31 Javascript Function Best Practices
Declarations on Top. It is a good coding practice to put all declarations at the top of each script or function. This will: Give cleaner code; Provide a single place to look for local variables; Make it easier to avoid unwanted (implied) global variables; Reduce the possibility of unwanted re-declarations Encapsulate your code inside anonymous function and execute the function immediately: (function () { // Do stuff here } ()); When function is executed immediately after declaration wrapp it into parens. Use this code convention to indicate immediate invokation.
Javascript And Jquery Best Practices
May 24, 2020 - Best ways to define functions.. “JavaScript Best Practices — Function Length and Parameters” is published by John Au-Yeung in The Startup.
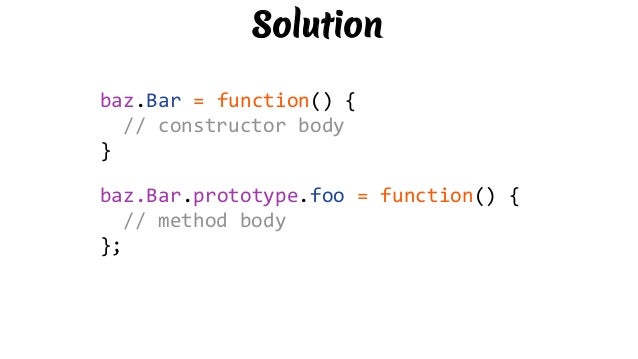
Javascript function best practices. When functions are executed in JavaScript, a set of first order variables including the immediate scope chain, the arguments of the function and any locally-declared variables are instantiated. Therefore, it takes time to climb up the scope chain when you try to access a globally-declared variable. By Saad Mousliki. Updated May 15th, 2020. As you know, JavaScript is the number one programming language in the world, the language of the web, of mobile hybrid apps (like PhoneGap or Appcelerator), of the server side (like NodeJS or Wakanda) and has many other implementations.It's also the starting point for many new developers to the world of programming, as it can be used to display a ... Arrow functions provide a cleaner syntax for declaring anonymous functions (lambdas), dropping the function keyword and the return keyword when the body function only has one expression. This can...
Apr 01, 2019 - Developers who have spent any time on large projects understand the importance of code comments. When you're building many features into the same Cleaning up our JavaScript code is easy with default parameters and property shorthands. In this article, we'll look at the best practices when designing functions. This omission is intentional. While I've made passing references to both the browser and Visual Studio, the above tips are purely about JavaScript. I will be writing a separate article that covers JavaScript in the browser. Thus concludes my attempt at compiling a list of JavaScript best practices.
Apr 29, 2020 - Put all the declarations on top of the script or a function to get cleaner code. You don’t want to have declarations all over the code. And you want to reduce the possibility or re-declarations. ... Best practice would also be to initialize variables when you declare them. Jul 07, 2020 - JavaScript is a beloved and widespread language, but it can be unruly and hard to read. Today, we'll go over 15 tips to simplify your code and make dev life much easier. Dec 01, 2020 - Even if you’re writing a small function, or writing an application: you always declare, assign, and read variables. Writing quality variables brings increased readability and easier maintainability of your code. In this post, you’ll read 5 best practices of how to declare and use variables in JavaScript...
24/11/2016 · OK, so the first best practice: Prefer separate named parameters for your function. Additionally, separate parameters also help if working in a functional programming style. One common thing in FP is to partially apply your functions, which is much harder to do if your function takes its parameters as an object. Objects are good for optionals 25/8/2021 · Photo by Sebastian Willius on Unsplash. You are here for facts and best practices. So, no time-wasting intro. Let’s go! 1. Injection — A Magically Sorting Inventory. We are going to start with an example of passing functions as parameters provided by the Array.prototype.sort() method.. Imagine you have an array of strings representing an inventory, and you want to sort it alphabetically. JavaScript functions - Exercises, Practice, Solution Last update on February 26 2020 08:09:06 (UTC/GMT +8 hours) JavaScript functions [29 exercises with solution] [An editor is available at the bottom of the page to write and execute the scripts.] 1. Write a JavaScript function that reverse a number.
