30 Javascript Countdown Timer 60 Seconds
var countdownTimer = setInterval ('secondPassed ()', 1000); </script>. For all of this to be possible, we need to use the "setInterval" function in JavaScript. You need to pass in our "secondPassed" function and the number of milliseconds into it (1000 milliseconds = 1 second). We set our "setInterval" function and the "seconds" variables in ... A function to run, or a reference to a function defined elsewhere. A number representing the time interval in milliseconds (1000 milliseconds equals 1 second) to wait before executing the code. If you specify a value of 0 (or omit the value), the function will run as soon as possible. (See the note below on why it runs "as soon as possible" and ...
How To Build A Countdown Timer In Pure Javascript Developer
Step 2. Now go to the Solution Explorer to the right side of the application and use the following procedure as in the following figure. Step 3. Add a new Web form in the empty web application as in the following figure. Write the following code in the Timer.aspx page: Step 4.
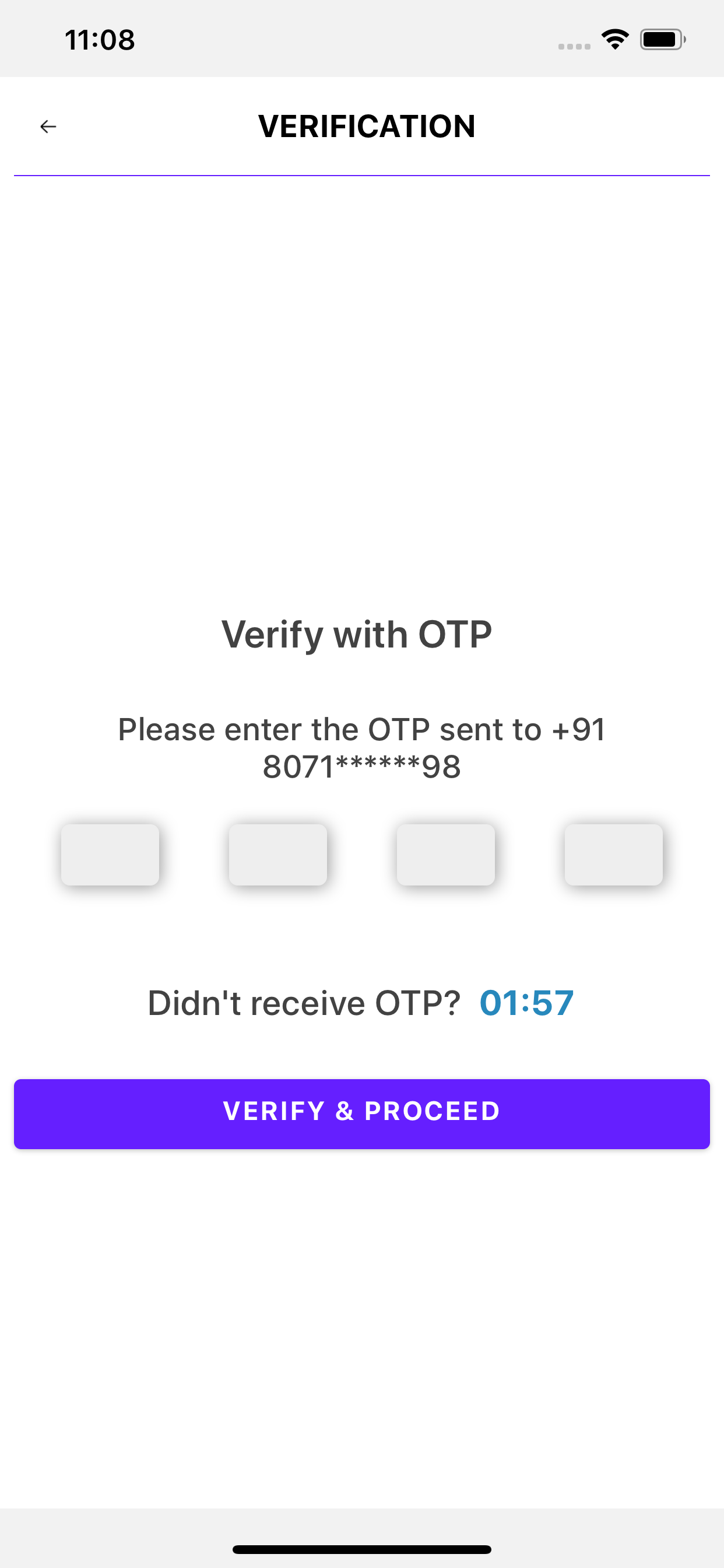
Javascript countdown timer 60 seconds. 4/11/2017 · Replace 10 with the seconds you want to count down from --> <span id="countdown">10</span> The JavaScript: var seconds = document.getElementById("countdown").textContent; var countdown = setInterval(function() { seconds--; document.getElementById("countdown").textContent = seconds; if (seconds <= 0) clearInterval(countdown… Feb 14, 2019 - You can find the entire JavaScript code at the end of the article. ... Let’s start with the HTML. The countdown timer will display four figures: days, hours, minutes, and seconds. Create a <span> element for each so that later you can target them with JavaScript. The project creates a JavaScript countdown timer. This timer takes a given day and returns the days, hours, minutes, and seconds.
JavaScript function. The Timer () displays the countdown in appropriate position and displays different messages when the time is reduced to certain point. In this code, when the seconds are less than 20, the countdown is displayed in orange color with a message. When the seconds are less than 10, its displayed in red and a message being displayed. Using packages here is powered ... native JavaScript ES6 import usage. All packages are different, so refer to their docs for how they work. If you're using React / ReactDOM, make sure to turn on Babel for the JSX processing. ... If active, Pens will autosave every 30 seconds after being ... May 08, 2020 - Get code examples like "basic count down 60 sec timer javascript" instantly right from your google search results with the Grepper Chrome Extension.
