28 How To Check Regular Expression In Javascript
Give it a TRY! » Note:If the return value is false the form is not submitted until a value is supplied.. JavaScript Form Validaiton: Checking for Zip Codes. A simple six digit zipcode can be checked using regular expression which matches exactly six digits : /^d{6}$/. Another way can be /^[0-9][0-9][0-9][0-9][0-9][0-9]$/, for zipcode with dash in between it can be /^\d{6}-?\d{5}$.. Example ... To check a password between 7 to 16 characters which contain only characters, numeric digit s, underscore and first character must be a letter. To validate the said format we use the regular expression ^ [A-Za-z]\w {7,15}$, where \w matches any word character (alphanumeric) including the underscore (equivalent to [A-Za-z0-9_]).
Javascript Regex Match Example How To Use Js Replace On A
Aug 04, 2019 - Join Stack Overflow to learn, share knowledge, and build your career · Find centralized, trusted content and collaborate around the technologies you use most
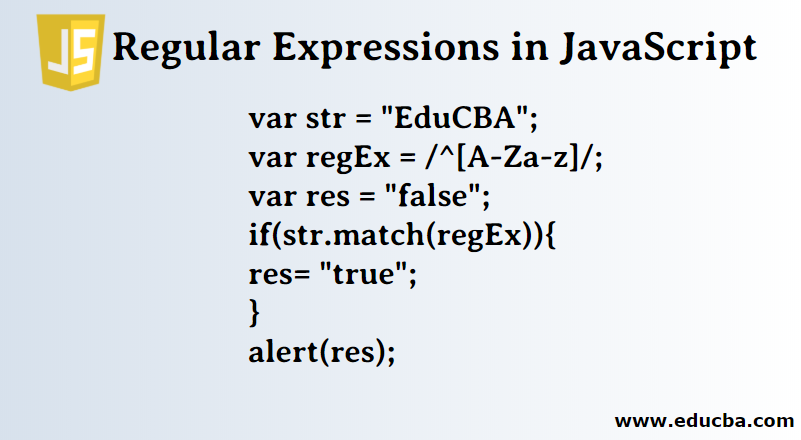
How to check regular expression in javascript. Regular Expression Tester with highlighting for Javascript and PCRE. Quickly test and debug your regex. Toggle navigation. RegEx Testing From Dan's Tools. Web Dev. ... Check if a string only contains numbers Match elements of a url Match an email address Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Sep 21, 2017 - Example of how to use the JavaScript RegExp Object. Test your regular expressions online in your web browser.
Apr 12, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. I want to use JavaScript (can be with jQuery) to do some client-side validation to check whether a string matches the regex: ^([a-z0-9]{5,})$ Ideally it would be an expression that returned true or false. I'm a JavaScript newbie, does match() do what I need? It seems to check whether part of a string matches a regex, not the whole thing. Feb 26, 2020 - JavaScript exercises, practice and solution: Write a pattern that matches e-mail addresses.
Feb 26, 2020 - Javascript function to check if a field in a html form contains all numbers or not. Regular expressions via Wikipedia: A sequence of characters that forms a search pattern, mainly for use in pattern matching with strings, or string matching. RegEx is nice because you can accomplish a whole lot with very little. In this case we are going to check various aspects of a string and see if it meets our requirements, being a strong ... Regular expression tester with syntax highlighting, explanation, cheat sheet for PHP/PCRE, Python, GO, JavaScript, Java. Features a regex quiz & library.
When a regex has the global flag set, test() will advance the lastIndex of the regex. (RegExp.prototype.exec() also advances the lastIndex property.) Further calls to test(str) will resume searching str starting from lastIndex. The lastIndex property will continue to increase each time test() returns true. Regular expressions are a right way to validate text fields such as phone numbers, names, addresses, age, date and other input information. You can use them to constrain input, apply formatting rules, and check lengths. A regular expression can easily check whether a user entered something that looks like a valid phone number. In JavaScript, you can use regular expressions with RegExp () methods: test () and exec (). There are also some string methods that allow you to pass RegEx as its parameter. They are: match (), replace (), search (), and split (). Executes a search for a match in a string and returns an array of information.
4/4/2020 · Javascript RegExp¶ Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. The test() method executes the search for a match between a regex and a specified string. Email input field validation is mandatory before submitting the HTML form. Email validation helps to check if a user submitted a valid email address or not. Using regular expression in JavaScript, you can easily validate the email address before submitting the form. We'll use the regular expression for validate an email address in JavaScript. Defining Regular Expressions. In JavaScript, regular expressions are represented by RegExp object, which is a native JavaScript object like String, Array, and so on. There are two ways of creating a new RegExp object — one is using the literal syntax, and the other is using the RegExp () constructor.
JavaScript Learn JavaScript ... A RegEx, or Regular Expression, is a sequence of characters that forms a search pattern. RegEx can be used to check if a string contains the specified search pattern. RegEx Module. Python has a built-in package called re, which can be used to work with Regular Expressions. Apr 15, 2020 - In other words, it checks whether or not the string contained in phoneNo matches our regular expression (thus returning an array, which in JavaScript will evaluate to true). If a match is detected, our function returns true to certify that the string is indeed a phone number. RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp). Supports JavaScript & PHP/PCRE RegEx. Results update in real-time as you type. Roll over a match or expression for details. Validate patterns with suites of Tests. Save & share expressions with others.
