30 Javascript Es6 Map Function
All three functions were introduced in ES6 (ECMAScript 2015) and are functions that are applied to an Array. Also, all of them as so-called higher-order functions. That means that they are a function that returns functions as results or take other functions as parameters. In this case, it's the latter. After ES6. Maps are ordered list of key value pair where key as well as value can be of any type.. Syntax let map = new Map([iterable]); If an Iterable object like Array(arrays with two elements, e.g. [[ 1, 'one' ],[ 2, 'two' ]]) whose elements are key-value pairs is passed then all its items will be added to the Map.. null values are treated as undefined.
Javascript Map Function Explained A Deep Dive Qvault
ES6 provides us a new collection type called Map, which holds the key-value pairs in which values of any type can be used as either keys or values. A Map object always remembers the actual insertion order of the keys. Keys and values in a Map object may be primitive or objects. It returns the new or empty Map.
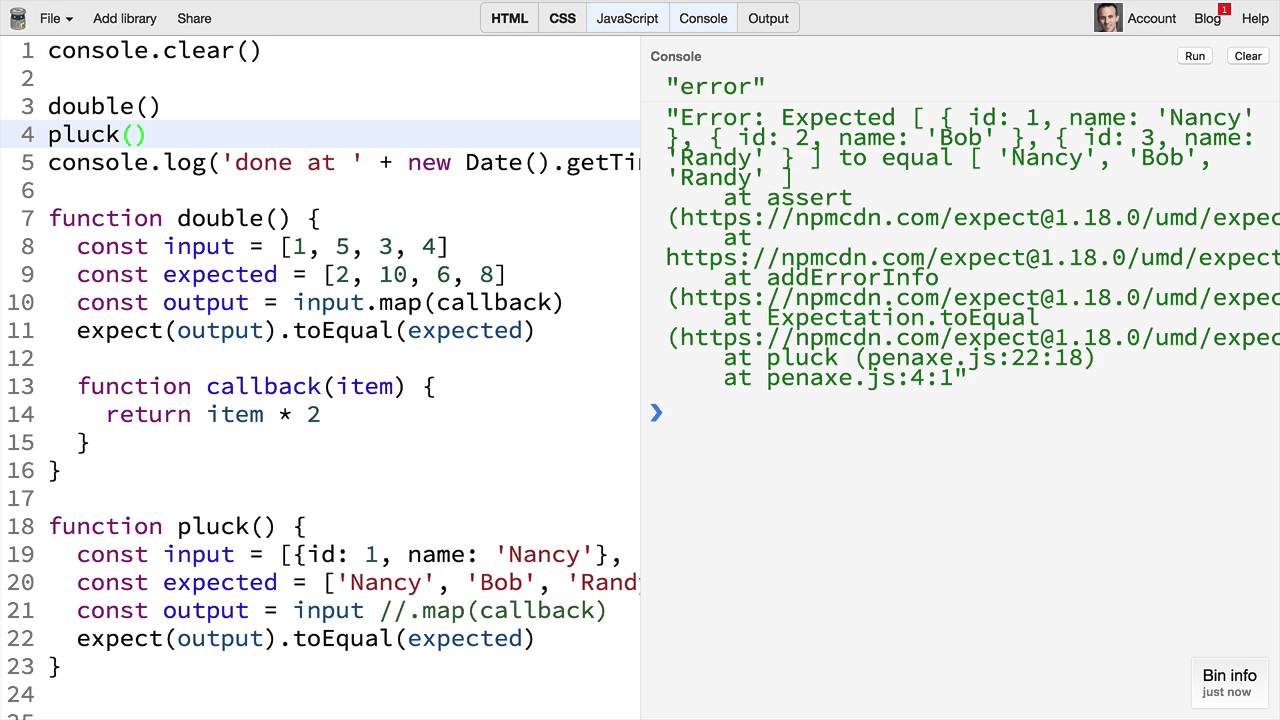
Javascript es6 map function. The map () method creates a new array with the results of calling a function for every array element. The map () method calls the provided function once for each element in an array, in order. map () does not execute the function for empty elements. map () does not change the original array. In this video, I discuss two array functions in JavaScript: map() and fill().🎥 Next Video: https://youtu.be/-LFjnY1PEDA🔗 MDN's Array Documentation: https:/... ES6 provides a new collection type called Map that addresses these deficiencies. By definition, a Map object holds key-value pairs where values of any type can be used as either keys or values. In addition, a Map object remembers the original insertion order of the keys. To create a new Map, you use the following syntax:
The map () function creates a new array from another array. It uses the same elements from the parent array and creates a new array of elements by calling a function. In many different ways you can use the map () function in JavaScript. I am sharing few examples here. JavaScript ES6 Map and Reduce Patterns. ... Generally speaking, I turn to the map function if I need to transform some Array of one object type into another Array of a different object type. Likewise, I use the reduce function if I find it necessary to take an array of objects and boil it down to a non-array structure (either a primitive or ... JavaScript arrow functions arrived with the release of ECMAScript 2015, also known as ES6. Their concise syntax and handling of the this keyword have made JavaScript arrow functions an ES6 favorite...
