27 Javascript Functional Programming Example
In this post, I will tell you more about functional programming, and some important concepts, with a lot of code examples in JavaScript. What is functional programming? Functional programming is a programming paradigm — a style of building the structure and elements of computer programs — that treats computation as the evaluation of ... When Brendan Eich created JavaScript in 1995, he intended to do Scheme in the browser. Scheme, being a dialect of Lisp, is a functional programming language. Things changed when Eich was told that the new language should be the scripting language companion to Java. Eich eventually settled on a language that has a C-style syntax (as does Java), yet has first-class functions. Java technically ...
Functional Programming In Javascript With Practical
A Gentle Introduction to Functional JavaScript: Part 1. Written by James Sinclair on the 29 th January 2016. This is part one of a three four-part series introducing 'functional' programming in JavaScript. In this article we take a look at the building blocks that make JavaScript a 'functional' language, and examine why that might be ...
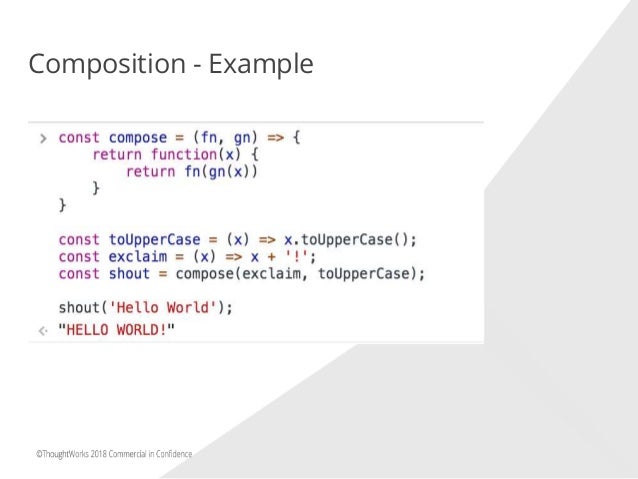
Javascript functional programming example. Jul 08, 2019 - Concepts of Functional Programming in Javascript. After a long time learning and working with object-oriented programming, I took a step back to think about system complexity.. Functional programming is declarative 7 ... write a book about functional programming in JavaScript. Because JavaScript is a lan-guage I'm very much obsessed with, to say the least, I was thrilled to jump into this opportunity. By writing this book, I hope to help you develop the same skills and take Functional programming is a programming paradigm that focuses on two core concepts. These two core concepts are: 1. avoiding mutating state and side effects. 2. using functions as the central building block of programs. There are a lot of other programming paradigms that exist, for example, Procedural Programming, Object Oriented Programming ...
Fogus helps you think in a functional way to help you minimize complexity in the programs you build. If you're a JavaScript programmer hoping to learn functional programming techniques, or a functional programmer looking to learn JavaScript, this book is the ideal introduction. Use applicative programming techniques with first-class functions I'm teaching you Functional Programming for the frontend, because programming on the front end can be quite challenging and Functional Programming is a particularly good fit for the front end. It's important to note, this course focuses on Modern JavaScript and doesn't use a front end framework, so you won't have to learn frontend technologies ... ¶ Functional programming, which is the subject of this chapter, produces abstraction through clever ways of combining functions. A programmer armed with a repertoire of fundamental functions and, more importantly, the knowledge on how to use them, is much more effective than one who starts from scratch.
16/10/2019 · In the example below, we add a new function, addName, to the sequence, and we use our tap function from earlier to log the result to the console. const sayHello = () => 'Hello'; const addName = (name, str) => str + ' ' + name; const container = new Container(sayHello); const greet = container .map(addName.bind( this , 'Joe Bloggs')) .map(tap(console.log)); CodinGame is a challenge-based training platform for programmers where you can play with the hottest programming topics. Solve games, code AI bots, learn from your peers, have fun. JavaScript Functional Programming in Action Say we have an image editing application which allows people to add effects to pictures. A super-action to create a night-mode wallpaper may consist of ...
Popular programming languages that support functional programming techniques are JavaScript, Python, Ruby and many others. Functional Programming is not a new concept, actually its roots go back o the 1930's when lamda calculus was born, and has influenced many programming languages. What is Functional Programming. In computer science, functional programming is a programming paradigm or pattern (a style of building the structure and elements of computer programs) Functional Programming treats computation as the evaluation of mathematical functions. Functional Programming avoids changing-state and mutable data. Oct 14, 2018 - Several years ago, I found a helpful tutorial by Arnau Sanchez that showed how common procedural programming patterns could be replaced with a functional approach. The tutorial is in Ruby. Recently I was reminded of it, and I thought I'd convert some examples from that tutorial to JavaScript (the ...
Professor Frisby’s Mostly Adequate Guide to Functional Programming – This is a book on the functional paradigm in general using the world’s most popular functional programming language: JavaScript. It’s a practical introduction that builds up intuition through real-world examples. Actually JS can be used as a FP language as long as you take care of side effects, there is no builtin mechanism for that. The following code is an example of such a programming style. The "zipWith" function comes from the Haskell world. It merges two lists using the given function, as it happens, add (point [i], vector [i]). All the main functional programming types and patterns fully documented, tested, and with examples. cats dart functional functional-programming flutter functional-language functional-programming-examples dartlang fp-ts flutter-package dart-package dart-functional dart-functional-programming flutter-functional fpdart. Updated 2 days ago.
