32 Add Style To Class Javascript
The class name attribute can be used by CSS and JavaScript to perform certain tasks for elements with the specified class name. Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. Syntax: element.className += "newClass"; Like we saw in the introduction, we have two ways to alter the style of an element using JavaScript. One way is by setting a CSS property directly on the element. The other way is by adding or removing class values from an element which may result in certain style rules getting applied or ignored.
Ability To Add A Class To Style A Navigation Menu Item
The style property isn't very useful when it comes to getting style information from the elements, because it only returns the style rules set in the element's style attribute not those that come from elsewhere, such as style rules in the embedded style sheets, or external style sheets.. To get the values of all CSS properties that are actually used to render an element you can use the window ...
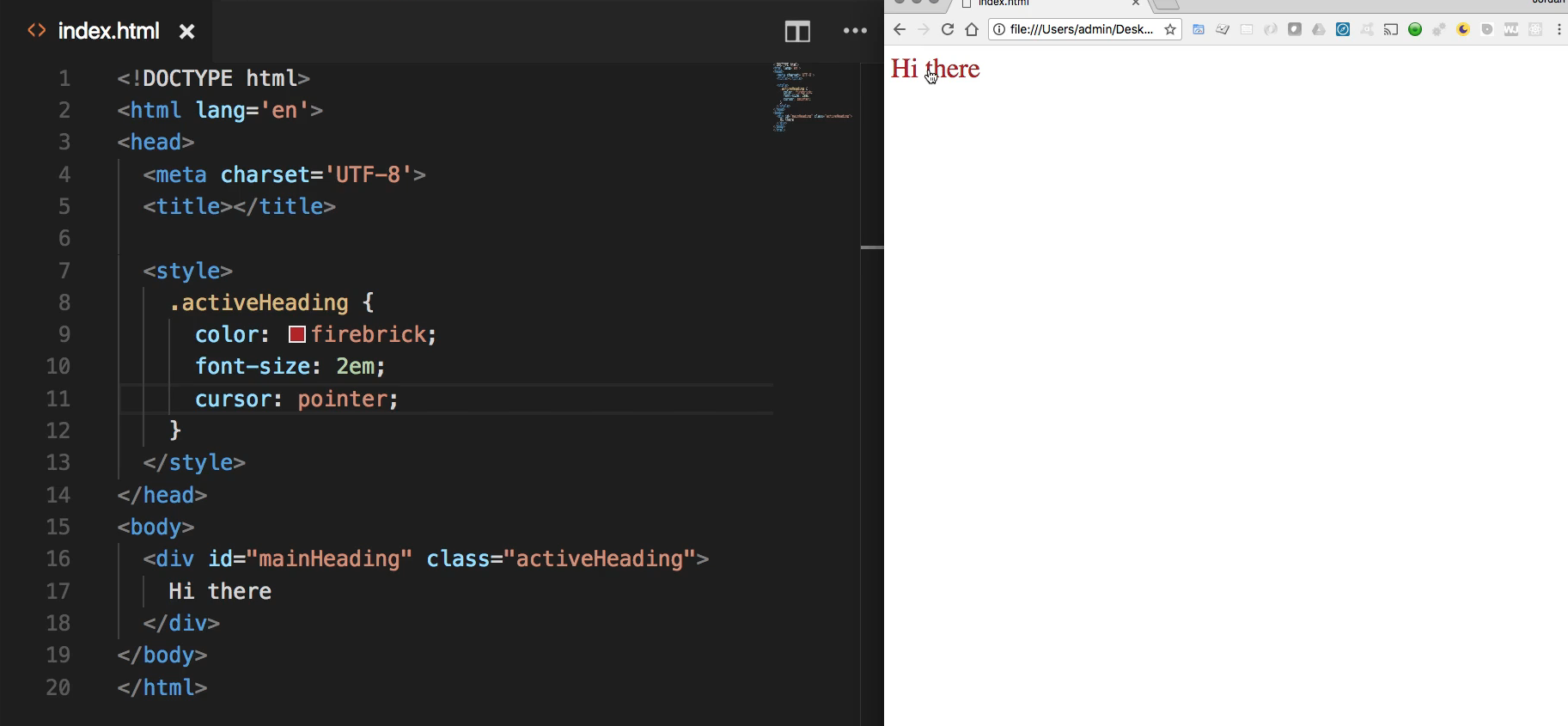
Add style to class javascript. Using The class Attribute The class attribute is often used to point to a class name in a style sheet. It can also be used by a JavaScript to access and manipulate elements with the specific class name. In the following example we have three <div> elements with a class attribute with the value of "city". Animating a Lightbox with CSS & Javascript is a perfect example where you need to add CSS to a stylesheet dynamically. Creating a New Stylesheet To Add CSS Rules When dynamically creating CSS rules it is alays better to create a new stylesheet, and adding rules to it. 29/3/2020 · By using the JavaScript DOM methods, you can add the inline styles or global styles to the element. Inline styles. To add inline styles to an element, you follow these steps: First, select the element by using DOM methods such as document.querySelector(). The selected element has the style property that allows you to set the various styles to the element. Then, set the values of the properties of the style …
