27 Javascript Operator Precedence Table
This is because the assignment operator returns the value that it assigned. First, b is set to 5. Then the a is also set to 5, the return value of b = 5, aka right operand of the assignment. Table. The following table is ordered from highest (19) to lowest (0) precedence. If OP1and OP2have different precedence levels (see the table below), the operator with the highest precedence goes first and associativity does not matter. Observe how multiplication has higher precedence than addition and executed first, even though addition is written first in the code. console.log(3 + 10 * 2); // logs 23
Logical Operators In Javascript Different Logical Operators
11/4/2015 · Table. Operator precedence determines the order in which operators are evaluated. Operators with higher precedence are evaluated first. A common example: 3 + 4 * 5 // returns 23. The multiplication operator (" * ") has higher precedence than the addition operator (" + ") and thus will be evaluated first.
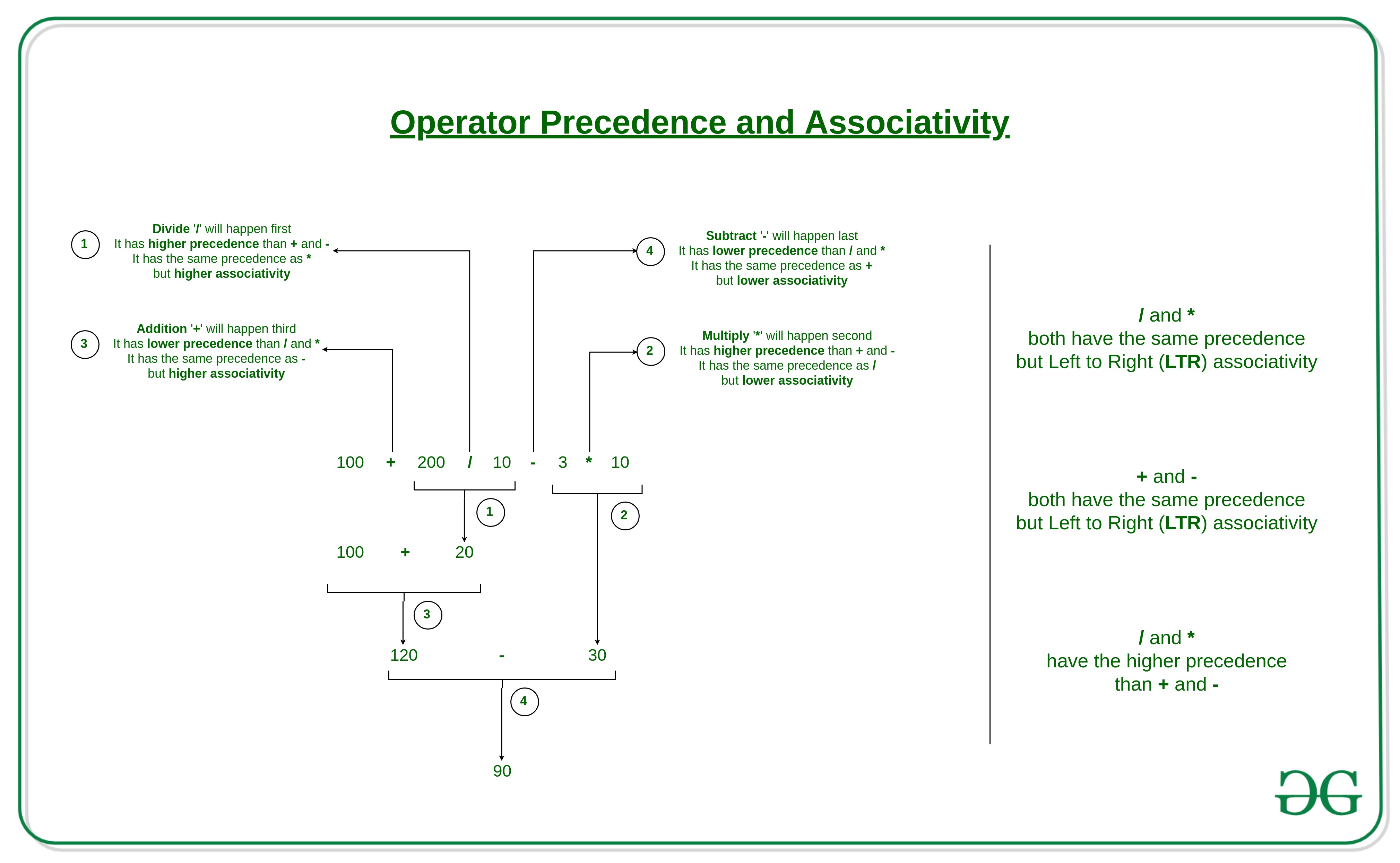
Javascript operator precedence table. The nullish coalescing operator is written as two question marks ??.. As it treats null and undefined similarly, we'll use a special term here, in this article. We'll say that an expression is "defined" when it's neither null nor undefined.. The result of a ?? b is:. if a is defined, then a,; if a isn't defined, then b.; In other words, ?? returns the first argument if it's not ... Above the table is written that operators are evaluated from left to right. This is definitely wrong. Operator associativity is not always left-to-right, most obvious at the assignment operators as in your example. The MDN table states this correct. Also, MSDN seems to oversimplify the precedence of postfix operators. Can anyone give me a ... JavaScript Arithmetic Operators. JavaScript arithmetic operators are used to perform arithmetical task. Here, the following table lists the arithmetic operators available in JavaScript: Operator. Meaning. +. This operator adds two operands. -. This operator subtracts second operand from the first.
From this table follows that: new Foo() has higher precedence than new Foo new Foo() has the same precedence as . operator new Foo has one level lower precedence than the . operator. new Date().toString() works perfectly because it evaluates as (new Date()).toString() new Date.toString() throws "TypeError: Date.toString is not a constructor" because . has higher precedence than new Date (and ... This is because the assignment operator returns the value that is assigned. First, b is set to 5. Then the a is also set to 5, the return value of b = 5, aka right operand of the assignment. Examples Table. The following table is ordered from highest (20) to lowest (1) precedence. Operator precedence is the set of rules that dictate in which order the operators are applied. JavaScript will always apply the logical NOT operator, !, first. Next, it applies the arithmetic operators, followed by the comparison operators. The logical AND and OR are applied last.
