31 Private Function Javascript Class
📣 Note: class is syntax sugar and is not something fundamentally different from prototypes. A class in most cases is compiled down to ES5 constructor functions and properties and methods are translated onto the prototype! Private Properties in Classes. Now we've got our class setup, let's make the _id property private property using #: Now, the getNameWithInitial function is effectively private, as it is not exported, so client.js cannot see it. However, we still have a problem for the Person class, since this is exported. At the moment you can just walk up to manas object and do:
How To Create Private Fields And Functions In A Javascript
10/2/2020 · Private Functions In Javascript. This function can only be called by the privileged function. Below is the syntax of creating private function. var getFullName=function() { fullname=this.FirstName+" "+this.LastName; return fullname; } Privileged Function In JavaScript. The function which is declared using this keyword is called a privileged function.
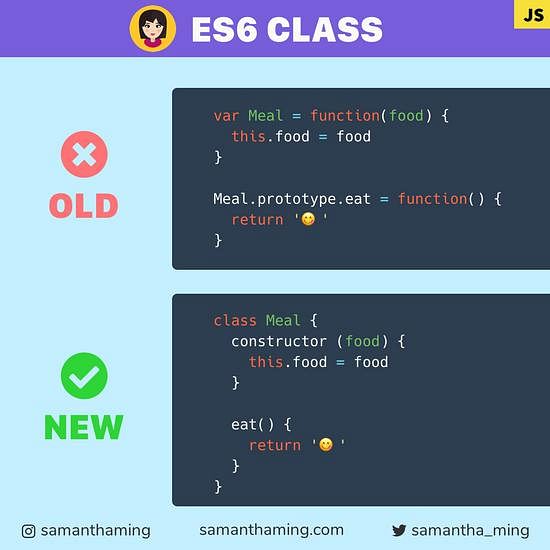
Private function javascript class. A key function accepts an object (which I call the "public instance") and returns a new object (the "private instance"). It's uniquely linked to the public instance, so you can store private properties on it. I call it a key function because it provides secure, one-way access to the private instance. A factory function is any function that returns an object. Yup, that's pretty much it. This is not to be confused with classes and constructor functions. Classes and constructor functions require the new keyword to instantiate objects while factory functions return the instantiated object itself. Public instance methods are added to the class prototype at the time of class evaluation using Object.defineProperty(). They are writable, non-enumerable, and configurable. You may make use of generator, async, and async generator functions.
You can save off a variable in the scope of the constructor to hold a reference to this.. Please Note: In your example, you left out var before privateMethod = function() making that privateMethod global. I have updated the solution here: function Class() { // store this for later. 29/11/2018 · See quickly get started with Javascript classes if you want to know more. We can potentially extend the class and have multiple validation rules. We also like to keep variables like type and internalUserFlag private. These variables can be changed by extension classes, but not by external methods. Earlier, there was no good way of enforcing this. A class member that has been declared private is only accessible from within the class that instantiated the object. A class member that has been declared protected is only accessible by the object that owns the values. If this sounds complicated, don't worry. I'll explain this in more detail.
8/1/2015 · let Name = (function() { const _privateName = new WeakMap(); const _privateHello = function(fullName) { console.log("Hello, " + fullName); } class Name { constructor(firstName, lastName) { _privateName.set(this, {firstName: firstName, lastName: lastName}); } static printName(name) { let privateName = _privateName.get(name); let _fullname = privateName.firstName + " " + … Private Fields Another aspect of the Class Fields proposal are "private fields". Sometimes when you're building a class, you want to have private values that aren't exposed to the outside world. Historically in JavaScript, because we've lacked the ability to have truly private values, we've marked them with an underscore. The proposal "Private methods and getter/setters for JavaScript classes" suggests that, To make methods, getter/setters or fields private, [we] just give them a name starting with #. Let's write a minimal Counter class as an example:
Even though ES6 introduced the class keyword that fairly well mimics classes and allows us to jump into object-oriented programming, JavaScript is missing the ability to create public, private, and protected members in a class. Private Members in JavaScript. Douglas Crockford www.crockford . JavaScript is the world's most misunderstood programming language.Some believe that it lacks the property of information hiding because objects cannot have private instance variables and methods. But this is a misunderstanding. Class fields (also referred to as class properties) aim to deliver simpler constructors with private and static members. The proposal is currently a TC39 stage 3: candidate and is likely to be...
