28 Javascript Send Post Request
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Aug 20, 2015 - The first argument specifies which HTTP method to use to process your request. The values you can specify are GET, PUT, POST, and DELETE. In our case, we are interested in receiving information, so the first argument we specify is going to be GET. Next, you specify the URL to send your request to.
Http Post Requests Using Microsoft Flows Cloudfronts
8/5/2018 · JavaScript has great modules and methods to make HTTP requests that can be used to send or receive data from a server side resource. In this article, we are going to look at a few popular ways to make HTTP requests in JavaScript. Ajax. Ajax is the traditional way to make an asynchronous HTTP request.
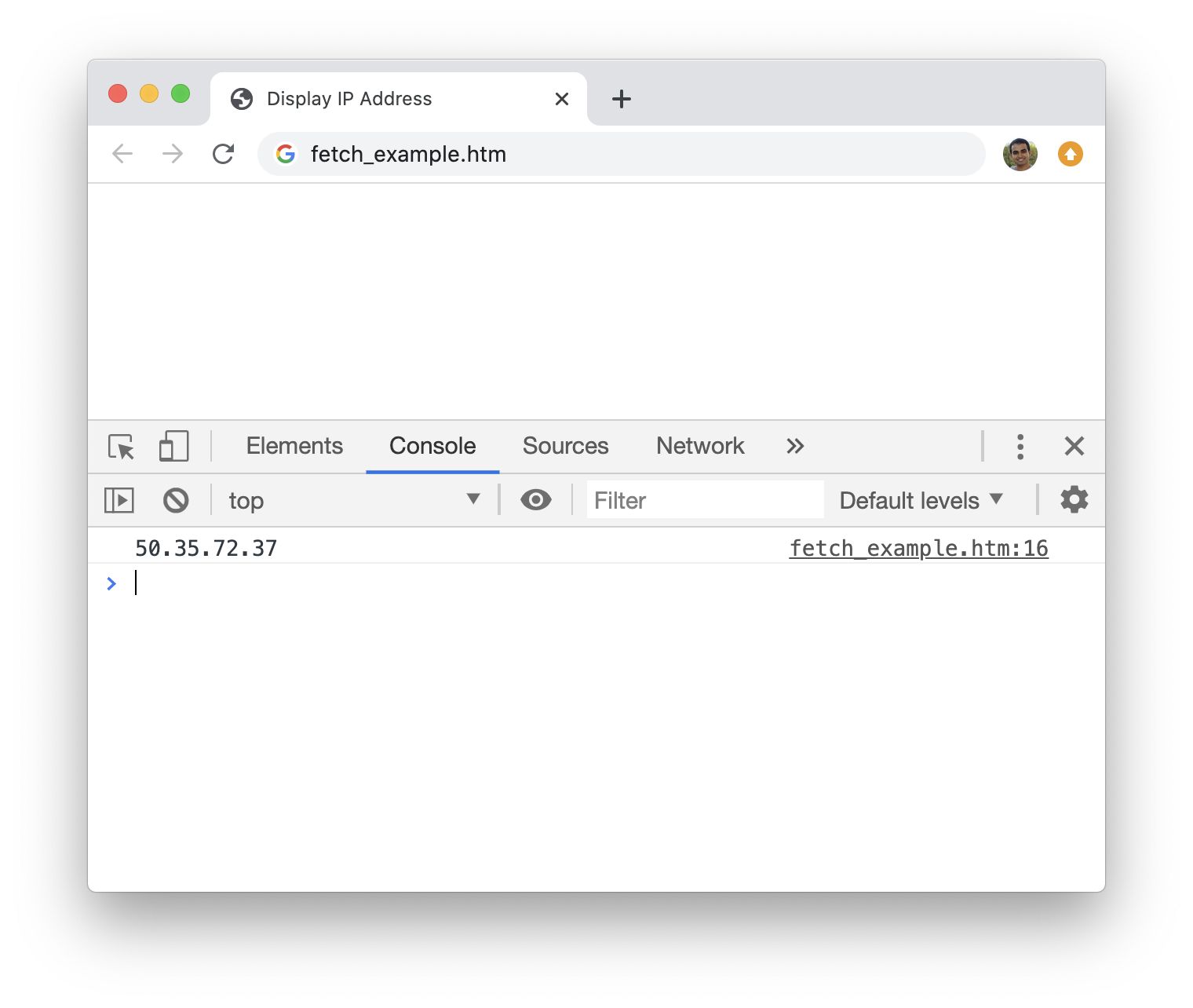
Javascript send post request. There is an easy method to wrap your data and send it to server as if you were sending an HTML form using POST . you can do that using FormData object as following: data = new FormData () data.set ('Foo',1) data.set ('Bar','boo') let request = new XMLHttpRequest (); request.open ("POST", 'some_url/', true); request.send (data) now you can ... Using JavaScript for a POST Request The easiest way to make a JavaScript POST request is to create a page that hosts a form with image data in <input> elements, and have the page POST the request... The send method is responsible for sending the request. If you set your request to be asynchronous (and why wouldn't you have?!!), the send method immediately returns and the rest of your code continues to run. That's the behavior we want. Asynchronous Stuff and Events
To send post data in JavaScript with XMLHTTPRequest, first, we have to create an XMLHTTPRequest object: var http = new XMLHttpRequest (); After that initialize it with the open () method with the request URL. We also pass the method "post" and set the asynchronous to true. Below is the code: In this tutorial, you'll learn how to make a POST request with JavaScript. Get my free 32 page eBook of JavaScript HowTos 👉https://bit.ly/2ThXPL3 Here's a li... 27/3/2009 · Though I am taking the code sample from @sundeep answer, but posting the code here for completeness. var url = "sample-url.php";var params = "lorem=ipsum&name=alpha";var xhr = new XMLHttpRequest();xhr.open("POST", url, true);//Send the proper header information along with the requestxhr.setRequestHeader("Content-type", ...
Aug 04, 2020 - I was testing an API using Insomnia, a very cool application that lets you perform HTTP requests to REST API or GraphQL API services. They have a nice button that generates code to replica an API request from the app, where you design all your request data visually. POST, FormData To make a POST request, we can use the built-in FormData object. For a POST request, you can use the "body" property to pass a JSON string as input. Do note that the request body should be a JSON string while the headers should be a JSON object.
