20 Convert Object To Array Javascript Es6
# Converting Object to an Array. Finally, with ES2017, it's official now! We have 3 variations to convert an Object to an Array 🎊. Array has an array of methods (sorry, bad pun 😝). So by converting the object into an array, you have access to all of that. Woohoo 🥳 Sort an array of objects in JavaScript dynamically. Learn how to use Array.prototype.sort() and a custom compare function, and avoid the need for a library.
How To Remove Array Duplicates In Es6 By Samantha Ming
This is what's called grapheme clusters - where the user perceives it as 1 single unit, but under the hood, it's in fact made up of multiple units. The newer methods spread and Array.from are better equipped to handle these and will split your string by grapheme clusters 👍 # A caveat about Object.assign ⚠️ One thing to note Object.assign is that it doesn't actually produce a pure array.
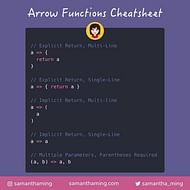
Convert object to array javascript es6. Jun 12, 2020 - Get code examples like "convert object to array using es6" instantly right from your google search results with the Grepper Chrome Extension. Hi, in this tutorial, we are going to talk about how we can convert the Array to String using array methods in Javascript ES6 with example.. Array to String in Javascript. There are many different ways to convert the Array to String for example like JSON.stringify() method, which is quite a popular method when you want to contents of an array to be used further later then you can use this ... ES6: Using Object.keys() to convert Object to array in JavaScript. ECMAScript 2015 or ES6 introduced Object.keys() method to fetch the key data (property)of an object. In the below example, we will fetch the property names of the object "Book". In this way, we can convert an object to an array.
You can use the Array.from method, which is being added in ES6, but only supports arrays and iterable objects like Maps and Sets (also coming in ES6). For regular objects, you can use Underscore's toArray method or lodash's toArray method, since both libraries actually have great support for objects, not just arrays. If you are already using underscore or lodash, then luckily they can handle ... In this tutorial, we are going to learn about three different ways to convert an array into an object in JavaScript. Es6 spread operator (…) We can use the es6 spread operator us to convert an array into an object. Example: The map() method creates a new array populated with the results of calling a provided function on every element in the calling array.
Sort an Array of Objects in JavaScript Summary : in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. Convert object to array javascript - Một câu hỏi tuy đơn giản nhưng đây là một câu hỏi với số lượng search trên google tại Việt Nam lên đến gần 300 lượt mỗi tháng. Điều đó có nghĩa là nhu cầu sử dụng array trong javascript rất nhiều. Vì thế, tipjs sẽ giới thiệu một số cách chuyển từ object sang array javascript. "convert array to object es6" Code Answer. convert array to object in javascript . javascript by Naughty Nightingale on Jul 30 2020 Comment . 4 Source: stackoverflow . Add a Grepper Answer . Javascript answers related to "convert array to object es6" ...
How to convert an array of objects to an object in JavaScript. The quickest way to convert an array of objects to a single object with all key-value pairs is by using the Object.assign () method along with spread operator syntax ( ... ). The Object.assign () method was introduced in ES6 (ESMAScript 2015), and it copies all enumerable own ... 1 week ago - The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. to convert a plain object into an ES6 MapES6convert object to map:const objToMap = (o) => new Map(Object.entries(o));convert map to object:const mapToObj = ... Do I really have to first convert it into an array of arrays of key-value pairs? ... Categories Javascript Post navigation.
Converting Object to an Array, With this in mind it becomes important to understand how to convert our data into our desired format. The Example. Using newer Javascript ES6: Using Object.keys () to convert Object to array in JavaScript ECMAScript 2015 or ES6 introduced Object.keys () method ... we have two objects here and I am going to use 2 methods for assign both objects into Array. Object.entries() Object.keys() 1st one starts here-----var firstar = Object.entries(address); here I assigned address into firstar and when you will run this you will get output like this (3) [Array(2), Array(2), Array(2)] 2nd one starts here In this tutorial, we are going to learn about three different ways to convert an object into an array in JavaScript. Using the Object.keys() method. The Object.keys() takes the object as an argument and returns the Object properties in an array. Example:
ES6 is a new generation of Javascript that will make writing cleaner and powerful code easier than ever before. This blog post should set you on your path to converting ES5 to ES6. So what is ES6? ECMAScript 6 (ES6) was the second major revision to Javascript in 2015, which simplifies the code and enables us to write less and do more. Nov 19, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. JavaScript ES6 introduced, among many other things, the spread operator (...), which allows an iterable to be expanded in places where zero or more arguments or elements are expected. We can use the spread operator to convert iterables or, as they are sometimes referred to, array-likes. Let's take a look at some examples: String
4 weeks ago - The Object.keys() method returns an array of a given object's own enumerable property names, iterated in the same order that a normal loop would. Converting an array-like object into an array using the spread operator (which was introduced in ES6) only works on objects that are iterable. To demonstrate this, let's consider the following example with an array-like object that does not implement the iterable protocol : How to convert array of objects to Map in javascript. array of objects are enclosed in square bracket -[ array of objects] Many ways we can convert array objects to map. ES6 map constructor with Array map method. Array objects are iterated using array map() method with call back. map method calls for every objects, finally returns new map ...
