23 Javascript Array Is Object
The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Description. Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types of its elements are fixed. The quickest way to convert an array of objects to a single object with all key-value pairs is by using the Object.assign () method along with spread operator syntax (...
Performance Using Array In The Object Namespace Vs Local
Differently from Array.pop, Array.shift and Array.splice, Array.filter creates a new array with all the elements that pass the condition in the callback function so your original array won't get modified as you can see from the code above. In this case, our new Array consisted of all the elements of the original that are greater than 2.
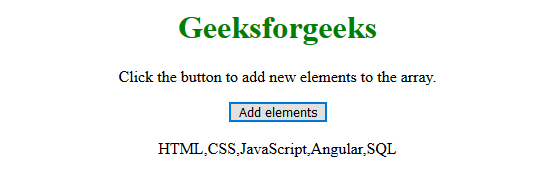
Javascript array is object. JavaScript program to find if an object is in an array or not : Finding out if an object is in an array or not is little bit tricky. indexOf doesn’t work for objects. Either you need to use one loop or you can use any other methods provided in ES6. Loop is not a good option. JavaScript provides a couple of different methods that makes it more easier. An array is a special kind of object. The square brackets used to access a property arr actually come from the object syntax. That's essentially the same as obj [key], where arr is the object, while numbers are used as keys. They extend objects providing special methods to work with ordered collections of data and also the length property. If the searched object contains also nested objects, then instead of shallowEqual() function you could use the deepEqual() function. 3. Summary. Searching for a primitive value like string or number inside of an array is simple: just use array.includes(value) method. Determining if an array contains an object by content needs more moving parts.
Step 4 — Reformatting Array Objects. .map () can be used to iterate through objects in an array and, in a similar fashion to traditional arrays, modify the content of each individual object and return a new array. This modification is done based on what is returned in the callback function. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person[0] returns John: JavaScript array is an object that represents the collection of similar types of items. JavaScript arrays are used to save multiple values in a single variable. The array is a single variable that is used to store different elements. It is often used when we want to store a list of elements and access them by a single variable.
JavaScript map with an array of objects - CodeVsColor JavaScript map with an array of objects JavaScript map method is used to call a function on each element of an array to create a different array based on the outputs of the function. It creates a new array without modifying the elements of the original array. These methods are predefined in the language itself. Let's look at the different ways of converting objects to arrays in javascript. To convert an object into an array in Javascript, you can use different types of methods. Some of the methods are Object.keys(), Object.values(),and Object.entries(). You'll probably agree that a JavaScript array is a container of any type and numerically indexed. These can be any type, such as boolean, number, string, object, or even an array called a multidimensional array. JavaScript arrays are objects. If you execute the code below, it will return an object as its type.
All examples below assume the object is already declared (as immediately above) I prefer to declare the array separately, like this, and then assign it to the object: let weekdays = ['sun','mon','tue','wed','thu','fri','sat']; myObject.weekdays = weekdays; But if you have already declared the object, it would be quicker to code: An array in JavaScript is a type of global object used to store data. Arrays can store multiple values in a single variable, which can condense and organize our code. JavaScript provides many built-in methods to work with arrays, including mutator, accessor, and iteration methods. Here will take e.g. sum of array values using for loop, sum of array object values using javaScript reduce() method, javascript reduce array of objects by key, javascript reduce array values using javascript map() method. If you should also read this javascript array posts: Convert Array to Comma Separated String javaScript
Both Object.assign () and object spread operator methods are the latest addition to JavaScript and only works in modern browsers. For more browsers support, you should simply use the for loop to iterate over the array elements, and add them to an object: First, we declare an empty object called newObject that will serve as the new object that'll hold our array items.. Then, we use a for loop to iterate over each item in the array. If the value doesn't have an undefined value, we add the item as a new property to the newObject using the array index as its key value.. If you console.log() the new object, you'll see that the same object as the ... The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
You can use the JavaScript some () method to find out if a JavaScript array contains an object. This method tests whether at least one element in the array passes the test implemented by the provided function. Here's an example that demonstrates how it works: Sort an Array of Objects in JavaScript. Summary: in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. All JavaScript objects have a toString() method. Finding Max and Min Values in an Array. There are no built-in functions for finding the highest or lowest value in a JavaScript array. You will learn how you solve this problem in the next chapter of this tutorial. Sorting Arrays.