Both the add() and remove() methods discussed above can be used for adding and removing multiple classes at a time. The below statement adds three classes — thorn , bud and sepal — to the <div/> element. jQuery add style to the HTML element using css () method. There are two parameters you have to use with this method. The first parameter requires the jQuery selector to specify the id or class of the HTML element and access the element. The second parameter requires property name with its value. 7/2/2010 · You can change the class on mydiv in javascript like this: document.getElementById ('mydiv').className = 'newclass'; After the DOM manipulation you will be left with: <div id="mydiv" class="newclass">text</div>. If you want to add a new css class without removing the old one, you can append …
javascript - changing a class' style. Ask Question Asked 9 years, ... For better coding style add another class to the elements with the code above and then use CSS to change the color of all elements like this: ... to change the class of the element and then just define the style for that new class in your CSS file. Share. Improve this answer ... 27/2/2019 · Create a style element using the following syntax Syntax: document.createElement('style'); Apply the CSS styles. Append this style element to the head element. Syntax: document.getElementsByTagName("head")[0].appendChild(styleElement); Example 1: This example changes the <h1> color to green by creating the style element using JavaScript. The example of using the .className property is given as follows.. Example - Adding the class name. In this example, we are using the .className property for adding the "para" class to the paragraph element having id "p1".We are applying the CSS to the corresponding paragraph using the class name "para".. We have to click the given HTML button "Add Class" to see the effect.
Using JavaScript - style property In pure JavaScript, you can use the style property to inline set the style of an element. Please refer to this article to get the list of JavaScript equivalents of common CSS properties. Adding Multiple Inline Styles The DOM style property is the simplest way to set and get CSS styles from an element in JavaScript. Usually, when you want to add multiple CSS properties to an element, you have to set them individually as shown below: Step 3) Add JavaScript: Get the <div> element with id="myDIV" and add the "mystyle" class to it: Example. function myFunction() {. var element = document.getElementById("myDIV"); element.classList.add("mystyle"); Try it Yourself ». Tip:Also see How To Toggle A Class. Tip:Also see How To Remove A Class.
