31 Javascript How To Add Class
In the following example, we first define a class named Polygon, then extend it to create a class named Square.. Note that super(), used in the constructor, can only be used in constructors, and must be called before the this keyword can be used. Class Methods. Class methods are created with the same syntax as object methods. Use the keyword class to create a class. Always add a constructor() method. Then add any number of methods.
Jquery Addclass Not Adding Class To Html Element Stack
The class name attribute can be used by CSS and JavaScript to perform certain tasks for elements with the specified class name. Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. Syntax: element.className += "newClass";
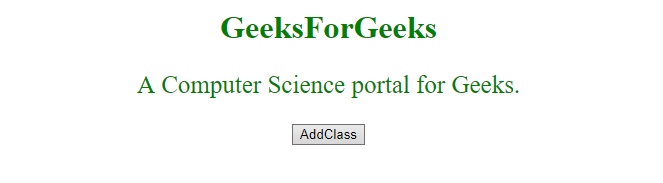
Javascript how to add class. 19/12/2016 · // Create a div and add a class var new_row = document.createElement("div"); new_row.className = "aClassName"; // Add another class. A space ' ' separates class names new_row.className = "aClassName anotherClass"; // Another way of appending classes new_row.className = new_row.className + " yetAClass"; In JavaScript, the standard way of selecting an element is to use the document.getElementById("Id"). Of course, it is possible to obtain elements in other ways, as well, and in some circumstances, use this. For replacing all the existing classes with a single or more classes, you should set the className attribute, as follows: In the example above, the addNewClass() function adds a new class highlight to the DIV element that already has a class box without removing or replacing it using the className property.
A JavaScript class is a type of function. Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. const x = function() {} In JavaScript, adding a class name can be done in a couple of ways. First, we select the desired HTML element. Then, we have a choice to either use the className property or the add () method to add the class name to the element. We will go through the syntax and use in the next section. 2/12/2019 · Javascript Front End Technology Object Oriented Programming. To add a class to a DOM element, you first need to find it using a querySelector like querySelector, getElementById, etc. Then you need to add the class. For example, if you have the following HTML −.
How it works: First, select the div element with the id content using the querySelector() method.; Then, iterate over the elements of the classList and show the classes in the Console window.; 2) Add one or more classes to the class list of an element. To add one or more CSS classes to the class list of an element, you use the add() method of the classList.. For example, the following code ... In this article, we are discussing how to add a class to an element using JavaScript. In JavaScript, there are some approaches to add a class to an element. We can use the .className property or the .add () method to add a class name to the particular element. The static keyword defines a static method or property for a class. Neither static methods nor static properties can be called on instances of the class. Instead, they're called on the class itself. Static methods are often utility functions, such as functions to create or clone objects, whereas static properties are useful for caches, fixed-configuration, or any other data you don't need to ...
May 18, 2020 - I am having a problem in how to declare the Script, I made. I want to add the class "inputfield_bordergreen" in the InputText_Field · i think you are doing ajax refresh after the RunJavaScript, due to which class is removing from your screen Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics. CSS class names can be removed or added using the classList method, or they can modified straight with the className property. ... classList is pretty smart and the most modern system of manipulating CSS class names in elements via JavaScript.
Mar 24, 2020 - Apply class element. ... Learn how Grepper helps you improve as a Developer! ... javascript create a function that counts the number of syllables a word has. each syllable is separated with a dash -. ... // How to create string with multiple spaces in JavaScript var a = 'something' + ... The class syntax does not introduce the new object-oriented inheritance model to JavaScript. Define a class in Javascript. One way to define the class is by using the class declaration. If we want to declare a class, you use the class keyword with the name of the class ("Employee" here). See the below code. Add a Class to an HTML Element Using className. The className property can be used to get or set the value of the class attribute of any DOM element. The returned string contains all the classes of current element separated by a space. We can simply use the += operator to append any new classes to our element.. JavaScript
In this video, you will learn how to add and remove class onclick in javascript. Also, you will learn how to check if class exists, toggle class and add or ... To add an additional class to an element: To add a class to an element, without removing/affecting existing values, append a space and the new classname, like so: document.getElementById("MyElement").className += " MyClass"; To change all classes for an element: To replace all existing classes with one or more new classes, set the className ... The addClass () method adds one or more class names to the selected elements. This method does not remove existing class attributes, it only adds one or more class names to the class attribute. Tip: To add more than one class, separate the class names with spaces.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Tutorials References Exercises Videos NEW Menu . ... // Add the active class to the current/clicked button To add a class to an element, you use the classList property of the element. Suppose you have an element as follows: < div > Item </ div > 8/8/2021 · Below is an example of using class methods in JavaScript. //create a new object. const john = new Student ('John', 'Brown', '2018'); //calling the report method and storing its result in a variable. let result = john.report (); //printing the result to the console. console.log (result);
Apply class element. ... This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id ... How to add JavaScript to html with javascript tutorial, introduction, javascript oops, application of javascript, loop, variable, objects, map, typedarray etc. ... JavaScript on Android What is a promise in JavaScript What is hoisting in JavaScript What is Vanilla JavaScript How to add a class to an element using JavaScript How to calculate the ...