Precedence Operator Type Associativity; 15 [] · Parentheses Array subscript Member selection: Left to Right. 14 ++--Unary post-increment Unary post-decrement: Right to left: 13 ++--+-! ~ ( type) Unary pre-increment Unary pre-decrement Unary plus Unary minus Unary logical negation Unary bitwise complement Unary type cast: Right to left: 12 ... Operator precedence in JavaScript (JS) defines how the mathematical expression will be treated and which operator will be given preference over another. This is similar to normal mathematics expressions where multiplication has given more preference than addition or subtraction. For a full table of of operator precedence, view the reference on MDN. Of the operators we learned, here is the correct order of operations, from highest to lowest precedence. Grouping ( () )
Compound assignment operators are often used with loops, similar to incrementation and decrementation, and are utilized when equations need to be repeated or automated. Operator Precedence. Although we read from left to right, operators will be evaluated in order of precedence, just as in regular mathematics. Java Operator Precedence Example. Let's understand the operator precedence through an example. Consider the following expression and guess the answer. 1 + 5 * 3. 1 + 5 * 3. You might be thinking that the answer would be 18 but not so. Because the multiplication (*) operator has higher precedence than the addition (+) operator. 24/6/2020 · Operator Precedence Table: The operator precedence table can help one know the precedence of an operator relative to other operators. As one goes down the table, the precedence of these operators decreases over each other, that is, the priority of an operator is lower than the operators above it and higher than the ones below it. The operators in the same row have the same priority. In this table, 1 is the highest …
Operators (JavaScript) Operators fall into the following categories: The following table lists operator precedence and associativity. Operators with lower precedence evaluate first left-to-right or right-to-left as listed. Operator precedence If an expression has more than one operator, the execution order is defined by their precedence, or, in other words, the default priority order of operators. From school, we all know that the multiplication in the expression 1 + 2 * 2 should be calculated before the addition. That's exactly the precedence thing. 19/1/2019 · Operators with precedence of 0 are evaluated last in order.. “JavaScript Operator Precedence and Associativity Table” is published by JavaScript Teacher. Get started
The order of precedence that JavaScript uses is determined by the various expression operators. This table summarizes the complete order of precedence used by JavaScript. For example, the table above tells you that JavaScript performs multiplication before addition. Therefore, the correct answer for the expression =3+5*2 (just discussed) is 13. Operator precedence The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses. The following table describes the precedence of operators, from highest to lowest. Table of contents Table of contents ... and others that have an exponentiation operator (**), the exponentiation operator is defined to have a higher precedence than unary operators, such as unary + and unary -, but there are a few exceptions. For example, in Bash, the ** operator is defined to have a lower precedence than unary operators. In ...
