29 Javascript Test If Object Has Property
10/1/2021 · But in objes, things are a little different. The common ways to check if a property exists in an object are: The easiest is to use the hasOwnProperty () function – var exist = OBJECT.hasOwnProperty ("PROPERTY"); Extract the keys from the object, then use the includes () function to check. Use the hasOwnProperty()method to check if an property exists in the own properties of an object. Use the inoperator to check if a property exists in both own properties and inherited properties of an object.
Check If Json Is An Array Or Object Java Code Example
var testObj = this.getView(); How can I check with DoJo (or just native JS) if testObj has callableFunction before I actually try to call callableFunction() and fail if it isn't there? I would prefer a native-DoJo solution as I need this to work on all browsers.
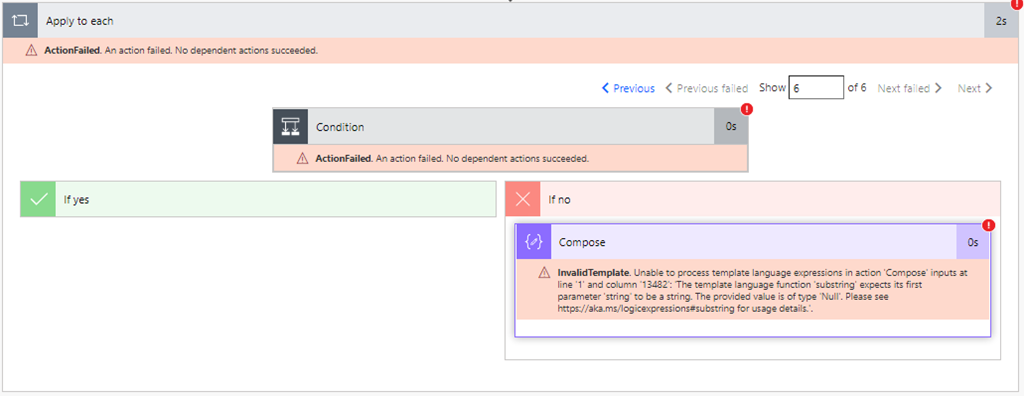
Javascript test if object has property. JavaScript provides the typeof operator to check the value data type. The operator returns a string of the value data type. For example, for an object, it will return "object". However, for arrays and null, "object" is returned, and for NaN/Infinity, "number" is returned. It is somehow difficult to check if the value is exactly a real object. The in operator is another way to check the presence of a property in an object in JavaScript. It returns true if the property exists in an object. Otherwise, it returns false. Let us use the in operator to look for the cake property in the food object: 19/4/2010 · You can use the built in Object.keys method to get a list of keys on an object and test its length. var x = {}; // some code where value of x changes and than you want to check whether it is null or some object with values if (Object.keys (x).length) { // Your code here if x has some properties } Share.
You can use the JavaScript some () method to find out if a JavaScript array contains an object. This method tests whether at least one element in the array passes the test implemented by the provided function. Here's an example that demonstrates how it works: the syntax you want is for 'var' in 'obj' the for starts the loop 'var' is the name you assign to the current property in the object obj is the object you are iterating over (looping over) Write a function that takes an object and a string and check if the object has a property with the same name as the string.
The hasOwnProperty () method in JavaScript is used to check whether the object has the specified property as its own property. This is useful for checking if the object has inherited the property rather than being it's own. Parameters: This method accepts single parameter prop which holds the name in the form of a String or a Symbol of the ... Write a function that takes an object and a string and check if the object has a property with the same name as the string. It is important to use the hasOwnProperty () method, to determine whether the object has the specified property as a direct property, and not inherited from the object's prototype chain.
Jul 03, 2020 - I agree with @josephtrieutran that the solution offered in the hints page (https://guide.freecodecamp /certifications/javascript-algorithms-and-data-structures/basic-data-structures/check-if-an-object-has-a-property/) does not accurately solve the problem because the test returns true if ... Returns true if an element with the specified value exists in the Set object; otherwise false. Note: Technically speaking, has () uses the Same-value-zero algorithm to determine whether the given element is found. Jun 02, 2021 - JavaScript has come a long way in recent years, introducing some great utility functions such as Object.keys, Object.values and many more. In this article, we’ll...
