24 How To Add Css In Javascript
The most straightforward path. Query the element from DOM and change it's inline styles. document.getElementById('target').style.color = 'tomato'; Enter fullscreen mode. Exit fullscreen mode. Short and simple. 2. Global styles. Another option is to create <style> tag, fill it with CSS rules and append the tag to the DOM. In this video, we create a starter template and link external CSS and Javascript files into our HTML file. We also look into what a CDN is and general boiler...
Add Css And Javascript Files Dynamically Inside Html Head
29/12/2020 · Let's begin by adding some JavaScript to a file in src/main/resources/static/js/cssandjs/actions.js: function showAlert() { alert("The button was clicked!"); } Then we hop back over to our Thymeleaf template and add a <script> tag that points to our JavaScript file: <script type="text/javascript" th:src="@{/js/cssandjs/actions.js}"></script>
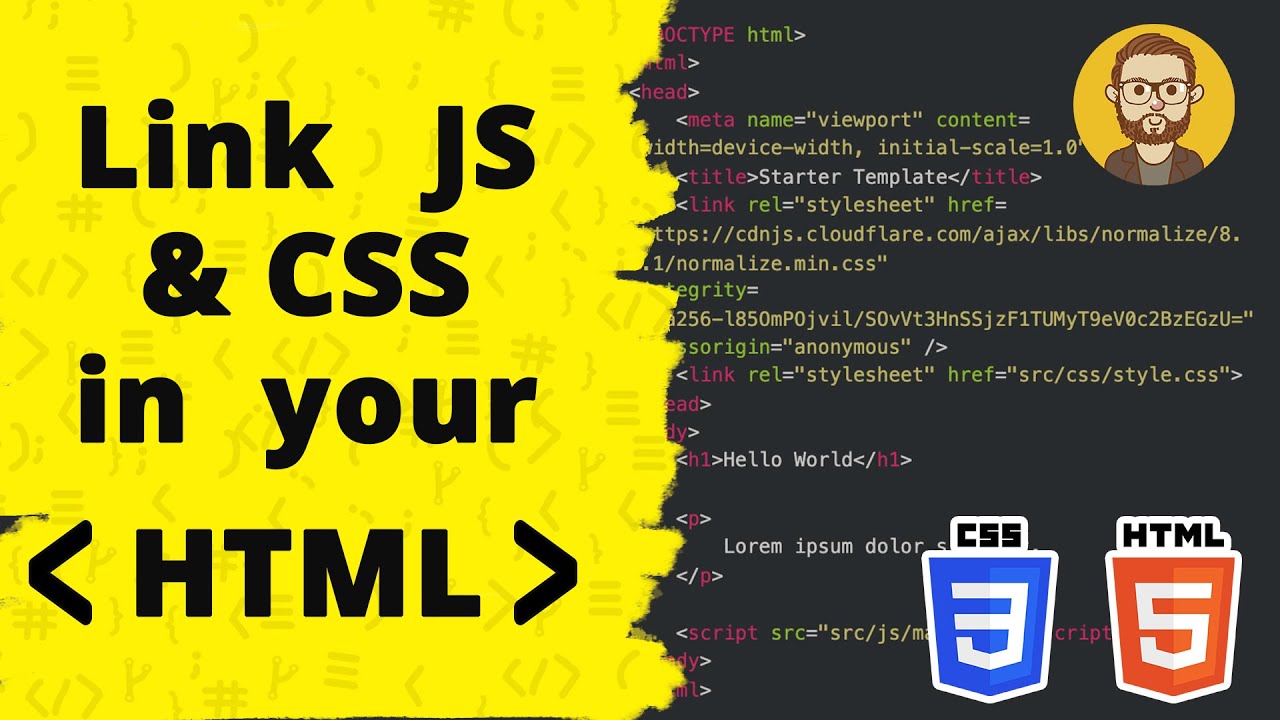
How to add css in javascript. Add reference for the JavaScript and CSS plugins 08-18-2020 02:14 AM. Hey, I created a multi select option set field using javascript refering to the blog post from Nicholas Hayduk. I want to change the look of this field, using Bootsrap Multiselect . However I don't know where I should put the reference of the library. Action: A JavaScript demonstration. Make a new HTML document, doc5.html. Copy and paste the content from here, making sure that you scroll to get all of it: Make a new CSS file, style5.css. Copy and paste the content from here: Make a new text file, script5.js. Copy and paste the content from here: // JavaScript demonstration var square ... Jul 09, 2015 - The accessing style sheets example ... and adding and removing a new style sheet to the page. ... The stylesheet object is available through JavaScript, and allows you to access information about a style sheet referenced from the current web page, such as if it is disabled, its location, and the list of CSS rules it ...