Assignment operators are used to assign values to JavaScript variables. Given that x = 10 and y = 5 , the table below explains the assignment operators: Operator Operator Precedence Operator precedence determines the order in which operators are evaluated when more than one operator is used in an expression. The higher an operator's precedence, the earlier it is evaluated in comparison with the operators with lower precedence. Consider the following example: If there was a need to change the operator precedence we could rewrite the expression like so to alter the result to 100. The main idea is to remember that by using parentheses, you can set the precedence in your JavaScript as needed. In the following table, the operators at the top have the highest precedence, and the lowest, of course, have ...
JavaScript Operator Precedence and Associativity. Operator precedence determines the order in which operators are evaluated. Operators with higher precedence are evaluated first. For example, the expression (3+4*5), returns 23, because of multiplication operator(*) having higher precedence than addition(+). Thus * must be evaluated first. 65 rows · Table. The following table lists operators in order from highest precedence (21) to lowest precedence (1). Note that spread syntax is intentionally not included in the table — because, to quote an an answer at Stack Overflow, “ Spread syntax is not an operator and therefore does not have a precedence. Multiplication part of PEMDAS, or precedence power 15 on the table. 5 + 18 happens. Addition part of PEMDAS, or precedence power 14. The assignment operator, with precedence power of 3, resolves assigning a value of 23 to our variable a.
JavaScript Operator Precedence Values Pale red entries indicates ECMAScript 2015 (ES6) or higher. Expressions in parentheses are fully computed before the value is used in the rest of the expression. Test Yourself With Exercises Fortunately, we can use the precedence and associativity of JavaScript's operators information shown in table 1 to avoid any conflicting results. According to this table, the multiplication operator (*) has higher precedence than plus and subtraction operators.
What Does Associativity And Precedence Of An Operator In C
What Is The Use Of Associativity Amp Operator Precedence In C
Javascript Third Edition Ppt Video Online Download
An Introduction To Javascript Logical Operators By Examples
Nielit Gorakhpur Operator Precedence
Operator Precedence And Associativity Simple Table
Operator Precedence And Associativity Ishwaranand
Operator Or Item Basic Definition Application Examples
How To Apply Bodmas Rule In Javascript To Solve A Calculation
Java Operator Precedence Javatpoint
C Javascript Operator Precedence Chart Internet Amp World
Understanding Operator Precedence Inside Javascript
Operator Precedence And Associativity In C Aticleworld
Why Not Javascript Practicalli Clojurescript
Operators In C Chapter 3 Expressions Can Be Built Up From
Python Operator Precedence Pemdas Amp Short Circuiting
Assignment Operator In Javascript How Does Assignment
Operator Precedence And Associativity In C Geeksforgeeks
All The Operators In Python Precedence Too Tutorial Australia
Operator Precedence And Associativity In C C Programming
Basic Operators What Is An Operator Using Expression Is
C Operators Arithmetic Relational Logical Assignment
Computer Aided Clinical Trial Recruitment Based On Domain
What Is Operator Precedence In Javascript By Reina
Operator Precedence In Brief Javascript Tutorial Part 24
3 Operator Precedence Comparison Operator Precedence Table Javascript Full Course 2019
0 Response to "27 Javascript Operator Precedence Table"
Post a Comment