11/5/2021 · The “class” syntax. The basic syntax is: class MyClass { constructor() { ... } method1() { ... } method2() { ... } method3() { ... } ... } Then use new MyClass () to create a new object with all the listed methods. The constructor () method is called automatically by new, so we can initialize the object there. Remove class names. Here's how to remove a single class name: const element = document.getElementById('foo') element. classList.remove('bar') Multiple class names can be removed by passing more parameters to the remove method: element. classList.remove('bar', 'baz') Or remove a given class name from all the elements at the same time; in this ... Jul 09, 2015 - Another way to alter the style of an element is by changing its class attribute. class is a reserved word in JavaScript, so in order to access the element’s class, you use element.className. You can append strings to className if you want to add a class to an element, or you could just overwrite ...
This is the css code injected into the page header: .current_class {background-color:green}; And this is the javascript code used to add the class to the td: var cell = $ ("#timetable_table").find ("tr").eq (current+1).find ("td").eq (date.getDay ()); I know cell is the correct td because i can cell.text ("foo") and the correct cell is modified ... Jul 20, 2021 - The class name attribute can be used by CSS and JavaScript to perform certain tasks for elements with the specified class name. Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. divtst specifies the element where you want to add a new class name. This is followed by the classList property. Then add method is used and the desired class name is provided in the parenthesis. The examples below show how to specify element and add CSS class in different elements.
Change the class name of an element ... and add a new class name. ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: ... Thank You For Helping Us! Your message has been sent to W3Schools. ... HTML Tutorial CSS Tutorial JavaScript Tutorial How ... How TO - Add a Class. PreviousNext . Learn how to add a class name to an element with JavaScript. Add Class. Click the button to add a class to me! Add Class. Step 1) Add HTML: Add a class name to the div element with id="myDIV" (in this example we use a button to add the class). Example. 4/3/2020 · The classList property works in all modern browsers, and IE10 and above. You can use the classList property to easily add, remove, and toggle CSS classes from an element in vanilla JavaScript. Say we have an element like below: <div class="hot spicy pizza"> 🍕 </div>
Add a CSS Class We will add another class called thornto it. To do so in JavaScript, we need to make use of an element's classListread-only property, which returns the DOMTokenListof the element. Aug 25, 2020 - If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with class Search instead for div id javascript id selector cobine with class How to combine class and ID in JQerry selector Tip: Also see How To Toggle A Class. Tip: Also see How To Add A Class. Tip: Learn more about the classList property in our JavaScript Reference. Tip: Learn more about the className property in our JavaScript Reference.
Oct 31, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. In this video tutorial, you will learn how to add active class in javascript. Add a class to the document body with JavaScript....
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. I'm looking for a fast and secure way to add and remove classes from an html element without jQuery. It also should be working in early IE (IE8 and up).
Change An Element S Class With Javascript Add An Element S
Javascript Tutorial 43 Toggle Method In Jquery Geeksread
C Java Php Programming Source Code Javascript Add Active
Add Remove And Toggle Css Class In Javascript Without Jquery
Adding Functions To A Class Javascript Code Example
Once Upon A Time In Javascript Classes And Inheritance
Jquery Vs Javascript What S The Difference Learn To
Super Simple Way To Add An Extra Css Class To Content Area
Jquery Addclass Not Working On Elements In An Array Stack
Add Remove Active Class On Click Html Css And Javascript
How To Add Class Attributes To Different Elements Using Jquery
How To Get Value On Class And Put Into Array Javascript Dom
How To Create A Simple Javascript Filter Medium
Javascript Add Custom Active Class Inside Loop Stack Overflow
Javascript Class Find Out How Classes Works Dataflair
Best Way To Add And Remove Css Class Using Javascript
Add Class And Remove Class Using Javascript For Entire Table
Add A Learning Wrapper Cms Support
How To Open A Link In A New Window Using Javascript
How To Add A Css Class To Divi On Scroll With Jquery
Only Javascript Please For Every Front End Developer By
How To Add Lt Li Gt Class To Active And Leave It After Hover
Javascript Class Find Out How Classes Works Dataflair
Add Class And Remove Class In Javascript Code Example
Javascript Add Remove Class Set Active Div With Source
Javascript Adding A Class Name To The Element Geeksforgeeks
Reactjs Dynamically Added Class To Html Elements Therichpost
How To Add Toggle Amp Remove Class In Javascript Skillsugar
How To Add Remove Css Class Dynamically In Jquery
0 Response to "31 Javascript How To Add Class"
Post a Comment