31 Get Mouse Position Javascript Canvas
The dimension of the canvas is found using the getBoundingClientRect () function. This method returns the size of an element and its position relative to the viewport. The position of x-coordinate of the mouse click is found by subtracting the event's x position with the bounding rectangle's x position. 30/5/2014 · // Creates an object with x and y defined, // set to the mouse position relative to the state's canvas // If you wanna be super-correct this can be tricky, // we have to worry about padding and borders // takes an event and a reference to the canvas function getMouse = function(e, canvas) { var element = canvas, offsetX = 0, offsetY = 0, mx, my; // Compute the total offset.
How To Find Mouse Coordinates In Html5
Lesson Code: http://www.developphp /video/JavaScript/Detect-Mouse-Coordinates-on-Canvas In this exercise we are going to cover one of the most crucial asp...
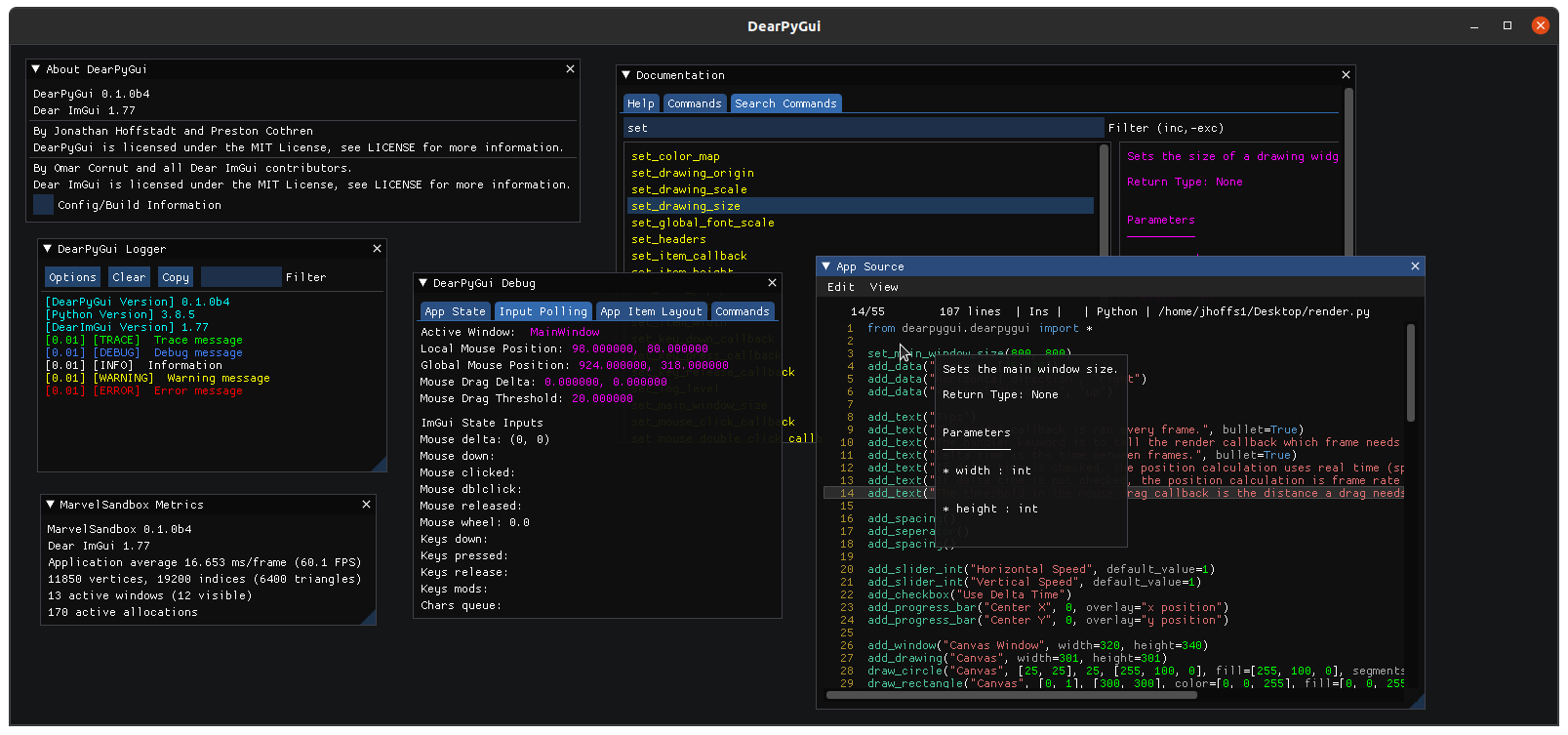
Get mouse position javascript canvas. Javascript: Canvas mouse position Raw mouse_position.js // Add mousePosition on the Canvas element (function {function mousePosition (event) {var totalOffsetX = 0, totalOffsetY = 0, coordX = 0, coordY = 0, currentElement = this, mouseX = 0, mouseY = 0; // Traversing the parents to get the total offset: do Finding out the mouse click position on a canvas with Javascript I'm playing around with the HTML5 <canvas> element. One interesting thing to do is interact with the canvas using the mouse. First we attach the mouse click event to the canvas (suppose we have an HTML page with a canvas element named canvas, and Game is a global "namespace object"): 16/6/2013 · The easiest way to compute the correct mouse click or mouse move position on a canvas event is to use this little equation: canvas.addEventListener('click', event => { let bound = canvas.getBoundingClientRect(); let x = event.clientX - bound.left - canvas.clientLeft; let y = event.clientY - bound.top - canvas.clientTop; context.fillRect(x, y, 16, 16); });
