26 Javascript Array Push Pop
1 week ago - The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. The JavaScript array pop () method removes the last item in an array. In this example, you'll notice that 4, the last element in the array, is removed from the array, and only the first three items are left: var items = [ 1, 2, 3, 4 ];
The Four Common Javascript Array Methods Push Pop Shift And
In our JavaScript we have declared a dynamic array onto which we are going to perform the push and pop operations. We have created two buttons in this html form
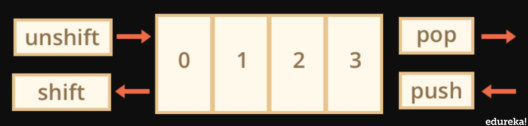
Javascript array push pop. Oct 26, 2020 - When working with arrays, it is important to understand the different types of methods and how they transform your data. push(), pop()… The pop() method in JavaScript removes an item from the end of an array, whereas the push() method adds an item to the end of an array. The returning value of the pop() method is the item that is removed from the array. The returning value of the push() method is the number of elements in the array after the new element has been added. These ... I have an array of numbers and I'm using the .push() method to add elements to it. ... If you want to remove at either end of the array, you can use array.pop() for the last one or array.shift() ... JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any ...
Javascript arrays have native support for these two methods. Javascript also has support for parallel methods that work on the beginning of the array, where the index is smallest. Unshift () and shift () are basically the same as push () and pop (), only, at the other end of the array. May 09, 2020 - In this article, we will learn about four array methods i.e. push(), pop(), shift() and unshift() which allow us to add and remove elements from beginning and end of an array. Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript ... The value associated with each key will be an array consisting of all the elements that resulted in that return value when passed into the callback.
JavaScript Array type provides the push() and pop() methods that allow you to use an array as a stack. push() method. The push() method allows you to add one or more elements to the end of the array. The push() method returns the value of the length property that specifies the number of elements in the array. If you consider an array as a stack, the push() method adds one or more element at the top of March 19, 2021 March 24, 2019 By Admin Leave a Comment on JavaScript: Multidimensional Array With Push Pop In this js array tutorial, you will learn about JavaScript multidimensional array. And also learn how to access javascript multidimensional array, How to add elements in a multidimensional array, Remove items in multidimensional array ... let items = [1, 2, 3] items.push(4); // items = [1, 2, 3, 4]
pop () Return Value. Removes the last element from array and returns that value. Returns undefined if the array is empty. Notes: This method changes the original array and its length. To remove the first element of an array, use the JavaScript Array shift () method. 1 week ago - The push() method adds one or more elements to the end of an array and returns the new length of the array. Array.prototype.pop () The pop () method removes the last element from an array and returns that element. This method changes the length of the array.
6 days ago - Pop Push Shift and Unshift Array Methods in JavaScript. We will see pop, push, shift, and unshift method one by one in Javascript Node.js with example. .push(), .pop(), .shift() และ .unshift() เพิ่มค่า ตัดค่า ได้ทั้งหน้าและหลังของ Array[…] 7/3/2014 · In javascript, Objects are passed by reference, so you are not passing a different object in each index. Here is something that will work. while ( (a=arr.pop ()) != null) { var clone = {"id": a, "name":"Matthew"}; clone.id = a; arrObj.push (clone); } console.log (arrObj); Result:
Array class in Javascript supports push and pop method to insert and pop up data from array objects. Array push and pop is same as stack data structure "Last come First Out". Example. 1. May 17, 2020 - Javascript has a number of methods related to arrays which allow programmers to perform various array... These are JavaScript methods that manipulate contents of arrays in different ways. In simple words, pop () removes the last element of an array. push () adds an element to the end of an array. shift () removes the first element. unshift () adds an element to the beginning of the array.
The JavaScript Array object provides very useful methods for basic operations: push(), pop(), shift() and unshift(). To be precise, these methods belong to the Array object's prototype property. push() - Add an item to the end of an Array The pop () method removes the last element of an array, and returns that element. Note: This method changes the length of an array. Tip: To remove the first element of an array, use the shift () method. The method pop () remove the last item from the array and return the remove item.
Implementing JavaScript Stack Using Array. In this section, we will learn about the implementation of Stack using JavaScript arrays.. What is a Stack. A data structure in which we place the elements in Last In First Out, i.e., LIFO principle.The LIFO principle means the arrangement of the element in such a manner that the element which is added recently gets removed first, and the element ... What are push () and pop () methods in JavaScript push () is used to add an element/item to the end of an array. The pop () function is used to delete the last element/item of the array. Let's try to add and remove elements from an array using push () and pop () methods to understand these methods better. Link for all dot net and sql server video tutorial playlistshttp://www.youtube /user/kudvenkat/playlistsLink for slides, code samples and text version of ...