1. hasOwnProperty () method Every JavaScript object has a special method object.hasOwnProperty ('myProp') that returns a boolean indicating whether object has a property myProp. In the following example, hasOwnProperty () determines the presence of properties name and realName: The hasOwnProperty() method returns true if the specified property is a direct property of the object — even if the value is null or undefined. The method returns false if the property is inherited, or has not been declared at all. Unlike the in operator, this method does not check for the specified property in the object's prototype chain. How to Check for the Existence of Nested JavaScript Object Key To check for the existence of property nested objects you should do it step by step in order to avoid TypeError. It will be thrown if at least one of the members is null or undefined and you try to access a member. There are two ways of avoiding it.
Now we can add, modify, and remove keys from objects. But what if we just wanted to know if an object has a specific property? JavaScript provides us with two different ways to do this. One uses the hasOwnProperty() method and the other uses the in keyword. If we have an object users with a ... If you want to know if an object physically contains a property (and it is not coming from somewhere up on the prototype chain) then object.hasOwnPropertyis the way to go. All modern browsers support it. (It was missing in older versions of Safari - 2.0.1 and older - but those versions of the browser are rarely used any more.) JavaScript has no built-in.length or.isEmpty methods for objects to check if they are empty. So we have to create our own utility method or use 3rd-party libraries like jQuery or Lodash to check if an object has own properties. Here are some of the methods you can use to make sure that a given object does not have its own properties:
Double bang !! property lookup. We've all seen it, probably in something such as Modernizr for simple feature detection, the infamous !! amongst our JS. Important note before we begin this one, it doesn't actually check if an Object has a property "as such", it checks the value of the Object property. Which means if the property value is false, or the object property doesn't even ... Javascript check if object has property. There are mainly 3 ways to check if the property exists. The first way is to invoke object.hasOwnProperty(propName). The method returns true if the propName exists inside object, and false otherwise. hasOwnProperty() searches only within the own properties of the object. The second approach makes use of ... JavaScript Object hasOwnProperty () The JavaScript Object hasOwnProperty () method checks if the object has the given property as its own property. The syntax of the hasOwnProperty () method is: obj.hasOwnProperty (prop) Here, obj is an object.
The object might be any or unknown. In JavaScript, you would check for properties like that: if(typeof obj === 'object' && 'prop' in obj) { console.assert(typeof obj.prop !== 'undefined') Write a function that takes an ... check if the object has a property with the same name as the string. ... ExpressionChangedAfterItHasBeenCheckedError: Expression has changed after it was checked. Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true'. ... Which is not an example of a JavaScript statement? ... Write a function that takes an object and a string and check if the object has a property with the same name as the string. ... Use multiple conditional operators in the checkSign function to check if a number is positive, negative or zero. The function should return "positive", "negative" ...