Jan 26, 2012 - To my knowledge, this matches all the variations on numbers that Java and JavaScript will ever throw at you, including "-Infinity", "1e-24" and "NaN". It also matches numbers you might type, such as "-.5". As written, reSnippet is designed to be dropped into other regular expressions, so you ... Given an email id and the task is to validate the email id is valid or not. The validation of email is done with the help of Regular Expressions. Approach 1: RegExp - It checks for the valid characters in the Email-Id (like, numbers, alphabets, few special characters.) Commonly Used Regular Expressions Regex to Check for Valid Username. Usernames are simply alphanumeric strings, sometimes with - and _ allowed, depending on the creators of the website.You can use the following regex to determine if the username should only consist of alphanumeric characters, - and _: [a-zA-Z0-9-_]{4, 24}.The numbers inside the curly braces will limit a valid username to be ...
var patt = /w3schools/i. Try it Yourself ». Example explained: /w3schools/i is a regular expression. w3schools is a pattern (to be used in a search). i is a modifier (modifies the search to be case-insensitive). For a tutorial about Regular Expressions, read our JavaScript RegExp Tutorial. In JavaScript, regular expressions are often used with the two string methods: search() and replace(). The search() method uses an expression to search for a match, and returns the position of the match. The replace() method returns a modified string where the pattern is replaced. Using a JavaScript function, we can validate a field in our ASP.NET page. In this blog, let us see how to do that. ... we can check the below conditions. Below are some sample conditions with functions and Key Code and Regular Expression respectively. Let's start with the Key Code first. Key Code .
Regular expressions allow you to check a string of characters like an e-mail address or password for patterns, to see so if they match the pattern defined by that regular expression and produce actionable information. Creating a Regular Expression. There are two ways to create a regular expression in Javascript. Regular Expressions and RegExp Object. A regular expression is an object that describes a pattern of characters. The JavaScript RegExp class represents regular expressions, and both String and RegExp define methods that use regular expressions to perform powerful pattern-matching and search-and-replace functions on text. Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp, and with the match(), matchAll(), replace(), replaceAll(), search(), and split() methods of String. This chapter describes JavaScript regular expressions.
In this article we'll cover various methods that work with regexps in-depth. str.match(regexp) The method str.match(regexp) finds matches for regexp in the string str.. It has 3 modes: If the regexp doesn't have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str): Javascript regex match. JavaScript match() method searches a string for a match versus a regular expression, and returns the matches, as the array. To use a javascript regex match, use a string match() method. Syntax string.match(regexp) Parameters. regexp: It is a required parameter, and it is a value to search for, as a regular expression. To check if a string contains at least one number using regex, you can use the \d regular expression character class in JavaScript. The \d character class is the simplest way to match numbers. // Check if string contain atleast one number ๐ฅ /\d/.test("Hello123World!"); // true. To get a more in-depth explanation of the process. Read on ๐.
Advanced regular expressions. In most browsers - those that support JavaScript 1.5 (Firefox, Chrome, Safari, Opera 7 and Internet Explorer 8 and higher) - you can use more powerful regular expressions. Very old browsers may not recognise these patterns. Given a URL, the task is to validate it. Here we are going to declare a URL valid or Invalid by matching it with the RegExp by using JavaScript. We're going to discuss a few methods. Example 1: This example validates the URL = 'https://www.geeksforgeeks ' by using Regular Expression. This free regular expression tester lets you test your regular expressions against any entry of your choice and clearly highlights all matches. It is JavaScript based and uses XRegExp library for enhanced features. Consult the regular expression documentation or the regular expression solutions to common problems section of this page for examples.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Regular expressions are both terribly awkward and extremely useful. Their syntax is cryptic, and the programming interface JavaScript provides for them is clumsy. But they are a powerful tool for inspecting and processing strings. Properly understanding regular expressions will make you a more ... Email validation. Validating email is a very important point while validating an HTML form. In this page we have discussed how to validate an email using JavaScript : An email is a string (a subset of ASCII characters) separated into two parts by @ symbol. a "personal_info" and a domain, that is personal_info@domain.
The rule of thumb is that simple regular expressions are simple to read and write, while complex regular expressions can quickly turn into a mess if you don't deeply grasp the basics. How does a Regular Expression look like. In JavaScript, a regular expression is an object, which can be defined in two ways. Once you learn the art of using regular expressions you can use it with many computer languages. Regular Expressions. The following is how regular expressions can be used [abc] - find any character in the brackets a, b or c [a-z] - find the range of characters within brackets i.e. a to z lowercase. Can also be used with numbers [0-9]
10 Regex Tester For Javascript Python Php Golang Ruby Etc
Regular Expression In Javascript
Check For Valid Email Javascript Code Example
Regular Expression Quick Javascript Interview Questions 2015
Basic Regex In Javascript For Beginners Dev Community
Asp Net Mvc 2 Model Validation Asp Alliance
Javascript Email Validation Using Javascript With Or
Regular Expression In Searching File Content Freecommander
Regviz App Reviews Features Pricing Amp Download Alternativeto
How To Learn Regex The Painless Way The Data School Australia
Understanding Regular Expressions Part 2 By Adam Shaffer
Javascript Regular Expression How To Create Amp Write Them In
Form Validation Using Js Regular Expressions Menyhart Media
Tools Qa What Are Regular Expressions In Javascript And Are
Top 6 Ways To Search For A String In Javascript And
Demystifying Regular Expressions With Javascript
User Defined Function To Validate Regular Expression In
Regular Expression With Jquery Validation Tricks Of It
Simple Form Validation In Jquery Using Regular Expression
Regular Expressions Eloquent Javascript
Regular Expressions Cheat Sheet By Davechild Download Free
Url Regex Validation Javascript Simple Example Code
Validate Form Using Javascript Regular Expressions Without
Regular Expressions In Javascript Guide To Regular Expressions
Javascript Regular Expression Tester Codeproject
0 Response to "28 How To Check Regular Expression In Javascript"
Post a Comment