In other words, it should not create new bugs. Therefore, let's start off with Pure Functions and further elaborate about functional programming with examples of JavaScript programs. function addition(num1,num2) {. return num1 + num2; } addition(3,4); // output is 7 regardless of the number of the number of times being called. Functional Programming in JavaScript Explained in Plain English. Joel P. Mugalu. One of the hardest things you have to do in programming is control complexity. Without careful consideration, a program's size and complexity can grow to the point where it confuses even the creator of the program. In fact, as one author put it: It solves the problem. Now here is the same snippet in a more functional way: function average (sum, count) { return sum / count; } function sum (x, y) { return x + y; } var coords = data.map (function (item) { return [ average (item.temperatures.reduce (sum), item.temperatures.length), item.population ]; }); Obviously, as @Fabio has pointed ...
Jul 23, 2020 - In this article, we will consider the following concepts of Functional Programming in JavaScript with simple code examples: ... Higher-order functions. Functional Programming is a programming paradigm where you mostly construct and structure your code using functions. These functions take input which is called as arguments then shows the output based on the inputs being taken which, given the same input always results in the same output. But functional programming is much more than "simple" functions. This paradigm also offers abstract concepts that may differ from language to language — functions as parameters, functions as returns, currying, monads, high-order functions, and so on are terms related to functional style.
The goal is to create a function to append data from an array to a table. The function will be built out of basic JavaScript functions and some functional programming constructs that are also implemented in JavaScript. This process will allow the testing of each part of the function and then the parts will be combined together. Functional Programming in JavaScript is more like a style, rather than a paradigm. This JavaScript Functional Programming Tutorial was made to demonstrate it... Nov 18, 2020 - Start here, with freeCodeCamp's introduction to functional programming with JavaScript. Look here for some libraries you can include and play around with, to really master functional programming.
Examples in JavaScript Functional Programming: Part 4. John Tucker. Follow. Jan 18, 2018 · 5 min read. Exploring performance differences between classes and factories (and functional mixins). This article is part of a series starting with Examples in JavaScript Functional Programming: Part 1. Rather than being standalone articles, they are ... Functional Programming was possible in Javascript just because of the first-class usage. It made the code more readable and boost the execution. Examples of functional programming boosting the execution performance are angular and react. The procedural loops can be replaced with functions that make coding, reading, and debugging more easier. Functional programming (also called FP) is a way of thinking about software construction by creating pure functions. It avoid concepts of shared state, mutable data observed in Object Oriented Programming. Functional langauges empazies on expressions and declarations rather than execution of statements. Therefore, unlike other procedures which ...
In Javascript, functions can also be defined as expressions. For example, // program to find the square of a number // function is declared inside the variable let x = function (num) { return num * num }; console.log(x(4)); // can be used as variable value for other variables let y … Nov 14, 2016 - by rajaraodv Functional Programming In JavaScript — With Practical Examples (Part 1)Functional Programming(FP) can change the way you program for the better. But it’s hard to learn and many posts and tutorials don’t go into details like Monads, Applicative and so on and don’t seem to 1 week ago - For example, the composition f . g (the dot means “composed with”) is equivalent to f(g(x)) in JavaScript. Understanding function composition is an important step towards understanding how software is constructed using the functional programming. Read “What is Function Composition?” ...
Basic Functional Programming Patterns in JavaScript . Several years ago, I found a helpful tutorial by Arnau Sanchez that showed how common procedural programming patterns could be replaced with a functional approach. The tutorial is in Ruby. Recently I was reminded of it, and I thought I'd convert some examples from that tutorial to JavaScript (the text of this article, however, is original ... Dec 29, 2019 - My aim is that after you read this article, you understand what is a functional programming. There is... Tagged with javascript, functional. Jan 15, 2020 - Functional Programming has been around for much longer than OOP. The best example is LISP, which its first specification was written in 1958. However, unlike OOP it’s not as simple to find developers who can understand functional concepts such as Purity, Currying, or Function Composition. Javascript ...
Functional Programming in JavaScript JavaScript already has some functions that enable functional programming. Example: String.prototype.slice, Array.protoype.filter, Array.prototype.join. On the other hand, Array.prototype.forEach, Array.prototype.push are impure functions. Note: This is part of the "Javascript and Functional Programming" series on learning functional programming techniques in JavaScript ES6+. Checkout the previous post on function currying <Part 4>. Start from the beginning here. Let's get practical! Previously, we examined higher order functions. JavaScript and Functional Programming. JavaScript encourages and provides possibilities to write functional code. Right now, we will take a look at functionalities that will allow us to write better applications. Const variables. Since ECMAScript2015 you can use const and let to declare your variables.
The What Why And How Of Javascript Functional Programming
Functional Programming In Javascript Dev Community
How Is Functional Programming Different From Object Oriented
Transition To Functional Programming For Java Developers
Functional Programming In Javascript Amp Esnext
Functional Programming In Javascript Learn Pure Amp Impure
Functional Programming Simulate Curry In Abap Sap Blogs
Don T Object Ify Me An Introduction To Functional
Lukcodeio On Twitter Today We Continue Tips In Functional
Beginner S Guide To Functional Programming In Javascript
Functional Programming In Javascript By Nc Patro Codeburst
Functional Programming By Charles Scalfani Pdf Ipad Kindle
Functional Programming In Javascript
Javascript And Functional Programming Pt 2 First Class
Taste Of Functional Programming In Javascript By Dmitry
From Map Reduce To Javascript Functional Programming
Functional Programming Freecodecamp Freya Yuki
Examples In Javascript Functional Programming Part 3 By
Master The Javascript Interview What Is Functional
A Simple Explanation Of Javascript Closures
Functional Programming In Javascript Introduction And
Practical Javascript Functional Programming
Functional Programming Simulate Curry In Abap Sap Blogs
When Functional Programming Isn T Functional Leadingagile
Functional Programming In Javascript How And Why By Sonny
First Steps Into Functional Programming In Javascript
0 Response to "27 Javascript Functional Programming Example"
Post a Comment