27 Define Object Array In Javascript
The Array in JavaScript is a global object which contains a list of items. It is similar to any variable, in that you can use it to hold any type of data. However, it has one important difference: it can hold more than one item of data at a time. A list. Objects, as generic blobs of values, can be used to build all sorts of data structures. A common data structure is the list (not to be confused with array). A list is a nested set of objects, with the first object holding a reference to the second, the second to the third, and so on.
Javascript Lesson 26 Nested Array Object In Javascript
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person[0] returns John:
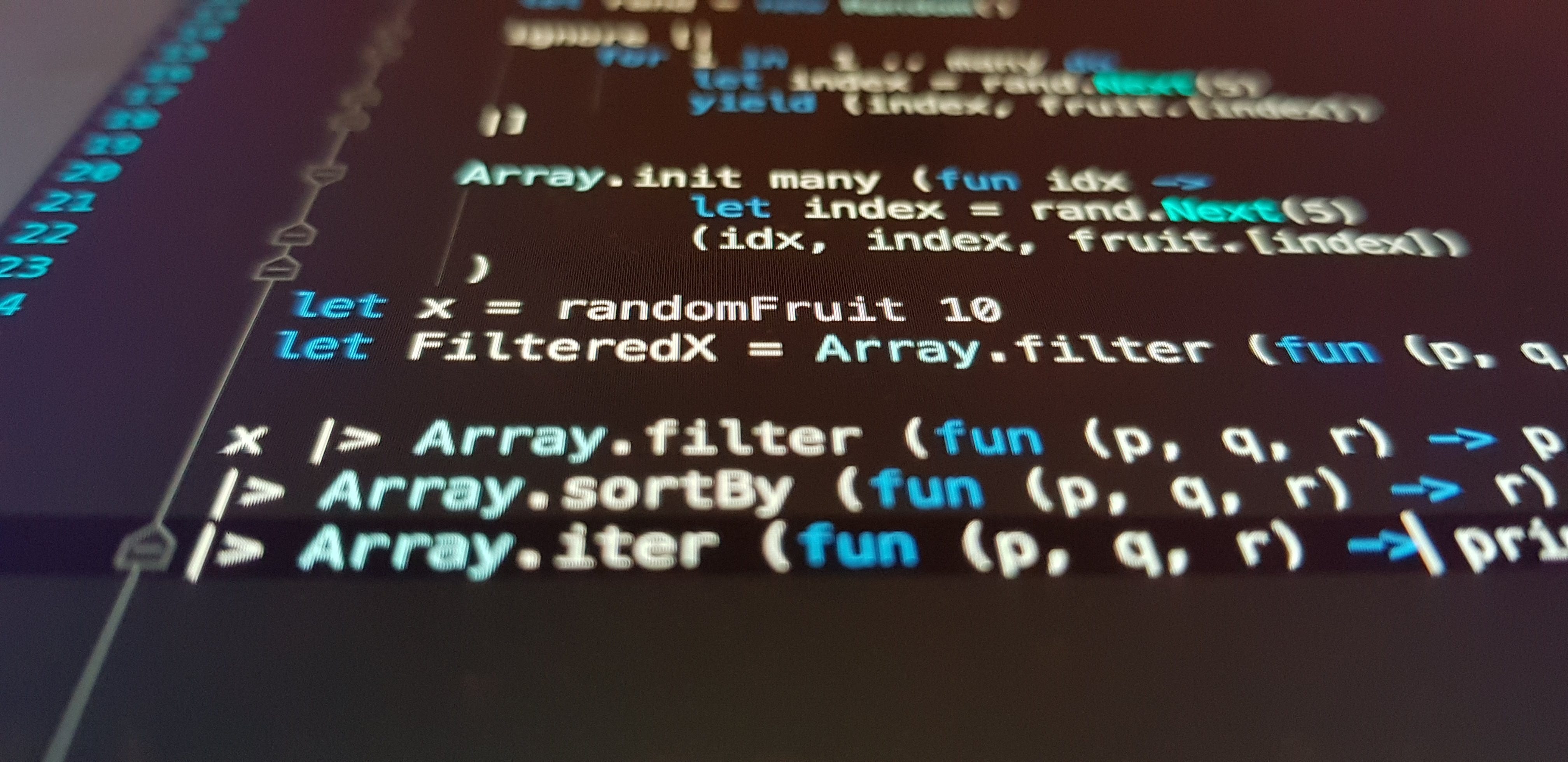
Define object array in javascript. JavaScript support various types of array, string array is one of them. String array is nothing but it's all about the array of the string. The array is a variable that stores the multiple values of similar types. In the context of a string array, it stores the string value only. I have a variable which is an array and I want every element of the array to act as an object by default. To achieve this, I can do something like this in my code. var sample = new Array(); sample[0] = new Object(); sample[1] = new Object(); This works fine, but I don't want to mention any index number. I want all elements of my array to be an ... An array in JavaScript is a type of global object used to store data. Arrays can store multiple values in a single variable, which can condense and organize our code. JavaScript provides many built-in methods to work with arrays, including mutator, accessor, and iteration methods.