Howdy folks, In this tutorial, you will learn how to build a Javascript countdown timer. Our countdown timer will be in the format hours + ':' + minutes + ':' + seconds. The user will be able to set the countdown time in hours, minutes, or seconds as he/she likes. Then also there will be buttons to stop and reset the countdown timer. Simple Pure Javascript Countdown Timer - Free Code Download By W.S. Toh / Tips & Tutorials - Javascript / December 20, 2020 January 28, 2021 Welcome to a tutorial and example on how to create a simple Javascript Countdown Timer.
When your time runs out and the countdown timer ends, the remaining message will be displayed. The time remaining on your countdown timer is all set. The remaining time (the difference) is calculated in milliseconds. Now you need to format the time remaining into days, hours, minutes, and seconds. // Update the count down every 1 second var x = setInterval(function() { // Get today's date and time var now = new Date().getTime(); // Find the distance between now and the count down date var distance = countDownDate - now; // Time calculations for days, hours, minutes and seconds var days = Math.floor(distance / (1000 * 60 * 60 * 24)); Js realizes 60 seconds countdown after sending SMS verification code ... To make multiple clicks, it can only be used once within 60 seconds, and multiple timers cannot be turned on.
First, create a project folder called countdown-timer. Inside that folder, create three subfolders: js, css, and img that will store the JavaScript, CSS, and image files. Second, create the style.css in the css folder, app.js and countdown.js files in the js folder, and the index.html in the countdown-timer folder: Third, download this firework ... Nov 04, 2019 - *This article has been updated and migrated to Adrienne’s blog. You can find the full, FREE article there as well as the latest blog posts, updates, and tutorials from Adrienne. See you there! Step 1: Start with the basic markup and styles. Let's start with creating a basic template for our timer. We will add an svg with a circle element inside to draw a timer ring that will indicate the passing time and add a span to show the remaining time value. Note that we're writing the HTML in JavaScript and injecting into the DOM by ...
