35 Singleton Object In Javascript
We can create singleton objects with a class like syntax. The decorator pattern lets us extend the functionality of constructors. Related Posts. Object-Oriented JavaScript — Events. ... ← Object-Oriented JavaScript — Private Entities and Chaining ... 20/3/2017 · Singleton object in JavaScript 20 March 2017 by Paul Schaeflein I developed a set of components that all read from the User Profile store. They are each designed to be independent, but are likely to be used together in a few variations.
Javascript Design Patterns 1 Singleton And The Module
The Singleton pattern is a design pattern that restricts the instantiation of a class to one object. After the first object is created, it will return the reference to the same one whenever called for an object. var Singleton = (function () { // instance stores a reference to the Singleton var instance; function createInstance () { // private ...
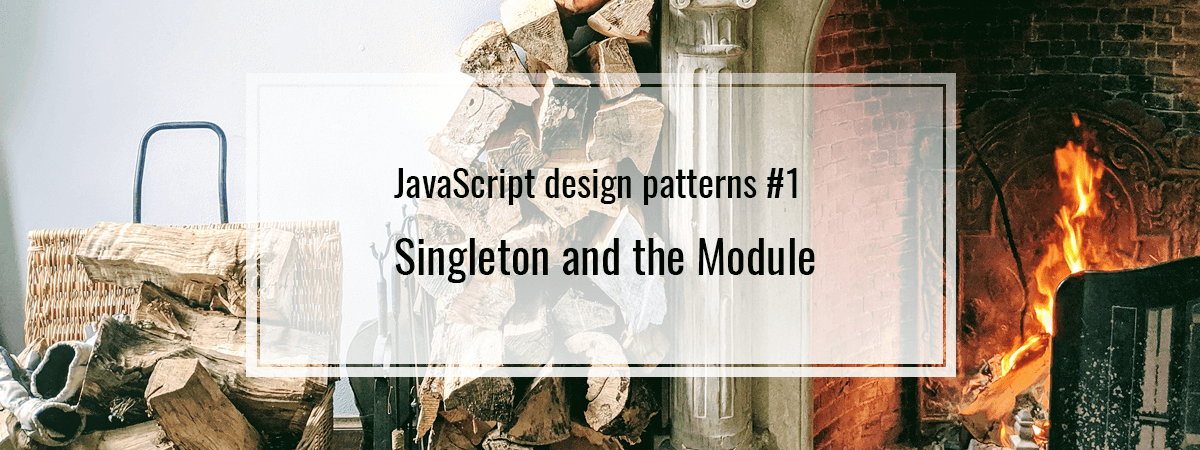
Singleton object in javascript. The Module pattern (see our Dofactory JS product) is JavaScript's manifestation of the Singleton pattern. Several other patterns, such as, Factory, Prototype, and Façade are frequently implemented as Singletons when only one instance is needed. Singleton Class in Java. In object-oriented programming, a singleton class is a class that can have only one object (an instance of the class) at a time. To design a singleton class: Make constructor as private. Write a static method that has return type object of this singleton class. A singleton is a class that allows only a single instance of itself to be created and gives access to that created instance. It contains static variables that can accommodate unique and private instances of itself. We use it in scenarios when a user wants to restrict instantiation of a class to only one object.
One of the most popular creational design pattern is the Singleton, which restricts instantiation of a class to a single object only. In this post post, I will show you how to implement the... 1. JavaScript design patterns #1. Singleton and the Module2. JavaScript design patterns #2. Factories and their implementation in TypeScript3. JavaScript design patterns #3. The Facade pattern and applying it to React Hooks4. JavaScript design patterns #4. Decorators and their implementation in TypeScript5. JavaScript design patterns #5. The Observer pattern with TypeScriptWhile having a vibe ... The Singleton object is implemented as an IIFE. An IIFE (Immediately Invoked Function Expression) is a JavaScript function that runs as soon as it is defined. The createInstance function is responsible for creating the instance of MyClass. The getInstance method will be invoked via the Singleton object.
It is wasting time and resources to create this object every time when the application needs to grab some config info. In javascript, there is a lot of ways to create a Singleton object. 1. Object literals, the simplest form of javascript singleton object. All javascript object literals are singleton objects. The singleton is one of the most well-known design patterns. There are times when you need to have only one instance of a class and no more than that. This one class could be some kind of resource manager or some global lookup for values. This is where singletons come in. Edit · Apr 16, 2014 · 5 minutes read · Follow @mgechev Design patterns JavaScript Singleton Wikipedia describes the singleton design pattern as: The singleton pattern is a design pattern that restricts the instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system.
Here is a simple example to explain the singleton pattern in JavaScript. var Singleton = (function() { var instance; var init = function() { return { display:function() { alert("This is a singleton pattern demo"); } }; }; return { getInstance:function(){ if(!instance){ alert("Singleton check"); instance = init(); } return instance; } }; })(); // In this call first display alert("Singleton check") // and then alert("This is a singleton pattern demo"); // It means one object … The Singleton pattern is used to design the classes which provides the configuration settings for an application. By implementing configuration classes as Singleton not only that we provide a global access point, but we also keep the instance we use as a cache object. What is a singleton? Singleton is an object which can only be instantiated once. A singleton pattern creates a new instance of the class if one doesn't exist. If an instance already exists, it returns the reference to that object.
