24 Zip Code Validation In Javascript
If you want to use regular expressions for validation, the most straightforward way is through the regular expression’s test method. const zipCodeRegex = /^\d {5}$/; zipCodeRegex.test('90210'); zipCodeRegex.test('apple'); zipCodeRegex.test('902101'); Copy. I'm attempting to have Javascript validate a user-entered zip code. If it is within the list of valid zip codes, it should take them to a new page to schedule an appointment. If the zip code isn't in the listing, it should display a message that says that it isn't covered by the service area and then keep them on the page.
Javascript A Sample Registration Form Validation W3resource
This video will tell about the validation of pIncode ,zipcode in a form.A zip code is consist of only 6 digits.
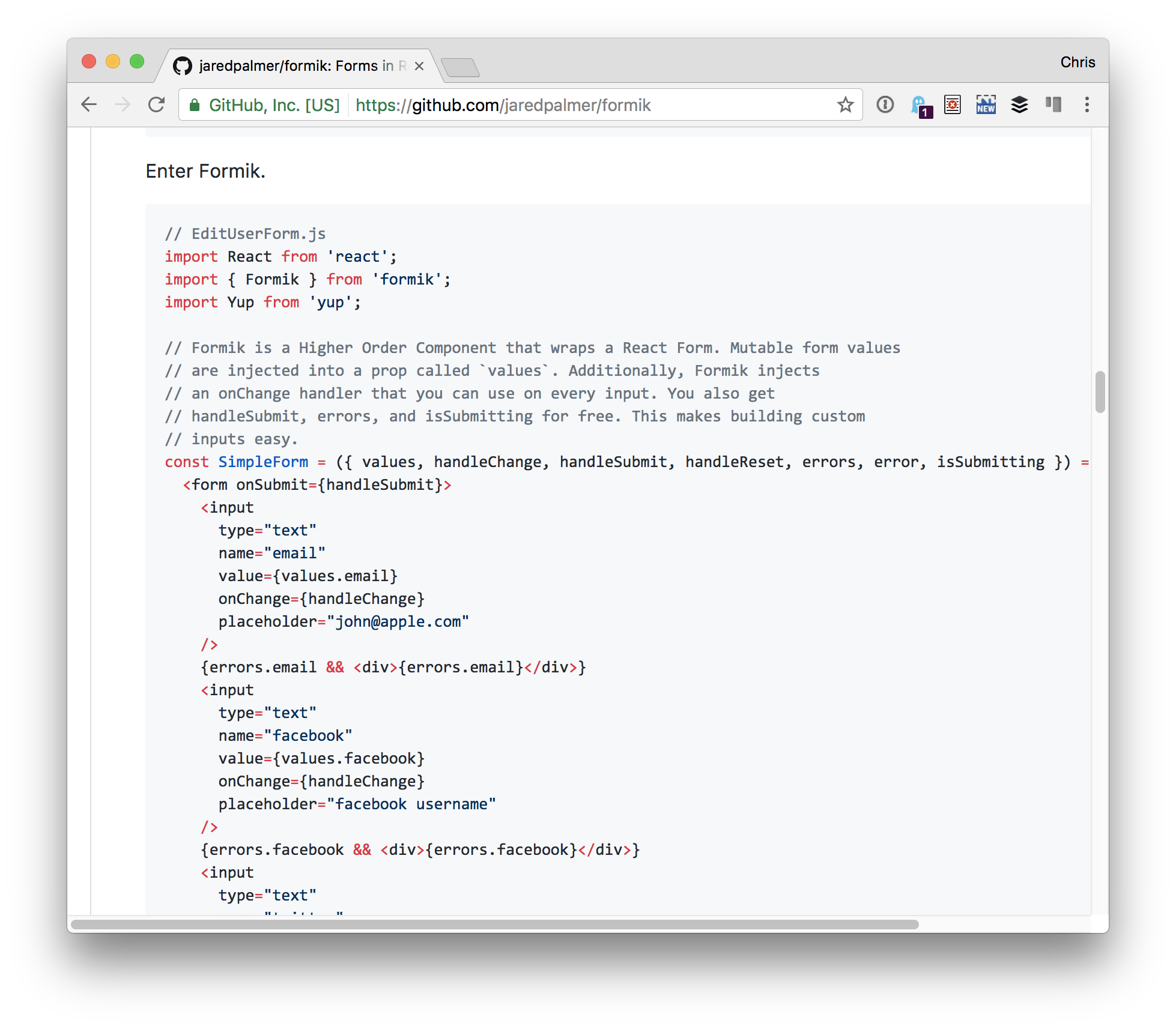
Zip code validation in javascript. When the rule is zipCode it needs to validate for BOTH a 5-digit or a 9-digit entry. These are considered valid zip codes and our users will need to be able to enter either. There is a proper RegEx method in JavaScript for handling this which I am using as a custom validation: 26/2/2020 · Write a JavaScript function to check whether a given value is US zip code or not. Sample Solution:-HTML Code: <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>JavaScript function to check whether a given value is US zip code or not</title> </head> <body> </body> </html> JavaScript Code: I think the best solution, if possible, would be to change or remove the pattern attribute based on the country a user selected. That way people in countries such as the U.S. with number-only zip codes can have their validation to prevent errors, but people in other parts of the world aren't limited.