Oct 26, 2020 - Objects... to array ... The goal of the function is to convert an object into an array, where each element in the array represents a key:value pair This Array.from() is another factory method which is offered by Javascript in ES6 through which we can create array. For this method, we need to pass an iterable or array-like object as the argument and then it will return. Array.from () lets you convert an "iterable" object (AKA an array-like object) to an array. In this lesson, we go over grabbing DOM nodes and turing them into an array so that we can use methods like Array.filter () and Array.forEach () on them. [00:00] Here we have a list of prices that belong to products inside of a store.
The iterable argument is expected to be an object that implements an @@iterator method, that returns an iterator object, that produces a two element array-like object, whose first element is a value that will be used as a property key, and whose second element is the value to associate with ... The target object is the first argument and is also used as the return value. The following example demonstrates how you can use the Object.assign () method to convert an array to an object: const names = ['Alex', 'Bob', 'Johny', 'Atta']; const obj = Object.assign({}, names); console.log( obj); Take a look at this guide to learn more about the ... The Object.fromEntries() method takes a list of key-value pairs and returns a new object whose properties are given by those entries. The iterable argument is expected to be an object that implements an @@iterator method, that returns an iterator object, that produces a two element array-like object, whose first element is a value that will be used as a property key, and whose second element ...
Array.from() lets you create Arrays from: array-like objects (objects with a length property and indexed elements); or ; iterable objects (objects such as Map and Set).; Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map ... Jul 27, 2021 - Object.keys(obj) – returns an array of keys. 1 week ago - The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Rewriting JavaScript: Converting an Array of Objects to an Object. ... So it may be best to convert that data on the fly. Luckily ES6 provides us a way to make this conversion. Finally with ES2017, it's official now! We have 3 variations to convert an Object to an Array in JavaScript Jun 12, 2020 - Write a function that converts an object into an array, where each element represents a key-value pair in the form of an array.
JavaScript fundamental (ES6 Syntax): Exercise-5 with Solution. Write a JavaScript program to convert an array of objects to a comma-separated values (CSV) string that contains only the columns specified. Use Array.prototype.join(delimiter) to combine all the names in columns to create the first row. The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop. (The only difference is that a for...in loop enumerates properties in the prototype chain as well.) Convert object to array in Javascript. Javascript, being a broad and varied-natured programming language consists of an ample amount of features to convert an object into an array. These methods are predefined in the language itself. Let's look at the different ways of converting objects to arrays in javascript.
The spread operator in ES6 is used to clone an array, whereas slice() method in JavaScript is an older way that provide 0 as the first argument. These methods create a new, independent array and copy all the elements of oldArray to the new one i.e. both these methods do a shallow copy of the original array. We can convert an Object {} to an Array [] of key-value pairs using methods discussed below: Method 1: In this method, we will use Object.keys () and map () to achieve this. Approach: By using Object.keys (), we are extracting keys from the Object then this key passed to map () function which maps the key and corresponding value as an array, as ... To convert an object to an array you use one of three methods: Object.keys (), Object.values (), and Object.entries (). Note that the Object.keys () method has been available since ECMAScript 2015 or ES6, and the Object.values () and Object.entries () have been available since ECMAScript 2017. Suppose that you have a person object as follows:
How To Convert Set To Array In Javascript Geeksforgeeks
How Do I Convert Array Of Objects Into One Object In
How To Loop Through Array Of Objects In Javascript Es6 Reactgo
How To Add An Object To An Array In Javascript Geeksforgeeks
Javascript Arrays And Es5 Es6 Amp Es7 Methods You Should Know
Hacks For Creating Javascript Arrays
Useful Javascript Array And Object Methods By Robert Cooper
Js Array From An Array Like Object Dzone Web Dev
Es6 Javascript How To Merge An Object On A Specific Key
Es6 Keyed Collections Maps And Sets Logrocket Blog
Es6 Destructuring An Elegant Way Of Extracting Data From
How To Convert An Object To An Array In Javascript
Converting An Array Like Object Into An Array With Array From
How To Convert Arguments Object Into An Array In Javascript
Converting Object To An Array Samanthaming Com
Convert Object To Array In Js Code Example
Converting Object To An Array In Javascript Dev Community
Convert Javascript Array To Object Of Same Keys Values
Convert Object To Array Javascript Js Example Codez Up
0 Response to "20 Convert Object To Array Javascript Es6"
Post a Comment