21 How To Define Regex In Javascript
In JavaScript, a Regular Expression (RegEx) is an object that describes a sequence of characters used for defining a search pattern. For example, /^a...s$/ The above code defines a RegEx pattern. The pattern is: any five letter string starting with a and ending with s. A pattern defined using RegEx can be used to … You want to build the regular expression dynamically and for this the proper solution is to use the new RegExp(string) constructor. In order for the constructor to treat special characters literally, you must escape them.There is a built-in function in jQuery UI autocomplete widget called $.ui.autocomplete.escapeRegex: [...] you can make use of the built-in $.ui.autocomplete.escapeRegex function.
The Regex Table Variable In Google Tag Manager Simo Ahava S
16/5/2019 · A Regular Expression is, as the name says, an expression (or pattern) defined by a sequence of characters. It helps you to find a match in a string and can be used in different JavaScript functions (but also with other programming languages). The syntax of a RegEx is the following: var regex = /write your RegEx …
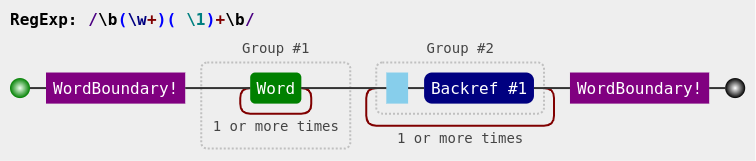
How to define regex in javascript. As we may recall, regular strings have their own special characters, such as \n, and a backslash is used for escaping. String quotes "consume" backslashes and interpret them on their own, for instance: …And when there's no special meaning: like \d or \z, then the backslash is simply removed. So new RegExp gets a string without backslashes. For representing Regular Expressions, RegExp objects are used in JavaScript. Such objects can be created using the RegExp() constructor or using the literal syntax preferably. We will take an example to understand how we can use the literals to define regular expression in JavaScript . Jan 05, 2018 - This site is best viewed in a modern browser with JavaScript enabled. Something went wrong while trying to load the full version of this site. Try hard-refreshing this page to fix the error. what does a character followed by .* mean in regex (JS)
An explanation of your regex will be automatically generated as you type. ... Detailed match information will be displayed here automatically. ... Please wait while the app is loading... Please enable JavaScript to use this web application. RegExp Object. A regular expression is an object that describes a pattern of characters. Regular expressions are used to perform pattern-matching and "search-and-replace" functions on text. Syntax / Use of Regular Expression in JavaScript Use of Regular Expression in Java (Java Regex) In Java language, Regex or Regular Expression is an application programming interface which is used for manipulating, searching, and editing a string. You can use the regular expression in java by importing the java.util.regex API package in your code.
The JavaScript RegExp class represents regular expressions, and both String and RegExp define methods that use regular expressions to perform powerful pattern-matching and search-and-replace functions on text. 2/12/2019 · The right way of doing it is by using a regular expression constructor new RegExp (). const str = "Hello yes what are you yes doing yes" const removeStr = "yes" //variable const regex = new RegExp(removeStr,'g'); // correct way const newstr = str.replace(regex,''); // it works. Regular expressions define a set of instructions. In our example: "first find the uppercase letter M, then find the lowercase i,…etc" Regular expressions have an input (the text that you are trying to search or replace) and can output the subset that you are trying to match;
In JavaScript, Regular Expressions also known as regex are patterns used to match, replace, compare character combinations in strings. When we search for some specific data in a text, we can use a regular expression to be used as a search pattern to describe what you are searching for. DOTALL is a flag in most recent regex libraries that makes the . metacharacter match anything INCLUDING line breaks. JavaScript by default does not support this since the . metacharacter matches anything BUT line breaks. To emulate this behavior, simply replaces all . metacharacters by [\S\s]. ... Sep 21, 2017 - Feel free to test JavaScript’s RegExp support right here in your browser. Obviously, JavaScript (or Microsoft’s variant JScript) will need to be enabled in your browser for this to work. Since this tester is implemented in JavaScript, it will reflect the features and limitations of your ...
And, one of the great things about Javascript regular expressions (as opposed to reg ex in other languages) is that you can pass the individual groups matches to a function call: strValue.replace( new RegExp( "([0-9]+)", "gi" ), // Pass a function as the "replace" argument for // the regular expression. Jul 02, 2018 - Not the answer you're looking for? Browse other questions tagged javascript regex or ask your own question. ... The full data set for the 2021 Developer Survey now available! ... Has any high official (politician) won any medal in an important competition before the Tokyo 2020 Olympic Games? Regular Expressions are a part of JavaScript just like they are a part of any other programming language. To initiate a RegEx you can use the literal function (you need the two / around your regEx) or the constructor function. Var regex = /measures?S?cho+l/g // literal Var regex = new RegExp ('measures?S?cho+l')// constructor function
The question's title is misleading and based on a fundamental misconception about awk.. The naïve answer is that a space can simply be represented as itself (a literal) in regular expressions in awk. More generally, you can use [[:space:]] to match a space, a tab or a newline (GNU Awk also supports \s), and [[:blank:]] to match a space or a tab.. However, the crux of the problem is that Awk ... We want to make this open-source project available for people all around the world · Help to translate the content of this tutorial to your language Their syntax is cryptic, and the programming interface JavaScript provides for them is clumsy. But they are a powerful tool for inspecting and processing strings. Properly understanding regular expressions will make you a more effective programmer. ... A regular expression is a type of object. It can be either constructed with the RegExp ...
