24 How To Make Array In Javascript
Array.from() lets you create Arrays from: array-like objects (objects with a length property and indexed elements); or ; iterable objects (objects such as Map and Set).; Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map ... Apr 19, 2019 - In some browsers, a decrementing ... can't make general statements about javascript performance like that because there are so many implementations with so many different optimisations. However, in general I like your approach. ;-) ... Creates an array of numbers (positive and/or negative) progressing from start up to, but not ...
Arrays In Javascript How To Create Arrays In Javascript
How to Create a Two Dimensional Array in JavaScript. The two-dimensional array is a set of items sharing the same name. The two-dimensional array is an array of arrays, that is to say, to create an array of one-dimensional array objects. They are arranged as a matrix in the form of rows and columns.
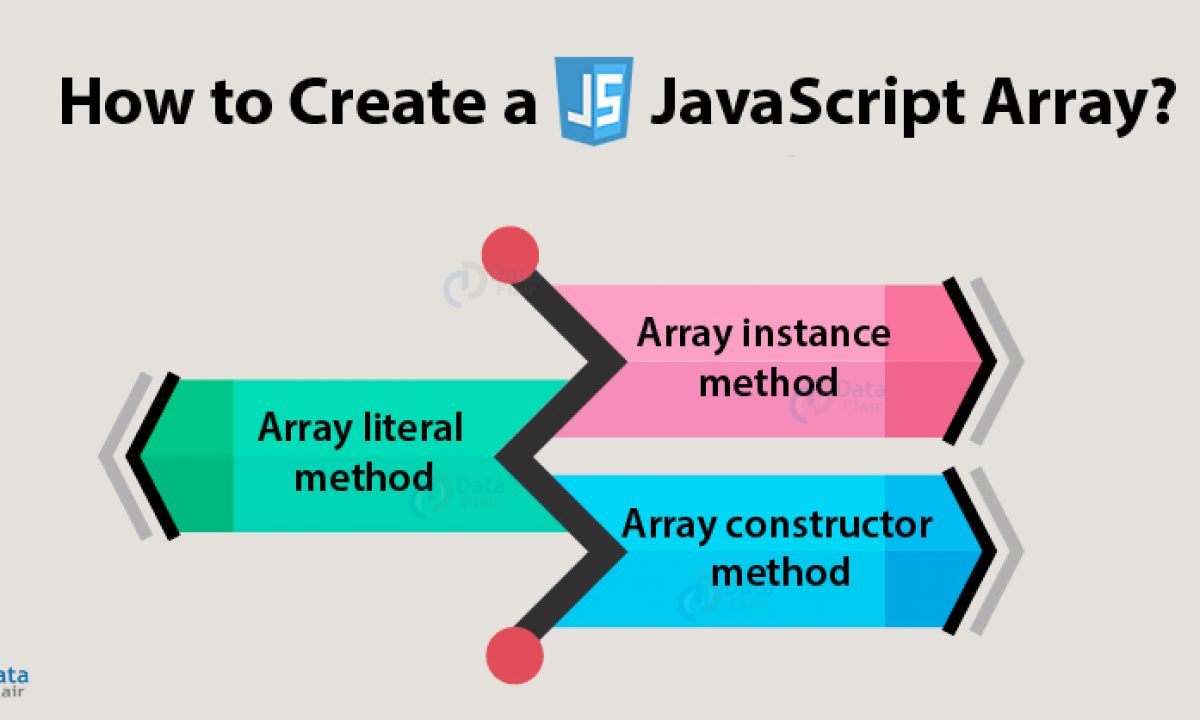
How to make array in javascript. Jul 28, 2019 - If you have been writing JavaScript functions long enough, you should already know about the arguments object — which is an array-like object available in every function to hold the list of arguments the function received. Although the arguments object looks much like an array, it does not ... JavaScript Array. JavaScript array is an object that represents a collection of similar type of elements. There are 3 ways to construct array in JavaScript. By array literal; By creating instance of Array directly (using new keyword) By using an Array constructor (using new keyword) 1) JavaScript array literal The two-dimensional array is a collection of items which share a common name and they are organized as a matrix in the form of rows and columns.The two-dimensional array is an array of arrays, so we create an array of one-dimensional array objects. The following program shows how to create an 2D array :
Aug 02, 2017 - The Array in JavaScript is a global object which contains a list of items. It is similar to any variable, in that you can use it to hold any type of data. However, it has one important difference: it can hold more than one item of data at a time. Nov 23, 2013 - Underscore.js is the most popular way of complementing JavaScript’s Spartan standard library. It comes with the function ... is not very self-explanatory and Underscore.js is as close to a standard as it gets. Therefore, it is best to create filled arrays via In Javascript, Dynamic Array can be declared in 3 ways: Start Your Free Software Development Course. Web development, programming languages, Software testing & others. 1. By using literal. var array= ["Hi", "Hello", "How"]; 2. By using the default constructor. var array= new Array ();
Dec 07, 2018 - Occasionally in JavaScript it’s necessary to populate an array with some defaults — an object, string or numbers. What’s the best approach for the job? Lately, I’ve been working with Oleksii Trekhleb… Sep 11, 2020 - An JavaScript array has the following characteristics: First, an array can hold values of different types. For example, you can have an array that stores the number and string, and boolean values. Second, the length of an array is dynamically sized and auto-growing. In other words, you don’t need to ... In this tutorial, we will learn how to create arrays; how they are indexed; how to add, modify, remove, or access items in an array; and how to loop through arrays. Creating an Array. There are two ways to create an array in JavaScript: The array literal, which uses square brackets. The array constructor, which uses the new keyword.
