29 Event Target Class Javascript
21/9/2020 · Events happen in two phases: the bubbling phase and the capturing phase. In capture phase, also called the trickling phase, the event "trickles down" to the element that caused the event. It starts from the root level element and handler, and then propagates down to the element. The capture phase is completed when the event reaches the target. Event target class javascript. One Of My Students Tomas Alejandro Mondragon Pointed Out Concurrency Model And The Event Loop Javascript Mdn React Onclick Event Handlers A Complete Guide Logrocket Blog Vanilla Js Addeventlistener Queryselector And Closest By How To Find Click Classes In Google Tag Manager Elevar ...
React Onchange Events With Examples Upmostly
Event delegation is really cool! It's one of the most helpful patterns for DOM events. It's often used to add the same handling for many similar elements, but not only for that. The algorithm: Put a single handler on the container. In the handler - check the source element event.target.
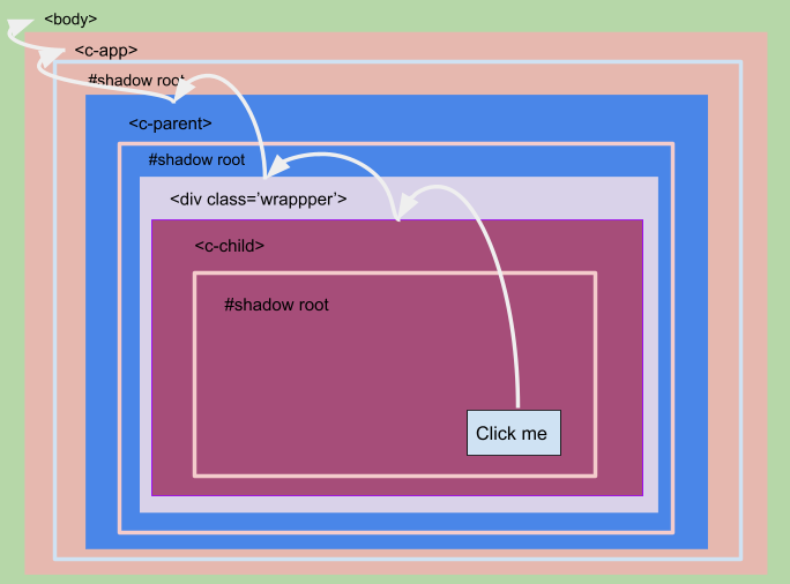
Event target class javascript. Alternatively, the event object which is passed into the callback function can be used to access the image element to add or remove the class. img.addEventListener("click", function(e) { e.target ... JavaScript provides an event handler in the form of the addEventListener () method. This handler can be attached to a specific HTML element you wish to monitor events for, and the element can have more than one handler attached. EventTargets are Objects that fire events. EventTargets usually expose an onevent property for each event where you can assign a Function to be called when the event fires. You can also use addEventListener () to hook up multiple listeners to the same event.
The addEventListener() initializes a function that will be called whenever the defined event is dispatched to the target.. To append additional data to the event object, you can use the CustomEvent interface and the detail property to pass custom data: My advice for cases where you have multiple elements dispatching events (e.g. you need a lot of event listeners in the same view) to try and use the following technique Event Delegation. Capturing and bubbling allow us to implement one of most powerful event handling patterns called event delegation. The idea is that if we have a lot of ... Checking event target selectors with event bubbling in vanilla JavaScript Event bubbling is an approach to listening for events that's better for performance and gives you a bit more flexibility. Instead of adding event listeners to specific elements, you listen to all events on a parent element (often the document or window).
The classList property returns the class name (s) of an element, as a DOMTokenList object. This property is useful to add, remove and toggle CSS classes on an element. The classList property is read-only, however, you can modify it by using the add () and remove () methods. Cross-browser solution: The classList property is not supported in IE9 ... class MyEventTarget extends EventTarget {constructor (mySecret) {super (); this. _secret = mySecret;} get secret {return this. _secret;}}; let myEventTarget = new MyEventTarget (5); let value = myEventTarget. secret; // == 5 myEventTarget. addEventListener ("foo", function (e) {this. _secret = e. detail;}); let event = new CustomEvent ("foo", {detail: 7}); myEventTarget. dispatchEvent (event… event.target returns the DOM element that triggered an specific event, so we can retrieve any property/ attribute that has a value. For example, when we console.log (e.target), we can see the class name of the element, type, position of the mouse, etc.
