31 While Loop Example In Javascript
statement. A statement that is executed at least once and is re-executed each time the condition evaluates to true. To execute multiple statements within the loop, use a block statement ({ ... }) to group those statements. condition JavaScript while loop examples. See the following example that uses the while statement: First, outside of the loop, the count variable is set to 1. Second, before the first iteration begins, the while statement checks if count is less than 10 and execute the statements inside the loop body. Third, in each iteration, the loop increments count ...
Mysql While Loop Explained By A Practical Example
JavaScript while Loop and do-while Loop. Whenever you want to execute a certain statement over and over again you can use the JavaScript while loop to ease up your work. JavaScript while loop lets us iterate the code block as long as the specified condition is true. Just like for Loop, the while and do...while loop are also used to execute code ...
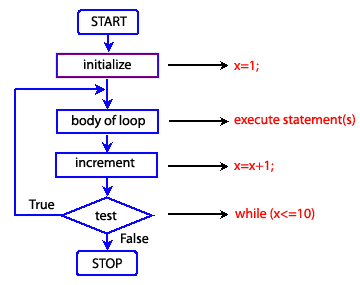
While loop example in javascript. Let's make an example using the numbers 6, 10, 15.. The number 6 will execute - in your first example (the working example) - the second if block because in the first one the condition will not be satisfied while the third and fourth block will be ignored, and - in your second example (the not-working example) - will execute the first if block and ignore the other blocks that follow. while and do...while loops are conditionally based, and therefore it is not necessary to know beforehand how many times the loop will run. While Loop. In JavaScript, a while statement is a loop that executes as long as the specified condition evaluates to true. The syntax is very similar to an if statement, as seen below. Comparing For and While. If you have read the previous chapter, about the for loop, you will discover that a while loop is much the same as a for loop, with statement 1 and statement 3 omitted. The loop in this example uses a for loop to collect the car names from the cars array:
The do/while statement creates a loop that executes a block of code once, before checking if the condition is true, then it will repeat the loop as long as the condition is true. The do/while statement is used when you want to run a loop at least one time, no matter what. JavaScript supports different kinds of loops: Using while. The following while loop iterates as long as n is less than three. var n = 0; var x = 0; while ( n < 3) { n ++; x += n; } Copy to Clipboard. Each iteration, the loop increments n and adds it to x . Therefore, x and n take on the following values: After the first pass: n = 1 and x = 1. After the second pass: n = 2 and x = 3. From the example above, you can read: Statement 1 sets a variable before the loop starts (let i = 0). Statement 2 defines the condition for the loop to run (i must be less than 5). Statement 3 increases a value (i++) each time the code block in the loop has been executed.
The while statement creates a loop that is executed while a specified condition is true. The loop will continue to run as long as the condition is true. It will only stop when the condition becomes false. JavaScript supports different kinds of loops: for - loops through a block of code a number of times. for/in - loops through the properties of ... JavaScript while loop (with examples and use cases) The while statement will help you to execute a piece of code repeatedly until you achieve the desired condition. Learn its syntax and use cases in this tutorial. Posted on January 16, 2021. The while statement is used to create a loop that continues to execute the statement as long as the ... 1 week ago - The value of label may be any JavaScript identifier that is not a reserved word. The statement that you identify with a label may be any statement. ... In this example, the label markLoop identifies a while loop.
while loop Syntax. In the below while loop syntax, you can see their is only condition pass to while nothing else. If condition is true then it execute block of code. while (condition) { // code block to be executed until condition return true } Some Example of Javascript while loop. Here, you'll learn some use cases or examples of a while loop. Flowchart of JavaScript while loop: Example write JavaScript code to print the squares and cubes of number from 2 to 7 using while loop: Example write js code to calculate the nth power of a number n, i.e. np by using while loop: Example write js codes which reverse the order of the input integer using while loop: In this tutorial, you will learn about while loop and do...while loop with the help of examples. In programming, loops are used to repeat a block of code. For example, if you want to show a message 100 times, then you can use a loop. It's just a simple example; you can achieve much more with loops. In the previous tutorial, you learned about the JavaScript ...
JavaScript mainly provides three ways for executing the loops. While all the ways provide similar basic functionality, they differ in their syntax and condition checking time. Let us learn about each one of these in details. while loop: A while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean ... Code language: JavaScript (javascript) JavaScript for loop examples. Let's take some examples of using the for loop. 1) Simple for loop examples. The following example uses the for loop statement that shows the numbers from 1 to 5 in the Console window. Apr 28, 2021 - Loops are used in JavaScript to perform repeated tasks based on a condition. Conditions typically return true or false when analysed. A loop will continue running until the defined condition returns false. The three most common types of loops are forwhiledo whileYou can type js for, js while or js
Syntax. do { } while (condition); The example below uses a do/while loop. The loop will always be executed at least once, even if the condition is false, because the code block is executed before the condition is tested: JavaScript - While Loop. JavaScript includes while loop to execute code repeatedly till it satisfies a specified condition. Unlike for loop, while loop only requires condition expression. Syntax: while (condition expression) { /* code to be executed till the specified condition is true */ } Example: while loop. Aug 30, 2018 - Again, pretty similar to the example above, but there is actually one major difference. In the do while loop, the statements in the do while are always run once because the conditional is at the end. The conditional here tells the browser to go back and run through the do part again.