Basic Form Validation. First let us see how to do a basic form validation. In the above form, we are calling validate() to validate data when onsubmit event is occurring. The following code shows the implementation of this validate() function. <script type = "text/javascript"> <!-- // Form validation code will come here. String or Function. An ISO-3166 country code. message. data-fv-zip-code___message. String. The error message. If you want to support custom formats of a zipCode number, you should use the Transformer plugin. The validator supports the following countries (click the sample number to validate it): Country. Learn how to use JavaScript Regular Expression Form Validation : Check for Zip Codes . EDITABLE CODE: LiveDemo: JavaScript Regex : Check Zip Codes --Tutorialspark . Note:If no input is provided an alert ... LiveDemo: JavaScript Regex : Check Zip Codes --Tutorialspark .
10+ JavaScript Form Validation Code Examples. Latest Collection of hand-picked free JavaScript Form Validation Examples with Code Snippet. 1. HTML5 Form Validation. 2. Elegant Login Form with ParticlesJS. Apr 14, 2017 - Zip code allows user to enter 10 digits and system displays as 5digits - 5digits(11 characters) which are incorrect · What I would like Zip code -maximum character needs to be 10 characters including the hyphen. Zip code validation using javascript see this link .. hepspace validation code generator http://hepspace /web/validation/zip-validation.php
Dynamics CRM 2011 Javascript ZipCode/Postal Code Lookup. By Meg Moss - May 25, 2012. Recently, I needed to write some javascript to do a zip code/postal code lookup for validation purposes and it also needed to work for international. I also added the ability to populate the city and state fields (may as well). Below is the code. JavaScript zip code validation Zip code is the postal code used by the United States Postal Service (USPS). The basic format consists of 5 digits. Later, an extended code was introduced that contains a hyphen and 4 more digits. Summary: in this tutorial, you'll learn about JavaScript form validation by building a signup form from scratch.. What is form validation. Before submitting data to the server, you should check the data in the web browser to ensure that the submitted data is in the correct format.. To provide quick feedback, you can use JavaScript to validate data. This is called client-side valida
Oct 02, 2008 - I know there are ZIP code databases ... issued, so you might want to use weak validation. I wrote a little bit about zip codes in a side project on my wiki/blog: ... There is also a new, weird type of zip code. ... I can do the javascript code, but it would be interesting to see ... So, just to make sure that user entered the email address in the correct format, it's necessary to include email validation code inside the validation codes. A general expression to check valid email address is: /^[w-.+] [email protected] [a-zA-Z0-9.]+.[a-zA-Z0-9]{2,4}$/ The expression checks the letters, numbers, and other symbol at a proper ... Jun 04, 2015 - Hi, I’m trying to validate just zip code in the surrounding area. Right now, it will validate any zip code that starts with “8”. I only wanted to validate the zip codes for 87109, 87110, 87111, 87112, 87113, 87114 and anything else will be invalid. Any help will be appreciated and thank you.
The zip code can be validated using the java.util.regex.Pattern.matches () method. This method matches the regular expression for the zip code and the given input zip code and returns true if they match and false otherwise. A program that demonstrates this is given as follows: Here is the code that does that step: document.getElementById ('myform').addEventListener ('submit', validateForm); function validateForm () { } First, we get the form DOM object using the ID attribute of the form. Then we listen to the form submit event. Next, we have to validate each of the fields in the form. sample-registration-form-validation.js is the external JavaScript file which contains the JavaScript ocde used to validate the form. js-form-validation.css is the stylesheet containing styles for the form. Notice that for validation, the JavaScript function containing the code to validate is called on the onSubmit event of the form.
JavaScript to provide form validation for both 5- and 9-digit zip codes, for U.S. zip codes only.... Provide postal code validation for javascript. Contribute to Cimpress-MCP/postal-codes-js development by creating an account on GitHub. 15/5/2016 · In the below functionI had implemented Zip code validation using JavaScript. You can implement the same logic in any language. The Control here I used to take input from user is txt_zip. In-case you copy the below code. Update this with your Control …
JavaScript Form Validation. HTML form validation can be done by JavaScript. If a form field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted: Jul 19, 2010 - Here’s how to validate US Zip code using JavaScript. We will be using a Regular Expression to do so. The code uses the indexOf function of Javascript Array to search for the zip code string in the available zip codes list. Also, note that the zipcodes saved as strings rather than numbers. This is because the zip code is an identification rather than arithmetic quantity. So 00123 may be a valid zip code.