Nov 02, 2017 - It is advised that you must not ... when sending FormData since the browser will take care of that. ... ❗️ FormData will create a multipart form request rather than an application/x-www-form-urlencoded request ... @ccpizza - thank you for clarification. since the OP did not mentioned which type of data is to be POST-ed, I think ... 5/4/2021 · [JavaScript/AJAX Code] The POST method is used to send data to the server to create/update a resource on the server. In this HTTP POST request example, the Content-Type request header indicates the data type in the body of the POST message, and the Content-Length request header indicates the size of the data in the body of the POST request. I make a POST request to the api.randomservice website (it's a random URL I just came up with) to the /dog endpoint. To this endpoint I send an object with 2 properties: { name : 'Roger' , age : 8 }
The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It also provides a global fetch() method that provides an easy, logical way to fetch resources asynchronously across the network. The first step is to attach an event handler the <form> to capture the submit event: const form = document.querySelector('#signup-form'); form.addEventListener('submit', () => { }); The next step is to create and send an actual POST request. If you are already familiar with jQuery, sending a POST request is quite similar to the $.post () method. To Send JavaScript Object Here the data is send using POST method. The default method of sending data to the server is GET. Before sending data to the server from the web applications convert the data in the form of JSON text and send it to the server. Program for sending JavaScript Object jsonsend.php
In this tutorial, you'll learn how to make a POST request with JavaScript. Get my free 32 page eBook of JavaScript HowTos 👉https://bit.ly/2ThXPL3 Here's a ... How to submit a form in a POST request using JavaScript. April 16, 2013 Comments. I was working on a Django project a couple of days ago where I needed to use JavaScript to submit a form in a POST request. The response of this request is a PDF file generated with ReportLab. I wanted the browser to prompt the user to download the file or open it ... 19/7/2021 · JavaScript POST request using the XMLHttpRequest object The XMLHttpRequest is a raw browser object that is used to communicate with the server in pure JavaScript. You can send data to the server or receive data from the server using the XMLHttpRequest object without reloading the entire web page. The XMLHttpRequest is mainly used in AJAX programming.