This post argues that the singleton pattern is usually not needed in JavaScript, because you can directly create objects. It then slightly backpedals from that position and shows you code skeletons that you can use if your needs go beyond the basics. Understanding singleton object in Javascript. 0. How does one manipulate a variable in a prototype? 0. Will this object always re-call the function? 0. how to use WinRT C# Singleton in Javascript. Related. 7626. How do JavaScript closures work? 5174. What is the most efficient way to deep clone an object in JavaScript? The Singleton Design Pattern is one of the creational design patterns. This is used for scenarios where only one object is needed globally, such as global caches, thread pools, window objects, etc.
Singleton in JavaScript Singleton is one of the better-known patters in programming. While, by some, seen as an anti-pattern, it is worth knowing something about it. Creating such class isn't really hard, but has some caveats. Basic JavaScript Singleton. Taking in account that in JavaScript you can instantiate a object without defining a class, the most simple singleton will look like: Singleton = {} There will be only one unique instance of this object. But it's simplicity bring many possible drawbacks: It can be rewritten A Singleton is an object which can only be instantiated one time. Repeated calls to its constructor return the same instance and in this way one can ensure that they don't accidentally create, say, two Users in a single User application. Doesn't sound too bad, right? Well, if you're responsible then it arguably is OK but there are many caveats.
Enforcing Singleton-ness. Good luck with that. In Javascript, an object is an object is an object, and since objects have no type and there is no access control, there is really nothing stopping any client from simply var newInstance = {} and copying over whatever state it can get its hands on. Properties A singleton is an object-oriented software design pattern which ensures a given class is only ever instantiated once and can be quite useful in many different situations, such as creating global objects and components shared across an application. There are various ways to create a singleton class/function in javascript. Do note that we will use the ES6+ class notation for all examples. This will help you carry your understanding to other languages like c#, java etc (though not exactly but enough to understand other language nuisances and adapt as needed).
a. Typescript and private constructors. → As the aim of the singleton is to create only one instance. Typescript has access modifier concept (i.e private, public, and protected). → So instead of creating instances outside, we can make a private constructor and create an instance inside the class. NOTE: In JavaScript, we have only a public ... For the full set, see JavaScript Design Patterns. A Singleton only allows for a single instantiation, but many instances of the same object. The Singleton restricts clients from creating multiple objects, after the first object created, it will return instances of itself. Finding use cases for Singletons is difficult for most who have not yet ... 20/4/2018 · The old way of writing a singleton in JavaScript involves leveraging closures and immediately invoked function expressions . Here’s how we might write a (very simple) store for a hypothetical ...
In JavaScript, there's one main technical advantage for singleton: lazy-loading. Unlike global variables, our singleton will be instantiated only when we first need it. Whether this is a meaningful...
Design Patterns In Typescript And Node Js Logrocket Blog
Javascript Design Pattern Singleton Pattern Develop Paper
Javascript Html5 React Js Chrome Node Js Javascript Design
What Are Drawbacks Or Disadvantages Of Singleton Pattern
Creating A True Singleton In Node Js With Es6 Symbols
Comprehensive Guide To Javascript Design Patterns Toptal
Javascript Singleton Design Pattern Reality On Web
Design Patterns In Javascript Node 2020 By Shivam Gupta
Ozenero Mobile Amp Web Programming Tutorials
Design Patterns In Javascript The Singleton Pattern
Explanation Of Singleton Design Pattern In C Thecodeprogram
Singleton Pattern Positive And Negative Aspects Codeproject
Design Patterns In Typescript Singleton By Cesar William
Mastering Javascript Creating A Singleton Packtpub Com
Javascript Design Patterns Singleton And Modules Girls In Code
Thread Safe And A Fast Singleton Implementation Singleton
Singleton Pattern In Javascript Duc Tran David Tran
Javascript Design Patterns Singleton Pattern
The Good The Bad And The Singleton
Js Singleton Pattern Javascript Webprogramming
A Comprehensive Guide To Javascript Design Patterns
Singleton Design Pattern Implementation Geeksforgeeks
Difference Between Singleton Pattern And Static Class In Java
Singleton Design Pattern In Java Codespeedy
Object In Kotlin And The Singleton Pattern Raywenderlich Com
Javascript Design Patterns Digitalocean
A Comprehensive Guide To Javascript Design Patterns
Design Pattern Series Singleton And Multiton Pattern
Singleton Design Pattern Introduction Geeksforgeeks
Singleton Design Pattern In Java Top Java Tutorial
Object Oriented Javascript Singletons And Decorators
Singleton Design Patterns Javatpoint
How To Create Objects In Javascript
0 Response to "35 Singleton Object In Javascript"
Post a Comment