31 Empty An Array In Javascript
Apr 01, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Jun 07, 2018 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers.
Javascript Empty Array In Inspector Still Has Elements
JavaScript has different ways to declare an empty array. One way is to declare the array with the square brackets, like below. var array1 = []; The other way is to use the constructor method by leaving the parameter empty. var array1 = new Array(); JavaScript Declare Empty Array Example
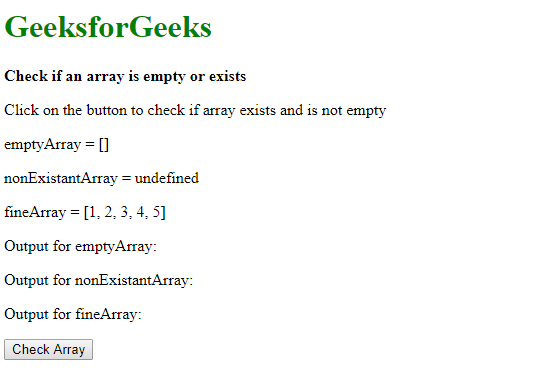
Empty an array in javascript. The easiest way to clear an array in javascript you just need to pass blank array literal [ ] to a variable like this (array = [ ]). JavaScript array is a collection of different types of values like string, number, boolean and many more which will be stored at a particular index of an array. Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career! The JavaScript exception "reduce of empty array with no initial value" occurs when a reduce function is used.
31/5/2021 · The square brackets symbol [] is a symbol that starts and ends an array data type, so you can use it to assign an empty array. The firstArr variable above will create a new empty array, while the secondArr variable will create an array with two elements. 1 week ago - The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. You can set the length property to truncate an array at any time. When you extend an array by changing its length property, the number of actual elements increases; for example, if you set length to 3 when it is currently 2, the array now contains 3 elements, which causes the third element to be a non-iterable empty slot.
list = [] assigns a reference to a new array to a variable, while any other references are unaffected. which means that references to the contents of the previous array are still kept in memory, leading to memory leaks. list.length = 0 deletes everything in the array, which does hit other references.. In other words, if you have two references to the same array (a = [1,2,3]; a2 = a;), and you ... Mar 10, 2020 - Today we are going to discuss on various ways to empty an array in JavaScript without deleting the array. Javascript queries related to "javascript is array empty" blank array check in javascript; how to check if an array id empty in javascript; array is not empty js using ? operator
The forEach() method calls a function once for each element in an array, in order. It performs a specified task on each array element. One thing to keep in mind is that, for array elements that are empty, forEach() method skips them and moves on to the next not empty element The Array () constructor creates Array objects. You can declare an array with the "new" keyword to instantiate the array in memory. Here's how you can declare new Array () constructor: let x = new Array (); - an empty array 4 weeks ago - You can use a lot of different techniques to empty an array in JavaScript. See which ones best suits your needs with this quick guide.
JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array (); const points = []; These two different statements both create a new array containing 6 numbers: 16/9/2019 · There are multiple ways to clear/empty an array in JavaScript. You need to use them based on the context. Let us look at each of them. Assume we have an array defined as −. let arr = [1, 'test', {}, 123.43]; Substituting with a new array − arr = []; This is the fastest way. This will set arr to a new array. You can declare arrays in multiple ways. The simplest is when all you need is only an empty array. I believe that's what you're looking for. Here's the code: var arrayName = []; If you know the expected length of the array you're creating, you can...
May 06, 2021 - They allow you to store multiple values in a convenient, indexed set. In JavaScript, arrays can be declared literally or they can be initialized using the Array constructor function. But wait… What if you want to empty an array instead of creating one? Hmm… perhaps not as straightforward. Here are multiple ways to check for empty object or array in javascript : 1. Check if an array is empty This is a very basic method to check if the object is empty using the if-else condition. For Array objects, the toString method joins the array and returns one string containing each array element separated by commas. This means we get the empty string ''. What's the ToNumber? Basically apply the + operation on the target, for boolean, 1 if the argument is true. +0 if the argument is false.