Since we cannot make actual mouse pointer using JavaScript, we use an image as a cursor. Suppose variables x, y, px, py, x1, x2. x: x-position of the actual mouse pointer y: y-position of the actual mouse pointer x1: x-position where we want the mouse to appear x2: y-position where we want the mouse to appear Now, let x + px = x1 px = x1 - x ... Real mouse position in canvas, I have read there's a way of creating a simple function in JavaScript to get the right position, but I have no idea about how to do it. share. Subtract the X and Y offsets of the canvas DOM element from the mouse position to get the local position inside the canvas. Apr 03, 2017 - A common question, but I still need help. I'm trying to get and store the mouse coordinates when someone clicks within the canvas. my HTML
Default or (0, 0) position is at top left corner. Problem is to adjust x and y position values on zoom in/out so that no matter what the current position of the view is, it will stay at point of zoom or mouse cursor. Scaling and translating is executed in draw function by x, y and zoom values so those values must be modified directly (can't run ... How to get mouse position in Javascript. GitHub Gist: instantly share code, notes, and snippets. To draw on the canvas, we first press the mouse (mousedown event) and then move the mouse (series of mousemove events). As we move the mouse, a line is drawn on the canvas between the current and the previous mouse position. When we release the mouse (mouseup event), drawing stops. We will demonstrate this application using Mouse Events, Touch ...
16/2/2014 · An easy solution is to use the getBoundingClientRect method of the canvas object. This returns a rectangle object that gives you the current offset of the canvas relative to the page. Here is an example usage inside a mousemove event to get the accurate mouse position: window.addEventListener('mousemove', mouseMoveEvent); function mouseMoveEvent(e) The e.clientX and e.clientY will get the mouse positions relative to the top of the document, to change this to be based on the top of the canvas we subtract the left and right positions of the canvas from the client X and Y. var canvas = document.getElementById ("myCanvas"); var ctx = canvas.getContext ("2d"); ctx.font = "16px Arial"; canvas. The example listens for mousemove or touchmove events on the canvas to track the mouse or finger position on the canvas. The example also listens for mousedown and mouseup, or touchstart and touchend, to determine if the mouse button or finger is down.Note that the mouse is still tracked when the mouse button is released, but when the finger is lifted off the screen, there is no touch to track.
