences. However, being aware of a centralized AJAX error-handling routine could avoid scenarios similar to this: $.post ("/api/operationA", function() { Aug 16, 2011 - The server should return valid JavaScript that passes the JSON response into the callback function. $.ajax() will execute the returned JavaScript, calling the JSONP callback function, before passing the JSON object contained in the response to the $.ajax() success handler.
Enterprise Javascript Error Handling Ajax Experience 2008
Here Are The Most Popular Ways To Make An Http Request In
Jquery Wait For All Ajax Requests To Complete Code Example
Partial Page Updates With Jquery Ajax Technique
Ajax Error Handling For Submit Function In Functions File In
Javascript Jquery Ajax Are They The Same Or Different
Web Api With Ajax Exception Handling In Jquery Ajax Function
How To Work With Ajax Request With Django
E Rror Lifecycle Browser Error
Programmers Sample Guide Jquery Ajax Request And Response
Ajax Status Is 200 But Error Event Triggers Instead Of
Fetching Data From The Server Learn Web Development Mdn
Logging Client Side Javascript Errors To The Server Codeproject
How To Do Ajax Form Validation With Inline Error Messages
Problem Spfx Javascript Ajax Microsoft Tech Community
Access Denied Ajax Request Javascript Stack Overflow
Parsing A Jquery Ajax Error Response Message Stack Overflow
Drupal Alerts An Ajax Http Request Terminated Abnormally
How To View Error From Ajax Javascript Laravel Adnantech
Exception Caught In Try Catch Returned In Ajax Error State
Saving Settings Handler Is Not Working Due To Ajax Error
Getting An Ajax Error After Returning From Generic Handler
How I M Going To Teach Authentication Zell Liew
A Guide To Proper Error Handling In Javascript Sitepoint
Here Are The Most Popular Ways To Make An Http Request In
Web Api With Ajax Exception Handling In Jquery Ajax Function
Spring Boot Ajax Example Mkyong Com
Cse 154 Lecture 22 Ajax Ppt Download
Ajax Error Response Code Example
Asp Net Mvc Jquery Ajax Error Callback Is Not Returning
0 Response to "32 Ajax Error Handling In Javascript"
Post a Comment