Synchronous XMLHttpRequest (async ... the JavaScript will stop executing until the server response is ready. If the server is busy or slow, the application will hang or stop. ... GET is simpler and faster than POST, and can be used in most cases. ... A cached file is not an option (update a file or database on the server). Sending a large amount ... However, always use POST requests when: A cached file is not an option (update a file or database on the server). Sending a large amount of data to the server (POST has no size limitations). Sending user input (which can contain unknown characters), POST is more robust and secure than GET. If a request with jQuery.post () returns an error code, it will fail silently unless the script has also called the global.ajaxError () method. Alternatively, as of jQuery 1.5, the.error () method of the jqXHR object returned by jQuery.post () is also available for error handling.
May 07, 2020 - JavaScript has great modules and ... used to send or receive data from a server side resource. In this article, we are going to look at a few popular ways to make HTTP requests in JavaScript. ... Ajax is the traditional way to make an asynchronous HTTP request. Data can be sent using the HTTP POST method and ... The jQuery post () method sends asynchronous http POST request to the server to submit the data to the server and get the response. url: request url from which you want to submit & retrieve the data. data: json data to be sent to the server with request as a form data. callback: function to be executed when request succeeds. The XMLHttpRequest method send () sends the request to the server. If the request is asynchronous (which is the default), this method returns as soon as the request is sent and the result is delivered using events. If the request is synchronous, this method doesn't return until the response has arrived.
Mar 20, 2021 - In this tutorial, I show how you can send GET and POST AJAX requests with Javascript and handle the request with PHP. Send the request by calling send () method. insertNewEmployee () - This function calls on Submit button click. Read values from the textboxes and assign them in variables. If variables are not empty then create a data JSON object. How JavaScript post request like a form submit - we can use the createElement function, which is necessary due to IE's brokenness with the name attribute on elements created normally with document.createElement. ... post request example javascript post form ajax javascript post data to url javascript submit form onclick javascript send post ...
Sending a HTTP POST request with parameters. To send a HTTP POST request from JavaScript, we will need to use the XMLHttpRequest object. I already made a HTTP client class to make things easier, so we will just need to instantiate a new HTTP client object from it to send an AJAX request. Make an HTTP POST request using Node.js There are many ways to perform an HTTP POST request in Node.js, depending on the abstraction level you want to use. The simplest way to perform an HTTP request using Node.js is to use the Axios library : JavaScript Fetch API provides a simple interface for fetching resources. It is the newest standard for handling network requests in the browser. The biggest advantage of Fetch over XMLHttpRequest(XHR) is that the former uses promises that make working with requests and responses far easier.
HTML forms can send an HTTP request declaratively. But forms can also prepare an HTTP request to send via JavaScript, for example via XMLHttpRequest. This article explores such approaches. It's a common task for JavaScript developers to send GET and POST requests to retrieve or submit data. There are libraries like Axios that help you do that with beautiful syntax. However, you can achieve the same result with a very similar syntax with Fetch API, which is supported in all modern browsers. Table of Contents hide Sending forms through JavaScript HTML forms can send an HTTP request declaratively. But forms can also prepare an HTTP request to send via JavaScript, for example via XMLHttpRequest. This article explores such approaches.
How To Make Http Request In Javascript Bytesofgigabytes
Javascript Send Http Get Post Request And Read Json Response
Here Are The Most Popular Ways To Make An Http Request In
5 Practical Scenarios For Xss Attacks Pentest Tools Com Blog
How To Post Data In Javascript Ajax Code Example
Javascript Ajax Post And Get Method Example
How To Master Http Requests With Axios
Fetch Api How To Make A Get Request And Post Request In
How Http Post Request Work In Nodejs Geeksforgeeks
Fail To Construct A Post Request Instance In Javascript
Simple Get And Post Request Using Fetch Api Method By Making
How To Submit Ajax Forms With Jquery Digitalocean
Javascript Post Request Being Sent As Get Stack Overflow
Here Are The Most Popular Ways To Make An Http Request In
Javascript Send Http Get Post Request And Read Json Response
Making Http Web Requests In Javascript
All Possible Ways Of Making An Api Call In Javascript By
Send Post Data Using Xmlhttprequest Stack Overflow
How To Send A Json Object To A Server Using Javascript
Http Post Requests Using Microsoft Flows Cloudfronts
Javascript Requests I Xhr Post Send Id 39 200
How To Send Post Request With Jquery Code Example
How Http Post Request Work In Nodejs Geeksforgeeks
Performing An Http Request In Python Datacamp
Apache Jmeter User S Manual Component Reference
0 Response to "28 Javascript Send Post Request"
Post a Comment