30 Javascript Xhr Get Request
What you need to do is: var requests; this.xhr = sinon.useFakeXMLHttpRequest (); this.xhr.onCreate = function (xhr) { requests.push (xhr); } And then later on, you can check your requests array for headers by: console.log (requests [0].requestHeaders); To access your request headers. Share. Improve this answer. answered Jan 15 '13 at 22:35. The signature of the utility function loadFile declares (i) a target URL to read (via an HTTP GET request), (ii) a function to execute on successful completion of the XHR operation, and (iii) an arbitrary list of additional arguments that are passed through the XHR object (via the arguments property) to the success callback function.
Cypress Is Not Capturing App Xhr Its Only Capturing 3rd Party
19. In XMLHttpRequest, using XMLHttpRequest.responseText may raise the exception like below. Failed to read the \'responseText\' property from \'XMLHttpRequest\': The value is only accessible if the object\'s \'responseType\' is \'\' or \'text\' (was \'arraybuffer\') Best way to access the response from XHR as follows.
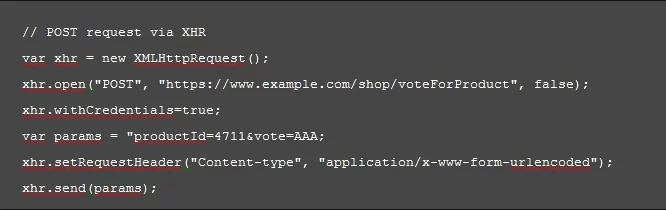
Javascript xhr get request. The XMLHttpRequest method send() sends the request to the server. A simple XHR request. GitHub Gist: instantly share code, notes, and snippets. XMLHttpRequest.send () The XMLHttpRequest method send () sends the request to the server. If the request is asynchronous (which is the default), this method returns as soon as the request is sent and the result is delivered using events. If the request is synchronous, this method doesn't return until the response has arrived.
// Set header for request xhr.setRequestHeader('Content-Type', 'application/json'); // Get header for response xhr.getResponseHeader('Content-Type') xhr.abort() This method is useful when we want to cancel a request. Imagine a scenario where a user wants to cancel a request because it's taking too long. xhr.abort() can be used to stop the request. 14/6/2021 · Following are the steps to use XHR requests in JavaScript: Step 1: To set up the HTTP request, we need to create an instance of XMLHttpRequest, as the code below shows: // setup http request var xmlReq = new XMLHttpRequest (); Step 2: Next, add the callback handlers or events to get the response from the HTTP request: Want to send some background (Ajax) Http requests with JavaScript? Learn how to use XMLHttpRequest for that!Join the full "JavaScript - The Complete Guide" c...
Sep 22, 2020 - XHR is the XMLHttpRequest Object which interacts with the server. Ajax technique in the nutshell leverages the XHR request to send and receive data from the webserver. This object is provided by the browser’s javascript environment. It transfers the data between the web browser and server. Oct 28, 2020 - XHR requires quite a bit of boilerplate code. We need to construct the object, set the HTTP method every time and explicitly send the request. It also relies on callback functions which can get messy in complex scenarios if not handled properly. In modern JavaScript, we use a function called ... Basic XHR Request To send an HTTP request using XHR, create an XMLHttpRequest object, open a connection to URL, and send the request. Once the request completes, the object will contain useful information such as the response body and the HTTP status code. Let's use JSONPlaceholder test REST API to send a GET request using XHR:
send a get request javascript; request XHR; how to define xmlhttprequest in javascript; http request in html; how is an xmlhttprequest made; function loadAjax() { Var xhttp = new XHLHTTPREQUEST(); difference between load and loadend ajax event; get request javascript; http post request javascript; example xhr request; add xmlhttprequest ajax; ajax xmlhttprequest May 21, 2018 - AJAX is the primary method you use to get and send data to APIs in JavaScript. Making AJAX requests with the XMLHttpRequest() method, often referred to as XHR, is a three step process: A request made via XMLHttpRequest can fetch the data in one of two ways, asynchronously or synchronously. The type of request is dictated by the optional async argument (the third argument) that is set on the XMLHttpRequest.open() method.
A common use of JSON is to read data from a web server, and display the data in a web page. This chapter will teach you, in 4 easy steps, how to read JSON data, using XMLHttp. Dec 05, 2020 - Some request methods like GET do not have a body. And some of them like POST use body to send the data to the server. We’ll see examples of that later. Listen to xhr events for response. XMLHttpRequest is a built-in browser object that allows to make HTTP requests in JavaScript. Despite of having the word "XML" in its name, it can operate on any data, not only in XML format. We can upload/download files, track progress and much more.
December 18, 2013 Javascript javascript, xhr, xmlhttprequest JoSe This example illustrates the use of XMLHttpRequest as a method to conduct basic performance monitoring. While doing so you will be introduced to the basics in sending XMLHttpRequests and how to setup event handlers for managing on-going requests. Feb 23, 2020 - By default, XHR makes an asynchronous request which is good for performance. But if you want to make an explicit synchronous request, just pass false as 3rd argument to open() method. It will pause the JavaScript execution at send() and resume when the response is available: The XMLHttpRequest method open () initializes a newly-created request, or re-initializes an existing one. Note: Calling this method for an already active request (one for which open () has already been called) is the equivalent of calling abort ().