Validate.js provides a declarative way of validating javascript objects. It is unit tested with 100% code coverage and can be considered fit for production. Zip Code Validator. This javascript checks the length of a zip or post code entered into a form and if the length is equal to 9 numeric characters will then format it with a hyphen inserted after the 4th character. Suitable for validating US postal codes only. Users may enter alpha characters or non numeric characters in the zip code such as ... Sometimes situations arise (input a phone number, zip code or credit card number) when the user should fill a single or more than one fields with numbers (0-9) in an HTML form. You can write JavaScript scripts to check the following validations. To check for all numbers in a field. An integer with an optional leading plus (+) or minus (-) sign.
10/3/2017 · Hi developers this is a simple demo for **zip code validation for us country** And this code is very usefull for you. --> <html xmlns="http://www.w3 /1999/xhtml"> <head> <title>Validate US Zip Code in JavaScript</title> <script type="text/javascript"> function IsValidZipCode(zip) { var isValid = /^[0-9]{5}(?:-[0-9]{4})?$/.test(zip); if (isValid) alert('Valid ZipCode'); else { alert('Invalid ZipCode'); } } </script> </head> <body> <form> <input id="txtZip" name="zip… Basic Form Validation in JavaScript In the past, form validation would occur on the server, after a person had already entered in all of their information and pressed the submit button. If the information was incorrect or missing, the server would have to send everything back with a message telling the person to correct the form before ... Zip Code Validation. Ask Question Asked 7 years, 2 months ago. Active 7 years, ... I want to accomplish the following 1. If the zip code is present in my database then print eligible for delivery 2. Else Not eligible for delivery. I wrote code as below: html ... Invoking JavaScript code in an iframe from the parent page. 928.
What is the ultimate postal code ... would still be missing on the validation part: Zip code 12345 may The unicode CLDR contains the postal code regex for each country. Or using JS API: https://developers.google /maps/documentation/javascript/geocoding# Locale code (Example: ... There is one valid ZIP+4 code that this regex will not match: 10022-SHOE. This is the only ZIP code that includes letters. In 2007, it was assigned specifically to the eighth floor Saks Fifth Avenue shoe store in New York, New York. At least thus far, however, the U.S. JavaScript Form Validation - Phone Numbers; Javascript password validation alphanumeric string; Javascript password validation using regular expression; Javascript validation simple example code; JavaScript zip code validation; The Most Indispensable jQuery Form Validation Reference Guide; Javascript Popups. jQuery Popups; JavaScript Popup ...
This validation case where a server-side call is made is called server side validation. What data should be validated? Form validation is needed anytime you accept data from a user. This may include: Validating the format of fields such as email address, phone number, zip code, name, password. Validating mandatory fields Um. There is verry little code, so one can not say much about your code. It does, what it should, I assume. One thing, I see is, that you are alerting a message, which has nothing to do with the validation. So if you are looking for SRP - the separation of concerns, you take the alert out and put it elsewhere. Javascript-Check Indian Zipcode. Ghanashyam Nayak. Updated date Apr 30, 2020. 35.4 k. 0. 0. This code shows you that how you can check indian Zp code at runtime, which given by user. facebook. twitter.
So, zip codes 38980 and 83900-8789 will pass validation. However, 83900- or 839008789 will not pass our validation test. If you don't want to have a zip+4 format you can use /^\d{5}$/ as the regular expression to validate simple zip code. Image credit:smcgee
Html Code For Registration Form With Validation
Bootstrap Validation Validate Form Using Boostrap Edureka
How To Use Regular Expressions To Validate User Input Pega
A Custom Textbox With Regular Expression Validation Using Mc
How To Set Up Phone Number Or Zip Code Validation Digioh
Whitelist Bypassing Client Side Javascript Validation
Complete Form Validation In Javascript With Source Code
Javascript Auto Filling One Field Same As Other Geeksforgeeks
Street Address Form Validation With Reactjs And Here Geocoder
Jquery Validation Plug In Is Coming With Jqwidgets 1 8
Checkout Usability Auto Detect City And State Inputs
Form Validation Using Javascript Formget
How To Restrict Deliveries To Some Specific Pin Codes In
Configuring Fields And Labels Chargebee Docs
How To Use Regular Expressions To Validate User Input Pega
Javascript Validation With Regular Expression Check Whether
How To Add A Custom Zipcode Validator In Checkout Page
Why Use A Third Party Form Validation Library Css Tricks
Adding Custom Validation To Form Fields Forms Unbounce
Regular Expression Zip Code Login Information Account Loginask
Checkout Usability Auto Detect City And State Inputs
Topic Notes Javascript Form Validation Courses
0 Response to "24 Zip Code Validation In Javascript"
Post a Comment