Recursion in JavaScript with ES6, destructuring and rest/spread by@hugo__df. ... Map is a function that takes a list and a function and returns a list containing the result of a function application to each element of the list. function map([ head, ... The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value. Javascript ES6 Previous Next ... JavaScript Map Objects. Being able to use an Object as a key is an important Map feature. Example ... ES6 allows function parameters to have default values. Example. function myFunction(x, y = 10) { // y is 10 if not passed or undefined
@neezer no, it doesn't. .forEach returns undefined flat out, all the time, as the expected use of forEach is a simple side-effect based iteration (same as it has been since ES5). In order to use that forEach, you'd want to build an array and populate it by hand, either through said forEach or through the iterator returned by .entries() or .keys( ) or .values( ). The map() method in JavaScript creates an array by calling a specific function on each element present in the parent array. It is a non-mutating method. Generally map() method is used to iterate over an array and calling function on every element of array. Syntax: array.map(function(currentValue, index, arr), thisValue) Before JavaScript ES6, ... But for the most part, arrow functions are usually used for array manipulation with some methods such as map(), filter() ... As a whole, the arrow function is one of the most favorable features of ES6, with bits of help of arrow functions, your code can look more neat and concise. ...
The conversion to and from JSON #. With these helper functions, the conversion to JSON works as follows: function strMapToJson(strMap) { return JSON.stringify (strMapToObj (strMap)); } function jsonToStrMap(jsonStr) { return objToStrMap (JSON.parse (jsonStr)); } This is an example of using these functions: Array.prototype.map (callback, thisValue); This function have three arguments for the callback (currentValue, index, array) currentValue -> required, the current element being processed in the... The map is a one of the new class introduced in Es6 version. Other Classes are Set, WeakSet and WeakMap The map is a data structure with key and value. Keys can be any arbitrary value and values can be an object or primitive types. Keys can be a Primitive type like Strings, numbers, Symbols, Functions, Objects, and Dates.
ES6 - Functions. Functions are the building blocks of readable, maintainable, and reusable code. Functions are defined using the function keyword. Following is the syntax for defining a standard function. To force execution of the function, it must be called. This is called as function invocation. June 10, 2021January 12, 2020 by Lane Wagner The built-in JavaScript map function returns a new array, where each element in the new array is the result of the corresponding element in the old array after being passed through a callback function. If you're starting in JavaScript, maybe you haven't heard of .map(), .reduce(), and .filter().For me, it took a while as I had to support Internet Explorer 8 until a couple years ago.
The map function is one of the most commonly used functions to manipulate array elements. The map () method iterates an array and modifies the array elements using the callback method and returns the new array. Learn How to use map function in JavaScript Suppose you have the below array and you want to modify its element and add $10 to each item. With ES6, there were many updates to the JavaScript, including the spread operator, object destructuring, new type of variables, and more. One of the most notable changes were arrow functions, a new and concise way to write functions. With arrow funct The Array.prototype.map() method was introduced in ES6 (ECMAScript 2015) for iterating and manipulating elements of an array in one go. This method creates a new array by executing the given function for each element in the array. The Array.map() method accepts a callback function as a parameter that you want to invoke for each item in the array. This function must return a value after ...
In JavaScript pre-ES6 we have function expressions which give us an anonymous function (a function without a name). var anon = function (a, b) { return a + b }; In ES6 we have arrow functions with a more flexible syntax that has some bonus features and gotchas. How to Sort a Map by Object Key in JavaScript ES6. After the last code example, you might have some guesses about how you could sort a Map by converting the Map to an array: Convert the Map to an array; Use the array's .sort() function; Convert the array back to a Map; Indeed, that's the easiest way to sort a Map in JavaScript. ES6 Functions A function is the set of input statements, which performs specific computations and produces output. It is a block of code that is designed for performing a particular task. It is executed when it gets invoked (or called).
The JavaScript ES6 has introduced two new data structures, i.e Map and WeakMap. Map is similar to objects in JavaScript that allows us to store elements in a key/value pair. The elements in a Map are inserted in an insertion order. However, unlike an object, a map can contain objects, functions and other data types as key. Array.prototype.map () The map () method creates a new array populated with the results of calling a provided function on every element in the calling array.
Functional Programming In Js Map Filter Reduce Pt 5
Performance Lodash Vs Es6 Map Hacker Noon
Learn Amp Understand Javascript S Map Function By Brandon
A Beginner S Guide To Arrow Functions In Es6 Part 2 By Joe
The Function Map In Javascript Es6 By Yoel Macia The
Javascript Array Map Example Array Prototype Map
Javascript Map Amp Filter Dev Community
12 Map Weakmap Es6 Javascript Typescript
Omit Empty Value In Map Javascript Code Example
Maps Vs Objects In Es6 When To Use Stack Overflow
Javascript Array Map Tutorial How To Iterate Through
Es6 10 Map Function Step By Step Tutorial For Es6
Deep Dive Into Javascript S Array Map Method
16 6 Array Functions Map And Fill Topics Of Javascript Es6
Map In Javascript Geeksforgeeks
Simplify Your Javascript Use Map Reduce And Filter
Introduction To Map Reduce And Filter Function In
Es6 Arrow Function Syntax Explained Simply Dev Community
How To Use The Javascript Array Map Api
Underscore Js Map Function Vegibit
Es6 Collections Using Map Set Weakmap Weakset Sitepoint
What Is Es6 Javascript And How Is It Different Cloudsavvy It
Map Not Working As Expected On Uninitiated Array Stack Overflow
Map Vs Object In Javascript Stack Overflow
Maps In Javascript Es6 Dev Community
Es6 Keyed Collections Maps And Sets Logrocket Blog
Javascript Map Method Explained By Going On A Hike
Higher Order Array Functions Map Filter Reduce
0 Response to "30 Javascript Es6 Map Function"
Post a Comment