JavaScript HTMLElement.style - To change style of HTML Element programmatically, assign custom style value HTMLElement.style. Example : document.getElementById('idv').style = 'color:#f00;'. Syntax and Try Online Examples are provided to get hands on. 2. Inline Style in React Component. One of the easiest ways to add style to the React Components is to add it inline to the elements in the component. We can add style directly to the element ... 26/11/2020 · The classList property also used to add a class in JavaScript but it never overwrites existing classes and adds a new class to the selected elements class list. // Add new class to existing classes var p = document.querySelector("p"); p.classList.add("test"); After adding new classes to the elements, we select the new class(test) and then apply CSS properties to it, with the help of style property in JavaScript. // Select all elements with class …
JavaScript can modify both classes and style properties. We should always prefer CSS classes to style. The latter should only be used if classes "can't handle it". ... adding a background icon - describe that in CSS and then add the class (JavaScript can do that). That's more flexible and easier to support. Another way to alter the style of an element is by changing its class attribute. class is a reserved word in JavaScript, so in order to access the element's class, you use element.className. You can append strings to className if you want to add a class to an element, or you could just overwrite className and assign it a whole new class. In what situations would adding a new stylesheet be better than manipulating classes with JS? Mozilla says: "Add a stylesheet rule to the document (may be better practice, however, * to dynamically change classes, so style information can be kept in * genuine stylesheets (and avoid adding extra elements to the DOM))"
Copy. With each click, classList.toggle () will add the CSS class if it does not exist in the classList array and return true. If the CSS class exists, the method will remove the class and return false. index.js. const anotherClass = myText.classList.toggle('newSize'); console.log(anotherClass); Copy. Therefore, to add specific styles to an element without altering other style values, it is generally preferable to set individual properties on the CSSStyleDeclaration object. For example, element.style.backgroundColor = "red". A style declaration is reset by setting it to null or an empty string, e.g., elt.style.color = null. Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
If the class does not exist, it is added. Toggling can be performed with the toggle method. This methods accepts 2 parameters: The CSS class to add or remove. An optional force argument — which can either be true or false. When set to true, then add the class — which becomes similar to the add method. When set to false, then remove the ... The easiest way to set an element's style with JavaScript is using the style property. JavaScript uses camel case instead of a dash for property names (you can view a full list of properties on MDN), but it's pretty straightforward otherwise: The purpose is simply that I want to add some css/html dinamically from javascript. It's kind of optional code that I need to add "onload" only under some circumstances. Have a go with this class. Tested on IE, Moz and Opera. YMMV. /** An object which encapsulates a dynamically created, modifiable stylesheet. */ function CSSStyleSheet() {/**
16/8/2019 · One of the ways to add style to your element is by directly defining the properties in a style attribute. The syntax to add a style to an element is as follows: <element style = “...”></element> With the help of class attribute Adding a CSS class to an element using JavaScript. Now, let's add the CSS class "newClass" to the DIV element "intro". For the purpose of this example, I have added a delay using the setTimeout() method so that you can see the style changing: In JavaScript, the standard way of selecting an element is to use the document.getElementById("Id"). Of course, it is possible to obtain elements in other ways, as well, and in some circumstances, use this. For replacing all the existing classes with a single or more classes, you should set the className attribute, as follows:
The easiest and straightforward way to change CSS styles of an element with JavaScript is by using the DOM style property. All you need to do is just fetch the element from DOM and change its inline styles: add (class) — applies a new class to the node. remove (class) — removes a class from the node. toggle (class) — removes or adds a class if it's applied or not applied respectively. We can ... Attributes may consist of name/value pairs, and a few of the most common attributes are class and style. In this tutorial, we learned how to access, modify, and remove attributes on an HTML element in the DOM using plain JavaScript. We also learned how to add, remove, toggle, and replace CSS classes on an element, and how to edit inline CSS styles.
Change Css Style Of A Class With Javascript But Not In The
Lodop Before Css Style After Before And After Adding
Setting Css Styles Using Javascript Kirupa
Javascript Project How To Create A To Do List Using
Scrimba Dom Modifying Styles Classname And Classlist
How To Add And Remove Classes In Vanilla Javascript
Javascript Adding A Class Name To The Element Geeksforgeeks
Javascript Style Attribute How To Implement Css Styles In
How To Add Multiple Class To Div Using Jquery
Getting Width Amp Height Of An Element In Javascript
Styling Web Components Pluralsight
Javascript And Jquery Develop Paper
How To Modify Attributes Classes And Styles In The Dom
Javascript Project How To Create A To Do List Using
Object Oriented Javascript For Beginners Learn Web
Confused About Style Backgroundcolor For The Correct Class
Making Sense Of Es6 Class Confusion Toptal
Jsreport Javascript Based Reporting Platform
Add Remove And Toggle Css Class In Javascript Without Jquery
Get Started With Viewing And Changing Css Microsoft Edge
Javascript How To Modify Html Of All Elements Of A Class Code
Adding And Removing Css Classes With Jquery Jquery Tutorials For Beginners
Add Remove And Toggle Css Class In Javascript Without Jquery
View And Change Css Chrome Developers
Javascript Add Class To Element Dynamically Code Example
Javascript Style Attribute How To Implement Css Styles In
How To Modify Attributes Classes And Styles In The Dom
Inline Css Explained Learn To Add Inline Css Style
0 Response to "32 Add Style To Class Javascript"
Post a Comment