How would I do // Update the count ... / (1000 * 60 * 60 * 24)); How To Create a Countdown Timer, How TO - JavaScript Countdown Timer. ❮ Previous Display the countdown timer in an element --> Time calculations for days, hours, minutes and seconds TIL how to create a seconds ... EasyTimer.js is a simple library for counting time in five different units: tenth of seconds, seconds, minutes, hours and days. It has interesting features: The refresh interval can be configured in tenth of seconds, seconds, minutes and hours. Dispatches events when timer starts, stops, pauses and when every type of unit changes. Aug 07, 2010 - The domain codingforums may be for sale. Please send an inquiry to info@first1
javascript - Make a countdown from timer - Stack Overflow. Is there any way to make a countdown with 60 seconds... Here is the code for timer:var count = 0;var timer = $.timer(function() { $('#counter').html(++count);});timer.set({ time : 1000, Stack Overflow. Setelah mengetahui apa itu countdown timer beserta manfaatnya, sekarang mari kita pelajari bagaimana cara memasangnya di website dengan menggunakan JavaScript.. Kabar baiknya, JavaScript menawarkan struktur kode yang sederhana, ringan, dan tak mengharuskan Anda memuat scripts maupun stylesheets eksternal—sehingga menjaga performa serta kecepatan situs Anda secara optimal. 29/6/2015 · A solution using Promises, includes both progress bar & text countdown. ProgressCountdown(10, 'pageBeginCountdown', 'pageBeginCountdownText').then(value => alert(`Page has started: …
In the first code snippet the countdown timer will countdown to a specific date that you set in the Javascript code. Once the date is reached the visitor is redirected. In the second snippet the countdown timer countdown from a specific amount of time. Once the timer ends the visitor will be redirected. There are also a variety of ways you can ... As this jQuery library file contains the javascript functions so we need to call the function within <script> </script> tag. Now after including the file, we need to define a variable which will store that for how long you want the timer on the page(c=60) and now the time you set needs to be changed in hours , minutes and seconds ... May 23, 2017 - I'm trying to countdown 60 seconds once I click the begin button and at 0 I don't want to just hide the counter, I want it to be reset. I ran into 2 problems: When I click the begin button again it...
1/6/2020 · Divide the total seconds by 60 and grab the remainder. You don’t want all of the seconds, just those remaining after the minutes have been counted: (t/1000) % 60 Round this down to the nearest ... The time interval is divided by 1000 to determine the number of seconds, i.e. timeLeft / 1000; The time interval then is divided by 60 * 60 * 24 to determine the number of days remaining. The Math.floor() function is used to round the number to a whole number. Similar methods are used for hours, minutes, and seconds. The time interval in which you want countdown to tick. Set the interval in seconds. Note that you should only use intervals that are multiples of a minute (60) and for only seconds timer, in the multiples of the seconds set for timer. By default it is 1. timeUp. The name of the callback function that is invoked when the countdown reaches zero.
Dec 30, 2014 - If I want to want finish/submit Quiz test after 60 seconds automatically, set a countDownTime = 60. While Quiz starts that countDownTimer() function is called, then countDownTime is minused by one. This minused countDownTime is converted into hour:minute:seconds format using following script. 1. Create the HTML. Let's start with the HTML. The countdown timer will display four figures: days, hours, minutes, and seconds. Create a < span> element for each so that later you can target them with JavaScript. Also add a < script> tag with the path to the JavaScript file right before the closing < body> tag. 60 Seconds Timer - Online Stopwatch. A cool little 60 Seconds Timer! Simple to use, no settings, just click start for a countdown timer of 60 Seconds. Try the Fullscreen button in classrooms and meetings :-) Countdown Timer - HTML5. www.online-stopwatch .