Feb 25, 2021 - We can use them to check a string of characters, for example to look for an e-mail address — by matching the pattern which is defined by our regular expression. ... In JavaScript, we can create a Regular Expression in two ways: Either by using the RegExp constructor, or by using forward slashes ... RegExp The RegExp object is used for matching text with a pattern. For an introduction to regular expressions, read the Regular Expressions chapter in the JavaScript Guide. In the example above, / is the delimiter, w3schools is the pattern that is being searched for, and i is a modifier that makes the search case-insensitive. The delimiter can be any character that is not a letter, number, backslash or space. The most common delimiter is the forward slash (/), but when your pattern contains forward slashes it is convenient to choose other delimiters such as # or ~.
Defining Regular Expressions. In JavaScript, regular expressions are represented by RegExp object, which is a native JavaScript object like String, Array, and so on. There are two ways of creating a new RegExp object — one is using the literal syntax, and the other is using the RegExp () constructor. Regular Expression Tester with highlighting for Javascript and PCRE. Quickly test and debug your regex. Regex Expressions are a sequence of characters which describe a search pattern. It is generally used for 'find', 'find and replace' as well as 'input validation'. Most languages either contain regex expressions or contain the ability to create regex expressions such as Perl, Javascript et cetera.
Test your regex by visualizing it with a live editor. JavaScript, Python, and PCRE. A JavaScript RegEx is a sequence of characters that forms a search pattern. You can define what needs to be searched in a text with the help of regular expressions. These expressions can be of any number of characters, be it alphabets, digits or special characters. Dec 07, 2010 - Stack Overflow | The World’s Largest Online Community for Developers
Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The .replace method is used on strings in JavaScript to replace parts of In JavaScript, a regular expression is simply a type of object that is used to match character combinations in strings. Make your first Regular Expression. There are two ways to construct a regular expression, by using a regular expression literal, or by using the regular expression constructor. Below, you'll see an example of each of the ... HTML Javascript Programming Scripts A regular expression is an object that describes a pattern of characters. The JavaScript RegExp class represents regular expressions, and both String and RegExp define methods that use regular expressions to perform powerful pattern-matching and search-and-replace functions on the text.
To get started with regular expressions in Javascript you need to know the following things: You can create regular expressions using new RegExp ('regular expression as string','flags as string') or the literal notation with surrounding forward slashes: /regular expression/flags. You can check if there is a match by calling test (string) on the ... The g modifier is used to perform a global match (find all matches rather than stopping after the first match). Tip: To perform a global, case-insensitive search, use this modifier together with the "i" modifier. Tip: Use the global property to specify whether or not the g modifier is set. In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String
In regex, the +would translate to [^/]*, and the #would translate to.*. The next step is to escape special characters in the input string. This regexhas that purpose. Since the +is a special character, we will have to unescape manually. Edit the Expression & Text to see matches. Roll over matches or the expression for details. PCRE & JavaScript flavors of RegEx are supported. Validate your expression with Tests mode. The side bar includes a Cheatsheet, full Reference, and Help. You can also Save & Share with the Community, ... Jul 07, 2021 - Regular expressions allow you to ... so if they match the pattern defined by that regular expression and produce actionable information. ... There are two ways to create a regular expression in Javascript. It can be either created with RegExp constructor, or by using forward ...
But if you need a regex (perhaps an HTML5 validation regex), then it's simply a matter of saying 1. "ignore whitespace at the beginning, 2. require 1-500 characters in the middle, and 3. ignore whitespace at the end." (with anchors to ensure we're matching the full string): Test your Javascript and PCRE regular expressions online. Java Regex. The Java Regex or Regular Expression is an API to define a pattern for searching or manipulating strings.. It is widely used to define the constraint on strings such as password and email validation. After learning Java regex tutorial, you will be able to test your regular expressions by the Java Regex Tester Tool.
Apr 30, 2018 - In JavaScript, a regular expression is an object, which can be defined in two ways. The first is by instantiating a new RegExp object using the constructor: In this article we'll cover various methods that work with regexps in-depth. str.match(regexp) The method str.match(regexp) finds matches for regexp in the string str.. It has 3 modes: If the regexp doesn't have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str): A JavaScript Regular Expression is an object, which specifies a pattern of characters. Moreover, a typical example of a regular expression implementation is the find and replace functionality provided by all the text editors. Apart from it, one can use regex in the below scenarios: First, we can split the string into multiple strings.
Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec () and test () methods of RegExp, and with the match () , matchAll (), replace () , replaceAll (), search (), and split () methods of String. How to define regex in javascript. Intro To Regex For Web Developers Dev Community Exact Match Of String In Javascript Code Example Regular Expression Wikipedia Regular Expressions In Javascript An Introduction By Ross ... Javascript Regular Expressions Cheatsheet And Examples Github Bansalnitish Regularexpressions All You Need To
Become A Regular With Regular Expressions
Regex Regular Expression In Javascript By Dhruv Jain
Javascript Regular Expressions Cheatsheet And Examples
How Javascript Works Regular Expressions Regexp By
Learn Regular Expressions With This Free Course
The Essentials Of Regular Expressions By Sgwethan Towards
Regex Matching Whole Line Starting With An Exclamation Mark
Regex Google Analytics Amp Google Tag Manager Tutorial
What Is Regex Regular Expression Pattern How To Use It In
Javascript Regular Expression How To Create Amp Write Them In
Javascript Learn Regular Expressions For Beginners By
Javascript Regular Expression How To Create Amp Write Them In
Regex Google Analytics Amp Google Tag Manager Tutorial
Basic Regex In Javascript For Beginners Dev Community
What Are Regex Javascript Regular Expressions In 5 Minutes
Everything You Need To Know About Regular Expressions By
Tools Qa What Are Regular Expressions In Javascript And Are
0 Response to "21 How To Define Regex In Javascript"
Post a Comment