Element, Document, and Window are the most common event targets, but other objects can be event targets, too. For example XMLHttpRequest, AudioNode, AudioContext, and others. Many event targets (including elements, documents, and windows) also support setting event handlers via on event properties and attributes. 1. you are using jquery's each method incorrectly. it takes two arguments, neither one of them is an event (that would respond to the target property). from the docs: $.each ( [52, 97], function (index, value) { alert (index + ': ' + value); }); See CMS's answer for the proper way of checking if elements have a given class name. When you release a key while typing in a multiline text area, the keyup event's target is the <textarea> element. When you click an image, the target of the click event is the <img> element defining the image. To determine the event's target element, your JavaScript event handler functions can use the following event properties:
event.target. DOM Event Interface. The DOM element on the lefthand side of the call that triggered this event, eg: element .dispatchEvent ( event ) event.currentTarget. DOM Event Interface. The EventTarget whose EventListeners are currently being processed. As the event capturing and bubbling occurs, this value changes. We have DOM events in browsers, EventEmitter in Node.js, and almost every JavaScript framework has its own event or messaging system. The event system in Node.js is based on the E ventEmitter... Related Searches to Getting the ID of the element that fired an event - javascript tutorial jquery event target class get id of clicked element javascript jquery get id of element clicked jquery event target id get clicked button id in jquery jquery get clicked element class event.target properties event.target.id not working event target ...
The event constructor also accepts an object that specifies some important properties regarding the event. bubbles. The bubbles property specifies whether the event should be propagated upward to the parent element. Setting this to true means that if the event gets dispatched in a child element, the parent element can listen on the event and perform an action based on that. The event source specifies for an event handler the object on which that handler has been registered and which sent the event to it. The event target defines the path through which the event will travel when posted. The event type provides additional classification to events of the same Event class. Since: An event can be triggered by the user action e.g. clicking the mouse button or tapping keyboard, or generated by APIs to represent the progress of an asynchronous task. It can also be triggered programmatically, such as by calling the HTMLElement.click () method of an element, or by defining the event, then sending it to a specified target ...
After a click pass the event to ctrl. I want to write a conditional that will return true if the element.target has the class modal-click-shield Question: How can I use .hasClass() with event.target < body onclick = "myFunction(event)" > < p > Click on a paragraph. An alert box will alert the element that triggered the event. </ p > < p >< strong > Note: </ strong > The target property returns the element that triggered the event, and not necessarily the eventlistener's element. </ p > < script > function myFunction (event) { alert (event ... var hasClass = event.target.classList.contains('name'); Option #3 Since the .indexOf() method exists on arrays, you can use the .call() method to invoke the method with using the classList (where the string 'name' is the class you are checking):
The event occurs on the target (the target phase) Finally, the event bubbles up through the target's ancestors until the root element, document and window (the bubble phase). This mechanism is named event propagation. The event delegation is a useful pattern because you can listen for events on multiple elements using one event handler. Event.target The target property of the Event interface is a reference to the object onto which the event was dispatched. It is different from Event.currentTarget when the event handler is called during the bubbling or capturing phase of the event. The target property can be the element that registered for the event or a descendant of it. It is often useful to compare event.target to this in order to determine if the event is being handled due to event bubbling. This property is very useful in event delegation, when events bubble.
Event Object All event objects in the DOM are based on the Event Object. Therefore, all other event objects (like MouseEvent and KeyboardEvent) has access to the Event Object's properties and methods. Event Properties and Methods The target event property returns the element that triggered the event. The target property gets the element on which the event originally occurred, opposed to the currentTarget property, which always refers to the element whose event listener triggered the event. In JavaScript you add an event listener to a single element using this syntax: document.querySelector('.my-element').addEventListener('click', event => { //handle click }) But how can you attach the same event to multiple elements? In other words, how to call addEventListener () on multiple elements at the same time? You can do this in 2 ways.
If you're looking for JavaScript has class or JavaScript hasclass then there's a high probability that you used to work with jQuery in the past.. It's great news that you don't need a library anymore to check if an element has a class or not, because you can now simply do it with a call to classList.contains("class-name"). Here's an example. After an event object is created, we should "run" it on an element using the call elem.dispatchEvent (event). Then handlers react on it as if it were a regular browser event. If the event was created with the bubbles flag, then it bubbles. In the example below the click event is initiated in JavaScript.
Onchange Event In React Js Code Example
Event Target Innerhtml Code Example
One Of My Students Tomas Alejandro Mondragon Pointed Out
Interact Js Javascript Library
Given Below Are The Project Description And Their Html And
Js Not Working Or Navigation Not Working In In Page2 Monaca
Javascript Class Click Event Code Example
Master Javascript Form Validation By Building A Form From Scratch
Allow Only Numbers In Input In React Dev Community
Webinar Developing With The Modern App Stack Mean And Mern
How To Add Event Listener To Class In Javascript Code Example
Event Bubbling And Capturing In Javascript Javatpoint
Refactoring Youtube Player To Use Flux Part 2
How To Pass Values To Onclick React Function
Get The Closest Element By Selector
Handling Javafx Events Processing Events Javafx 2
Image Upload In Reactjs Clue Mediator
Get Class Of Clicked Element Using Javascript
Salesforce Code Crack Lightning Button Group In Lightning
How To Target An Html Class Or Id With Javascript By
Click Element Variable In Google Tag Manager Analytics Mania
React File Upload Proper And Easy Way With Nodejs
Working With Refs In React Css Tricks
Attaching Event Handlers To Dynamically Created Javascript
Base 64 Amp Url Amp Blob Amp Filereader Amp Createobjecturl Xgqfrms
E Target Value In Javascript Code Example
0 Response to "29 Event Target Class Javascript"
Post a Comment