Code language: JavaScript (javascript) Output: [ 'firstName', 'lastName' ] Code language: JSON / JSON with Comments (json) To convert property’s values of the person object to an array, you use the Object.values () method: const propertyValues = Object .values (person); console .log (propertyValues); JavaScript Array. JavaScript array is an object that represents a collection of similar type of elements. There are 3 ways to construct array in JavaScript. By array literal; By creating instance of Array directly (using new keyword) By using an Array constructor (using new keyword) 1) JavaScript array literal 14/5/2020 · Add a new object at the end - Array.push. To add an object at the last position, use Array.push. let car = { "color": "red", "type": "cabrio", "registration": new Date('2016-05-02'), "capacity": 2 } cars.push(car); Add a new object in the middle - Array.splice. To add an object in the middle, use Array.splice. This function is very handy as it can also remove items.
In JavaScript, an array is another object you use to hold values. Instead of assigning and calling properties, an array lets you call values using an index. An index describes the location of a value stored in memory. See the article "Determining with absolute accuracy whether or not a JavaScript object is an array" for more details. Given a TypedArray instance, false is always returned. Examples Using Object.fromEntries() In ES2019+, you can achieve that by using the Object.fromEntries() method in the following way: // ES2019+ const obj = Object.fromEntries(pairs); console.log(obj); // {foo: 'bar', baz: 'qux'} Using a for Loop. To create an object from an array of key/value pairs, you can simply use a for loop in the following way:
In this article, we looked at a few ways in JavaScript to determine if an object is an array. The easiest method is the Array.isArray() method that will most likely be used in production. However, we can always leverage the instanceof operator and other object properties to determine if it's an array. Working of JavaScript Arrays. In JavaScript, an array is an object. And, the indices of arrays are objects keys. Since arrays are objects, the array elements are stored by reference. Hence, when an array value is copied, any change in the copied array will also reflect in the original array. For example, 3/2/2020 · Learn what is the difference between an array and an object in Javascript. Objects are the most powerful data type of the javascript as they are used in almost everything. Functions are object, Arrays are objects, Regular Expression are objects and of course objects are objects.
The JavaScript Array Object The JavaScript Array object is a global object that is used in the construction of arrays. An array is a special type of variable that allows you to store multiple values in a single variable. To learn more about Arrays, please check out the JavaScript array chapter.
How To Convert An Array To An Object In Javascript
Why Does Typeof Array With Objects Return Object And Not
How To Remove Duplicates From An Array Of Objects Using
3 Ways To Update The Content Of An Array Of Objects With Javascript
5 Ways To Convert Array Of Objects To Object In Javascript
Javascript Array Of Objects Check Duplicates Code Example
Different Ways To Create Arrays In Javascript Time To Hack
Javascript Fundamentals Array Amp Object Methods By Timothy
Javascript Arrays Are Objects Laptrinhx
Convert An Array To Object In Javascript Code Example
How To Check If Array Includes A Value In Javascript
Rewriting Javascript Converting An Array Of Objects To An
Javascript Array A Complete Guide For Beginners Dataflair
Javascript Merge Array Of Objects By Key Es6 Reactgo
In Javascript How To Access Object Properties Inside An
Javascript Array Find How To Find Element In Javascript
Javascript Array Distinct Ever Wanted To Get Distinct
How To Add An Object To An Array In Javascript Geeksforgeeks
Traverse Array Object Using Javascript Javatpoint
Javascript Lesson 25 Difference Between Arrays And Objects
How To Cast An Array Of Objects Into A Dictionary Object In
Javascript Object Vs Array In Firebase Stack Overflow
0 Response to "23 Javascript Array Is Object"
Post a Comment