Loosely speaking, objects in JavaScript may be defined as an unordered collection of related data, of primitive or reference types, in the form of "key: value" pairs. These keys can be variables or functions and are called properties and methods, respectively, in the context of an object. An array is a special kind of object. The square brackets used to access a property arr actually come from the object syntax. That's essentially the same as obj [key], where arr is the object, while numbers are used as keys. They extend objects providing special methods to work with ordered collections of data and also the length property. Method 3: unshift () The unshift () function is one more built-in array method of JavaScript. It is used to add the objects or elements in the array. Unlike the push () method, it adds the elements at the beginning of the array. " Note that you can add any number of elements in an array using the unshift () method."
Difference between objects and arrays. Despite assignment and access methods being quite similar, arrays and objects have a set of differences. Objects are used in JavaScript when defining anything that is made up of characteristics. Arrays are used when creating a list of multiple items in a single variable. The Difference Between Array() and []¶ Using Array literal notation if you put a number in the square brackets it will return the number while using new Array() if you pass a number to the constructor, you will get an array of that length.. you call the Array() constructor with two or more arguments, the arguments will create the array elements. If you only invoke one argument, the argument ... Object.keys (o): The object.keys (o) method is used to traverse an object o and returns an array containing all enumerable property names. Object.getOwnPropertyNames (o): A lesser known method, it iterates over an object o and returns an array of all natural property names of the object, enumerable or not.
In JavaScript, array is a single variable that is used to store different elements. It is often used when we want to store list of elements and access them by a single variable. Unlike most languages where array is a reference to the multiple variable, in JavaScript array is a single variable that stores multiple elements. Declaration of an Array JavaScript array is an object that represents the collection of similar types of items. JavaScript arrays are used to save multiple values in a single variable. The array is a single variable that is used to store different elements. It is often used when we want to store a list of elements and access them by a single variable. 27/12/2019 · Here, the parameter arrow function checks if the id and name of the object obj is equal to the current object or not. If yes, it adds it to the final array. If no item is found, the result array will hold nothing. Using some() : some() method takes one function as the parameter and returns one boolean. true if atleast one element passes the function.
15/3/2018 · Due to the fact that your property is an array of objects (e.g., [ {...}, {...}] ), what you may want to do is iterate over that array and freeze those objects: obj.property.forEach (o=>Object.freeze (o)) obj.property [0].value=33; // when frozen you won't be able to update the value. Share. JavaScript - Objects Overview. JavaScript is an Object Oriented Programming (OOP) language. A programming language can be called object-oriented if it provides four basic capabilities to developers −. Encapsulation − the capability to store related information, whether data or methods, together in an object. Objects in JavaScript are standalone entities that can be likened to objects in real life. For example, a book might be an object which you would describe by the title, author, number of pages, and genre. Similarly, a car might be an object that you would describe by the color, make, model, and horsepower.