While it does prevent allocation of a new object, in order to empty the array all the indices in it must be deleted (that's what setting the length to 0 does). This operation may or may not be optimized, depending on how a particular js engine works. Following are the method offered by JavaScript programming to check an empty array: The Array.isArray () function checks the array type (passed parameter is an array or not) and array.length find the length of the array. So, we can identify the empty array easily. You can use them individually and separately as well. Use the length Property to Empty an Array in JavaScript It clears the existing array by setting its length to 0. The length property of an array is a read/write property, so it also works when using the strict mode in ECMAScript 5. Arr1.length = 0
5/10/2020 · When you're programming in JavaScript, you might need to know how to check whether an array is empty or not. To check if an array is empty or not, you can use the .length property. The length property sets or returns the number of elements in an array. By knowing the number of elements in the array, you can tell if it is empty or not. An empty array will have 0 elements inside of it. Let’s run … When you set a value to an element in an array that exceeds the length of the array, JavaScript creates something called "empty slots". These actually have the value of undefined, but you will see something like: [1, 2, 3, 7 x empty, 11] depending on where you run it (it's different for every browser, node, etc.). JavaScript Program to Empty an Array. In this example, you will learn to write a JavaScript program that will empty an array. To understand this example, you should have the knowledge of the following JavaScript programming topics: JavaScript Function and Function Expressions;
The code above will set the number array to a new empty array. This is recommended when you don't have any references to the original array 'array1'. You should be careful with this way of empty the array, because if you have referenced this array from another variable, then the original reference array will remain unchanged. The second way to empty an array is to set its length to zero: a.length = 0; The lengthproperty is read/write property of an Arrayobject. When the lengthproperty is set to zero, all elements of the array are automatically deleted. Dec 22, 2020 - The Arrays are for JavaScript developers like how screws and nails are for carpenters. Hence it is im...
This will clear the existing array by setting its length to 0. Some have argued that this may not work in all implementations of JavaScript, but it turns out that this is not the case. It also works when using "strict mode" in ECMAScript 5 because the length property of an array is a read/write property. Method 3(as suggestedby Anthony) Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Initializing empty arrays in javascript; Next Recommended Reading Swap Array Elements In JavaScript Using Array Prototype And Other Methods. LATEST BLOGS A Problem That I Faced In ASP.NET Core Localization And Its Solution; Azure Open Data Set - Curated List Of Public Data Set; JavaScript Fetch API Made Easy ...
There are various ways to empty a JavaScript array. The easiest one is to set its length to 0: const list = ['a', 'b', 'c'] list. length = 0. Another method mutates the original array reference, assigning an empty array to the original variable, so it requires using let instead of const: In the above example first, we create an empty array in javascript or we can also empty an array in javascript. Then using Array.isArray () method first we check that value is an array or not. After it confirms that value is array then we check it's length. If array length is zero then it means the array is empty. 23/3/2021 · This wikiHow teaches you how to empty a JavaScript array. There are three primary ways to clear an array—replacing the array with a new array, setting its property length to zero, and splicing the array. These three methods work similarly, although replacing the array with a new array (the fastest method) shouldn't be used if other code ...
How to Remove Empty Elements from an Array in Javascript To remove empty elements from a JavaScript Array, the filter () method can be used, which will return a new array with the elements passing the criteria of the callback function. The filter () method creates an array filled with all array elements that pass a test. Sep 11, 2009 - As the above jsPerf test case shows, creating a new array is FASTER than setting length to 0. The reason for this is that most of the built-in functions and constructors for JS nowadays tend to use native code under the surface to optimize (thanks in no small part to Javascript becoming so popular). 16 hours ago - Removing & Clearing Items From JavaScript arrays can be confusing. Splice, substring, substr, pop, shift & filter can be used. Learn how they differ and work.
Code to check if an array is empty using javascript We will quickly go over the code and its demonstration to check if an array is empty or not and also see why these specific functions are used. //To check if an array is empty using javascript function arrayIsEmpty(array) { //If it's not an array, return FALSE.
How To Flattening Multidimensional Arrays In Javascript
Is Empty Array In Javascript Truthy Or Falsy A Journey Of
Benchmark Empty An Array In Javascript Measurethat Net
Check If Array Is Empty Or Undefined In Javascript Scratch Code
How To Check If Array Is Empty Or Not Quora
How To Empty An Array In Javascript Creatifwerks
Javascript Remove Empty Arrays From Array Code Example
How To Check If A Javascript Array Is Empty Or Not With Length
Better Array Check With Array Isarray By Samantha Ming
Javascript Unexpected Include Splitted And Empty Array Filter
How To Empty An Array In Javascript
How To Deep Clone An Array In Javascript Dev Community
How Do I Empty An Array In Javascript 30 Seconds Of Code
Dynamic Array In Javascript Using An Array Literal And
Remove Empty Cells In Array Javascript
How To Remove Commas From Array In Javascript
Array In Object Empty When Passed Or Json Stringified
Powershell Tip 116 Remove Empty Elements From An Array
How To Empty An Array In Javascript Spursclick
How To Check If Array Is Empty Or Null Or Undefined In
How To Check If An Array Is Empty In Javascript
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Useeffect Dependency Array And Object Comparison Dev Community
Empty An Array In Javascript Code With Stupid
How To Reduce Javascript Arrays With Array Reduce Codeburst
How Do I Empty An Array In Javascript Stack Overflow
Hacks For Creating Javascript Arrays
How To Declare An Empty Array In Javascript Quora
Function Returns Empty Array Instead Of Arguments Object When
Javascript Clear Array How To Empty Array In Javascript
0 Response to "31 Empty An Array In Javascript"
Post a Comment