34 How To Check File Type In Javascript
With javascript, we have control over the type of file as well as the size and other metadata the file comes with. The whole idea behind this procedure revolves around the file object that is created when we upload a file. This file object contains information about the file such as its name, size, date modified or created etc. JavaScript type checking is not as strict as other programming languages. Use the typeof operator for detecting types. There are two variants of the typeof operator syntax: typeof and typeof (expression). The result of a typeof operator may be misleading at times.
How To Check If A File Exists In Node Js
What I want to do is to check the actual file type": - Want to check whether the type attribute really returns anything other than checking the extension internally. Since, while I was attempting to answer the OP, i found that when i renamed a text file to a.png and uploaded it, the type attribute check for image was a success even though the ...
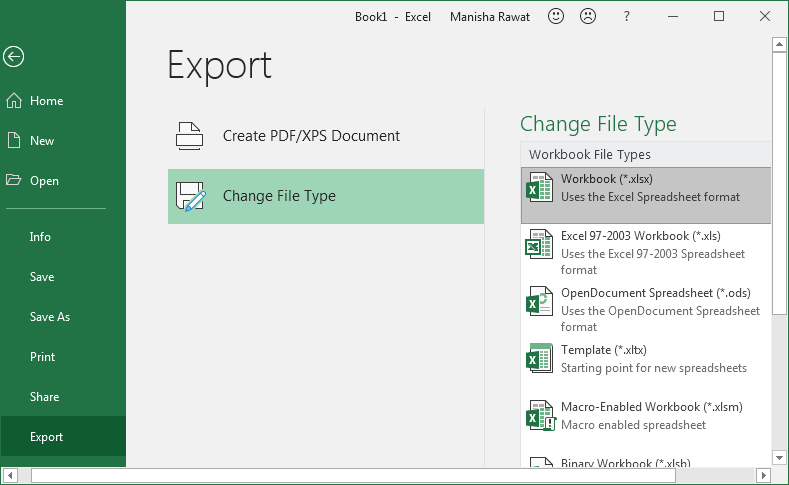
How to check file type in javascript. 1/11/2011 · This is an example for one way to check the type of the file by using the 'match' method (of strings): function getFileType(file) { if(file.type.match('image.*')) return 'image'; if(file.type.match('video.*')) return 'video'; if(file.type.match('audio.*')) return 'audio'; // etc... return 'other'; } Combining the 2 JavaScript methods will also return the file extension. The substr () method will extract or fetch certain values from a string. The general syntax is: string. substr (start, length). The first parameter start, will be a numeric value and second parameter â€Å“length†too accepts a numeric value. Watch the change Event of a File Input. To check the file MIME type with JavaScript before we upload the file, we can listen to the change event of a file input. For instance, we can write the following HTML: <input type="file" multiple> And the following JavaScript code to watch the files selected:
The full filename is first obtained by selecting the file input and getting its value property. This returns the filename as a string. By the help of split () method, we will split the filename into 2 parts. The first part will be the filename and the second part will be the extension of the file. 30/6/2017 · Using JavaScript you can easily get the file info and validate in client-side. The following single line of javaScript code, help you to get the file name, size, type, and modified date. Get File Name in JavaScript: document. getElementById ('file').files[0]. name. Get File Size in JavaScript: document. getElementById … This validates the control before post back to Server side and shows the warning message to end-user using JavaScript. Steps To Build This Requirement. Step 1. First, create an MVC Empty Template project named as "FileUploadValidation". Step 2. Then, create a Model class file named "FileUpload1.cs". Code Ref. using System;
Definition and Usage. The <input type="file"> defines a file-select field and a "Browse" button for file uploads.. To define a file-select field that allows multiple files to be selected, add the multiple attribute. Tip: Always add the <label> tag for best accessibility practices! Hi guys, Here i'm giving you some JavaScript code stuffs for validating upload file's size and extension. First, let us see our simple html form. In this form, there's a file component with name file, a submit button with value "Upload" and a div element with id "valid_msg". Please note the following points: 1) Form's… The fi.files property has a list of files in the form of an array and therefore I am checking the length (total selected files), to ensure if the user has selected any file. Once confirmed, now you can loop through each file and get the name and size of the file. Browser Support:
here is my script. What I am trying to do is to trigger it using onchange event. But it seems does not work very well. I have tried edit here and there but still facing problems. As for my latest e... If the file is less than 2MB: On successful upload: Approach-2: In the below example, we will learn how to do the same using jQuery. Listen for the change event on the input. Get the size of the file by this.files[0].size. You can round off the obtained value as well by toFixed() method. Check if the size follows your desired criteria. Example-2: In my markup section, I have an input box of type file. Since I wish to check multiple files at a time, I'll add the multiple attribute to the input type. I'll check the details of the file(s) as soon as I select it. Therefore, I am using the onchange event to call a function to check the file details.