Diterjemahkan dari The four common Javascript array methods Push, Pop, Shift and Unshift JavaScript memiliki banyak method yang memungkinkan programmer bekerja dengan array. Ada empat method yang paling sering dipakai untuk menambahkan dan menghapus elemen dari sebuah array. Keempat method tersebut adalah push(), pop(), shift(), dan unshift(). Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, and XML. Javascript has a number of methods related to arrays that allow programmers to perform various array operations. There are four methods that are particularly used for adding and removing elements to and from an array. They are: push (), pop (), shift () and unshift ().
Javascript array push() method appends the given element(s) in the last of the array and returns the length of the new array. Syntax. Its syntax is as follows −. array.push(element1, ..., elementN); Parameter Details. element1, ..., elementN: The elements to add to the end of the array. Return Value. Returns the length of the new array. Example 102. JavaScript Array Push() pop() shift() unshift() Method Part 20 || JavaScript Tutorial BanglaReference: Contents of this video:00:00 - Intro00:32 - Let... JavaScript gives us four methods to add or remove items from the beginning or end of arrays: pop(): Remove an item from the end of an array let cats = ['Bob', 'Willy', 'Mini']; cats.pop(); // ['Bob', 'Willy'] pop() returns the removed item. push(): Add items to the end of an array
3 weeks ago - Array.pop(): We use pop JavaScript to remove the last element in an array. Moreover, this function returns the removed element. At the same, it will reduce the length of the array by one. This function is the opposite of the JavaScript array push function. Aug 28, 2017 - Quick, easy ways to remember the differences among shift, unshift, push, and pop in JavaScript arrays. Tagged with javascript, beginners, webdev, tutorials. push () Return Value. Returns the new (after appending the arguments) length of the array upon which the method was called. Notes: This method changes the original array and its length. To add elements to the beginning of an array, use the JavaScript Array unshift () method.
JavaScript Array push() method. The JavaScript array push() method adds one or more elements to the end of the given array. This method changes the length of the original array. Syntax. The push() method is represented by the following syntax: JavaScript Array methods Push, Pop, Unshift and Shift javascript 1min read In this tutorial, we will learn about how to add or remove items from the beginning or end of the arrays by using four different methods in JavaScript. 15 hours ago - Removing & Clearing Items From JavaScript arrays can be confusing. Splice, substring, substr, pop, shift & filter can be used. Learn how they differ and work.
The JavaScript Array object provides four very useful methods: push (), pop (), shift () and unshift (). To be precise, these methods belong to the Array object's prototype property. We know this because every array you create shares a copy of these four methods (that is because every array you create is an instance of the Array constructor). The push () method adds new items to the end of an array. push () changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift (). Simple Javascript Nested Array Examples - Create Push Pop Loop Check By W.S. Toh / Tips & Tutorials - Javascript / May 26, 2021 May 26, 2021 Welcome to a quick tutorial on the nested array in Javascript.
Javascript Array Stack Methods Push Pop
Ds With Js Arrays Arrays Are Great For Lookups Push Pop
Stack Data Structure In Javascript Javascript Array Push Pop Tutorial Hindi
Javascript Array Push Is 945x Faster Than Array Concat
How And When To Use Push Pop Unshift Amp Shift In
Javascript Arrays And Uses Of Push Pop Unshift Shift
Javascript Array Shift Unshift Push And Pop Methods
How To Remove And Add Elements To A Javascript Array
16 Javascript Array Methods That Every Good Web Developer
Check And Fill In Vacancies Javascript Array Method
A List Of Javascript Array Methods By Mandeep Kaur Medium
Javascript Lesson 19 Push And Pop Methods In Javascript
A Quick Guide To Stacks In Javascript By Kenneth Young
Pop Push Shift And Unshift Array Methods In Javascript
Unshift Javascript Unshift Array Methods In Javascript
Javascript Array Push Pop Shift Unshift How To Remember
Javascript Array Pop Removing Items From An Array
The Four Common Javascript Array Methods Push Pop Shift And
Javascript Array Push Pop Shift Unshift Visual
Javascript How To Add New Array Element At The Start Of An
Javascript Lesson 19 Push And Pop Methods In Javascript
Advanced Javascript Module 01 Part 07 Array Functions Push Pop Shift Amp Unshift
0 Response to "26 Javascript Array Push Pop"
Post a Comment