This is an example from out Page object: Page.initialLoad = function() { //abstract alert('Page.initialLoad not implemented');}; In java world it is analagous to : public void abstract initialLoad(); The Java code gives a compile timeerror, however in the Javascript we would get a runtime error. Modularize — one function per task This is a general programming best practice — making sure that you create functions that fulfill one job at a time makes it easy for other developers to debug and change your code without having to scan through all the code to work out what code block performs what function. Top ↑ Functions # Functions. Functions should be formatted as follows: Summary: A brief, one line explanation of the purpose of the function. Use a period at the end. Description: A supplement to the summary, providing a more detailed description. Use a period at the end. @deprecated x.x.x: Only use for deprecated functions, and provide the version the function was deprecated which should ...
Callbacks are one of the critical elements to understand JavaScript and Node.js. Nearly, all the asynchronous functions use a callback (or promises). In this post, we are going to cover callbacks in-depth and best practices. This post assumes you know the difference between synchronous and asynchronous code. JavaScript is an event-driven language. Spread the love Related Posts Why JavaScript Arrow Functions are Useful?Since ES6, there are two types of general-purpose functions. One is the traditional function declared… JavaScript Best Practices — Designing FunctionsCleaning up our JavaScript code is easy with default parameters and property shorthands. In this… Using Arrow Functions in JavaScriptArrow functions are a new type of ... Pragmatic Standards: JavaScript ... Best Practices for Speeding Up Your Site, by the Yahoo! Developer Network Constructors considered mildly confusing, by Joost Diepenmaat jQuery API If You're A JavaScript Ninja, Read These: The Surprisingly Elegant JavaScript Type Model, by Kannan Vijayan JavaScript Object vs Function experiment, ...
The JavaScript modules are great to split the logic of your application into small, self-contained chunks. By using named exports instead of default exports, you could benefit from easier renaming refactoring and editor autocomplete assistance when importing the named component. JavaScript best practices: function; In JavaScript function is a central working unit. It's very important to understand what it is and how it works due to the fact that almost every script uses one or more of them. So, to be more specific, function is a named sections of a program (a "subprogram") that performs a specific task. The best way to do function overloading with parameters is not to check the argument length or the types; checking the types will just make your code slow and you have the fun of Arrays, nulls, Objects, etc. What most developers do is tack on an object as the last argument to their methods. This object can hold anything.
Your JavaScript can work well without the use of "eval" function. For those not aware, "eval" gives access to JavaScript compiler. If a string is passed as parameter of "eval" then its result can be executed. This will degrade your code's performance though it acts as a boon during development phase. JavaScript Clean Coding Best Practices. András Tóth. Full-Stack Developer at RisingStack. Writing clean code is what you must know and do in order to call yourself a professional developer. There is no reasonable excuse for doing anything less than your best. "Even bad code can function. Feb 29, 2020 - Now we can understand the code easily. The function itself becomes the comment. Such code is called self-descriptive.
Jun 29, 2021 - If you have JS files whose only purpose is to add functionality—for example, after a button is clicked—go ahead and place those files at the bottom, just before the closing body tag. This is absolutely a best practice. ... <p>And now you know my favorite kinds of corn. </p> <script type="text/javascript... An Immediately Invoked Function Expression (IIFE) is an anonymous function (a function with no name) that is turned into an expression by wrapping it with parenthesis and then immediately invoked with another set of parenthesis. This syntax is very common in JavaScript and is used to create a scope that doesn't conflict with other scopes. NOTE: In this article, we will look at how to comment JavaScript code, as which types of comments exist, and some best practices. Single-Line Comments. Single-line comments are generally used to comment a part of the line or full line of code. Single-line comments in JavaScript start with //. The interpreter will ignore everything to the right of ...