In this article, we'll look at ways to add CSS styles dynamically with JavaScript. Using the window.document.styleSheets Object and the insertRule Method We can get the first stylesheet for a document with the window.document.styleSheets property. Then we can call the insertRule method on the returned stylesheet object. Dec 25, 2019 - This is generally done by including style tag in the head tag of the HTML markup. Adding styles by Javascript involves creating the style element, inserting CSS properties to the style tag and then append the style to the head by Javascript. Let’s see the code on how to add styles globally ... Hello Friends, Today in this post, we'll learn how to Add Preloader In Website Using HTML, CSS & Javascript. recently I have shared a Moving Text Animation | Using HTML5 | CSS3. but our today's topic is Preloader Animation UI Design | Loader Animation.. Basically, preloaders or loaders are what you first see on the page when the rest of the site's content didn't load yet.
Nov 09, 2011 - By default, the Javascript is executed in a sandboxed IFRAME, but really it's not any different from how the browser processes your *.js files. Just be sure to author your own CSS and host it on your own server then XSS isn't an issue. But the sandbox provides that much more security and peace ... Apr 18, 2016 - It’s technically easy, but it simply doesn’t scale. If there is CSS in JavaScript, we should be able to control it like a real style sheet. We should be able to define styles and then add, remove or update them inside. And those changes should be applied to the page just like the style ... In JavaScript, CSS transitions are generally easier to work with than CSS animations. CSS Matrices are generally a pain to deal with, especially for beginners. Thinking about what should be done and planning how to do it are essential in coding animations.
Nov 30, 2013 - Maybe you want to enable them to add basic styling to a piece of formatted content – something you see sometimes in forums and comments sections. I'm here to tell you that CSS styles are just as bad as any other form of user generated content when it comes to injecting JavaScript into a page. When you can't use a JavaScript or CSS concatenater, this method can be useful for adding scripts and styles to your site on the fly. CSS JavaScript This is how we typically add JavaScript and CSS files to websites: The CSS Object Model is a set of APIs allowing the manipulation of CSS from JavaScript. It is much like the DOM, but for the CSS rather than the HTML. It allows users to read and modify [the] CSS style dynamically. The CSSStyleSheet.insertRule () method inserts a new CSS rule to a stylesheet.
Adding a CSS class using JQuery. Luckily enough, the JQuery library has a method called addClass, which can be used to add CSS classes to an element. Let’s modify the example above to use JQuery instead of vanilla JavaScript: setTimeout(function(){ //Add the CSS class using the JQuery addClass method. $('#intro').addClass('newClass'); }, 5000); 8/7/2019 · You write CSS associated with your individual component and your build process compiles the application. When this happens, most CSS-in-JS frameworks will output the associated CSS of only the components that are rendered on the page at any given time. CSS-in-JS frameworks do this by outputting that CSS within a <style> tag in the <head> of your application. Jul 30, 2017 - As one of the core technologies of the web alongside HTML and CSS, JavaScript is used to make webpages interactive and to build web apps. Modern web browsers, which adhere to common display standards, support JavaScript through built-in engines without the need for additional plugins.
Call them html, css, and javascript. Inside your html directory, create a file called css-and-js.html. Inside your css directory, create a file called styles.css. And inside your javascript directory, create a file called script.js. 2. Aug 23, 2017 - Yesterday, we looked at how to get an element’s CSS attributes with vanilla JavaScript. One thing I neglected to mention: getComputedStyle() can only be used to get values, not set them. Today, let’s look at how to set CSS with vanilla JS. Approach 1: Inline Styles The easiest way to set ... JavaScript is what animates HTML and CSS, and it's what brings your website to life. JavaScript can be compared to the body's ability to perform actions such as walking or talking. So when you add JavaScript to HTML and CSS, it transforms the body from being a beautifully dressed mannequin into a real-life walking talking human being.
Files that are called this way are added to the page as they are encountered in the page's source, or synchronously. For the most part, this setup meets our needs just fine, though in the world of synchronous Ajax design patterns, the ability to also fire up JavaScript/ CSS on demand is becoming ... How does the Add CSS class JavaScript code work?. The HTML and CSS parts in our Add CSS class JavaScript example are straight forward. We created a div element containing a button and a paragraph with some text. With just a couple of CSS properties we defined the visual appearance of our content block before and after the button is clicked. Code language: JavaScript (javascript) Global Styles. To add the global styles to an element, you create the style element, fill it with the CSS rules, and append the style element to the DOM tree, like this:
The style.css file is required in all themes, but it may be necessary to add other files to extend the functionality of your theme. Tip: WordPress includes a number of JavaScript files as part of the software package, including commonly used libraries such as jQuery. There are many ways to add CSS to your webpage via JavaScript. But, basically, developers add CSS to a particular element or tag, so you can get it by style property of javascript. The Style property adds CSS to an element by an object's DOM. The ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
HTML CSS JAVASCRIPT SQL PYTHON PHP BOOTSTRAP HOW TO W3.CSS JAVA JQUERY C++ C# R React Kotlin ... JS HOME JS Introduction JS Where To JS Output JS Statements JS Syntax JS Comments JS Variables JS Let JS Const JS Operators JS Arithmetic JS Assignment JS Data Types JS Functions JS ... Tip: Learn more about the className property in our JavaScript Reference. ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: ... Thank You For Helping Us! Your message has been sent to W3Schools. ... HTML Tutorial CSS ... Oct 02, 2015 - Ignoring inline styles, the other approach that we can use to introduce elements to the goodness that is CSS styling involves JavaScript. We can use JavaScript to directly set a style on an element, and we can also use JavaScript to add or remove class values on elements which will alter which ...