To draw a circle on a canvas, use the following methods: beginPath () - begins a path. arc (x,y,r,startangle,endangle) - creates an arc/curve. To create a circle with arc (): Set start angle to 0 and end angle to 2*Math.PI. The x and y parameters define the x- and y-coordinates of the center of the circle. The r parameter defines the radius of ... Canvas How to - Display mouse position when mouse move. Back to Event ↑ ... We could use the following code snippet to get the coordinates of the mouse when the mouse entered the space of the canvas element: document.getElementById ('c-container').addEventListener ('mousemove', e => { this.setHoverCoordinates (e.clientX, e.clientY); })
We change our position to the initial position and move the canvas point to the moved position. Then we draw a line between these two points. Last we call the stroke to colour it. That's it. With this code can draw lines on an HTML canvas with our mouse. If you want to read more about the canvas element, check out these articles: 19/8/2021 · Canvas Resize Via Css And Mouse Position Issue 1661 Creating And Drawing On An Html5 Canvas Using Javascript By Javascript React Canvas Detect Bounding Area At Mouse Click The clientX property returns the horizontal coordinate (according to the client area) of the mouse pointer when a mouse event was triggered. The client area is the current window. Tip: To get the vertical coordinate (according to the client area) of the mouse pointer, use the clientY property. Note: This property is read-only.
To get the mouse coordinates relative to an HTML5 Canvas, we can create a getMousePos() method which returns the mouse coordinates based on the position of the client mouse and the position of the canvas obtained from the getBoundingClientRect() method of the window object. Instructions: Mouseover the canvas to see the mouse coordinates Feb 11, 2020 - GitHub Gist: instantly share code, notes, and snippets. The reason is (besides me being horrifically lazy), you can see my full explanation in the Get an Element's Position Using JavaScript tutorial instead. The getPosition function I used here was stolen from that tutorial, and it is a good read if you want to understand more about how positions in HTML can be calculated.
The canvas element relative position of a mouse click, touch start, or similar event can be obtained with the use of the getBoundingCLientRect method of the event target element which in this case out be the canvas element. get mouse position javascript . javascript by Ankur on Apr 14 2020 Donate Comment . 3 access mouse position javascript . javascript by Real Raccoon on May ... html canvas get mouse position; get mouse click coordinates canvas js; javascript get mouse position without event; window mouse position js; Get last news, demos, posts from Konva. CAD Systems Canvas Editor Simple Window Frame Window Frame Designer Seats Reservation Drawing Labels on Image Interactive Building Map Games and Apps Wheel of Fortune Free Drawing Animals on the Beach Game Planets Image Map Physics Simulator Common use cases Editable Text Rich Text rendering Canvas Scrolling Gif Animation Display Video SVG on Canvas ...
I have a small JS application that allows a user, after clicking on a canvas, to draw a picture. Once the user clicks the mouse, the user is allowed to drag the mouse wherever they want within the canvas and a line will be drawn from where they started moving the mouse to where the mouse stopped. Only by clicking again will the drawing cease. HTML5 Canvas Mouse Coordinates Tutorial, I have read there's a way of creating a simple function in JavaScript to get the right position, but I have no idea about how to do it. share. For situations where the canvas element is 1:1 compared to the bitmap size, you can get the mouse positions ... Video: Detect Mouse Coordinates on Canvas. This tutorial resides in the JavaScript video index under the Canvas Programming section. If you find this lesson useful, we have many more exercises that are sure to please you.
I am trying to use this method for getting the position of a mouse event inside an image regardless of how the user has zoomed in or out (Which this method accomplishes successfully!) Awesome... but my issue is that I need the method to work without using canvases as I am trying to have a website ... //set up the canvas and context ... }, false); //Get Mouse Position function getMousePos(canvas, evt) { var rect = canvas.getBoundingClientRect(); return { x: evt.clientX - rect.left, y: evt.clientY - rect.top }; } ... CodePen requires JavaScript to render the code and ... To draw points on a canvas when the user clicks on it, we need to retrieve the click event and get the coordinates of that click.To get the mouse coordinates relative to an HTML5 Canvas, we can create a getPosition() method which returns the mouse coordinates (x,y) based on the position of the client mouse and the position of the canvas ...