In the following sections, we will ... in detail. ... This is the simplest looping statement provided by JavaScript. The while loop loops through a block of code as long as the specified condition evaluates to true. As soon as the condition fails, the loop is stopped. The generic syntax of the while loop is: ... The following example defines a ... In this tutorial, you will learn about the loops and about for loops in JavaScript with the help of examples. In programming, loops are used to repeat a block of code. For example, if you want to show a message 100 times, then you can use a loop. In this tutorial, we will learn-How to use Loop? Different Types of Loops; for loop; while loop; do…while loop; How to use Loop? Loops are useful when you have to execute the same lines of code repeatedly, for a specific number of times or as long as a specific condition is true. Suppose you want to type a 'Hello' message 100 times in ...
If you’re not sure where to begin or what to learn next, this is a great place to start. Check out our top coding courses, Skill Paths, and Career Paths. Code language: JavaScript (javascript) In this example, the count variable is set to 0 and is incremented by one in each loop iteration. The loop continues as long as the count is less than 10.. You often use the do-while statement in the situation that the body of the loop needs to execute at least one. This is an important feature of the do-while loop.. The most typical example of using the ... Loops in Java come into use when we need to repeatedly execute a block of statements.. Java while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. The while loop can be thought of as a repeating if statement. Syntax: while (test_expression) { // statements update_expression; }
3 weeks ago - For example, outputting goods from a list one after another or just running the same code for each number from 1 to 10. Loops are a way to repeat the same code multiple times. ... While the condition is truthy, the code from the loop body is executed. For instance, the loop below outputs i ... The While loop in JavaScript starts with the condition, if the condition is True, statements inside the while loop will execute. If it is false, it won't run at least once. It means, JavaScript while loop may execute zero or more time. JavaScript While Loop Syntax. The syntax of the While loop in JavaScript is as follows: A JavaScript while loop executes a block of code while a condition evaluates to true. while loops stop executing when their condition evaluates to false. A while loop lets you repeat a block of code multiple times without copy-pasting your code. while loops are often used if you want to run code for an unspecified number of times.
JavaScript While Loop with an Example. Bikash May 27, 2021 May 31, 2021. J avaScript while Loop or any other loops are used to execute a block of code till the condition is true. In This Article. 1 Introduction to the JavaScript while loop statement. 1.1 Syntax: 2 Example of JavaScript While Loop. May 31, 2019 - The general syntax of a while loop in JavaScript is given below. Here, condition is a statement. It can consist of multiple sub-statements i.e. there can be multiple conditions. For as long as condition evaluates to true, the code written inside the while block keeps on executing. In JavaScript (and most other languages), "loops" enable your program to continuously execute a block of code for a given number of times, or while a given condition is true. The JavaScript While loop executes code while a condition is true. For example, you could make your program display ...
The most basic loop in JavaScript is the while loop which would be discussed in this chapter. The purpose of a while loop is to execute a statement or code block repeatedly as long as an expression is true. Once the expression becomes false, the loop terminates. ... Try the following example to ...
How To Make A While True Loop Javascript Code Example
Javascript Online Presentation
For Loop Javascript Old School Loops In Javascript For
Why The Huge Time Difference Between While And Do While In
While Loop In Javascript The Engineering Projects
Php While Loop And Do While Loop Studytonight
How To Work With While Amp Amp Do While Loops In Javascript
Do While Statement In Javascript
Php Table Issue With While Loop Stack Overflow
Tools Qa Javascript Loop While Do While For And For In
Javascript While Loop Notesformsc
Javascript Do While Loop W3resource
Javascript Tutorials For Beginners Javascript Continue
Powershell Loops For Foreach While Do Until Continue
Javascript While Loop And Do While Loop With Programming Examples
Javascript For Loop Flowchart And Programming Examples
How To Iterate Through Java List Seven 7 Ways To Iterate
Javascript While Loop Example Web Code Geeks 2021
For Loops Vs While Loops In Javascript By Tirzah Morrison
Use While Loop To Pop All Elements In An Array In Javascript
Javascript While Loop W3resource
Chapter 5 Nested Loops Which Loop To Use
Basic While Loop Query Why Is 1 Logged Stack Overflow
Difference Between While And Do While In Java
Sql While Loop With Simple Examples
0 Response to "31 While Loop Example In Javascript"
Post a Comment