Adding the keyframes rule to an element with Javascript or jQuery is not possible. // There is no such thing as this $ ("#element").css ('keyframes',....); In this case you have no option but to dynamically add the keyframes rule to a stylesheet using Javascript. The HTML DOM allows you to execute code when an event occurs. Events are generated by the browser when "things happen" to HTML elements: An element is clicked on. The page has loaded. Input fields are changed. You will learn more about events in the next chapter of this tutorial. This is where JavaScript comes in. We use JavaScript to control our animation. Step 1: Check if the form submit button has been clicked. Step 2: Select all form fields. Step 3: Check if the input fields are empty. Step 4: Add the CSS selector using JavaScript classList property. You can read more about the classList property here.
3/3/2020 · The DOM style property is the simplest way to set and get CSS styles from an element in JavaScript. Usually, when you want to add multiple CSS properties to an element, you have to set them individually as shown below: const btn = document. querySelector ('.btn'); // set inline CSS styles btn. style. width = '150px'; btn. style. height = '40px'; btn. style. color = 'blue'; However, this approach is not … 18/11/2016 · There are several ways to apply a CSS property to an element using JavaScript and jQuery. All these alternatives directly or indirectly target the style global attribute, which contains the CSS styling declarations for the element. 1. Using jQuery – .css() method 30/7/2021 · The CSS file is used to describe how HTML elements will be displayed. There are various ways to add CSS file in the HTML document. JavaScript can also be used to load a CSS file in the HTML document. Approach: Use document.getElementsByTagName() method to get HTML head element. Create new link element using createElement(‘link’) method.
Add a CSS Class. We will add another class called thorn to it. To do so in JavaScript, we need to make use of an element's classList read-only property, which returns the DOMTokenList of the element. > document.getElementById('rose').classList; The DOMTokenList interface has two properties, value and length. 31/3/2009 · var css = new function() { function addStyleSheet() { let head = document.head; let style = document.createElement("style"); head.appendChild(style); } this.insert = function(rule) { if(document.styleSheets.length == 0) { addStyleSheet(); } let sheet = document.styleSheets[document.styleSheets.length - 1]; let rules = sheet.rules; sheet.insertRule(rule, rules.length); } } css.insert… 26/11/2020 · In this article, we will see how to dynamically create a CSS class and apply to the element dynamically using JavaScript. To do that, first we create a class and assign it to HTML elements on which we want to apply CSS property. We can use className and classList property in JavaScript.
Great, now that we've added some JavaScript, lets add some CSS. Adding CSS to Our Webpage. First create a folder named 'styles'. Inside this folder, create a file named 'styles.css'. Type or paste the following code into 'styles.css': body { text-align: center; background-color: #f0e8c5; } Jul 28, 2003 - This page requires javascript to function properly. It seems that your browser does not have Javascript enabled. Please enable Javascript and press the Reload/Refresh button on your browser. ... Putting Javascript in .css file..
How To Add Custom Css And Javascript In Shopify Easy
Magento Css And Magento Javascript On Frontend
How To Add Css Amp Javascript To Your Html
Learn Web Development Basics Html Css And Javascript
Setting Css Styles With Javascript Soshace Soshace
Html To Pdf By Javascript How Can I Add Css Or Table
How To Add Custom Notifications Alerts Using Html Css
Where To Add Custom Code In Divi Css Javascript Amp Php
Add Css Amp Javascript To Your Drupal Site
Css Amp Javascript Certification Course For Beginners Free
Add Javascript And Css In Shiny
Introduction To The Promotion Customization With Css Styles
Add Custom Javascript And Css To Wordpress Dynamic Drive Blog
How To Add Custom Css Or Javascript To Sharepoint Online
Simple Custom Css And Js Wordpress Plugin Wordpress Org
Wix Answers Help Center Adding Custom Css And Javascript To
Add Css Class Javascript Code Change Class On Click In
2 Different Ways To Add Css Class Using Javascript
Simple Custom Css And Js Wordpress Plugin Wordpress Org
How To Make A Music Website Using Html Css Javascript Add
How To Add Multiple Css Styles Using Javascript
Javascript Jquery Ajax Are They The Same Or Different
0 Response to "24 How To Add Css In Javascript"
Post a Comment