Clients of the class need not be concerned with how the class accomplishes its tasks. For this reason, the private variables and private methods of a class (i.e., the class's implementation details) are not directly accessible to the class's clients. — Java, How to program. Let's say we have a class like this in Java: In JavaScript, Private Simply Means Inaccessible to the Current Scope. Private variables and functions in JavaScript aren't just used to modify or report the state of an instance. They do much more. They could be a helper function, a constructor function; even an entire class or module. In other words, "private" in JavaScript doesn't ... 24/6/2019 · With Closure. JavaScript’s closure comes naturally a “scope” where your variable lives. If we define a class member in a right place, we can have private-like member. There are three ways to do this. 1. Constructors. function ConstructorCar (initValue) {. this.publicMember = initValue; let _privateMember = initValue; //private member.
While using JavaScript, you might be dearly missing our friend "private" from statically-typed languages like C#, C++, and Java. Using a little finesse, we can actually achieve true encapsulation using local variables in vanilla JavaScript (ES6) classes. 23/6/2020 · It is an encapsulation of data (fields) and behavior (functions). With a new proposal currently in stage-3, a class can have private members, which are not accessible outside the scope of the class. Private Class Fields. We can create private fields, getters & setters and functions in JavaScript by prefixing it with #. In its current state, there is no "direct" way to create a private variable in JavaScript.
Currently the proposal focuses on private class properties and not private functions or private members of object literals, these may come later. Update Chrome Canary has just shipped with support for private fields behind the " experimental Javascript " flag. An important difference between function declarations and class declarations is that function declarations are hoisted and class declarations are not. ... With the JavaScript field declaration syntax, the above example can be written as: class Rectangle {height = 0; ... see private class features. In JavaScript, the function is a first-class object. That affords us numerous ways in which to use them that other languages simply cannot. For example we can pass functions as arguments to other functions and/or return them.
10/9/2008 · //Private function function setFName(pfname) { fName = pfname; alert('setFName called'); } //Privileged function this.setLName = function (plName, pfname) { lName = plName; //Has access to private variables setFName(pfname); //Has access to private function alert('setLName called ' + this.id); //Has access to member variables } //Another privileged member has access to both member … JavaScript's closures provide an excellent way to make variables and functions private, keeping them out of the global scope. This is particularly important in the browser because all scripts share the same scope, and it's quite easy to inadvertently pick a variable or function name used by another library. Private instance fields are declared with # names (pronounced " hash names "), which are identifiers prefixed with #. The # is a part of the name itself. Private fields are accessible on the class constructor from inside the class declaration itself. They are used for declaration of field names as well as for accessing a field's value.
Private and protected properties and methods. One of the most important principles of object oriented programming - delimiting internal interface from the external one. That is "a must" practice in developing anything more complex than a "hello world" app. To understand this, let's break away from development and turn our eyes into ... Privates In ES2015 Javascript Classes. ES2015 (aka ES6), Javascript · September 3, 2015. One feature missing in ES2015 (formerly ES6) classes is the concept of a private variable or function. However, thanks to ES2015 modules we can actually easily define private properties and functions that can only be accessed within our class. 21/9/2020 · Declaring private class field is simple. All you have to do is to prefix the name of the class field with #. This will tell JavaScript that you want this class field to be private. When you want to access that private class field, remember that you have to include the #.
How To Create Private Fields And Functions In A Javascript Class
Why Can I Access Typescript Private Members When I Shouldn T
Reflection Exception Issue 115 Knuckleswtf Scribe Github
Private And Protected Properties And Methods
10 Typescript Pro Tips Patterns With Or Without React
What Is A Private Method In Java Quora
Node Js Private Function Static Class Code Example
Classes In Javascript Samanthaming Com
Ruby Private Amp Protected Methods Understanding Method Visibility
Making Sense Of Es6 Class Confusion Toptal
How To Use C Classes In Arduino Ide Without Creating A
The Complete Guide To Javascript Classes
Unit Testing Private Methods In Javascript Web Design Ledger
Local Class In Abap Java And Javascript Sap Blogs
Understand Javascript Classes With Practical Examples
Details Of The Object Model Javascript Mdn
How To Work With Classes In Typescript Packt Hub
Is There Any Difference Between Weakmap And Private Member Of
Function Bind Scopes Closures In Javascript Codeburst
Javascript Class How To Define And Use Class In Javascript
The Meaning Of Underscores In Python Dbader Org
Python Inner Functions What Are They Good For Real Python
Var Functionname Function Vs Function Functionname
5 Tips To Organize Your Javascript Code Without A Framework
Typescript Access Modifiers Public Private Protected
How To Create Private Fields And Functions In A Javascript
What Is The Difference Between Public Private And Protected
0 Response to "31 Private Function Javascript Class"
Post a Comment