Learn to transform the mouse cursor into transformed canvas coordinates, allowing you to drag, zoom to cursor, and interact with graphics in the canvas regardless of the canvas's current transformation state. Extremely useful for html games and image editing programs. This post takes a modern approach to solving the problem using vanilla JavaScript, and explains the solution simply and in detail. Get Mouse Position using HTML5/JavaScript. Archived Forums A-B > Building Windows Store apps with HTML5/JavaScript (archived) ... I am clicking the canvas element and want to get mouse coordinates of the mouse. How can I do this? Thank you! Microsoft Student Partner. Thursday, April 26, 2012 8:57 PM. Mar 19, 2018 - Entonces con este código somos capaces ahora de detectar cada vez que el mouse se mueve sobre nuestro canvas. Pero tranquilo, que esto asi no nos lleva a ningún lado. El siguiente paso es detectar las coordenadas del mouse sobre el canvas y esto es lo que nos devuelve la función getMousePos.
Dealing with mouse position in JavaScript is annoying. So as a public service, I offer this page which has JavaScript examples for finding the coordinates of the mouse for different reference points. You're welcome. Finding the position of the mouse relative to the screen How To Lock An Angle When Drawing On Canvas In Javascript By Thang Minh Vu We Ve Moved To Freecodecamp Org News Medium. Listen To Mouse Click Event For Div Element In Javascript. ... Cannot Get Mouse Position In Dragover Event Issue 1925 Jquery Jquery Github. Macro Recorder Mouse Keyboard Actions. 8/8/2018 · Canvas mode's API has a full-featured mouse event system built right in.EaselJS v1.0.0 API Documentation : MouseEvent EaselJS Tutorial: Mouse InteractionExample code for reporting mouse click location:stage.addEventListener("stagemousedown", mouseClicked);function mouseClicked(evt) { console.log(evt.stageX, evt.stageY);}If the canvas can scale, just divide the coordinates by stage.scaleX and stage.scaleY to get …
Feb 19, 2021 - This is the 9th step out of 10 of the Gamedev Canvas tutorial. You can find the source code as it should look after completing this lesson at Gamedev-Canvas-workshop/lesson9.html. The game itself is actually finished, so let's work on polishing it up. We have already added keyboard controls, ... How to find relative mouse position? In some cases you may need to find position of a point relative to a node. For purpose we can use mathematical Konva.Transform methods. In this demo we have deep nesting transformed nodes: moved stage, scaled layer, rotated group. Now we want to add circles into the group on click. Get mouse position relative to canvas in JS. GitHub Gist: instantly share code, notes, and snippets.
Canvas Resize Via Css And Mouse Position Issue 1661
How To Show Mouse Coordinates Over Canvas Using Pure
Javascript Get Mouse Coordinates On Mouse Click
Html5 Canvas Mouse Interactions Medium
Mouse Position Canvas Vps And Vpn
Creating And Drawing On An Html5 Canvas Using Javascript By
Mouse Position Not Working While Dragging With Left Mouse
How To Calculate Mouse Move Y Coordinates In Javascript Code
Canvas Rotation Based On Mouse Position Facebook
How To Get The Pixel Color From A Canvas On Click Or Mouse
How To Lock An Angle When Drawing On Canvas In Javascript
Creating And Drawing On An Html5 Canvas Using Javascript By
Creating A Shape At Cursor Position Omnigraffle Automation
Paper Js Gap Between Cursor And Canvas Stack Overflow
Javascript Track Mouse Position Stack Overflow
A Gentle Introduction To Making Html5 Canvas Interactive
Banal Mus Castigător Mouse Coords On Canvas Lmvdesigns Com
Get Mouse Position In Javascript X And Y Javascript Tutorial For Beginners
Find Position Of Objects Drawn In Html5 Canvas Stack Overflow
Returning Un Transformed Mouse Coordinates After Rotating An
Still Can T Get Raycaster To Work On Non Page Filling Canvas
Working With Mouse Coordinates Canvas Cookbook
Jquery Plugin To Set Cursor Position Within Editable Content
Create A Game Ui With The Html5 Canvas Gamedev Academy
How To Lock An Angle When Drawing On Canvas In Javascript
Javascript Game Mouse Controls Screen Coordinates Javascript Mouse Position Events Canvas
Making And Moving Selectable Shapes On An Html5 Canvas A
Create Quick And Powerful Guis Using Dear Pygui In Python
0 Response to "31 Get Mouse Position Javascript Canvas"
Post a Comment