A countdown timer is an accurate timer that can be used for a website or blog to display the count down to any special event, such as a birthday or anniversary. Basics of a countdown timer are : Set a valid end date. Calculate the time remaining. Convert the time to a usable format. Output the clock data as a reusable object. Using packages here is powered ... native JavaScript ES6 import usage. All packages are different, so refer to their docs for how they work. If you're using React / ReactDOM, make sure to turn on Babel for the JSX processing. ... If active, Pens will autosave every 30 seconds after being ... Building a simple countdown timer is easy with JavaScript's native timing events. You can read more about those in this article. Building the countdown timerStart by declaring an empty function called startCountdown that takes seconds as an argument: function startCountdown(seconds) { };We want to keep track of the seconds that
JavaScript code that set the timer of 2 minutes and when the times up the Page alert "times up". The setTimeout() method calls a function or evaluates an expression after a specified number of milliseconds. JavaScript code to implement the timer: Now create a CSS file named ' style.css ' and put these codes. The final step, Create a JavaScript file named ' function.js ' and put the codes. That's It. Now you have successfully created JavaScript Countdown Timer With CSS UI, A Pure JS Countdown Clock. If you have any doubt or question comment down below. 28/8/2021 · function countdown (minutes) {var seconds = 60; var mins = minutes: function tick {//This script expects an element with an ID = "counter". You can change that to what ever you want. var counter = document. getElementById ("counter"); var current_minutes = mins-1: seconds--; counter. innerHTML = current_minutes. toString + ":" + (seconds < 10? "0": "") + String (seconds); if (seconds > 0)
JavaScript HTML CSS Result Visual: Light Dark Embed snippet Prefer iframe?: No autoresizing to fit the code. Render blocking of the parent page. Srinivas Chekuri Laurel, MD Fiddle meta Private fiddle Extra. Groups Extra. Resources URL cdnjs 1. jquery-1.9.1.min.js Remove; Paste a direct CSS/JS URL ... JavaScript Project - Countdown Timer. Join DataFlair on Telegram!! 1. How to Create the HTML file. The first task to creating any webpage is adding its HTML content. In your text editor, save the new file with the name timer.html and start coding. Now, you can either use custom code or the following code to do so. Create a seconds countdown in 6 ... hours, minutes and seconds var days = Math.floor(distance / (1000 * 60 * 60 * 24)); Create a simple 10 second countdown, I would like just a Javascript/HTML timer....
Jul 26, 2020 - Error: Timeout of 2000ms exceeded. For async tests and hooks, ensure "done()" is called; if returning a Promise, ensure it resolves · How do you wait for 5 seconds in JavaScript · Timeout - Async callback was not invoked within the 5000 ms timeout specified by jest.setTimeout
How To Make A Countdown Timer In Unity In Minutes Seconds
How To Create A Countdown Timer Gif Stack Overflow
15 8 Displaying A Countdown Timer Nn 4 Ie 4 15 8 1 Problem
How To Create A Circular Countdown Timer Using Html Css Or
How To Create A Countdown Timer In Javascript Technobush
Making A Javascript Countdown Timer Stack Overflow
Countdown Timer Using Javascript Wanna Get A Countdown Timer
Create Shopify Product Countdown Timer Without App Happypoints
Solved Countdown Timer With Canvas Adobe Support Community
Countdown Timer In Sap Ui5 Sap Blogs
How To Build A Countdown Timer In Javascript
Countdown Timer In Sap Ui5 Sap Blogs
Github Johnschult Jquery Countdown360 This Is A Simple
Javascript Project How To Design Countdown Timer In
Javascript Countdown How Is Countdown Done In Javascript
Countdown Clock As Dotted Line Showing 30 Second Template
Github Shubhambathe1 React Native Countdown Timer Hooks A
Jquery Countdown Timer Amp Digital Clock Plugin Timeto Free
1 Minutes 30 Second Countdown Timer Javascript
Javascript Project How To Design Countdown Timer In
Building A Fancy Countdown Timer With Momentumslider Js
Red Countdown Timer 60s Rotary Electronic Clock Diy Kit 60s
Building A Fancy Countdown Timer With Momentumslider Js
How To Create An Animated Countdown Timer With Html Css And
How To Create An Animated Countdown Timer With Html Css And
How To Create An Animated Countdown Timer With Html Css And
See How To Build A Countdown Timer With React By Oyinda
Add Countdown Timer Or Clock Widget To Facebook Page 2021
Simple Date Time Countdown Timer Using Javascript May 2020
0 Response to "30 Javascript Countdown Timer 60 Seconds"
Post a Comment