Today, We want to share with you file upload size limit validation in javascript.In this post we will show you file upload validation in javascript, hear for Validation of file size while uploading using JavaScript / jQuery we will give you demo and example for implement.In this post, we will learn about input type file size limit javascript with an example. In a .js file, the compiler infers properties from property assignments inside the class body. The type of a property is the type given in the constructor, unless it's not defined there, or the type in the constructor is undefined or null. In that case, the type is the union of the types of all the right-hand values in these assignments. The first example is in JavaScript, followed by an example using jQuery. Each example has demos. Also Read: Check Image Width, Height and its Type before Uploading using JavaScript. 1) Get File Size using JavaScript
When dealing with files in JavaScript, you may want to check whether or not a file is an image. This tutorial will show how to JavaScript check if file is image with pure JavaScript function and JQuery. 16/2/2017 · If you want to validate other file types, change the regex with allowed extensions. HTML Code. On file select, the fileValidation() function will be executed. If the allowed file types are selected, image preview will be shown in imagePreview div. <!-- File input field --> < input type = "file" id = "file" onchange = "return fileValidation()" /> <!-- Now here in this tutorial, I'll explain how to check or validate file extensions during/before file upload from client-side using javascript and from server-side using custom validator. Like say for example, if you want to allow users to upload only images , you can allow users to upload only files with .jpg, .jpeg, .png, .gif extensions.
Using JavaScript, you can easily check the selected file extension with allowed file extensions and can restrict the user to upload only the allowed file types. For this we will use fileValidation () function. We will create fileValidation () function that contains the complete file type validation code. In this article, i will exmplain how to check file size validation using Javascript. we will check file size validation using Javascript. I can check file size validation using Javascript.Now, we get the file size on the image change event and after <!DOCTYPE html > < html > < head > < title > Title of the Document </ title > </ head > < body > < h2 > Select a file and click on the button to check the file extension. </ h2 > < div > < input type = "file" id = "chooseFile" /> < input type = "button" value = "Check Extension" onclick = "checkFileExtension();" /> </ div > < h3 > The file extension is a: < p class = "output" > </ p > </ h3 > < script > function checkFileExtension { fileName = …
This article shows how to validate a file extension in ASP.NET using JavaScript. In this article, we create a JavaScript function to ensure that only the specified extension is valid. All you need to do is implement and hook it up to your code. First of all, you start Visual Studio .NET and make a new ASP.NET web site using Visual Studio 2010. Because .mjs is a non-standard file extension, some operating systems might not recognize it, or try to replace it with something else. For example, we found that macOS was silently adding on .js to the end of .mjs files and then automatically hiding the file extension. So all of our files were actually coming out as x.mjs.js. Given an HTML document containing input element and the task is to check whether an input element is empty or not with the help of JavaScript. Approach 1: Use element.files.length property to check file is selected or not. If element.files.length property returns 0 then the file is not selected otherwise file is selected.
Get file extension in Javascript: In a web application, we use form tag to save user input. Primary form tag contains textbox, textarea and input file.i.e (file upload ), etc.While data entering user can upload any file format, so we need to validate it on the client side as well as serverside. The script separate the name and the extension of the file, adds the name in a text field and checks the extension. If the file extension is found in the allowed list, enables the 'Submit' button, else, it deletes the value in the 'file' field, disables the Submit button, and displays an alert message.
Default File Format On Upload Jquery Or Javascript Stack
3 Ways To Fix Excel Cannot Open The File Filename Xlsx
Javascript Programming With Visual Studio Code
Including External Javascript Files In Your Code Dummies
Why Are Some Javascript Files Missing In The Inspector
Get The Name Size And Number Of Files From Multiple File
Find Files With Javascript Codeproject
Filereader Javascript Example Code Example
Ts File Extension What Is A Ts File And How Do I Open It
What Is Method Of Targeting Html Input That Clone Using
Interesting Test Cases Of File Uploading Vulnerabilities By
Drag And Drop File Uploading Css Tricks
Python Read Amp Write To Files Learn How To Open Load Read
How To Determine Free Space And File Size For Sql Server
Download Javascript Data As Files On The Client Side
3 Ways To Fix Excel Cannot Open The File Filename Xlsx
Learn How To Get Current Date Amp Time In Javascript
File Browse Validation Springerlink
Javascript Programming With Visual Studio Code
How To Customize Your Default Apps In Windows And Macos Pcmag
The Art Of Simplicity Type Check Your Javascript Files
Javascript File Type Is Empty How To Upload Files To A
Detect Supported Video Formats With Javascript
View Page Resources Chrome Developers
20 Best Css Amp Javascript File Upload Examples Bashooka
How To Check File Mime Type With Javascript Before Upload
How To Change File Associations In Windows 10 Digital Trends
How To Check If A Variable Is An Array In Javascript
How To Remove Commas From Array In Javascript
Javascript Reference Error For Objects Declared In Another
Use Sharepoint Workflows To Inject Javascript Into Pdfs And
1 Writing Your First Javascript Program Javascript
0 Response to "34 How To Check File Type In Javascript"
Post a Comment