An immediately-invoked function expression is a pattern which produces a lexical scope using JavaScript's function scoping. Immediately-invoked function expressions can be used to avoid variable hoisting from within blocks, protect against polluting the global environment and simultaneously allow public access to methods while retaining privacy ... Super JavaScript Tips and Best Practices Determine JavaScript Version. It could make you curious to think which version of JavaScript your browser is using. And it is always good to know about the exact environment you are running the scripts. So use the below HTML code. Copy-paste it in a file and save as version_detect.HTML. In the preceding example, the syntactic levels from highest to lowest are as follows: assignment, division, function call, parameters, number constant. ... When a line is broken at an operator the break comes after the symbol. (Note that this is not the same practice used in Google style for Java.)
Oct 31, 2018 - Coding Best Practices (JavaScript) - Write small functions. The idea is simple. Do not write long functions. Do not write functions longer than 20 lines.. Jun 01, 2018 - But if you see let and const in the intializations, arrow functions in callbacks, and classes as the basis for object-oriented patterns, you’ll probably also see other modern JavaScript code in the examples. The best practice in modern JavaScript is paying attention to what the language is ... If you debug using comments, there is a nice little trick: module = function () { var current = null ; return {init:init, show:show, current:current} } (); Comments should never go out to the end user in plain HTML or JavaScript. See Development code is not live code.
JavaScript exercises and projects with solutions PDF. Compilation of free resources to practice JavaScript. Exercises for basic, intermediate and advanced level developers. JavaScript university assignments. JavaScript arrays, strings, operators, functions objects practice. To make debugging productive, a good practice is to use variables to hold the arrow functions. This allows JavaScript to infer the function names. An inline arrow function is handy when the function body has one expression. The operators >, <, <= and >= look similar to the fat arrow =>. 10/6/2020 · JavaScript functions are written in camelCase too, it’s a best practice to actually tell what the function is doing by giving the function name a verb as prefix This verb as prefix can be anything (e.g., get, push, apply, calculate, compute, post) JavaScript Naming Conventions: Class
JavaScript Coding Conventions. Coding conventions are style guidelines for programming. They typically cover: Naming and declaration rules for variables and functions. Rules for the use of white space, indentation, and comments. Programming practices and principles; Coding conventions secure quality: Improves code readability; Make code ...
Ten Useful Javascript Tips Amp Best Practices
Javascript Development Best Practices Thecodebuzz
15 Javascript Tips Best Practices To Simplify Your Code
The 10 Most Common Mistakes Javascript Developers Make Toptal
Automating At Scale With Vro And Vra Vmware Cloud Management
15 React Best Practices You Need To Follow In 2021
Nodejs Coding Standards And Best Practices Node Js
Javascript Clean Coding Best Practices By Eduard
Javascript Best Practices Function Javascript Coding
A Set Of Best Practices For Javascript Projects Full Stack Feed
Ten Useful Javascript Tips Amp Best Practices
Javascript Best Practices Speaker Deck
Js Best Practices Viking Code School
Write More Robust Javascript 7 Best Practices Jsmanifest
Javascript Concepts Amp Best Practices By Rohan Fernando
Deeply Understanding Javascript Async And Await With Examples
10 Best Practices To Containerize Node Js Web Applications
Javascript Debugging Best Practices By Grootech Bits And
Javascript Best Practices Cloudapp Cloudapp Blog
Object Oriented Javascript For Beginners Learn Web
Javascript Best Practices Function Length And Parameters
Best Practices To Measure Execution Time In Javascript
Javascript Best Practices Cloudapp Cloudapp Blog
Javascript Best Practices Function Layout And Documentation
Five Best Practices For Increasing Code Readability In
Best Practices For Writing Good Clean Javascript Code
Best Practices For Writing Azure Functions Building
50 Useful Javascript Tips Tricks And Best Practices Jpswade
0 Response to "31 Javascript Function Best Practices"
Post a Comment