Apr 13, 2020 - Get code examples like "how to check if object has property javascript" instantly right from your google search results with the Grepper Chrome Extension. Apr 13, 2020 - Get code examples like "if object has property" instantly right from your google search results with the Grepper Chrome Extension. Jul 29, 2020 - JavaScript is flexible. It gives you the ability to code more freely than other languages. But it comes with a cost. Sometimes you have to check if a property of an object exists or not. I have found…
Javascript object provides the hasOwnProperty native method. The method returns a boolean indicating if the object has the specified property as a first parameter. The hasOwnProperty method does not check down the prototype chain of the object. let myObj = { welcome: "Welcome to W3Docs." How can I test that mapModule has the correct exported keys, ... "that a JavaScript object matches a subset of the properties of an object." ... How do I remove a property from a JavaScript object? 3858. How do I test for an empty JavaScript object? 3230. Dec 04, 2020 - More often we are struck with the simplest problem that can be solved very easily. We know the solution for the problem would be very easy, yet it is hard to come up with the solution. It gets…
Check does an object has property in JavaScript - The hasOwnProperty() method returns a boolean whether the object has the specified property as its own property to be indicated. A simple Javascript object has commonly the hasOwnProperty pure method. as well as The hasOwnProperty method data returns a boolean value like as a true or false indicating whether the object has the particularized characteristic of an object as first parameter. var person = { "first_name": "Bob", "last_name": "Dylan" }; person.hasOwnProperty('first_name'); //returns true person.hasOwnProperty('age'); //returns false
Apr 24, 2020 - The right way to check if an object has a property in JavaScript ... You might think that this could easily be done by using the in operator. const user = { name: 'Olivier', }'name' in user // true · The problem with the in operator is that it checks if the property is in the specified object ... A Javascript object has normally the hasOwnProperty native method. The hasOwnProperty method returns a boolean indicating whether the object has the specified property as first parameter. Unlike the in operator, this method does not check down the object's prototype chain. @StanE Arrays are definitely objects. Not sure why you think objects can't have a length property nor methods like push, Object.create(Array.prototype) is a trivial counterexample of a non-array object which has these. What makes arrays special is that they are exotic objects with a custom [[DefineOwnProperty]] essential internal method, but ...
So, when you pass the key "programmer" to the object, it returns the matching value that is 2. Aside from looking up the value, you may also wish to check whether a given key exists in the object. The object may have only unique keys, and you might want to check if it already exists before adding one. Find Object In Array With Certain Property Value In JavaScript July 7, 2020 by Andreas Wik If you have an array of objects and want to extract a single object with a certain property value, e.g. id should be 12811, then find () has got you covered. How do I test for an empty Javascript object from JSON? Is there an easy way to check if an object has no properties, in Javascript? Or in other words, an easy way to check if a map/associative array is empty? For example, let's say you had the following: var nothingHere = {}; var somethingHere = {foo: "bar"};
9/8/2019 · Since JavaScript allows you to create dynamic objects, you have to be careful and check if an object’s property actually exists. There are at least 2 ways to do this. hasOwnProperty. hasOwnProperty is a function built into all objects. if (obj.hasOwnProperty('prop')) {// do something} The easiest way to check if an object property exists but is undefined in JavaScript is to make use of the Object.prototype.hasOwnProperty method which will let you find properties in an object. The problem of checking if an undefined property exists in an object comes from when you reference a property that does not exist then it will return ...
Learn About Properties In Javascript
Javascript Check If Object Has Property Es6
Objects In Javascript Geeksforgeeks
What Is Property In Hasownproperty In Javascript Stack
How To Check If A Javascript Array Is Empty Or Not With Length
3 Ways To Access Object Properties In Javascript
Javascript Typeof Understanding Type Checking In Javascript
How Do I Check If An Object Has A Specific Property In
Typeerror Cannot Read Property Textcontent Of Null When You
Javascript Global Object Contentful
Object Prototypes Learn Web Development Mdn
How To Check If Array Includes A Value In Javascript
Waiting For An Object To Have A Specific Property Value
Debugging In Visual Studio Code
Github Jonschlinkert Get Value Use Property Paths A B C
Telmo Goncalves On Twitter Javascript Tip Use
Power Automate How Do We Check If A Property Exists In The
Test Script Examples Postman Learning Center
How To Check If Object Is Empty In Javascript Samanthaming Com
Object Values In Javascript The Complete Guide
How To Check For An Object In Javascript Object Null Check
5 Ways To Check If Property Exists In Javascript Object
Nested Object Equals Check Js Code Example
Javascript Object Properties Javascript The Freecodecamp
Power Automate How Do We Check If A Property Exists In The
Everything About Javascript Objects By Deepak Gupta
How To Check If Json Object Contains A Specified Key
Details Of The Object Model Javascript Mdn
0 Response to "29 Javascript Test If Object Has Property"
Post a Comment