The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Description. Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types of its elements are fixed. Convert object to array in Javascript Javascript, being a broad and varied-natured programming language consists of an ample amount of features to convert an object into an array. These methods are predefined in the language itself. Let's look at the different ways of converting objects to arrays in javascript. JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects ...
In JavaScript, an array is another object you use to hold values. Instead of assigning and calling properties, an array lets you call values using an index. An index describes the location of a value stored in memory. The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type. An array in JavaScript can be defined and initialized in two ways, array literal and Array constructor syntax. Array Literal. ... As you can see in the above syntax, an array can be initialized using new keyword, in the same way as an object. The following example shows how to define an array using Array constructor syntax.
Node.js Tutorial - Node.js Arrays. To create arrays, you can either use traditional notation or array literal syntax : As with objects, the literal syntax version is preferred. We can test if an object is an array using the Array.isArray function: We can create arrays quite easily in JavaScript using []. Arrays are data structures that store information in a set of adjacent memory addresses. In practice, this means is that you can store other variables and objects inside an array and can retrieve them from the array by referring to their position numberin the array. Each variable or object in an 14/5/2020 · Add a new object at the end - Array.push. To add an object at the last position, use Array.push. let car = { "color": "red", "type": "cabrio", "registration": new Date('2016-05-02'), "capacity": 2 } cars.push(car); Add a new object in the middle - Array.splice. To add an object in the middle, use Array.splice. This function is very handy as it can also remove items.
The syntax of JavaScript map method is as below : let finalArray = arr.map(callback( currentValue[, index[, array]]), arg) Following are the meanings of these parameters : callback: This is the callback method. It is called for each of the array arr elements. The returned value of this method is added to the final array finalArray. In JavaScript, functions are objects. You can work with functions as if they were objects. For example, you can assign functions to variables, to array elements, and to other objects. They can also be passed around as arguments to other functions or be returned from those functions. Objects in JavaScript are dynamic collections of key-value pairs. The key is always a string and has to be unique in the collection. The value can a primitive, an object, or even a function. We can access a property using the dot or the square notation.
All Languages >> Javascript >> Next.js >> array object define javascript “array object define javascript” Code Answer’s. array of objects javascript . javascript by 2 Programmers 1 Bug on Mar 05 2020 Donate Comment ... The this Keyword. In a function definition, this refers to the "owner" of the function. In the example above, this is the person object that "owns" the fullName function. In other words, this.firstName means the firstName property of this object. Read more about the this keyword at JS this Keyword.
Why You Should Use An Object And Not An Array For Lists In
Different Ways To Create Arrays In Javascript Time To Hack
How To Use Map Filter And Reduce In Javascript
Using The Map Function In Javascript And React By Jonathan
How To Check If Array Includes A Value In Javascript
Javascript Array Sort Sorting An Array More Effectively
Hacks For Creating Javascript Arrays
Can T Access Object Property Even Though It Shows Up In A
Traverse Array Object Using Javascript Javatpoint
Why Do Arrays Work As Objects In Javascript Quora
Rewriting Javascript Converting An Array Of Objects To An
Dynamic Array In Javascript Using An Array Literal And
Javascript Array Distinct Ever Wanted To Get Distinct
Javascript Lesson 26 Nested Array Object In Javascript
How To Add An Object To An Array In Javascript Geeksforgeeks
How To Create Own Custom Array Implementation In Python
Javascript Array Of Objects Tutorial How To Create Update
Javascript Sort Array Of Objects Ascending And Descending
How To Create Array Of Objects In Java Geeksforgeeks
Javascript Array Push How To Add Element In Array
Object Oriented Javascript For Beginners Learn Web
Javascript Typed Arrays Javascript Mdn
Hacks For Creating Javascript Arrays
Creating A List Of Objects Inside A Javascript Object Stack
How To Create Array Of Objects In Java Javatpoint
0 Response to "27 Define Object Array In Javascript"
Post a Comment