The XMLHttpRequest object can be used to request data from a web server. The XMLHttpRequest object is a developer's dream, because you can: Update a web page without reloading the page. Request data from a server - after the page has loaded. Receive data from a server - after the page has loaded. Send data to a server - in the background. When the page loads the JS function named ShowAllReservation () is called. In this function I am making use of XMLHttpRequest (XHR) object to call the Web API: var xhttp = new XMLHttpRequest (); I am making an HTTP GET type request to the URL of my API's method which will return all these reservations. This URL is: Fetch API is a modern alternative to XHR for sending asynchronous HTTP requests in JavaScript. It also supports promises , which is another good thing to handle asynchronous requests in JavaScript. Here is how you can use the Fetch API to retrieve a list of posts from JSONPlaceholder :
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. This method is what allows us to specify the details of the request we would like to make, so let's add it next: let xhr = new XMLHttpRequest (); xhr.open ('GET', "https://ipinfo.io/json", true); The open method takes three-ish arguments: The first argument specifies which HTTP method to use to process your request. 2 weeks ago - The readyState getter steps are to return the value from the table below in the cell of the second column, from the row where the value in the cell in the first column is this’s state: ... XMLHttpRequestUpload object will result in a CORS-preflight request.
Let's get hands on with an XHR request in our browser. In the same folder as your index.html file, create a new xhr.js file. This new JavaScript file will create an XHR object and send a GET request to a JSON API. We will then log the results of the request in the console. Jul 07, 2020 - XMLHttpRequest tutorial shows how to make HTTP request in JavaScript with XMLHttpRequest. The content is handled as raw text data (since nothing here is overriding the default responseType ). var url = 'somePage.html'; function load(url, callback) { var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if ( xhr. readyState === 4) { callback( xhr. response); } } xhr.open('GET', url, true); xhr.send(''); }
XMLHttpRequest (XHR) objects are used to interact with servers. You can retrieve data from a URL without having to do a full page refresh. This enables a Web page to update just part of a page without disrupting what the user is doing. Below are the steps to make a synchronous HTTP request. Firstly, make an object of XMLHttpRequest Class. set responseType to 'text' or ' '. Create an anonymous function on onreadystatechange. Check the status and readyState are successful. On successful .. do something. For example, log the responseText to console or write it to DOM. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
XMLHttpRequest (XHR) is an API in the form of an object whose methods transfer data between a web browser and a web server. The object is provided by the browser's JavaScript environment. Particularly, retrieval of data from XHR for the purpose of continually modifying a loaded web page is ... Network requests in modern JavaScript. XHR requires quite a bit of boilerplate code. We need to construct the object, set the HTTP method every time and explicitly send the request. It also relies ... xmlhttprequest get request; javascript get search results xmlhttprequest; javascript get xmlhttprequest; XMLHTTP server; what is an xmlhttprequest; Skill assessment: JavaScript Approximately 3 questions remaining How can the responseText of an an XMLHttpRequest AJAX call be accessed without adding an explicit event listener for load or loadend ...
The request type, response type, request URL, and handler for the response data must be provided in order to make an HTTP GET request with the JavaScript XMLHttpRequest API. The URL may contain additional data in the query string. For an HTTP GET request, the request type must be GET. Send a Request To a Server. To send a request to a server, we use the open () and send () methods of the XMLHttpRequest object: xhttp. open ( "GET", "ajax_info.txt", true ); xhttp. send (); Method. Description. open ( method, url, async) Specifies the type of request. method: the type of request: GET or POST. There is a 3rd parameter to XmlHttpRequest's open(), which aims to indicate that you want the request to by asynchronous (and so handle the response through an onreadystatechangehandler). So if you want it to be synchronous (i.e. wait for the answer), just specify false for this 3rd argument.
Example Of Vanilla Javascript Fetch Post Api In Laravel 5
How To Inspect Ajax Requests With Chrome Tools
Xhr Get Requests Ii Learn Javascript Codecademy
Execute Http Requests In Javascript Applications
Xmlhttprequest Http Requests In Excel Vba Coding Is Love
Here Are The Most Popular Ways To Make An Http Request In
What Is Amp How To Use Xmlhttprequest Ajax By Bitfish
Javascript Send Http Get Post Request And Read Json Response
How To Get Xhr Request Url With Nodejs
Solution Build An Xhr Request Vanilla Javascript Ajax And Fetch
Network Features Reference Chrome Developers
Ajax Xmlhttprequest Javascript Using Post Request Detailed Part 4 Of 4
Xhr And Fetch Simple Example To Of Ajax Request Javascript
Basic Authentication With Header Javascript Xmlhttprequest
Get Request Using Ajax By Making Custom Http Library
Send An Http Get Request To A Page And Get A Result Back Js
Xhr Get Requests Ii Learn Javascript Codecademy
Xmlhttprequest Based Csrf Test 1 0
Here Are The Most Popular Ways To Make An Http Request In
Ajax Stuck On Pagination Pending In Ie Edge Urgent Help
Angular Basics How To Use Xhr Or Fetch Request In Javascript
Javascript Http Get Request Example Code Example
15 Xmlhttprequest High Performance Browser Networking Book
The Axios Vue Resource Using Vue Is Unsuccessful Across
Fetch Vs Ajax Vs Xhr Vs Axios Turbo 360
Xml Http Request Ajax Tutorial 6
New Relic Browser Monitoring Code Appears To Be Making A Xhr
0 Response to "30 Javascript Xhr Get Request"
Post a Comment