Arrays in javascript are just objects with some additional properties and methods which make them act like an array. So when we directly assign array to another variable it is shallowly copied. So when we directly assign array to another variable it is shallowly copied. We will begin by looking at different ways to create an array in JavaScript. The first two of these examples create arrays where only the length is set and there are no numbered entries at all ... Jul 21, 2021 - In JavaScript, array is a single variable that is used to store different elements. It is often used when we want to store list of elements and access them by a single variable. Unlike most languages where array is a reference to the multiple variable, in JavaScript array is a single variable ...
The search criteria in the JavaScript filter function are passed using a callbackfn. Arrow functions can also be used to make JavaScript filter array code more readable. Syntax of JavaScript filter(): array.filter(function(value, index, arr), thisValue) Here, array refers to the original array that you are looking to filter. Parameters: Simple Javascript Nested Array Examples - Create Push Pop Loop Check By W.S. Toh / Tips & Tutorials - Javascript / May 26, 2021 May 26, 2021 Welcome to a quick tutorial on the nested array in Javascript. Arrays are the special type of objects. The typeof operator in the JavaScript returns an "object" for arrays. But, JavaScript arrays are the best described as arrays. JavaScript variables can be objects. Arrays are special kinds of objects. Because of this, you can have the variables of different types in the same Array.
In JavaScript, you can use the Array.map() method to iterate over all elements and then use the string methods to change the case of the elements. Here is an example that demonstrates how to use the String.toUpperCase() method along with Array.map() to uppercase all elements in an array: Oct 06, 2017 - As you can see these two lines do two very different things. If you had wanted to add more than one item then badArray would be initialized correctly since Javascript would then be smart enough to know that you were initializing the array instead of stating how many elements you wanted to add. Summary: in this tutorial, you will learn how to convert an object to an array using Object's methods.. To convert an object to an array you use one of three methods: Object.keys(), Object.values(), and Object.entries().. Note that the Object.keys() method has been available since ECMAScript 2015 or ES6, and the Object.values() and Object.entries() have been available since ECMAScript 2017.
May 13, 2021 - The “trailing comma” style makes it easier to insert/remove items, because all lines become alike. ... A queue is one of the most common uses of an array. In computer science, this means an ordered collection of elements which supports two operations: The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Description. Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types of its elements are fixed. Learn how to use Js export and import. ... Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript
JavaScript provides many functions that can solve your problem without actually implementing the logic in a general cycle. Let's take a look. Find an object in an array by its values - Array.find. Let's say we want to find a car that is red. We can use the function Array.find. let car = cars.find(car => car.color === "red"); push () ¶. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array. You can also add multiple elements to ... Learn what is an array and how to use it in JavaScript. Also, learn how to access values from an array.
Replace the array with a new array. This is the fastest way to clear an array, but requires that you don't have references to the original array elsewhere in your code. For example, let's say your array looks like this: let a = [1,2,3];. To assign a to a new empty array, you'd use: a = []; But you can safely use [] instead. These two different statements both create a new empty array named points: ... The typeof operator returns object because a JavaScript array is an object. ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a ... 6/7/2008 · how to create a 3d array in javascript? Oct 18 '07 #1. Follow Post Reply. 3 18402 . gits. 5,390 Expert Mod 4TB. splitted from 'read first before posting' thread ...
As discussed above, JavaScript array is an object that represents a collection of similar type of elements. Now to initialize that array object, JavaScript provides three ways: First, Array declaration and initialization using JavaScript Array Literals. Second, it's declaration and initialization using JavaScript Array directly (new keyword). In other words, fill doesn't call ... array. The fill function simply fills the array with exactly what you tell it to. Does that make sense? For more info on the map function, see: developer.mozilla /en-US/docs/Web/JavaScript/Reference/…... 11/7/2020 · To create an array in JavaScript, type [] (opening and closing square brackets.) var arry = ['a','b','c']; In the above example, we are creating an array containing three strings of data; a b and c and setting it as the variable arry so it can be used later in the code. The square brackets are shorthand for the new Array () utility in JavaScript.
Multidimensional arrays are not directly provided in JavaScript. If we want to use anything which acts as a multidimensional array then we need to create a multidimensional array by using another one-dimensional array. So multidimensional arrays in JavaScript is known as arrays inside another array. generate a sequence numbers between a range javascript; create array javascript numbers; es6 compare two arrays; js subtract arrays; find non common elements from 2 arrays; javascript array value dom; javascript create variable containing an object that will contain three properties that store the length of each side of the box Nov 30, 2019 - Six ways to create an Array in JavaScript. Learn Different ways to create JavaScript Arrays.
In an earlier article, we looked at how to convert an array to string in vanilla JavaScript. Today, let us look at how to do the opposite: convert a string back to an array.. String.split() Method The String.split() method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as ... Could somebody please help me to create an array of array.I have a matrix calculator and I want to create an array of arrays ('smaller').How can I do it?The functiions create2Darray and calculateDet are working ok,so now problem with them.Would be really grateful for the help.I need an array of these arrays (main matrix's minors) to calculate ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Then, use the Array.from() method to create a new array of even numbers from the even object. In this tutorial, you have learned how to use the JavaScript Array Array.from() method to create an array from an array-like or iterable object. In the above code, we have an empty array inputArray. Next we have a size variable which will be used to limit the iterations for defining a finite array size, because by nature arrays are dynamic in JavaScript. In this loop, we take the inputArray[i] and store the return value from the prompt function. As the iterations increases, the size of ... The literal notation array makes it simple to create arrays in JavaScript. It comprises of two square brackets that wrap optional, comma-separated array elements. Number, string, boolean, null, undefined, object, function, regular expression, and other structures can be any type of array element.
May 21, 2021 - It does have to reallocate memory when the size required exceeds the backing store. ... @creationix You are right, arrays created whether with new Array or [] are instances, which should make them behave as object (being stored by reference). However, this is not the case. Javascript handles ... Jul 20, 2021 - A JavaScript array is initialized with the given elements, except in the case where a single argument is passed to the Array constructor and that argument is a number (see the arrayLength parameter below). Note that this special case only applies to JavaScript arrays created with the Array ... JavaScript has many ways to do anything. I've written on 10 Ways to Write pipe/compose in JavaScript, and now we're doing arrays. 1. Spread Operator (Shallow copy)Ever since ES6 dropped, this has been the most popular method. It's a brief syntax and you'll find
Create an object from an array of key/value pairs in JavaScript. How to Create a JavaScript Object From Array of Key/Value Pairs? Create an object from an array of key/value pairs in JavaScript. Daniyal Hamid ; 08 May, 2021 ; 3 Topics; 2 min read; Let's suppose you have an array of key/value pairs like the following: ...
Javascript Make Object Array Stack Overflow
Javascript How To Make An Array Of Consecutive Numbers Code
Six Ways To Create An Array In Javascript By Javascript
Javascript Array Distinct Ever Wanted To Get Distinct
Converting Object To An Array Samanthaming Com
How To Use Map Filter And Reduce In Javascript
Javascript Tutorial Array Definitive Guide Ictshore Com
Javascript Array A Complete Guide For Beginners Dataflair
Convert String With Commas To Array Stack Overflow
Data Structures Objects And Arrays Eloquent Javascript
How To Find Even Numbers In An Array Using Javascript
Javascript Array Methods To Make You A Better Developer By
Javascript Array Methods How To Use Map And Reduce
Group Array Of Objects With Same Key Value Pair Javascript
Wrong Way To Make A 2d Array In Javascript
5 Way To Append Item To Array In Javascript Samanthaming Com
How To Make A Copy Of An Array In Javascript Sergey
Javascript Array Displaying On A Html Table Javascript
Displaying All Elements Of Javascript Array By Looping
Javascript Array Contains A Step By Step Guide Career Karma
6 Use Case Of Spread With Array In Javascript Samanthaming Com
0 Response to "24 How To Make Array In Javascript"
Post a Comment